This article was last updated on February 17, 2022, and has not been updated for more than 11 days. If the article content or picture resources fail, please leave a message and feedback. I will deal with it in time. Thank you!
Pointer variable as function parameter
- The parameters of a function can be not only integer, floating point, character and other data, but also pointer type.
- Its function is to transfer the address of a variable to another function.
- For example: void swap(int *a,int *b)
#include <stdio.h> void main() { void swap(int *x, int *y); int a,b; int *a1 = &a; int *b1 = b; printf("Please enter a,b Value of:\n"); scanf_s("%d %d", &a, &b); if (a < b) { swap(a1, b1); } printf("max = %d, min=%d\n", a, b); } void swap(int *x, int *y) { int p; p = *x; *x = *y; *y = p; }
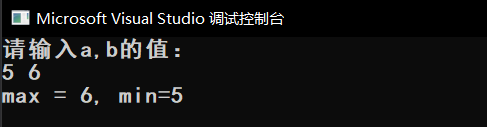
- be careful: Function call can (and can only) get a return value (i.e. function value), while using pointer variables as parameters can get multiple changed values. It is difficult to do this without pointer variables.
- If you want to get n values to change through function call
- 1. Set n variables in the main function and point to them with n pointer variables;
- 2. Design a function with n pointer parameters. Change the values of these n parameters in this function;
- 3. call this function in the main function, and call these n pointer variables as arguments in the call, and transmit their addresses to the formal parameters of the function.
- 4. In the process of executing the function, change the values of the n variables they point to through the formal parameter pointer variables;
- 5. These variables that change the value can be used in the main function.
- example
Input three integers a, B and C, and output them in the order from large to small. Realized by function.
#include <stdio.h> void main() { void exchange(int *x, int *y,int *z); int a,b,c; int *a1 = &a; int *b1 = &b; int *c1 = &c; printf("Please enter a,b,c Value of:\n"); scanf_s("%d %d %d", &a, &b, &c); exchange(a1,b1,c1); printf("%d, %d, %d\n", a,b,c); } void swap(int *x, int *y) { int p; p = *x; *x = *y; *y = p; } void exchange(int *x, int *y, int *z) { void swap(int *x, int *y); if (*x < *y) { swap(x, y); } if (*x < *z) { swap(x, z); } if (*y < *z) { swap(y, z); } }
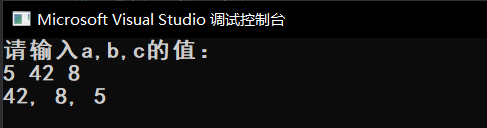
Pointer to array element
- A variable has an address, an array contains several elements, and each array element has a corresponding address.
- Pointer variables can point to array elements (put the address of an element into a pointer variable).
- The pointer to the array element is the address of the array element.
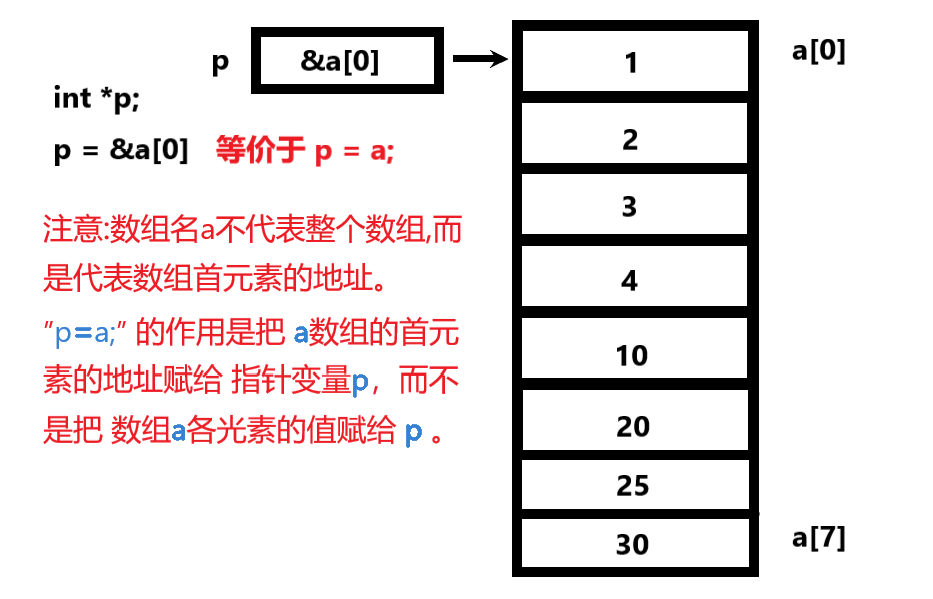
Pointer operation when referencing array elements
- When the pointer points to an array element, the following operations are allowed:
- One plus an integer (with + or + =), for example: p + 1
- One minus one integer (use - or - =), for example: p - 1
- Subtract one or two pointers, such as p1-p2 (only meaningful if both p1 and p2 point to elements in the same array)
- If the pointer variable p points to an element in the array
- p+1 points to the next element in the same array;
- p-1 points to the previous element in the same array.
