When you create a function, you must write its definition. All function definitions include the following components:
- Name: each function must have a name. In general, the same rules that apply to variable names apply to function names.
- Formal parameter list: the program module calling the function can send data to it. A formal parameter list is a list of variables that hold the values passed to a function. If no value is passed to the function, its formal parameter list is empty.
- Body: the body of a function is a set of statements that handle the task being performed by the function. These statements are contained in a set of braces.
- Return type: the function can send the value to the program module used by the callback. The return type is the data type of the value to send back.
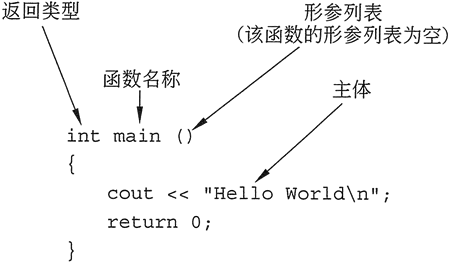
Figure 1 components of function
The first line in the definition is called the function header. Now take a closer look at these three parts. The first part is the return type of the function, followed by the function name, and a pair of parentheses at the end of the function header. If the function has any arguments, they are arranged in the parentheses. Of course, even if the formal parameter list is empty, the parentheses must be retained, as shown in Figure 1.
1. Null function
As mentioned earlier, the function can return a value. The main function in all programs introduced in the tutorial is declared to return an int value to the operating system. "return 0;" Statement causes the main function to return a value of 0 when it completes execution.However, not all functions must return a value. Some functions simply execute one or more statements and then return. In C + +, such functions are called null functions.
The displayMessage function shown below is an example of an empty function:
The name of the function is displayMessage, which means "display message". It is a descriptive name that describes the function of the function. Functions should be named in this way, that is, their functions should be revealed by their names. Because the function does not need to receive any information to perform its task, there is no formal parameter list in parentheses.
The return type of this function is void. This means that the function does not return a value after execution and returns to the part that calls the program. Because there is no return value, the return statement is not required. When the statement in the function has completed execution and encounters the closed brace of the end function, the program will return automatically.
Now let's look at a program that contains two functions: main and displayMessage.
Program output results:
void displayMessage() { cout << "Hello from the function displayMessage.\n"; }
The return type of this function is void. This means that the function does not return a value after execution and returns to the part that calls the program. Because there is no return value, the return statement is not required. When the statement in the function has completed execution and encounters the closed brace of the end function, the program will return automatically.
2. Call function
Calling a function will result in the execution of the function. The function main is called automatically when the program starts, but all other functions must be executed by the function call statement. When a function is called, the program branches to the function and executes the statements in its body.Now let's look at a program that contains two functions: main and displayMessage.
// This program has two functions: main and displayMessage. #include <iostream> using namespace std; void displayMessage();//statement //mian function int main() { cout << "Hello from main.\n"; displayMessage(); // Call.displayMessage cout << "Now we are back in the main function again. \n"; return 0; } void displayMessage() { cout << "Hello from the displayMessage function.\n"; }
Like all C + + programs, this program is executed from the main function, and other functions are executed only when they are called. In the above program, the function displayMessage is called by the following statement in the main function:Hello from main. Hello from the displayMessage function. Now we are back in the main function again.
Notice the form of the function call, which is just the name of the function, followed by a set of parentheses and semicolons. Now use it to compare with the function header:displayMessage();
- Function header - > void displaymessage;
- Function call - > displaymessage();
A function call is a statement that executes the function, so it terminates with a semicolon like all other C + + statements.
Now let's take a look at the execution flow of the above program. Of course, it starts with the main function. When a statement calling the displayMessage function is encountered, the program branches to the function and executes its statement. Once the displayMessage completes execution, the program will return to the main function and continue to execute the statement after the function call line, as shown in Figure 2.
Figure 2 program execution flow
Function call statements can be used in control structures such as loops, if statements, and switch statements. For example, the following program puts the displayMessage function call in the loop
Note that function calls do not include return types.
