Knowledge System
Promise basic syntax, how to handle errors, a brief introduction to asynchronous functions
content
There are two ways to handle errors:
reject('error message').then(null, message => {}) throw new Error('error message').catch(message => {})
Recommend the second
4 cases promise
// 1 doSomething().then(function () { return doSomethingElse(); }); // 2 doSomething().then(function () { doSomethingElse(); }); // 3 doSomething().then(doSomethingElse()); // 4 doSomething().then(doSomethingElse);
.then()
The state response function can return a new Promise, or other value
If a new Promise is returned, the next level.then() executes after the new Promise state changes
If any other value is returned, the next level.then() is immediately executed.
then() accepts two functions as parameters:
fulfilled and rejected
.then() returns a new Promise instance, so it can be called chained
When the current Promise state changes,.then() executes by selecting a specific state response function based on its final state
There can be multiple then, queued, and each then returns a new promise instance
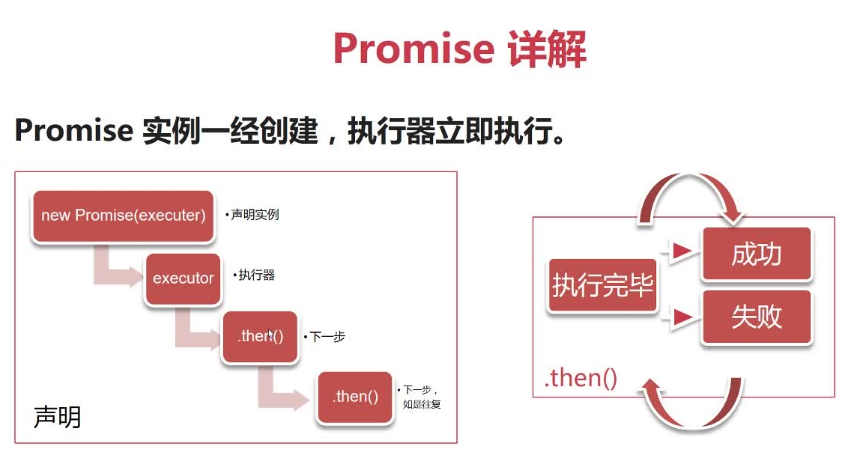
promise status
The Promise state changes, triggering subsequent steps after processing by the response function in.then().
Once the Promise state is changed, it does not change.
promise structure!!
new Promise( // Actuator function (resolve, reject) { // A long asynchronous operation resolve(); // Data processing complete reject(); // Data processing error } ).then(function A() { // Success, next step }, function B() { // fail });
Promise.race()
Common usage:
Put asynchronous operation with timer
If the timer triggers first, it is considered timeout and informs the user
The nable is an object with a then() function
.then( value => { console.log(value, 'World'); return Promise.resolve(new Promise( resolve => { setTimeout(() => { resolve('Good'); }, 2000); })); }) .then( value => { console.log(value, 'evening'); return Promise.resolve({ then() { console.log('everone'); } }) });
Promise.all and map
promise.all() is used because all files are read out before they are processed
const FileSystem = require('./FileSystem'); function findLargest(dir){ return FildSystem.readDir(dir, 'utf-8') .then( files => { return Promise.all( files.map( file=> { return new Promise(resolve => { fs.stat(path.join(dir, file), (err, stat) => { if(err) throw err; if(stat.isDirectory()) { return resolve({ size: 0 }); } stat.file = file; resolve(stat); }); }); })); })
Promise Interpretation
Promise object for asynchronous calculation
A Promise represents a value that is not currently, future, or permanently available
Mainly used for asynchronous computing
Asynchronous operations can be queued, executed in the desired order, and returned with expected results
Promise can be passed between objects and manipulated to help us process queues
Reasons for Asynchronization
Common syntax for asynchronous operations:
document.getElementById('start').addEventListener('click', start, false); function start() { // Response Events } // jquery $('#start').on('click', start);
Callback:
// More common are ajax $.ajax('http://baidu.com', { success: function (res) { // This is the callback function } }); // Or callback after the page is loaded $(function () { // This is also a callback function });
Asynchronous operations are event-based
Callbacks occur mainly in Ajax and File API s
The problem is not serious at this time
Asynchronous callback issues
Walk through the directory to find the largest file
const fs = require('fs'); const path = require('path'); function findLargest(dir, callback) { fs.readdir(dir, function (err, files) { if (err) return callback(err); let count = files.length; let errored = false; let stats = []; files.forEach( file => { fs.stat(path.join(dir, file), (err, stat) => { if(errored) return; if(err) { errored = true; return callback(err); } stats.push(stat);
Introduction to Promise
new Promise( // Actuator function (resolve, reject) { // A long asynchronous operation resolve(); // Data processing complete reject(); // Data processing error } ) .then(function A() { // Success, next step },function B() { // Fail, handle accordingly });
Promise is a proxy object that has nothing to do with the original operation.
