introduce
Axios is a promise based HTTP library that can be used in browsers and node.js. It is also the library recommended by the author of vue.js
Realization function
- Create from browser XMLHttpRequests
- Create from node.js http request
- Support Promise API
- Intercept requests and responses
- Transform request data and response data
- Cancellation request
- Automatically convert JSON data
- Client support defense XSRF
Browser support
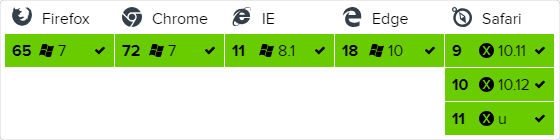
Browser compatibility.png
Functions to be configured in the project
Create an axios instance, and implement the following functions through unified configuration
-
Configuration of axios itself
- Configure URL prefix
- Timeout time
- Cross domain cookie
- ...
- HTTPRequest interceptor
- Call load information
- Add authentication Token to request header
- Processing of GET request cache for IE browser
- ... other business codes
- HTTP response interceptor
- Hide load information
- Unified processing for return value
- ... other business codes
The specific package code is as follows:
Some of the business codes have been removed, and the implementation ideas are for reference only
import axios from 'axios' import store from '@/store' import { Loading, Message, MessageBox } from 'element-ui' // Load top progress bar import NProgress from 'nprogress' NProgress.configure({ showSpinner: false }) // Close nprogress and loading function closeLoading () { NProgress.done() loadingService.close() } // Cross domain request, allow cookie s to be saved axios.defaults.withCredentials = true // Create axios instance, configure baseURL, timeout const service = axios.create({ baseURL: process.env.VUE_APP_API_BASEURL, // The base URL of the api obtained from the environment process according to the running environment timeout: 50000 // Request timeout }) let loadingService /** * request Interceptor * Set the cookie in the request header before sending the request */ service.interceptors.request.use(config => { NProgress.start() loadingService = Loading.service({ lock: true, text: 'Loading', spinner: 'el-icon-loading', background: 'rgba(0, 0, 0, 0.1)' }) let token = store.getters.token if (token && token.access_token && Date.now() < token.expires_in) { // Let each request carry authentication information if (!config.headers['Authorization']) { config.headers['Authorization'] = `Bearer ${token.access_token}` } } // Judge if get request increases random number to prevent IE browser from caching api request if (config.method === 'get') { if (config.params && Object.keys(config.params).length > 0) { config.params['cache'] = new Date().getTime() } else { config = Object.assign({}, config, { params: { cache: new Date().getTime() } }) } } return config }, error => { console.error(error) Promise.reject(error) }) /** * respone Interceptor * Mark the request status through the response custom code, and handle the corresponding problems */ service.interceptors.response.use( response => { closeLoading() const { status }= response.data // Status code: - 1: not logged in / 1: request succeeded / - 2: request failed / - 3: no permission / - 4: system error if (status === 1) { return response.data.data } else { let errorMessage = response.data.message switch ( case -1: // Clear locally saved Token // Jump to login page break case -2: // Prompt error message break default: // Prompt error message } return Promise.reject(new Error(errorMessage)) } }, // Server error error => { console. console.error('err' + error) // for debug return Promise.reject(error) } ) export default service
Calling process
Note: the configuration passed in in the instance call will override the previous configuration on the constructor axios
import request from '@/XXX/request.js' /* User login */ export function loginByUsername (reqParams) { return request({ // baseURL: '/api', // headers: { }, url: '/auth/oauth/token', method: 'post', params: reqParams }) }
If you have other questions or better ideas, you are welcome to discuss them together.