C + + learning notes
----Standard template library STL
I Standard template library
- introduce
The standard template library implements the commonly used data structures (vector encapsulated array, list encapsulated linked list, map and set encapsulated binary tree, etc.) and the basic algorithms for operating these data structures (insertion, sorting, deletion, search, etc.) in the form of class templates in C + + for reuse, reflecting the idea of generic programming. - form
(1) Container
The container manages several objects of a certain type in the form of common data structures, including list (linked list), vector (similar to dynamic array), deque (double ended queue), set, map, queue, stack, etc. Containers are implemented with class templates. Container managed objects are also called elements, and their types can be arbitrary.
(2) Algorithm
The algorithm manipulates elements in various containers through iterators. For example, find is used to find elements equal to a specific value in the container, and for_each is used to apply a function to each element in the container, sort is used to sort the elements in the container, and so on. Each algorithm can be used for all containers, and the algorithm is implemented with function template.
(3) Iterator
An iterator is an object that can access elements traversing all different types of containers in the same way. An iterator indicates a specific location in the container, and the algorithm uses the iterator to locate and manipulate the elements in the container.
II iterator
- Each container in STL has an iterator member named iterator whose type is determined by the container class name < type name >. To access the elements of a container with an iterator, you must first define an iterator variable. The format of the iterator variable definition is:
Container class name<Type name>::iterator Iterator name; Container class name<Type name>::reverse_iterator Iterator name;
vector<int> vec; vector<int>::iterator it = vec.begin();
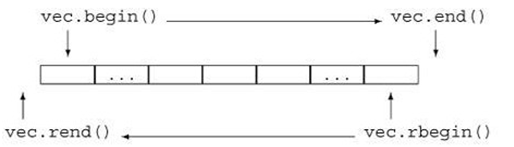
- Usage:
Similar pointer:
begin() Returns an iterator pointing to the first element end() Returns an iterator pointing to the last element rbegin() Returns an inverse iterator pointing to the last element rend() Returns an inverse iterator pointing to the position before the first element Iterator overloaded*, ++, --, ==, !=, =Operator: operator *Returns the element value at the current location operator ++, operator --Let the iterator point to the next, previous element operator ==, operator !=Determines whether two iterators point to the same element operator =Assign values to iterators
III vector container
-
brief introduction
(1) The vector container simulates dynamic arrays and supports random access.
(2) The vector container class can automatically reallocate when the memory is insufficient, and supports reallocation.
(3) The element of the vector container class can be any type of T, but the assignment and copy functions need to be defined.
(4) The elements of the vector container class support iterator access and subscript access.
(5) Header file #include < vector >
-
operation
(1) Tectonics and Deconstruction
(2) Non variable operation
(3) Assignment operation
(4) Element access
(5) Insert delete element
