1. Write a small program that requires users to use an integer to indicate their height (in inches), and then convert the height into feet and inches. The program uses underscore characters to indicate the input position. In addition, a const symbolic constant is used to represent the conversion factor.
#include "iostream"
using namespace std;
const int inch_to_feet = 12;
int main()
{
cout << "Enter your height (inches):___\b\b\b";
int inch;
cin >> inch;
int feet = inch / inch_to_feet;
inch = inch % inch_to_feet;
cout << "Your height is:" << feet << "Feet plus" << inch << "inch" << endl;
return 0;
}

2. Write a small program to input his height in a few feet and inches and his weight in pounds. (three variables are used to store this information.) This procedure reports the Body Mass Index (BMI). To calculate BMI, the program indicates the user's height in inches (1 foot is 12 inches) and converts the height in inches to the height in meters (1 inch = 0.0254 meters). Then, convert the weight in pounds to the weight in kilograms (1kg = 2.2 pounds). Finally, calculate the corresponding BMI - weight (kg) divided by the square of height (m). Various conversion factors are represented by symbolic constants.
#include "iostream"
using namespace std;
const int trans = 12;
const double inch_to_meter = 0.0254;
const double pound_to_kilogram = 2.2;
int main()
{
cout << "Enter height:" << endl;
cout << "Enter the feet section:_\b";
int feet;
cin >> feet;
cout << "Enter the inch portion:_\b";
int inch;
cin >> inch;
cout << "Enter weight(pound): _\b";
double pound;
cin >> pound;
int height;
height = feet * trans + inch;
double meter;
meter = height * inch_to_meter;
double kilogram;
kilogram =pound / pound_to_kilogram;
cout << endl;
cout << "Your body data are as follows:" << endl;
cout << "Height:" << height << "foot,Namely" << meter << "rice\n";
cout << "weight" << kilogram << "Kilogram\n";
double BMI;
BMI = kilogram / (meter * meter);
cout << "Your body mass index is:"<<BMI << endl;
return 0;
}
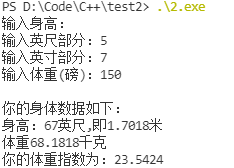
3. Write a program that requires the user to enter a latitude in degrees, minutes and seconds, and then display the latitude in degrees. One degree is 60 minutes, and one minute equals 60 seconds. Please express these values in the form of symbolic constants. For each input value, a separate variable should be used to store it. The following is what happens when the program runs:
Enter a latitude in degrees, minutes, and seconds:
First, enter the degrees: 37
Next, enter the minutes of arc: 51
Finally, enter the seconds of arc: 19
37 degrees, 51 minutes, 19 seconds = 37.8553 degrees
#include "iostream"
using namespace std;
const int degree_to_minutes = 60;
const int minutes_to_seconds = 60;
int main()
{
cout << "Enter a latitude in degrees,minutes,and seconds:\n";
cout<<"First,enter the degrees:";
int degree;
cin>>degree;
cout<<"Next,enter the minutes of arc:";
int minute;
cin>>minute;
cout<<"Fianlly,enter the seconds of arc:";
int second;
cin>>second;
double show_in_degree;
show_in_degree=(double)degree+(double)minute/degree_to_minutes+(double)second/degree_to_minutes/minutes_to_seconds;
cout<<degree<<" degrees,"<<minute<<" minutes,"<<second<<" seconds = "<<show_in_degree<<" degrees\n";
return 0;
}

4. Write a program that requires the user to enter the number of seconds in integer mode (stored with long or long long variable), and then display this time in days, hours, minutes and seconds. Use symbolic constants to represent the number of hours per day, minutes per hour, and seconds per minute. The output of this program should be similar to the following:
Enter the number of seconds: 31600000
31600000 seconds = 365 days, 17 hours, 46 minutes, 40 seconds
#include "iostream"
using namespace std;
const int day_to_hour = 24;
const int hour_to_minute = 60;
const int minute_to_second = 60;
int main()
{
cout << "Enter the number of seconds: ";
long seconds;
cin >> seconds;
int day, hour, minute,second;
day = seconds / minute_to_second / hour_to_minute / day_to_hour;
hour = seconds / minute_to_second / hour_to_minute % day_to_hour;
minute = seconds / minute_to_second % hour_to_minute;
second = seconds % minute_to_second;
cout << seconds << " seconds = " << day << " days," << hour << " hours," << minute << " minutes," << second << " seconds\n";
return 0;
}

5. Write a program that requires users to input the current global population and the current population of the United States (or the population of other countries). Store this information in the long long variable and let the program display the percentage of the world's population in the United States (or other countries). The output of this program should be similar to the following:
Enter the world's population: 6898758899
Enter the population of the US: 310783781
The population of the US is 4.50492% of the world population.
#include "iostream"
using namespace std;
int main()
{
cout << "Enter the world population:__\b\b";
long long world_population;
cin >> world_population;
cout << "Enter the population of the US:__\b\b";
long long US_population;
cin >> US_population;
double proportion;
proportion = (double)US_population / (double)world_population * 100;
cout << "The population of the US is "<<proportion <<"% of the world population."<<endl;
return 0;
}

6. Write a program that requires the user to input the driving mileage (miles) and the amount of gasoline used (gallons), and then point out that the car's fuel consumption is one gallon. If you like, you can also let the program ask the user to enter the distance in kilometers and the amount of gasoline in liters, and then point out the result of European style - that is, the fuel consumption per 100 kilometers (liters).
#include "iostream"
using namespace std;
int main()
{
cout << "Enter fuel consumption (gallons): ";
double gasoline;
cin >> gasoline;
cout << "Enter the distance (miles) that has been driven: ";
int distance;
cin >> distance;
cout << "Your car can run on a gallon of gas" << distance / gasoline << "mile" << endl;
return 0;
}
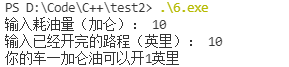
7. Write a program that requires users to input the fuel consumption of the car according to the European style (gasoline consumption (liters) per 100 kilometers), and then convert it to the fuel consumption of the American style - miles per gallon. Note that the US method (distance / fuel) is the opposite of the European method (fuel / distance) except for using different units of measurement. 100 kilometers equals 62.14 miles and a gallon equals 3.785 liters. Therefore, 19mpg is about 12.4l/100km and 27mpg is about 8.71/100km.
#include "iostream"
using namespace std;
int main()
{
cout<<"Enter European style fuel consumption calculation\n"<<"(liters per 100 kilometers):";
double Euro_style;
cin>>Euro_style;
cout<<"Translate into(miles per gallon):"<<endl;
cout<<Euro_style<<" L/100Km = "<<62.14*3.785/Euro_style<<" mpg\n";
return 0;
}
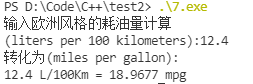