A friend who knows the official account shares a question with me. I think it is very interesting. I realized it with Python. You can think about it together.
Firstly, this problem is analyzed from the perspective of statistics. Assuming that two dice are independent, roll the dice once, and the possible points of each sieve are integers from 1 to 6. There are 36 possibilities in total: the yellow ones are the six cases where the sum of points is 7, and it can be found that the probability of winning is 6 / 36 ≈ 0.16667. That is, 16.667% probability that the player will win 4 yuan and 83.33% probability that the player will lose 1 yuan.
Simulate the process with python and let the computer cycle 100000 times to see how many times the sum of the points of the two dice is 7. The specific statements are as follows:
from random import * #Import random module count = 0 #Initialize the count with the sum of sieves as 7 for i in range(100000): num1 = randint(1, 6) #Generate a random number between 1 and 6 as the number of sieve 1 num2 = randint(1, 6) #Generate a random number between 1 and 6 as the number of sieve 2 if num1 + num2 == 7: count += 1 #If the sum of the values thrown by the two sieves is 7, the count is increased by 1 print('10 In 10000 throwing, the probability that the sum of the throwing values of the two sieves is 7 is:', count/100000*100,'%') #Output print results
Run the code 5 times and the results are:
10 In 10000 throws, the probability that the sum of the throwing values of the two sieves is 7 is 16.743 % 10 In 10000 throws, the probability that the sum of the throwing values of the two sieves is 7 is 16.974 % 10 In 10000 throws, the probability that the sum of the throwing values of the two sieves is 7 is 16.583000000000002 10 In 10000 throws, the probability that the sum of the throwing values of the two sieves is 7 is 16.445 10 In 10000 throws, the probability that the sum of the throwing values of the two sieves is 7 is 16.900000000000002 %
It can be found that the difference between the results of computer simulation and statistical analysis is no more than 0.01. Further try. If the number of points obtained in the "lucky 7" game is 7 and the amount of money users win is set, can the player be in the state of winning money after multiple rounds (assuming 100000 rounds)? The specific statements are as follows:
from random import * #Import random module for j in range(2,10): y_money = 0 #Initialize winning amount s_money = 0 #Initialize the amount of money lost count = 0 #Initialize the count with the sum of sieves as 7 final_money = 0 #Initialize total amount print('###############################') print('If the sum of points is 7, the winning amount is:', j) #Print the current winning limit value for i in range(100000): num1 = randint(1, 6) #Generate a random number between 1 and 6 as the number of sieve 1 num2 = randint(1, 6) #Generate a random number between 1 and 6 as the number of sieve 2 if num1 + num2==7: count += 1 #If the sum of the values thrown by the two sieves is 7, the count is increased by 1 y_money += j #If the sum of the values thrown by the two sieves is 7, the winning account plus j else: s_money += 1 #If the sum of the values thrown by the two sieves is not 7, add 1 to the money losing account final_money = y_money - s_money #Total account amount after cycle print('Total amount at this time:', final_money) #Print the total amount of the account if final_money>0: print('When the number of points is 7, the winning amount is:',j, ',At this time, players can win money') #If the total amount of the account is greater than 0, it means that the user can win money at this time else: print('When the number of points is 7, the winning amount is:',j, ',At this time, the player will lose money')
Run the code 5 times and the results are:
Result 1 ############################### If the sum of points is 7, the winning amount is 2 Total amount at this time:-50458 When the number of points is 7, the winning amount is 2 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 3 Total amount at this time:-33580 When the number of points is 7, the winning amount is 3 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 4 Total amount at this time:-16690 When the number of points is 7, the winning amount is 4 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 5 Total amount at this time:-736 When the number of points is 7, the winning amount is 5 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 6 Total amount at this time: 17586 When the number of points is 7, the winning amount is 6 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 7 Total amount at this time: 33456 When the number of points is 7, the winning amount is 7 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 8 Total amount at this time: 50813 When the number of points is 7, the winning amount is 8 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 9 Total amount at this time: 65060 When the number of points is 7, the winning amount is 9 ,At this time, players can win money
Result 2 ############################### If the sum of points is 7, the winning amount is 2 Total amount at this time:-49267 When the number of points is 7, the winning amount is 2 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 3 Total amount at this time:-33752 When the number of points is 7, the winning amount is 3 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 4 Total amount at this time:-16750 When the number of points is 7, the winning amount is 4 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 5 Total amount at this time: 206 When the number of points is 7, the winning amount is 5 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 6 Total amount at this time: 16886 When the number of points is 7, the winning amount is 6 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 7 Total amount at this time: 32480 When the number of points is 7, the winning amount is 7 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 8 Total amount at this time: 50012 When the number of points is 7, the winning amount is 8 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 9 Total amount at this time: 67170 When the number of points is 7, the winning amount is 9 ,At this time, players can win money
