C language is a process oriented and abstract general programming language, which is widely used in the bottom development. C language can compile and process low-level memory in a simple way. C language is an efficient programming language that produces only a small amount of machine language and can run without any running environment support. Although C language provides many low-level processing functions, it still maintains the cross platform characteristics. C language programs written in a standard specification can be compiled on many computer platforms including operating platforms such as embedded processors and supercomputers.
The latest C language standard is C18
The first C language program: Hello world!
#include <stdio.h> int main() { printf("Hello World!"); return 0; }
Output:
Hello World!
title: Supplementary knowledge collapse: open xxxx.c: Source file; xxxx.h: Header file;
Analyze the structure of simple C language
- Write out the main function (main function)
int main() { return 0; }
So what is the main function?
The main function is: main(). The structure is as above. Note: a C program has and has only one main function. Any C program always starts from the main function, and a pair of parentheses behind the main function cannot be omitted. C language is executed from the first line of the main function. Equivalent to the entry to execute C language.
-
Function name, function body, return type of function
-
Print function: printf
printf("Hello World!");
printf: library function to print information on the screen. Because this function is not in this program, but in the referenced library function, you need to refer to the header file < stdio h> , so you can use the functions in the library functions.
Full code:
#include <stdio.h> int main() { printf("Hello World!"); return 0; }
- function!
title: Supplementary knowledge `\n`: Perform line feed operation; ```C printf("Hello World!\n"); ```
C language data type
Data type is used to describe the type of data and determine the interpretation method of data, so that computers and programmers will not have ambiguity
explain | character | Short | integer | Long integer | Single precision floating point | Double precision floating point | No type |
---|---|---|---|---|---|---|---|
data type | char | short | int | long | float | double | void |
char ch = 'a'; Data type variable character Meaning: give character type variables ch Assign as character a.
Taking characters as an example, the assignment structure of integers is the same, and the space allocated by different data types is different. To save memory space.
title: Supplementary knowledge 1. Print character and number: ```C printf("Print character\n"); printf("%d\n",100);//Print an integer 100,% d: means to print an integer ``` 2. `sizeof`: Keywords -- operators -- calculate the space occupied by types or variables ```C printf("%d\n",sizeof(char));//Print out the result of calculating the size of char ```
Print out the size of each data type:
#include <stdio.h> int main() { printf("%d\n",sizeof(char)); printf("%d\n",sizeof(short)); printf("%d\n",sizeof(int)); printf("%d\n",sizeof(long)); printf("%d\n",sizeof(long long)); printf("%d\n",sizeof(float)); printf("%d\n",sizeof(double)); }
title: Supplementary knowledge 1. Unit in computer: **position**: The smallest data unit of the computer. Each bit can only be 0 or 1; **byte**(Byte): 8 One binary constitutes one byte, which is the basic unit of storage space; **KB**: 1KB Represents 1024 bytes; wait
Constant and Variable
Data type is used to create variables
Constants: fixed data
Variable: the amount that can be changed
variable
- Create a variable:
#include <stdio.h> int main() { //Create a variable //Name of type variable = 0; //Name of type variable; //Both methods can create variables. The first one is recommended; int age = 20; double weight = 75.3; age = age + 1; printf("%d\n",age); printf("%if\n",weight); return 0; }
Operation results:
title: Supplementary knowledge - %d: Print integer - %f: float - %lf: double - %c: character
Local and global variables
#include <stdio.h> //Global variable: {} externally defined int b = 100 int a = 5 int main() { //Local variable: {} internally defined int a = 10; pintf("%d\n",a) return 0; }
When the names of global variables and local variables conflict, local variables take precedence;
Operation result of the above code: 10;
Write a code to find the sum of two integers
#include <stdio.h> int main() { int a = 0; int b = 0; int sum = 0; scanf("%d %d",&a,&b); sum = a+b; printf("sum = %d\n",sum); return 0; }