It does this by introducing a callback.
Promise has three states
Pending [pending] initial state fulfilled [implementation] operation succeeded rejected [vetoed] operation failed
When the promise state changes, the next step in the response function in.then() is triggered.
Once the Promise state is changed, it does not change.
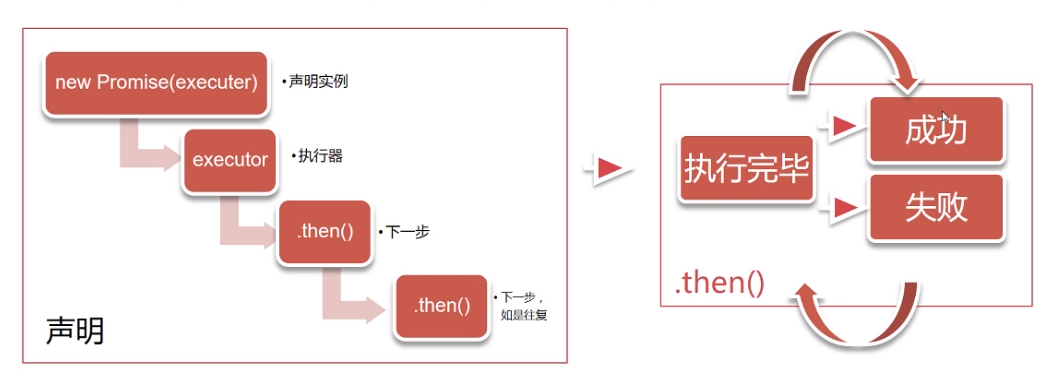
The simplest example
timer
new Promise( resolve => { setTimeout( () => { resolve('hello'); }, 2000); }) .then( value => { console.log( value + 'world'); });
Example of two-step execution
new Promise( resolve => { setTimeout( () => { resolve('hello'); }, 2000); }) .then( value => { console.log(value); return new Promise( resolve => { setTimeout( () => { resolve('world') }, 2000); }); }) .then(value => { console.log(value + 'world'); });
Execute then on completed Promise
let promise = new Promise(resolve => { setTimeout(() => { console.log('the promise fulfilled'); resolve('hello,world'); }, 1000); }); setTimeout( () => { promise.then( value => { console.log(value); }); }, 2000);