- If the initial value of p is & A [0], then
- p+i and a+i are the addresses of the array element a[i],
- In other words, they point to the elements of the a array with sequence number i.
- for example
#include <stdio.h> void main() { int a[5] = {1,2,3,4,5}; int *a1 = &a[0]; // Or int *a1 = a printf("%d, %d\n", *a1, a1); a1 = a1 + 2; printf("%d, %d\n",*a1, a1); }
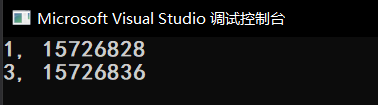
- When p points to the first element of array a, there are four kinds of address expressions for array element a[i]:
- &a[i]
- a + i
- p + i
- &p[i]
- When p points to the first element of array a, there are four kinds of value expressions of array element a[i]:
- a[i]
- *(a + i)
- *(p + i)
- p[i]
- a and p are essentially different:
- A is a pointer constant whose value is immutable;
- p is a pointer variable whose value is variable.
- Therefore, a + + and a = p are illegal expressions;
- p + + and p = a are legal expressions.
- If both pointers p1 and p2 point to the same array
- p2 - p1 is used to calculate the number of elements between the element indicated by p2 and the element indicated by p1.
- Subtracting two pointer variables means that the difference between the two addresses is divided by the number of bytes occupied by each array element.
- example
#include <stdio.h> void main() { int a[10] = {1,2,3,4,5,6,7,8,9,10}; int *p1 = &a[0]; int *p2 = &a[6]; printf("p1 Address:%d\n",p1); printf("p2 Address:%d\n",p2); printf("%d\n", p2 - p1); printf("%d\n",*p2 - *p1); // int is 4 bytes }
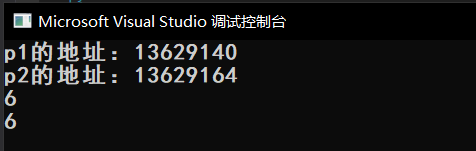
- *++p. Equivalent to * (+ + p), first make p+1 point to the next group element, and then take * P as the value of this expression.
- *P + +, equivalent to * (P + +), take P as the value of this expression, and then make p+1.
- (* p) + +, take the element value pointed to by p as the value of this expression, and then add 1 to the element value.
- ++(* p), add 1 to the element value pointed to by p as the value of this expression.
#include <stdio.h> void main() { int a[10] = {1,2,3,4,5,6,7,8,9,10}; int *p = &a[0]; printf("%d\n",*p); printf("%d\n",*++p); printf("%d\n",*p++); printf("%d\n",(*p)++); printf("%d\n",++(*p)); }
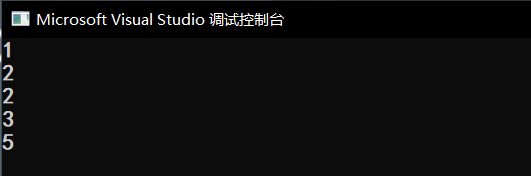
Reference array elements through pointers
- To reference an array element, you can use the following two methods:
- 1. Subscript method, for example: a[i]
- 2. Pointer method, for example: * (a+i) or * (p+i) Where a is the array name, P is the pointer variable pointing to the array element, and its initial value p = a
- example
There is an integer array a with 10 elements. It is required to output all the elements in the array.
#include <stdio.h> void main() { int a[10] = {1,2,3,4,5,6,7,8,9,10}; int *p; // subscripts for (int i = 0; i < 10; i++) { printf("%d ", a[i]); } printf("\n"); // Calculate the address of array element by array name for (int i = 0; i < 10; i++) { printf("%d ", *(a+i)); } printf("\n"); // Pointer method for (p=a; p <a+10; p++) { printf("%d ", *p); } printf("\n"); }
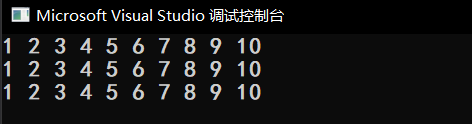
- Comparison of three methods:
- The execution efficiency of methods (1) and (2) is the same
- The C compilation system converts a[i] into * (a+i), that is, calculate the element address first.
- Therefore, using the methods (1) and (2) to find array elements takes more time.
- Method (3) is faster than methods (1) and (2)
- Use pointer variables to point directly to elements without recalculating the address every time.
- Changing the address value regularly, such as p + + can greatly improve the execution efficiency.
- The subscript method is more intuitive and can directly know the number of elements.
- Using address method or pointer variable method is not intuitive, and it is difficult to quickly judge which element is currently being processed.
- The execution efficiency of methods (1) and (2) is the same