Operation results:
title: Supplementary knowledge: scanf function - Input function:`scanf` ```C scanf("%d %d",&a,&b); ``` 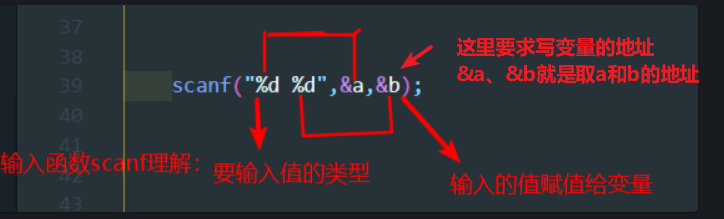
Scope of variable
#include <stdio.h> int main() { int a = 10; printf("%d\n",a) return 0; //The scope of a is within this {} } //Operation result: 10
Scope: Generally speaking, where it works is its scope
#include <stdio.h> int main() { { int a = 10; //The scope of a is within this {}; } printf("%d\n",a) return 0; } //Operation result: an error is reported and there is no definition a
Local variable scope: it is the local scope where it is located
Global variable scope: the whole project
Variable life cycle
Life cycle: refers to the time period between the creation and destruction of variables;
The life cycle of local variables: the life of local variables starts and the life of local variables ends;
Life cycle of global variable: the life cycle of the program;
constant
- Literal constant
- const modified constant
- #Identifier constant defined by define
- enumeration constant
- Literal constant
#include <stdio.h> int main() { //Literal constant; 10; 12; 3.12; "a"; return 0; }
- const modified constant
#include <stdio.h> int main() { int a = 10;//variable const int a = 10;//const is added in front to specify that this variable cannot be changed, so it is called a constant variable, which is still a variable in essence; return 0; }
- #Identifier constant defined by define
#include <stdio.h> #define MAX 10000 int main() { int a = MAX; printf("%d\n",a); return 0; }
Operation result: 10000
- Enumeration constants: constants that can be enumerated one by one
#include <stdio.h> enum Sex { //The possible values of variables of this enumeration type in the future; MALE; FEMALE; SECRET; } int main() { enum Sex s = MALE;//The value can only be one of Mal, FEMALE and SECRET; return 0 ; }
String + transfer character + comment
character string
String: a string of characters -- a string of characters enclosed by ""
"character string"
#include <stdio.h> int main() { char arr[] = "hello" return 0; }
The mode view array is as follows:
A character "/ 0" is hidden behind the string: hello;
A character of / 0 is hidden at the end of the string;
/0 is the end flag of the string;
title: Supplementary knowledge: array - An array is a set of elements of the same type - Create an array and initialize ```C char arr[] = "hello"; //Create a character array, [] does not indicate the size of the array, and the size is determined according to the subsequent assignment. Assign the value "hello". //It is equivalent to creating an array of ["h","e","l","l","l","o","/0"]; ```
What is enclosed by double quotation marks' 'is called a string of characters, and what is enclosed by single quotation marks'' is called a character;
contrast:
#include <stdio.h> int main() { char arr1[] = "abc"; char arr2[] = {'a','b','c'}; printf("%s\n",arr1); printf("%s\n",arr2); return 0; }
Monitoring: arr1 and arr2
You can see that arr2 has no / 0,
View print results
Because arr1 has / 0 as the end flag, the program will know the end when it reaches / 0, while arr2 has no end flag, so the program will not know whether it has ended and will continue to print the rest.
Modify arr2
char arr2[] = {'a','b','c','/0'};//Add end flag manually / 0
That's it.
title: Supplementary knowledge: strlen function - strlen Function: find the length of a string Note that the header file needs to be referenced:`#include <string.h>`
Description / 0 is only used as an end flag
#include <stdio.h> #include <string.h> int main() { char arr1[] = "abc"; char arr2[] = {'a','b','c'}; printf("%s\n",strlen(arr1)); printf("%s\n",strlen(arr2)); return 0; }
Escape character
The Character Set assigns a unique number to each character, which we might as well call encoded value. In C language, a character can be represented not only by its entity (that is, the real character), but also by coded value. This way of using coded values to represent characters indirectly is called Escape Character;
The escape character starts with \ or \ x, starts with \ indicates the encoded value followed by octal form, and starts with \ x indicates the encoded value followed by hexadecimal form. For escape characters, only octal or hexadecimal can be used.
The escape character starts with \ or \ x, starts with \ indicates the encoded value followed by octal form, and starts with \ x indicates the encoded value followed by hexadecimal form. For escape characters, only octal or hexadecimal can be used.
