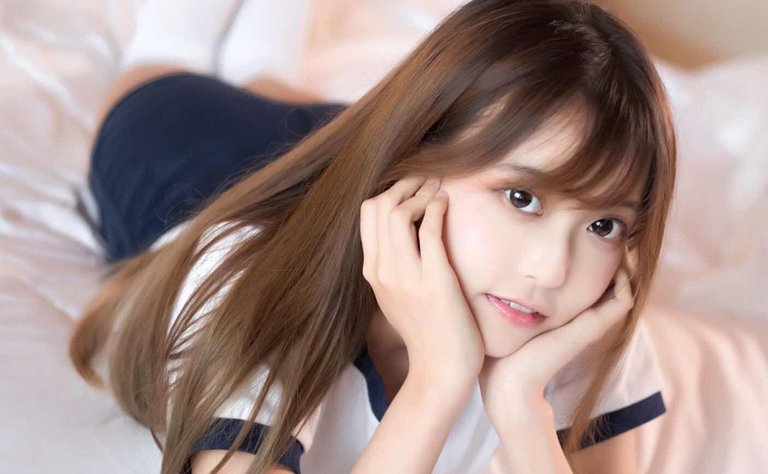
JavaScript is a lightweight programming language
Programming code that inserts HTML pages
JavaScript Usage, Output, Statement, Comment, Variable, Data Type, Function, Operator, Comparison, Conditional Statement, Loop, Break, Error, Type Conversion, Form Validation.
<script> alert("My first JavaScript"); </script> <head> <script> function myFunction() { document.getElementById("demo").innerHTML="My First JavaScript Function"; } </script> </head> <button type="button" onclick="myFunction()">click</button> <!DOCTYPE html> <html> <body> <script src="myScript.js"></script> </body> </html>
output
window.alert()
Pop-up warning box
document.write()
Write content to an HTML document
innerHTML is written to an HTML element
console.log() written to console
document.getElementById("myDIV").innerHTML="wiki";
Single line comment begins with //
Multiline comments start/end/end
Variables:
Usually starts with a letter, starts with $and _and is case sensitive
Data type:
Number, string, Boolean, array, object, empty, undefined,
JavaScript functions
function functionName() { Is the code to execute }
JavaScript Operators
The operator'='is used for assignment.
The operator'+'is used for addition.
Comparison operator
Comparison operators are used in logical statements to determine whether variables or values are equal.
JavaScript conditional statement
if statement
if...else statement
if...else if....else statement
switch statement
JavaScript loop
for
for/in
while
do/while
Break
The break statement is used to jump out of the loop.
for (i=0;i<10;i++) { if (i==3) { break; } x=x + "The number is " + i + "<br>"; }
continue Statement
Continue is used to skip an iteration in a loop if a specified condition occurs, then continue to the next iteration in the loop.
label: Sentence
error
Error in try statement testing code block.
catch statement processing error.
throw statement creates a custom error.
throw exception
The exception can be:
JavaScript string, number, logical value, or object
Type conversion:
Data type: string, number, boolean, object, function
Three object types: Object, Date, Array
Two data types that do not contain any numeric values: null, undefined
typeof operator
View data types for JavaScript variables
constructor property return
Constructor for all JavaScript variables
String() toString() Date() String(new Date()) obj = new Date() obj.toString() Number(false) // Return 0 Number(true) // Return 1
Form Validation
function validateForm(){ var x=document.forms["myForm"]["fname"].value; if (x==null || x=="") { alert("Last name is required"); return false; } }
function validateForm(){ var x=document.forms["myForm"]["email"].value; var atpos=x.indexOf("@"); var dotpos=x.lastIndexOf("."); if (atpos<1 || dotpos<atpos+2 || dotpos+2>=x.length){ alert("Not a valid e-mail address"); return false; } }
Document Object Model
var x=document.getElementById("name"); var y=x.getElementsByTagName("p"); var x=document.getElementsByClassName("name");
<!DOCTYPE html> <html> <body> <script> document.write(Date()); </script> </body> </html> <script> document.getElementById("p1").innerHTML=""; </script> <script> document.getElementById("image").src=".jpg"; </script> document.getElementById(id).style.property=new style <script> document.getElementById("p2").style.color="pink"; document.getElementById("p2").style.fontFamily="Microsoft YaHei"; document.getElementById("p2").style.fontSize="30px"; </script> <h1 onclick="this.innerHTML='wiki'">Please click on this text</h1> <button onclick="displayDate()">click here</button> <script> document.getElementById("myBtn").onclick=function(){displayDate()}; </script> onload and onunload Events are triggered when a user enters or leaves the page onchange Events are often used in conjunction with validation of input fields onmouseover and onmouseout Event onmousedown,onmouseup as well as onclick Event
DOM Node