Promise is not returned in the
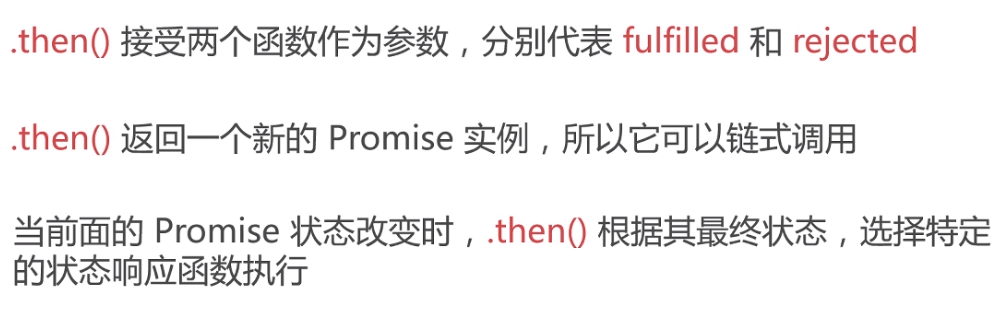
Nesting of the n
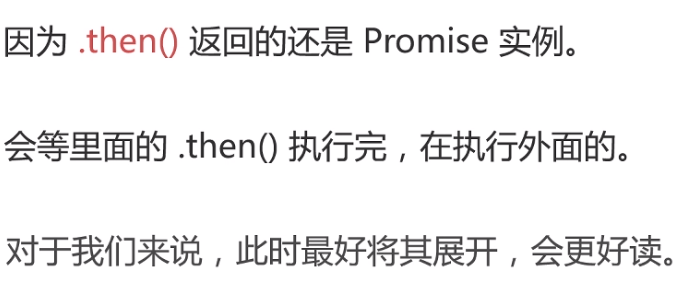
Chain call to.then
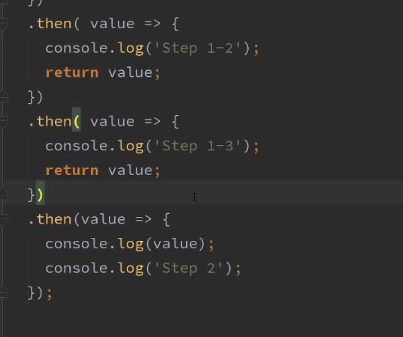
console.log('start'); new Promise( resolve => { console.log('Step 1'); setTimeout(() => { resolve(100); }, 1000); }) .then( value => { return new Promise(resolve => { console.log('Step 1-1'); setTimeout(() => { resolve(110); },1000); }) .then( value=> { console.log('Step 1-2'); return value; }) .then( value=> { console.log('Step 1-3'); return value; }); }) .then(value=> { console.log(value); console.log('Step 2'); });
Four cases of Promise:
doSomething() .then(function(){ return doSomethingElse(); }) .then(finalHandler);
First step, doSomething, second step, doSomething Else (undefined), third step, finalHander (resultOfDoSomething Else)