#include<iostream> #include<vector> using namespace std; int main() { vector<int> vec1; //The vec1 object is initially empty vector<int> vec2(10, 6); //vec2 initially has 10 elements with a value of 6 vector<int> vec3(vec2.begin(), vec2.begin()+3); //In the copy construction, vec3 initially has three elements with a value of 6 vector<int>::iterator i; //Declaration iterator i //Display data in vec1 from front to back cout << "vec1.begin()--vec1.end():" << endl; for(i=vec1.begin(); i!=vec1.end(); ++i) cout << *i << " "; cout << endl; //Display data in vec2 from front to back cout << "vec2.begin()--vec2.end():" << endl; for(i=vec2.begin(); i!=vec2.end(); ++i) cout << *i << " "; cout << endl; //Display data in vec3 from front to back cout << "vec3.begin()--vec3.end():" << endl; for(i=vec3.begin(); i!=vec3.end(); ++i) cout << *i << " "; cout << endl; //Test adding and inserting member functions. vector does not support inserting from the front vec1.push_back(2); //Add a member from behind vec1.push_back(4); //Insert 5 in the first position of vec1 vec1.insert(vec1.begin()+1, 5); //Insert all members of vec3 from the first position of vec1 vec1.insert(vec1.begin()+1, vec3.begin(), vec3.end()); cout << "after push() and insert() now the vec1 is: "; for(i=vec1.begin(); i!=vec1.end(); ++i) cout << *i << " "; cout << endl; //Test the assignment member function, re assign the value to vec2, and the initial value of 8 members is 1 vec2.assign(8, 1); cout << "vec2.assign(8, 1): " << endl; for(i=vec2.begin(); i!=vec2.end(); ++i) cout << *i << " "; cout << endl; //Test reference class function, vec1 the zeroth member cout << "vec1.front()=" << vec1.front() << endl; //Last member of vec1 cout << "vec1.back()=" << vec1.back() << endl; //The fifth member of vec1 cout << "vec1.at(4)=" << vec1.at(4) << endl; cout << "vec1[4]=" << vec1[4] << endl; //Test move out and delete, and move the last member out of vec1 vec1.pop_back(); //Delete member vec1.erase(vec1.begin()+1, vec1.end()-2); //Displays the status information of the sequence cout << "vec1.pop_back() and vec1.erase():" << endl; for(i=vec1.begin(); i!=vec1.end(); ++i) cout << *i << " "; cout << endl; //Print number of members cout << "vec1.size()=" << vec1.size() << endl; //empty cout << "vec1.empty():" << vec1.empty() << endl; return 0; }
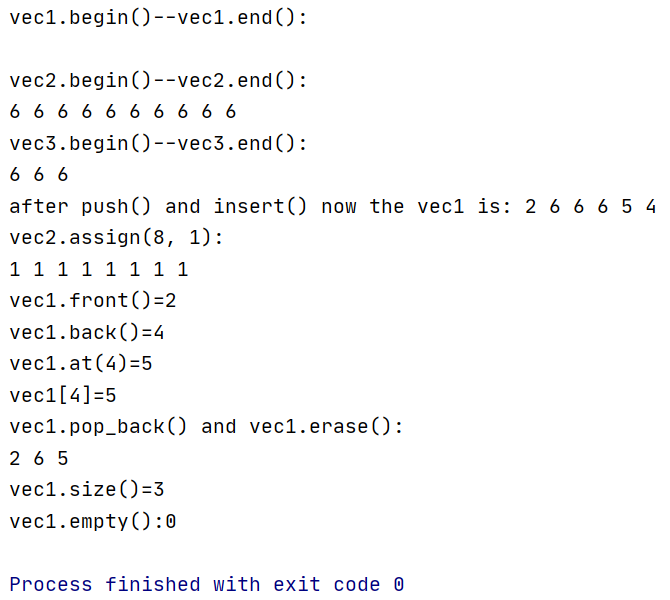
#include<iostream> #include<vector> #include<string> #include<algorithm> using namespace std; int main() { vector<string> sentence; sentence.reserve(5); //Reserve memory for five elements to avoid reallocation //Add element sentence.push_back("Hello, "); sentence.push_back("how"); sentence.push_back("are"); sentence.push_back("you"); sentence.push_back("?"); vector<string>::iterator it; for(it=sentence.begin(); it<sentence.end(); it++) cout << *it << " "; cout << endl; cout << "max_size(): " << sentence.max_size() << endl; cout << "size(): " << sentence.size() << endl; cout << "capacity(): " << sentence.capacity() << endl; //Swap the second and fourth elements swap(sentence[1],sentence[3]); //insert sentence.insert(find(sentence.begin(),sentence.end(),"?"),"always"); sentence.back()="!"; for(it=sentence.begin(); it<sentence.end(); it++) cout << *it << " "; cout << endl; cout << "max_size(): " << sentence.max_size() << endl; cout << "size(): " << sentence.size() << endl; cout << "capacity(): " << sentence.capacity() << endl; return 0; }
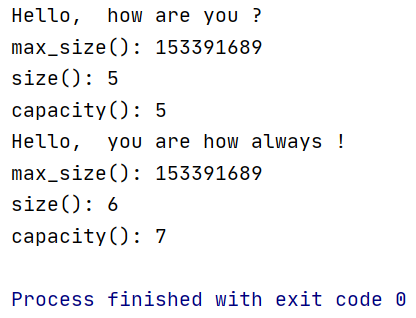
IV List container
- brief introduction
(1) The list container class uses a two-way linked list to manage elements and does not support random access (no subscript).
(2) The element of the list container class can be any type T, but the assignment and copy functions need to be defined.
(3) The elements of the list container class can only be accessed with iterators.