The octal forms of ASCII codes corresponding to characters 1, 2, 3, a, b and c are 61, 62, 63, 141, 142 and 143 respectively, and the hexadecimal forms are 31, 32, 33, 61, 62 and 63 respectively. The following example demonstrates the usage of escape characters:
char a = '\61'; //Character 1 char b = '\141'; //Character a char c = '\x31'; //Character 1 char d = '\x61'; //Character a char *str1 = "\x31\x32\x33\x61\x62\x63"; //String "123abc" char *str2 = "\61\62\63\141\142\143"; //String "123abc" char *str3 = "The string is: \61\62\63\x61\x62\x63" //Mix octal and hexadecimal forms
Escape characters can be used for either a single character or a string, and both octal and hexadecimal forms can be used in a string.
A complete example:
#include <stdio.h> int main() { puts("\x68\164\164\x70://c.biancheng.\x6e\145\x74"); return 0; }
Operation results:
http://c.biancheng.net
The escape character is originally intended for ASCII encoding, so its value range is limited:
- The escape character in octal form can be followed by three digits at most, that is \ ddd, and the maximum value is \ 177;
- The escape character in hexadecimal form can be followed by two numbers at most, namely \ xdd, and the maximum value is \ x7f.
The behavior of escape characters beyond the range is undefined. Some compilers will directly output the encoded value, and some compilers will report an error.
For ASCII coding, the characters in the range of 0 ~ 31 (decimal system) are control characters. They are invisible and cannot be displayed on the display or even input from the keyboard. They can only be expressed in the form of escape characters. However, the direct use of ASCII code is inconvenient and difficult to understand. Therefore, for common control characters, C language defines the abbreviation method. The complete list is as follows:
Escape character | significance | ASCII value (decimal) |
---|---|---|
\a | Bell (BEL) | 007 |
\b | Backspace (BS) to move the current position to the previous column | 008 |
\f | Page feed (FF) moves the current position to the beginning of the next page | 012 |
\n | Line feed (LF) moves the current position to the beginning of the next line | 010 |
\r | Press enter (CR) to move the current position to the beginning of the line | 013 |
\t | Horizontal tabulation (HT) | 009 |
\v | Vertical tabulation (VT) | 011 |
' | Single quotation mark | 039 |
" | Double quotation mark | 034 |
\ | Backslash | 092 |
\n and \ t are the two most commonly used escape characters:
- \n is used for line feed, so that the text is output from the beginning of the next line. It has been used many times in the previous chapters;
- \t is used to occupy space, which is generally equivalent to four spaces or the function of tab key.
Single quotation marks, double quotation marks and backslashes are special characters that cannot be directly expressed:
- Single quotation marks are the beginning and end of character types, which should be represented by \ ', that is' \';
- Double quotation marks are the beginning and end of the string, which should be represented by \ ", i.e." abc\"123";
- The backslash is the beginning of the escape character, which should be represented by \, that is' \ \ ', or "abc\123".
Examples of escape characters:
#include <stdio.h> int main(){ puts("C\tC++\tJava\n\"C\" first appeared!"); return 0; }
Operation results:
C C++ Java
"C" first appeared!
notes
P16 start;
Select statement
give an example:
#include <stdio.h> int main() { int input = 0; printf("Join the glorious evolution!"); printf("Accession: 1; Do not join: 0"\n); scanf("%d",&input); if (input == 1) { printf("Mechanical soaring"); } else { printf("Life is short"); } return 0; }
In the above, we have to choose. Different choices have different results
Circular statement
while Loop
int line = 0 while(line <30000) { line++; }
When line equals 30000, it will jump out of the loop.
function
A function is a piece of reusable code used to complete a function independently. It can receive or not receive the data passed by the user. Functions receiving user data need to specify parameters when defining. Functions that do not receive user data do not need to be specified. According to this point, functions can be divided into parametric functions and nonparametric functions.
The process of encapsulating code segments into functions is called function definition.
int Add(int x,int y) { int z = 0; z = x+y; return z; }//Defines a function int main() { int num1 = 0; int num2 = 0; scanf("%d%d",&num1,&num2); int sum = Add(num1,num2);//A function is called; printf("%d\n",sum); return 0; }
Function structure:
If the function does not receive the data passed by the user, it can be defined without parameters. As follows:
dataType functionName(){ //body }
- dataType is a return value type. It can be any data type in C language, such as int, float, char, etc.
- functionName is the function name, which is a kind of identifier. The naming rules are the same as those of the identifier. The parentheses () after the function name cannot be less.
- Body is the function body. It is the code to be executed by the function and the main part of the function. Even if there is only one statement, the function body should be surrounded by {}.
- If there is a return value, use the return statement in the function body to return. The type of data returned should be the same as dataType.