<div id="div1"> <p id="p1">This is a paragraph.</p> <p id="p2">This is another paragraph</p> </div> <script> //This code creates a new <p>element var para=document.createElement("p"); //This code creates a text node var node=document.createTextNode("This is a new paragraph"); //Append this text node to the <p>element para.appendChild(node); //An existing element is found in this code var element=document.getElementById("div1"); element.appendChild(para); </script>
//Contains <div>elements that have two child nodes (two <p>elements) <div id="div1"> <p id="p1">This is a paragraph.</p> <p id="p2">This is another paragraph</p> </div> <script> //Found element with id="div1" var parent=document.getElementById("div1"); //Found <p>element with id="p1" var child=document.getElementById("p1"); //Remove child elements from parent elements parent.removeChild(child); </script>
object
JavaScript objects are data with properties and methods
Almost everything in JavaScript is an object
Almost all transactions are objects: strings, numbers, arrays, dates, functions, and so on
number
JavaScript numbers are 64 bits
constructor
Returns a reference to the Number function that created this object
MAX_VALUE
Maximum Number Representable
MIN_VALUE
Minimum number representable
NaN
Non-numeric value
Character string
Find String in String
indexOf()
To locate the first occurrence of a specified character in a string
Content Matching
The match() function is used to find specific characters in a string
Return this character
replace content
The replace() method replaces some characters with others in a string
toUpperCase() or toLowerCase()
charAt() returns a character at a specified position
charCodeAt() returns the Unicode encoding of a character at a specified location
concat() connection string
fixed() Displays a string as typewriter text
fontcolor() uses the specified color to display the string
fontsize() uses the specified size to display the string
fromCharCode() Creates a string from character encoding
indexOf() retrieves the string
italics() uses italics to display strings in italics
lastIndexOf() searches backwards and forwards for Strings
link() Displays a string as a link
match() finds a match for one or more regular expressions
search() retrieves the value that matches the regular expression
slice() extracts a fragment of a string and returns the extracted portion in a new string
strike() uses strikethroughs to display strings
substring() extracts the character between two specified index numbers in a string
sup() displays the string as superscript
toLocaleLowerCase() Converts a string to lowercase
toLocaleUpperCase() Converts a string to uppercase
toString() returns a string
valueOf() returns the original value of a string object
date
new Date() // Current date and time new Date(milliseconds) //Returns the milliseconds since January 1, 1970 new Date(dateString) new Date(year, month, day, hours, minutes, seconds, milliseconds) ate() Return the date and time of the day
Define Array
join()
Place all elements of the array in a string.Elements are separated by a specified separator
pop()
Delete and return the last element of the array
push()
Add one or more elements to the end of the array and return the new length
reverse()
Reverse the order of elements in an array
shift()
Delete and return the first element of the array
slice()
Return selected elements from an existing array
sort()
Sort elements of an array
splice()
Delete elements and add new elements to the array
toSource()
Returns the source code of the object
toString()
Converts an array to a string and returns the result
unshift()
Add one or more elements to the beginning of the array and return the new length
valueOf()
Returns the original value of an array object
Create Boolean Object
If the logical object has no initial value or its values are 0, -0, null,''', false, undefined, or NaN, then the value of the object is false
regular expression
text() method
The test() method retrieves the specified value in the string
exec() method retrieves the specified value in the string
Return value is the value found
If no match is found, null is returned.