doSomething() .then(function () { doSomethingElse(); }) .then(finalHander);
The first step is doSomething, the second is doSomething Else (undefined), findHandler(undefined)
doSomething() .then(doSomethingElse()) .then(finalHandler);
First step, doSomething, doSomething Else (undefined), second step, finalHandler(resultOfDoSomething)
doSomething() .then(doSomethingElse) .then(finalHandler);
Step one doSomething, step two doSomething Else, and step three finalHandler (resultOfDoSomething Else)
What is the difference between these four commitments?
doSomething().then(function () { return doSomethingElse(); }); doSomething().then(function () { doSomethingElse(); }); doSomething().then(doSomethingElse()); doSomething().then(doSomethingElse);
error handling
Promise automatically catches internal exceptions and handles them to the rejected response function.
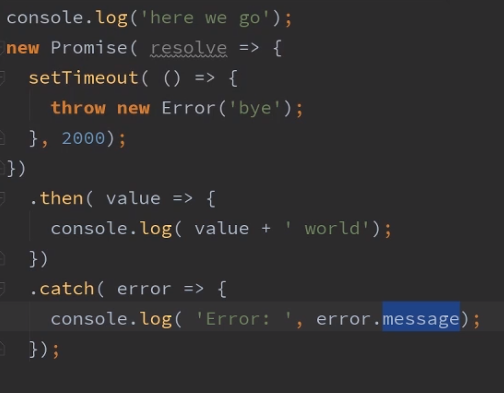
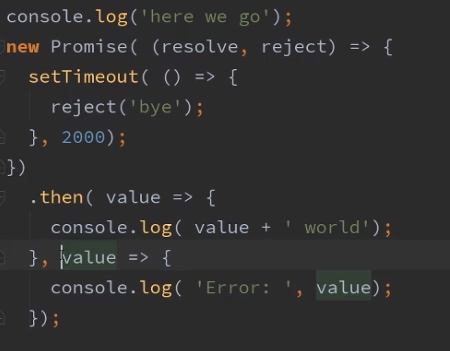
Error and then
.catch + .then()
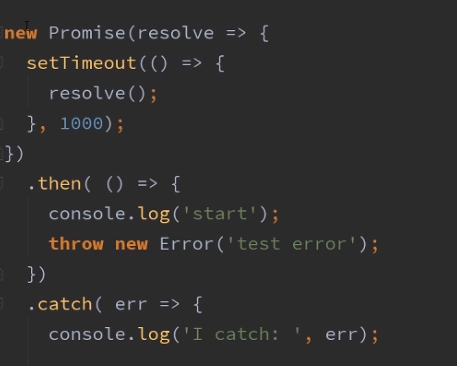