For example, define a function to calculate the result from 1 to 100:
1. int sum(){ 2. int i, sum=0; 3. for(i=1; i<=100; i++){ 4. sum+=i; 5. } 6. return sum; 7. }
The accumulation result is saved in the variable sum and finally returned through the return statement. Sum is of type int, and the return value is also of type int. they correspond to each other one by one.
Return is a keyword in C language. It can only be used in functions to return processing results.
Complete the above code:
1. #include <stdio.h> 3. int sum(){ 4. int i, sum=0; 5. for(i=1; i<=100; i++){ 6. sum+=i; 7. } 8. return sum; 9. } 11. int main(){ 12. int a = sum(); 13. printf("The sum is %d\n", a); 14. return 0; 15. }
Operation results:
The sum is 5050
Functions cannot be nested. Main is also a function definition, so you should put sum outside main. Functions must be defined before use, so sum should be placed in front of main.
Note: main is a function definition, not a function call. When the executable file is loaded into memory, the system starts to execute from the main function, that is, the system will call the main function we defined.
Function with no return value
Some functions do not need a return value, or the return value type is uncertain (very rare), so it can be represented by void, for example:
1. void hello(){ 2. printf ("Hello,world \n"); 3. //No return statement is required if there is no return value 4. }
void is a keyword in C language, which means "empty type" or "no type". In most cases, it means that there is no return statement.
Definition of parametric function in C language
If the function needs to receive the data passed by the user, it must be defined with parameters. As follows:
dataType functionName( dataType1 param1, dataType2 param2 ... ){ //body }
dataType1 param1, dataType2 param2 ... Is a list of parameters. A function can have only one parameter or multiple parameters separated by. The parameter is also a variable in essence, and the type and name should be specified when defining. Compared with the definition of nonparametric function, the definition of parametric function is only one more parameter list.
The data is passed to the inside of the function through parameters for processing. After processing, it is notified to the outside of the function through the return value.
Change the above example and calculate the result from m to n:
1. int sum(int m, int n){ 2. int i, sum=0; 3. for(i=m; i<=n; i++){ 4. sum+=i; 5. } 6. return sum; 7. }
The parameters given in the parameter list can be used in the function body in the same way as ordinary variables.
When calling the sum() function, you need to pass it two copies of data, one to m and one to n. You can pass integers directly, for example:
int result = sum(1, 100); //1 to m, 100 to n You can also pass variables: int begin = 4; int end = 86; int result = sum(begin, end); //begin is passed to m and end is passed to n You can also pass integers and variables together: int num = 33; int result = sum(num, 80); //num to m, 80 to n
The parameters given during function definition are called formal parameters, which are called formal parameters for short; The parameters given during function call (that is, the data passed) are called actual parameters, which are referred to as actual parameters for short. When a function is called, the value of the argument is passed to the formal parameter, which is equivalent to an assignment operation.
In principle, the type and number of arguments should be consistent with the formal parameters. If automatic type conversion or forced type conversion can be performed, the argument type can also be different from the formal parameter type. For example, automatic type conversion will occur when an argument of type int is passed to a formal parameter of type float.
Complete the above code:
1. #include <stdio.h> 3. int sum(int m, int n){ 4. int i, sum=0; 5. for(i=m; i<=n; i++){ 6. sum+=i; 7. } 8. return sum; 9. } 11. int main(){ 12. int begin = 5, end = 86; 13. int result = sum(begin, end); 14. printf("The sum from %d to %d is %d\n", begin, end, result); 15. return 0; 16. } Operation results: The sum from 5 to 86 is 3731 definition sum() Parameters when m,n The values of are unknown; call sum() When, will begin,end The values of are passed to m,n,This is the same as the process of assigning values to variables. It is equivalent to: m = begin; n = end;
Functions cannot be nested
array
Array: a collection of elements of the same type
int arr[10] = {1,2,3,4,5,6,7,8,9,10}; char ch[5] = {'a','b','c'};//Incomplete initialization, the remaining defaults to 0; Type and name of the array[Size of array] = {Values in the array}
The array is accessed by subscript and printed 1 ~ 10;
#include <stdio.h> int main() { int arr[10] = {1,2,3,4,5,6,7,8,9,10}; int i = 0; while(i<10) { printf("%d",arr[i]); i++; } }
The subscript of the array starts from 0;
Operator
Arithmetic operator:
+ - * / % / Division operator % Remainder operator
Shift operators
^ | & ~ >> <<
Shift operators include shift left < < and shift right > >. There is something here when shifting.
