The last one was rewritten IDEA 2021.2 new Java Web project and Tomcat deployment,
The next article is the core, the basic usage of JavaBean s and IDEA configuration
JavaBean overview
- JavaBean is a component that uses Java language mechanism to realize specific functions
- JavaBean is actually a Java class. Jsp can reduce the code redundancy in Jsp by accessing variables and calling methods in JavaBean
- The relationship between JSP, Serlet and JavaBean
- JavaBean is a special Java class. It follows the JavaBean API specification and implements the java.io.Serializable interface. It is a public class. The class must contain a parameterless constructor and provide set and get functions for attribute access
- JavaBean s need to be placed in the specified directory so that the server can find and execute them, generally Web Root/WEB-INF/classes
Reference JavaBean in JSP
jsp:useBean: load a javaBean that will be used in the JSP page
- use
<jsp:useBean id="name" class="package.class" scope="scope"/>
<jsp:useBean id="stu" class="YoungHD.StudentBean" scope="page"> ... </jsp:useBean>
- Where id is the reference name used to create javaBean; Class is used to specify the class of javaBean; scope is used to specify the range of JavaBeans. The default value is page.
- scope value range:
- Page, JavaBeans can only be used on the current page and will be destroyed when a new page is loaded.
- Request, which is used by javaBean when the user sends a request.
- Session, javaBean always exists in the session.
- Application, javaBean can be used in the whole application.
jsp:setProperty sets the javaBean property value specified in useBean
- Basic Usage
<jsp:setProperty property="PropertyName" name="BeanName" value="Value" param="Parameter"/>
- Where name specifies the id of the javaBean used in useBean; Property is used to specify the name of the property for which you want to set a value. Value specifies the value to set for the property. param specifies the value of the form element used to enter data.
- Note: the value attribute and param attribute cannot be used at the same time.
- give an example
<jsp:setProperty property="username" name="YoungHD" value="younghd"/> <jsp:setProperty name="stu" property="username" param="username"></jsp:setProperty>
jsp:getProperty gets the property value specified in the javaBean
- Basic grammar
<jsp:getProperty name="BeanName" property="PropertyName" />
- Where name specifies the id of the javaBean used in useBean; Property specifies the property name of the javaBean to get
- Example: the two methods have the same effect
full name:<%=stu.getUsername()%><hr> full name:<jsp:getProperty property="username" name="stu"/><hr>
Automatic generation and configuration of JavaBean s in IDEA
- Create JavaBean: create a package under the src directory in the project and create a Java class under the package (Note: you must create a package, you cannot create a Java class directly, otherwise you cannot import JavaBeans
- Configure the IDEA project, create the classes directory and set the export path. The export path is Web Root/WEB-INF/classes (press and hold F4 on the project)
- Automatically generate Getter and Setter methods: select variable: ALT+INSERT shortcut key or right-click variable
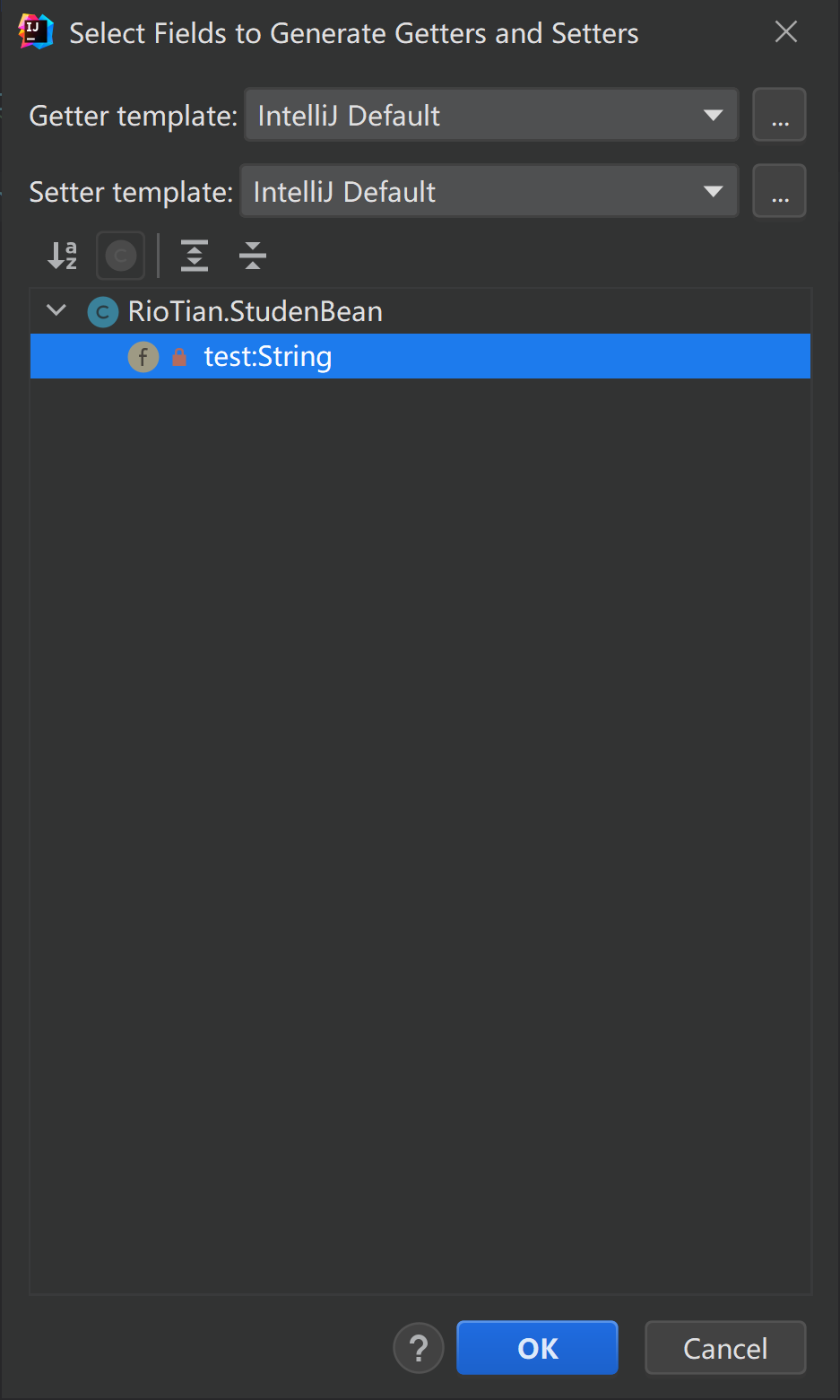
\[QAQ
\]
Actual combat: using JavaBean s to submit forms
- StudenBean.java section
package RioTian; public class StudenBean implements java.io.Serializable { public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getSchool_id() { return school_id; } public void setSchool_id(String school_id) { this.school_id = school_id; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public String getCollege() { return college; } public void setCollege(String college) { this.college = college; } public String getMy_introduce() { return my_introduce; } public void setMy_introduce(String my_introduce) { this.my_introduce = my_introduce; } public String[] getLoves() { return loves; } public void setLoves(String[] loves) { this.loves = loves; } private String username,password,school_id,address,sex,college,my_introduce; private String []loves; }