Some people may wonder, what is the purpose of the sixth line statement in the above program? It is called function prototype. Its task is very simple. It is to let the compiler know that this function will appear later in the program. It looks like a function header, but it's actually a statement, so it ends with a semicolon.Now let's take a look at the execution flow of the above program. Of course, it starts with the main function. When a statement calling the displayMessage function is encountered, the program branches to the function and executes its statement. Once the displayMessage completes execution, the program will return to the main function and continue to execute the statement after the function call line, as shown in Figure 2.
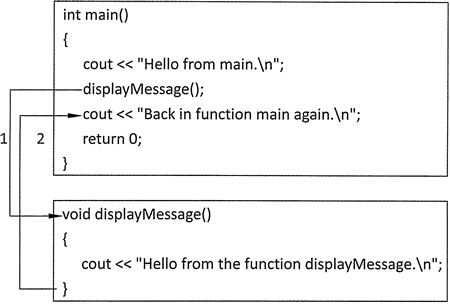
Figure 2 program execution flow
Function call statements can be used in control structures such as loops, if statements, and switch statements. For example, the following program puts the displayMessage function call in the loop
#include <iostream> using namespace std; // Function prototype void displayMessage(); int main() { cout << "Hello from main.\n"; for (int count = 0; count < 3; count++) { displayMessage() ; // Call displayMessage } cout << "Back in function main again.\n"; return 0; } void displayMessage() { cout << "Hello from the function displayMessage.\n"; }
Program output results:
Program output results:
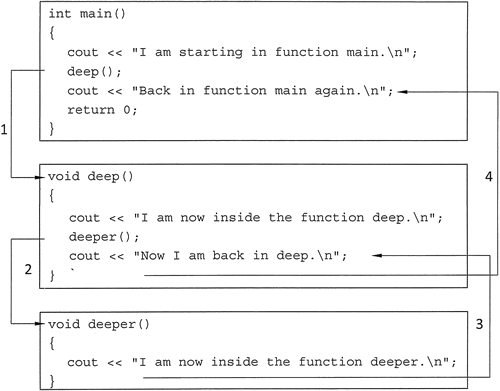
Figure 3 program execution path of calling function in hierarchical form
There can be many functions and function calls in the program, and nested calls can also be made between functions. As shown in the following program, it has three functions: main, deep and deep:Hello from main. Hello from the displayMessage function. Hello from the displayMessage function. Hello from the displayMessage function. Back in function main again.
#include <iostream> using namespace std; // Function prototype void deep(); void deeper(); int main() { cout << "work am starting in function main.\n"; deep(); // Call function deep cout << "Now I amback in function main again.\n"; return 0; } void deep() { cout << "I am now inside the function deep.\n"; deeper(); // Call function deeper cout << "Now I am back in deep. \n"; } void deeper() { cout << "I am now inside the function deeper.\n"; }
In this program, the function main only calls the function deep. Then, deep is called by deep, and the path adopted by the program is shown in Figure 3.I am starting in function main. I am now inside the function deep. I am now inside the function deeper. Now I am back in deep. Now I am back in function main again.
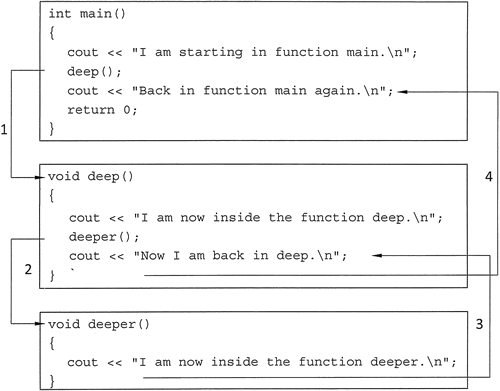
Figure 3 program execution path of calling function in hierarchical form
Original link: C + + templates and generic programming (biancheng.net)