Task 1: use rich text editor
Observe various labels
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Onine</title> <style> .table1{ width: 500px; } .tr1, .tr3{ background-color: gray; } .tr2, .tr4{ background-color: white; } </style> </head> <body> <div> <table class="table1" border="1" cellpadding="0" cellspacing="0" > <tr class="tr1"> <th>full name</th> <th>Gender</th> <th>Age</th> </tr> <tr class="tr2"> <td>zerone</td> <td>female</td> <td>21</td> </tr> <tr class="tr3"> <td>zerone1</td> <td>female</td> <td>24</td> </tr> <tr class="tr4"> <td>zerone2</td> <td>male</td> <td>25</td> </tr> </table> </div> </body>
Click the button to realize the message pop-up window
<input type="button" value="Sign in" onclick="alertTips()"> <script> function alertTips(){ alert("Login succeeded"); } </script>
Extension: CSS box model
-
Deep understanding of css box model
-
Border margin:
-
The border border affects the box size
-
Compound writing of border
border:1px solid red;//No order border-top:1px solid red;
-
-
context content
-
padding inner margin
-
Will affect the box size
-
If the box itself does not specify the width/height attribute, the box size will not be opened
-
Compound writing of padding
-
-
Margin margin
-
It does not affect the box size
-
The compound writing of margin is consistent with padding
-
You can center block level elements horizontally
The first step is to set the box width Part II, order margin:0 auto;
-
The horizontal center of inline blocks and row elements is to add text begin: Center to its parent;
-
-
The problem of merging the vertical outer margins of adjacent block elements: instead of adding two margins, take the large value as the margin. Solution: try to add the margin value to only one box
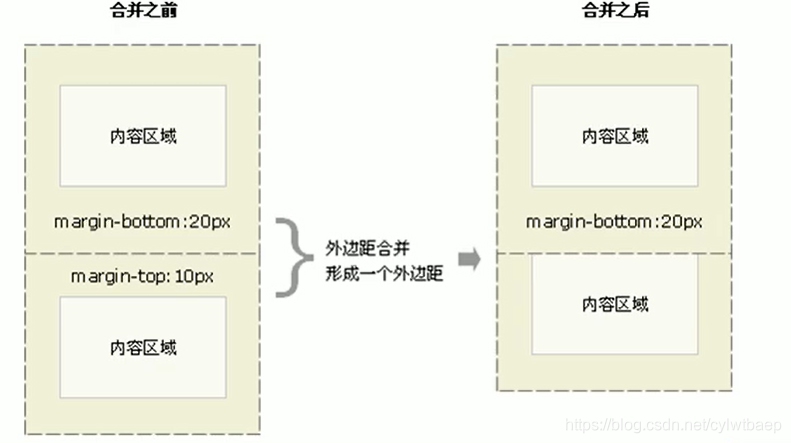
-
- Collapse of vertical outer margin of nested elements
Solution:
1. Border parent element
2. Add an inner margin to the parent element
3. Add overflow: hidden to the parent element;
- float:
The float attribute is used to create a floater and move it to one side until the left or right edge touches the edge containing the block or another floater
Floating characteristics
- Will deviate from the standard flow
- The floating box no longer retains its original position
- No matter what mode the element is originally, it has similar characteristics to the inline block element after adding floating (therefore, if the inline element is set with floating, it does not need to be converted to another mode to set the width and height)
Floating disadvantage
When all the child elements are set to float, the height of the parent element will change to 0, because the float does not occupy a position, which will cause the lower box to move upward
Clear float
Clear the effect of typography caused by floating
-
-
Additional labeling method (w3c Recommended Practice), disadvantages: adding many meaningless labels, poor structure
Adding a block level element after the last floating box is equivalent to adding a wall css Chinese writing clear: both;
-
If the child is not the godfather's fault, css adds overflow: hidden to the parent;
Disadvantages: the overflow part cannot be displayed
[the external chain picture transfer fails. The source station may have an anti-theft chain mechanism. It is recommended to save the picture and upload it directly (img-xm0ofto6-1629440360092) (handwritten. assets/image-20210818153001997.png)]
-
: after pseudo element method, the structure is the clearest. As long as you write the following code, which parent box needs, you can add a space clearfix on the class. Disadvantages: you need to take care of the lower version of the browser
.clearfix:after{ content:""; display:block; height:0; vlear:both; visibiity:hidden; } .clearfix{ *zoom:1;//For compatibility with ie6, 7 }
-
Double pseudo element, the use method is the same as that of single pseudo element
.clearfix:before, .clearfix:after{ content:""; display:table; } .clearfix:after{ clear:both; } .clearfix{ *zoom:1; }
-
location
You can make the box move freely in a box, or fix it in a position on the screen and press a boxPositioning = positioning mode + edge offset
Positioning mode:
-
Static positioning: that is, the default positioning method, which means no positioning
position: static;
-
Relative positioning:
-
Take your original position as the reference system
-
The original position in the standard flow continues to be occupied, and the back box will not float up
position: relative;
-
-
Absolute positioning:
- If there is no ancestor element or the ancestor element is not located, the browser is used as the reference system
- If the ancestor element has positioning (relative, absolute and fixed), the nearest element is used as the reference system