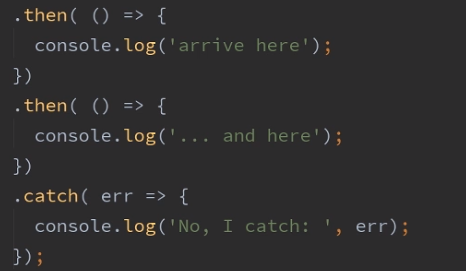
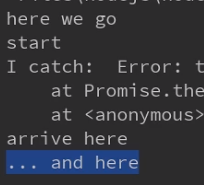
Promise.all

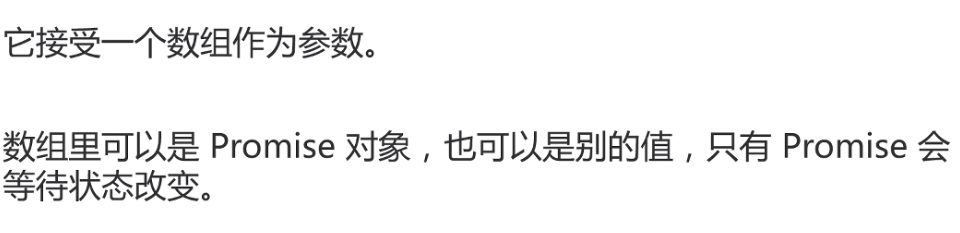


Use with.map
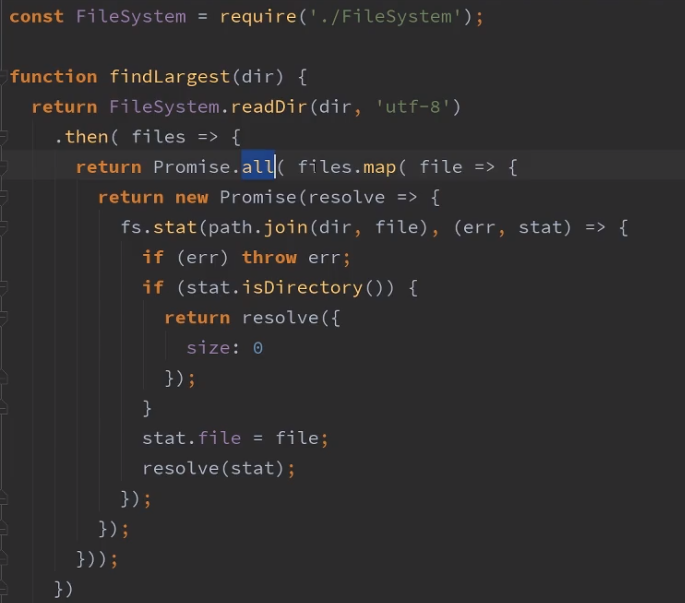
Implement Queue

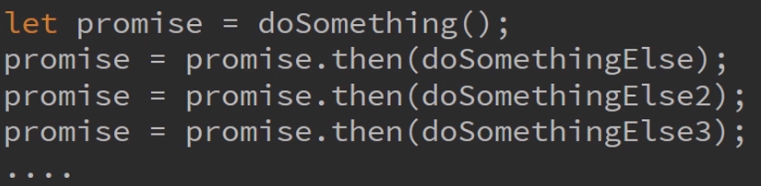
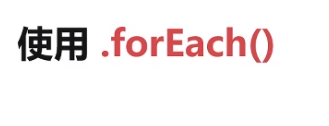
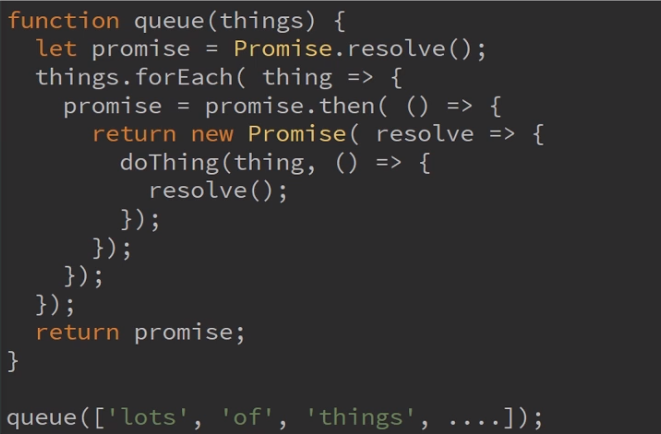
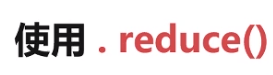
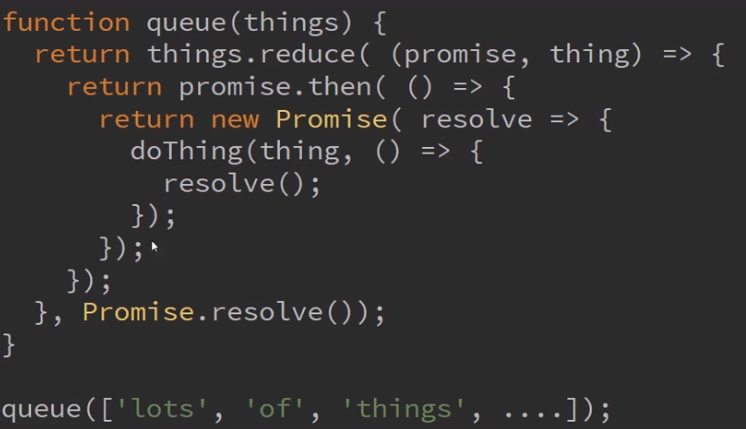
Implement Crawlers
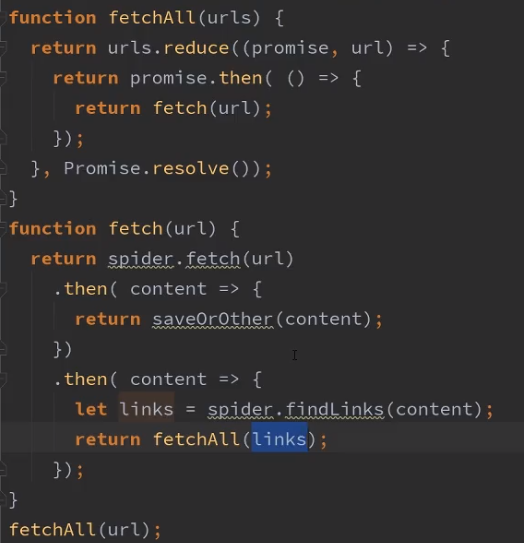
Promise.resolve
Promise.reject
Promise.race
class Confirm { constructor() { this.promise = new Promise( (resolve, reject) => { this.confirmButton.onClick = resolve; this.cancelButton.onClick = reject; }); } }
Asynchronous function
async/await
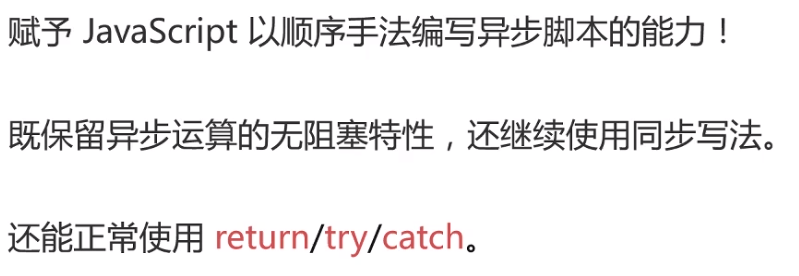
Please compliment!Because your encouragement is my greatest motivation to write!
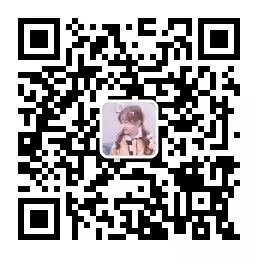
Blow the crowd: 711613774
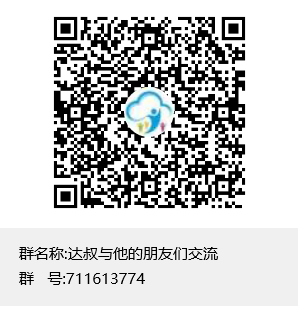