Result 3 ############################### If the sum of points is 7, the winning amount is 2 Total amount at this time:-49786 When the number of points is 7, the winning amount is 2 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 3 Total amount at this time:-32784 When the number of points is 7, the winning amount is 3 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 4 Total amount at this time:-17415 When the number of points is 7, the winning amount is 4 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 5 Total amount at this time: 1070 When the number of points is 7, the winning amount is 5 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 6 Total amount at this time: 16466 When the number of points is 7, the winning amount is 6 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 7 Total amount at this time: 32184 When the number of points is 7, the winning amount is 7 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 8 Total amount at this time: 49382 When the number of points is 7, the winning amount is 8 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 9 Total amount at this time: 67850 When the number of points is 7, the winning amount is 9 ,At this time, players can win money
Result 4 ############################### The sum of the winning points is 7, if the sum is 7 Total amount at this time:-50068 When the number of points is 7, the winning amount is 2 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 3 Total amount at this time:-33016 When the number of points is 7, the winning amount is 3 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 4 Total amount at this time:-17170 When the number of points is 7, the winning amount is 4 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 5 Total amount at this time: 284 When the number of points is 7, the winning amount is 5 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 6 Total amount at this time: 17376 When the number of points is 7, the winning amount is 6 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 7 Total amount at this time: 33936 When the number of points is 7, the winning amount is 7 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 8 Total amount at this time: 49472 When the number of points is 7, the winning amount is 8 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 9 Total amount at this time: 66790 When the number of points is 7, the winning amount is 9 ,At this time, players can win money
Result 5 ############################### The sum of the winning points is 7, if the sum is 7 Total amount at this time:-49876 When the number of points is 7, the winning amount is 2 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 3 Total amount at this time:-33372 When the number of points is 7, the winning amount is 3 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 4 Total amount at this time:-15535 When the number of points is 7, the winning amount is 4 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 5 Total amount at this time:-292 When the number of points is 7, the winning amount is 5 ,At this time, the player will lose money ############################### If the sum of points is 7, the winning amount is 6 Total amount at this time: 16403 When the number of points is 7, the winning amount is 6 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 7 Total amount at this time: 33984 When the number of points is 7, the winning amount is 7 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 8 Total amount at this time: 49733 When the number of points is 7, the winning amount is 8 ,At this time, players can win money ############################### If the sum of points is 7, the winning amount is 9 Total amount at this time: 65720 When the number of points is 7, the winning amount is 9 ,At this time, players can win money
Analyzing the results of five code runs, we know that if the sum of points is 7 and the amount of money won is 4, the player will lose money at this time. The amount of money to win is 5. At this time, players may lose money or win money. The number of times to lose money is 2 and the number of times to win money is 3. If the winning amount is greater than or equal to 6, the player will win.
From the Python simulation results, it can be concluded that if the sum of points is 7, you win 4 yuan, not 1 yuan. This rule is unfair. If you play enough times, the final result is that the player loses money. If the amount of money to win is set to 6, it can ensure that players are in the state of winning money after multiple rounds (100000 rounds).
Interested friends can adjust the winning amount (the step size is set to decimal) and the number of rounds, and analyze whether the results will be different.
You may be interested in:
Drawing Picchu in Python
Drawing word cloud with Python
Python face recognition - I only have eyes for you
Python draws a beautiful picture of the starry sky (beautiful background)
[Python] Valentine's Day confession Fireworks (with sound and text)
Operate neo4j with py2neo Library in Python to build the correlation map
Python romantic confession source code collection (love, roses, photo wall, advertisement under the stars)
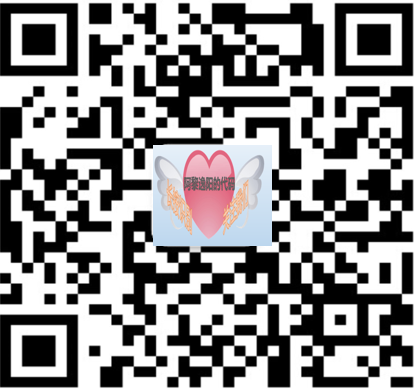