- When using the shift left operator, it is very simple to shift the binary number of the number to the left. The number of bits shifted to the left will be supplemented by the number of bits of 0 on the right.
- When using the shift right operator, there are two ways to shift right:
- Logical shift right: after logical shift right, fill 0 on the left
- Arithmetic shift right: the arithmetic shift right fills the sign bit on the left after the arithmetic shift right
- Note: the shift operator cannot move negative digits. For example: n = n < < - 1; This is not allowed!
- &: bitwise AND
- |: bitwise OR
- ^: bitwise XOR. This operator has a characteristic that the number obtained after one number XOR another twice is still the original number. That is, the same number twice is equivalent to no XOR. XOR operator refers to two values involved in the operation. If the two corresponding bits are the same, the result is 0, otherwise it is 1. Namely: 0 ^ 0 = 0, 1 ^ 0 = 1, 0 ^ 1 = 1, 11 = 0, for example: 101000010001 = 10110000
Relational operator
Relational operators are binocular operators, and there are only two results, namely true (1) or false (0). Note here that you can't use these operators with mathematical thinking. Some easy mistakes, such as judging whether a variable a is within a certain range. You can't write 10 < a < 20; Here, the compiler will execute 10 < a first; As mentioned above, the value of this expression is only 0 and 1, so no matter what the size relationship between 10 and a is, 10 < A; This is not necessarily less than our expected result! Therefore, it should be written as 10 < A & & A < 20; The results of these two expressions are completely different.
Logical operator
First of all, it is different from logic and logic or operators & & and |, and it is clear that these are two operators! There are some rules in the use of these operators. If one of the & & operators is false, the result must be false, and the previous expression is false, the following expression will not be executed; If one of the results of the | operator is true, the result must be true, and when the previous condition is true, the latter condition will no longer execute and judge whether it is true or false.
Assignment operator
+= -= = /= %= |= &= >>= <<=
- Simple assignment =, this operator is very simple, which is used to assign values to data, but many beginners are easy to use this assignment operator to assign values to strings. Note that this operator cannot be used to assign values to strings!
- Compound assignment + = - = / =% = | = & = > > = < < =. These operators are assigned to the left number after calculating the number on the left and the number on the right of the operator.
unary operator
a+b: there are two operands, binocular operator; Unary operator: only one operand;
In C language: 0 is false, non-0 is true
-
Left operator: the operator is on the left, - a, + a! a etc
-Indicates a negative number
+Indicates a positive number -
sizeof calculates the length of bytes, which is bytes, unit: bytes; For example, calculate the number of elements of an array.
int sz = sizeof(arr)/sizeof(arr[0]);
-
*Dereference, indirect access operator
-
&Take address
-
~Bitwise negation, binary bitwise negation
The integer stored in memory is: complement -
! Logic is reversed, true becomes false, and false becomes true;
-
(type) cast operator
int main() { int a = (int)3.14;//Convert 3.14 float type to int type printf("%d\n",a); return 0; }
- ++-- cumulative subtraction operator
- Right operator: + + -- addition and subtraction
int main() { int a = 3, b = 4, c; c = (a++) + (++b);//3+5 printf("%d %d %d\n", a, b, c); return 0; }
Distinguish the difference between the left operator and the right operator: the left operator operates first and then uses, while the right operator uses first and then operates
That's the difference between + + A and a + +++ a=4,a++=3, equivalent to a=3, then output, and finally a+1,
Conditional operator (trinocular operator)
exp1 ? exp2 : exp3
exp1 holds, and exp2 calculates the structure of the entire expression: the result of exp2
exp1 doesn't hold. exp3 calculates the whole expression by the result of exp3
max = a>b ? a : b;
comma expression
A comma separated string of expressions;
int a = 0; int b = 3; int c = 5; int d = (a = b+2,c = a-4,b=c+2);
Comma expressions are evaluated from left to right
The result of the entire expression is the result of the last expression
Subscript quote operator
function call operator
keyword
keyword | explain |
---|---|
auto | Declare automatic variables |
short | Declare a short integer variable or function |
int | Declare an integer variable or function |
long | Declare a long integer variable or function |
float | Declare a floating-point variable or function |
double | Declare a double variable or function |
char | Declare a character variable or function |
struct | Declare structure variables or functions |
union | Declare a common data type |
enum | Declare enumeration type |