- Absolute positioning no longer occupies its original position
position:absolute;
-
Fixed positioning
- Move the element with the browser's visual window as the reference point
- It has nothing to do with the parent element
- Do not scroll with the scroll bar
- The fixed position no longer occupies the original position
position: fixed;
Algorithm fixed on the right side of the layout Center:
- Make the box in the fixed position left: 50%, and then move it to half of the layout center
- Let the margin left of the fixed box: half the width of the layout center
-
Viscous positioning: it can be regarded as a mixture of fixed positioning and relative positioning
- Take the visual window of the browser as the reference
- Viscous positioning occupies the original position
- One of top, left, right and bottom must be added to be valid
- Poor compatibility, ie does not support
position: sticky;
Positioning stacking order z-index
z-index:Integer;
- The value is an integer. The default value is auto. The larger the value, the higher the value
- If the values are the same, follow the writing order and come from behind
- No unit can be added after the number
- Only the positioned box has the z-index attribute
Absolutely positioned box centered
The box with absolute positioning cannot pass margin: 0 auto Achieve horizontal centering
method:
1. left:50% 2. margin-position:-General size of box
Positioning particularity
- Absolute or fixed positioning is added to the elements in the line, and the height or width can be set directly
- Absolute or fixed positioning is added to block level elements. If width and height are not given, the default is the size of the content
- Floating element. None of the elements with absolute positioning (fixed positioning) will trigger the problem of outer margin merging
- Floating elements will only press the box of the following standard flow, but not the text (because floating is used to surround the text at the beginning)
- Absolute positioning (fixed positioning) will suppress all contents of the following standard flow
- Layout mode
- Normal flow (standard flow)
- float
- location
-
-
Finished css box model layout
html part
<body> <div class="main"> <div class="left"> <div class="box1">1</div> <div class="box2">2</div> </div> <div class="right"> <div class="box3">3 <div class="box7"> 7 </div> <div class="box8"> 8 </div> </div> <div class="box4">4 <div class="box9"> 9 </div> </div> <div class="box5">5</div> <div class="box6">6</div> </div> </div> </body>
css part
<meta charset="UTF-8"> <title>Title</title> <style> *{ padding: 0; margin: 0; } body{ background-color:#fcdd9c ; text-align: center; } .main{ width: 755px; height: 482px; margin: 20px auto; } .box1, .box2, .box3, .box4, .box5, .box6 { border: 1px #aaaaaa solid ; background-color: #c5d08e; } .left{ float: left; width: 233px; height: 482px; } .box1{ float: left; width: 230px; height: 109px; line-height: 109px; } .box2{ float: left; margin-top: 20px; width: 230px; height: 349px; line-height: 349px; } .right{ float: right; width: 507px; height: 482px; } .box3{ position: relative; float: left; width: 505px; height: 187px; line-height: 187px; } .box4{ position: relative; float: left; width: 255px; height: 271px; margin-top: 20px; line-height: 271px; } .box5{ float: right; width: 232px; height: 124px; margin-top: 20px; line-height: 124px; } .box6{ float: right; width: 232px; height: 125px; margin-top: 20px; line-height: 125px; } .box7, .box8{ background-color: #f3a464; } .box7{ position: absolute; top: 28px; left: 20px; width: 167px; height: 129px; line-height: 129px; } .box8{ position: absolute; right: 0px; bottom: 150px; margin-right: 20px; width: 167px; height: 129px; line-height: 129px; } .box9{ position: absolute; z-index: -1; left: 20px; top: 237px; margin-right: 20px; width: 167px; height: 129px; line-height: 129px; background-color: #e4bc91; }
Self test
1.HTML5 what attributes need to be added to make img elements drag and drop
draggable="true";
2.HTML.5 which input type can select a date selector without time zone?
<input type="time"> Allow user to select time (no time zone)
3. What do Margin, Border and padding mean in the CSS sub model?
margin: Margin border: frame padding: padding
4. Name at least five common HTML events
window event form event keyboard event mouse event media event
five Н What types of attributes are onblur and onfocus of TML?
form event
6. How to set the value of the display attribute to make the container elastic?
display: flex;
7. How many different types of loops are there in JavaScript?
3 Species cycle for,do...while,while
8. After a user searches for a word, JavaScrip t highlights the searched word. Which semantic tag is the most appropriate?
mark