(4) Header file #include < list >
- operation
(1) Structure
(2) Non variable operation
(3) Assignment operation
(4) Element access
(5) Insert delete element
(6) Other special operations
#include <iostream> #include <list> using namespace std; int main(){ list<char> coll; //append elements from 'a' to 'z' for(char c='a'; c<='z'; ++c) coll.push_back(c); list<char>::const_iterator pos; for(pos=coll.begin(); pos!=coll.end(); ++pos) cout << *pos << ' '; cout << endl; return 0; }

#include <iostream> #include <list> #include <algorithm> using namespace std; void printLists(const list<int>& l1, const list<int>& l2){ list<int>::const_iterator it; cout << "list1: "; for(it=l1.begin(); it!=l1.end(); it++) cout << *it << " "; cout << endl << "List2: "; for(it=l2.begin(); it!=l2.end(); it++) cout << *it << " "; cout << endl << endl; } int main(){ list<int> list1, list2; for(int i=0; i<6; i++){ list1.push_back(i); list2.push_front(i); } printLists(list1, list2); //insert all elements of list1 before the first element with value 3 of list2 //find() returns an iterator to the first element with value 3 list2.splice(find(list2.begin(), list2.end(), 3), list1); printLists(list1, list2); //move first element to the end list2.splice(list2.end(), list2, list2.begin()); printLists(list1, list2); //sort second list, assign to list1 and remove duplicates list2.sort(); list1 = list2; list2.unique(); printLists(list1, list2); //merge both sorted lists into the first list list1.merge(list2); printLists(list1, list2); return 0; }
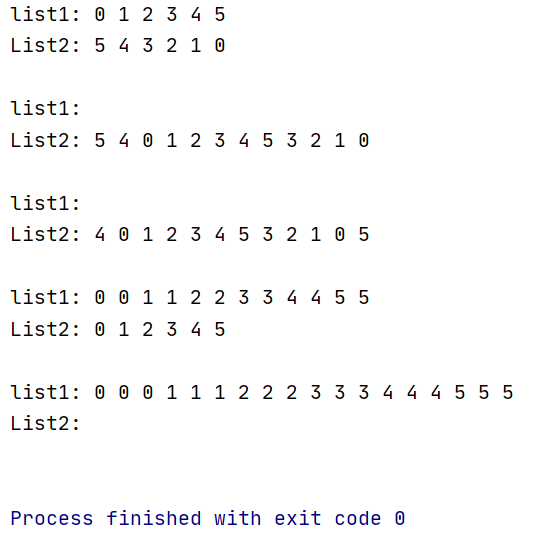
V Stack container
- brief introduction
(1) Last in, first out.
(2) Iterators are not supported.
(3) Header file #include < stack >
- operation
(1) push(value): push elements onto the stack
(2) top(): returns the top element of the stack without deleting it
(3) pop(): deletes the top element of the stack, but does not return
(4) size(): returns the number of stack elements
(5) empty(): judge whether the container is empty
#include <iostream> #include <stack> using namespace std; int main(){ stack<int> st; //push three elements into the stack st.push(1); st.push(2); st.push(3); //top and pop cout << st.top() << ' '; st.pop(); cout << st.top() << ' ' << endl; st.pop(); //modify st.top() = 77; //push st.push(4); st.push(5); //only pop st.pop(); //top, pop and cout while(!st.empty()){ cout << st.top() << ' '; st.pop(); } cout << endl; return 0; }
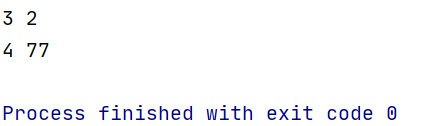
Vi Queue container
- brief introduction
(1) First in first out
(2) Iterators are not supported
(3) Header file #include < queue >
- operation
(1) push(value): put the element in the queue
(2) front(): returns the queue header element without deleting it
(3) back(): returns the tail element of the queue without deleting it
(4) pop(): deletes the queue header element, but does not return
(5) size(): returns the number of stack elements
(6) empty(): judge whether the container is empty
#include <iostream> #include <queue> #include <string> using namespace std; int main(){ queue<string> q; //push q.push("These "); q.push("are "); q.push("more than "); //front and pop cout << q.front(); q.pop(); cout << q.front(); q.pop(); //push q.push("four "); q.push("words!"); //pop to skip q.pop(); cout << q.front(); q.pop(); cout << q.front() << endl; q.pop(); //size cout << "Number of elements in the queue: " << q.size() << endl; return 0; }
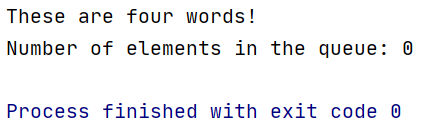
VII Map container
- brief introduction
(1) Map is an associated container of STL. It provides one-to-one data processing capability (the first can be called a keyword, each keyword can only appear once in the map, and the second can be called the value of the keyword).