typedef | Used to alias data types |
const | Declare read-only variables |
unsigned | Declare an unsigned variable or function |
signed | Declare a variable or function of signed type |
extern | Declared variables are declared in other files |
register | Declare register variable |
static | Declare static variables |
volatile | Description variables can be changed implicitly during program execution |
void | The declared function has no return value or parameter, and the declared function has no type pointer |
if | Conditional statement |
else | Conditional statement negative branch (used with if) |
switch | For switch statements |
case | Switch statement branch |
for | A circular statement |
do | Loop body of a loop statement |
while | Loop condition of loop statement |
goto | Unconditional jump statement |
continue | End the current cycle and start the next cycle |
break | Jump out of current loop |
default | Other branch in switch statement |
sizeof | Calculate data type length |
return | Subroutine return statement (with or without parameters) loop condition |
- Provided by C language, keywords cannot be created;
- Variable name cannot be keyword;
- define and include are not keywords, but preprocessing instructions;
auto - declare automatic variables
int a = 10;//Automatically created, automatically destroyed - automatic variables //auto int a = 10; //auto omitted
break - jump out of the current loop
Register - declare register variables
register int num 100;//It is recommended that the value of num be stored in the register
typedef type redefinition
typedef unsigned int u_int; //Equivalent to alias int main() { unsigned int num = 100; u_int num2 = 100; //The variables created by the above two have the same meaning }
Static static
- Modify local variables
- Modify global variables
- Modifier function
void test() { int a = 1; a++; printf("%d",a); } int main() { int i = 0; while (i<0) { test(); i++; } return 0; }
Operation results: print 10 2;
Add static
void test() { static int a = 1; a++; printf("%d",a); } int main() { int i = 0; while (i<0) { test(); i++; } return 0; }
Operation results: 2 ~ 11
static modifies local variables and changes the life cycle of local variables (essentially changing the storage type of variables)
Global variables can be used in the whole project. You only need to declare them with the keyword extern;
static modifies global variables. Yes, global variables can only be used in this source file
Global variables can be used inside other source files because they have external link attributes; However, after being modified by static, it becomes an internal link attribute, and other source files cannot be linked to this static global variable!
Static modifies the function. Yes, the function can only be used in this source file. It can be used inside other source files because the function has external link attribute; However, after being modified by static, it becomes an internal link attribute, and other source files cannot be linked to this function!
Constants and macros
#define defines constants and macros
//#define preprocessing instruction //1. define constant #define MAX 1000 int main() { printf("%d\n",MAX); return 0; } //2.define macro //Macro: some commands are organized together to complete a specific task as a single command #define ADD(X,Y) X+Y int main() { printf("%d\n",4*ADD(2,3)); return 0; }
Operation result: 11; Because: 4*ADD(2, 3) actually runs the operation of 4 * 2 + 3;
Pointer
The data is stored in memory,
- Memory number
- 32-bit address: 32 address lines. The physical line is powered on, representing 1 / 0;
- 64 bit address: 64 address lines;
There are 232 addresses in 32 bits and 264 addresses in 64 bits;
A memory unit is a byte;
int main() { int a = 10; //a - 4 bytes of space to be allocated in memory; printf("%p\n",&a);//%p used exclusively to print addresses; int * pa = &a;//pa is used to store the address and is a pointer variable in C language //*Description pa is a pointer variable //Int indicates that the object executed by pa is of type int; return 0; }
int main() { int a = 10; int* pa = &a; *pa = 20;//*The dereference operation * pa is to find a through the address in pa printf("%d\n",a); return 0; }
The size of the pointer is the same. The pointer is used to store the address. How much space the pointer needs depends on how much space the address needs to be stored. 4 bytes on 32-bit machines and 8 bytes on 64 bit machines;
structural morphology
Structs allow C language to create new types
//Create a student type struct Stu { char name[20];//Member variable int age; double score; }; struct Book { char name[20]; float price; char id[30]; }; int main() { struct Stu s = {"Zhang San",20,85.5};//Create an instance using the type printf("%s %d %lf\n",s.name,s.age,s.score);//Structural variables Member variable struct Stu * ps = &s; printf("%s %d %lf\n",(*ps).name,(*ps).age,(*ps).score); //Or: printf("%s %d %lf\n",*ps->name,*ps->age,*ps->score);//Pointer of structure - > member variable; return 0; }