- index.HTML section
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Classmate information</title> <style> body{background-color: beige} </style> <link rel="stylesheet" type="text/css" href="../css/f5.css"> <script src="../js/f5.js"></script> </head> <body> <div align="center"> <p id="t_head">Personal information form</p><br> </div> <div align="center"> <form action="../index.jsp" method="post" name="form_1" onsubmit="return user_Check()" > full name:<input type="text" name="username"><hr> password:<input type="password" name="password" onchange="return pwd_check1()"><hr> Confirm:<input type="password" name="re_password" onchange="return pwd_check2()"><hr> Student No.:<input type="text" name="school_id"><hr> Dormitory:<input type="text" name="address"><hr> Gender: <input name="sex" type="radio" checked="checked" value="boy"/>male <input name="sex" type="radio" value="girl"/>female<hr> college: <select name="college" style="width: 15%"> <option value ="computer">Computer science and technology</option> <option value ="math">Academy of Mathematics</option> <option value="business">Business School</option> <option value="art">College of Arts</option> <option value="chemistry">Academy of Chemical Sciences</option> </select><hr> Hobbies: <input type="checkbox" name="loves" value="10">read <input type="checkbox" name="loves" value="20">Swimming <input type="checkbox" name="loves" value="30">music <input type="checkbox" name="loves" value="40">writing<hr> Personal profile:<textarea name="my_introduce" rows="5" style="width: 20%"></textarea><hr> <input type="submit" name="Submit1" value="register"> <input type="reset" name="Submit2" value="Reset"> </form> </div> </body> </html>
- index.jsp section
<%-- Created by IntelliJ IDEA. User: RioTian Date: 2021/11/4 Time: 20:38 --%> <%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" import="RioTian.StudenBean" %> <!DOCTYPE html> <html> <head> <title>JavaBean Processing page</title> <style> body{ background-color: beige; } </style> </head> <body> <h1 style="align-content: center"> JavaBean Use of </h1> <%request.setCharacterEncoding("UTF-8");//The client page is controlled as UTF-8% > <jsp:useBean id="stu" class="RioTian.StudenBean"> <jsp:setProperty name="stu" property="username" param="username"></jsp:setProperty> <jsp:setProperty name="stu" property="password" param="password"></jsp:setProperty> <jsp:setProperty name="stu" property="school_id" param="school_id"></jsp:setProperty> <jsp:setProperty name="stu" property="address" param="address"></jsp:setProperty> <jsp:setProperty name="stu" property="sex" param="sex"></jsp:setProperty> <jsp:setProperty name="stu" property="college" param="college"></jsp:setProperty> <jsp:setProperty name="stu" property="loves" param="loves"></jsp:setProperty> <jsp:setProperty name="stu" property="my_introduce" param="my_introduce"></jsp:setProperty> </jsp:useBean> Name: <%=stu.getUsername()%> <hr> Name: <jsp:getProperty name="stu" property="username"/><hr> Password: <jsp:getProperty name="stu" property="school_id"/><hr> Stu_Id: <jsp:getProperty name="stu" property="school_id"/><hr> Dormitory: <jsp:getProperty name="stu" property="address"/><hr> Sex: <jsp:getProperty name="stu" property="sex"/><hr> College: <jsp:getProperty name="stu" property="college"/><hr> Loves: <% String []temp=stu.getLoves(); for (int i = 0;i < stu.getLoves().length;i++){ out.print(temp[i]+"--"); } %><hr> Personal-Profile: <jsp:getProperty name="stu" property="my_introduce"/> <hr> </body> </html>