(2) Support iterators
(3) Header file #include < Map >
- operation
(1) clear(): delete all elements of a map container
(2) Erase (keyword value): delete elements by keyword
(3) Count (keyword value): returns the number of elements corresponding to a keyword
(4) Find (keyword value): returns an iterator pointing to an element corresponding to a keyword
(5) insert(): inserts one or more elements into the container
(6) size(): returns the number of map container elements
(7) empty(): determines whether the map is empty
#include <iostream> #include <map> #include <string> using namespace std; int main(){ map<int, string> mapStudent; pair<map<int, string>::iterator ,bool> Insert_Pair; //first method of insert Insert_Pair = mapStudent.insert(pair<int, string>(1, "student_one")); if(Insert_Pair.second==true) cout << "Insert successfully" << endl; else cout << "Insert Failure" << endl; //second method of insert mapStudent.insert(map<int, string>::value_type(2, "student_two")); //third method of insert mapStudent[3] = "student_three"; map<int, string>::iterator iter; iter = mapStudent.find(1); if(iter!=mapStudent.end()) cout << "Find, the value is " << iter->second << endl; else cout << "Do not find" << endl; for(iter=mapStudent.begin(); iter!=mapStudent.end(); iter++) cout << iter->first << " " << iter->second << endl; return 0; }
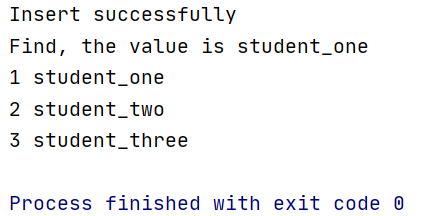
VIII STL algorithm
- brief introduction
(1) STL provides some standard algorithms in the form of function templates to deal with elements in containers
(2) The algorithm processes the elements in the container through iterators. The first two parameters of all algorithms are a pair of iterators: [first, last], which are used to indicate the elements within a semi open interval in the container
(3) Header file #include < algorithm > - type
(1) Non modified algorithm
(2) Modified algorithm
(3) Delete algorithm
(4) Mutation algorithm
(5) Sorting algorithm
Algorithm for sorting interval:
(6) Digital algorithm
- sort() algorithm
The sort algorithm in STL automatically selects the insertion sort or quick sort algorithm according to the number of sorting elements. It is a function template, sort(begin, end, cmp). The meaning of the three parameters is:
- The first parameter is the iterator position of the first element, which can be the first address of the array.
- The second parameter is the iterator position of the last element, which can be the address of the last element in the array.
- The third parameter is the address of the comparison function, indicating the sorting method. The third parameter can be defau lt ed, which means that the front element is sorted in an incremental way that is smaller than the rear element. If the element is a user-defined class object, you need to overload the "<" operator in the form of friend or member function in the class.
- The prototype of the comparison function is "bool function name (T & A, T & b)". The return value of the function indicates the condition that element a is ahead of element b. If you want to sort incrementally, it can be expressed as returning true when a < b; To decrement sorting, it can be expressed as returning false when a < b. If the element type is a user-defined class object, the comparison function cannot be defined as a class member function, but only as a static member function or a friend function.
//compare cannot be defined as a member function of Student class bool compare(const Student& s1, const Student& s2){ return s1.name < s2.name; //Sort from small to large } sort(vector1.begin(), vector1.end, compare); //You can also overload < < in the class to sort Student objects incrementally or decrementally
- sort is only applicable to STL's vector, string and deque containers, not set, map and other containers.
- for_ Example of each () algorithm
for_each(beginIterator beg, endIterator end, Proc op)
- Call op(elem) for each element in the interval [beg, end].
- Returns a copy of the container after op.
- op can change elements.
- The return value of op is ignored.
void print(){ ... } void square(int &n){ n=n*n; } vector<it> coll; ......INSERT_ELEMENTS(coll, 1, 9); //call print() for each element for_each(coll.begin(), coll.end(), print); for_each(coll.begin(), coll.end(), square);