compile()
Method is used to change the regular expression
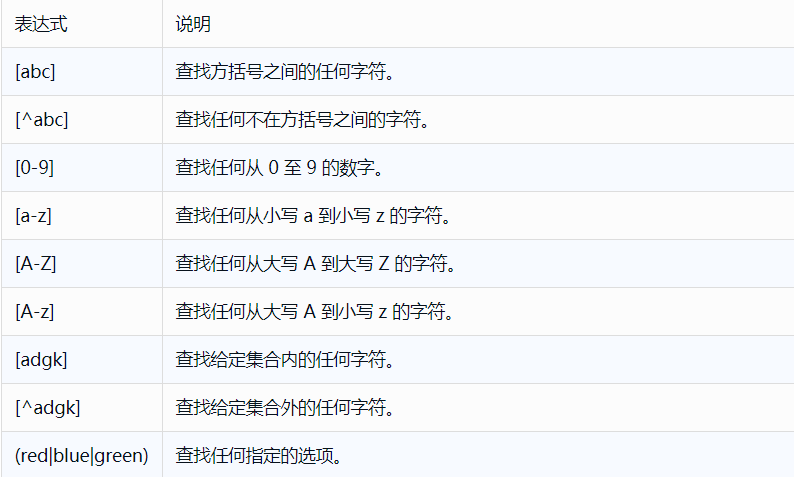
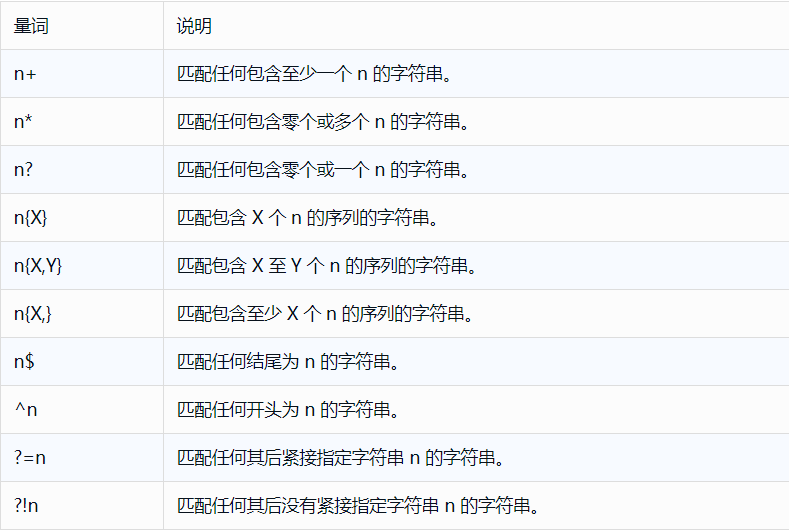
Browser Object Model BOM
window.document.getElementById("header"); //Same as this document.getElementById("header");
window.innerHeight - Internal height of browser window (including scrollbars)
window.innerWidth - Internal width of browser window (including scrollbars)
document.documentElement.clientHeight
document.documentElement.clientWidth
document.body.clientHeight
document.body.clientWidth
The window.screen object contains information about the user's screen
The window.location object is used to get the address (URL) of the current page and redirect the browser to a new page
The window.history object contains the history of the browser
The window.navigator object contains information about the visitor's browser
Three message boxes: warning box, confirmation box, prompt box
alert("Warning prompt text") confirm("Confirmation prompt text") prompt("Prompt Text","Default value")
setTimeout()
clearTimeout()
var myVar; function myFunction() { myVar=setTimeout(function(){alert("Hello")},5000); } function myStopFunction() { clearTimeout(myVar); }
What is a cookie?
Cookie s are data stored on a text file on a computer
document.cookie properties to create, read, and delete cookies
Read cookie s
Example:
var x = document.cookie;
function checkCookie() { var username=getCookie("username"); if (username!="") { alert("Welcome again " + username); } else { username = prompt("Please enter your name:",""); if (username!="" && username!=null) { setCookie("username",username,365); } } }
Please compliment!Because your approval/encouragement is my greatest motivation to write!
Welcome to your attention Dashu Xiaosheng Simple book!
This is a quality, attitudinal blog
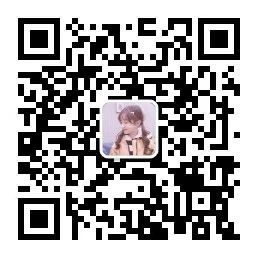