- 1. Overview of combination mode
- 2. Structure and implementation of combination mode
- 3. Application examples of combination mode
- 4. Transparent combination mode and security combination mode
- 5. Advantages, disadvantages and applicable environment of combination mode
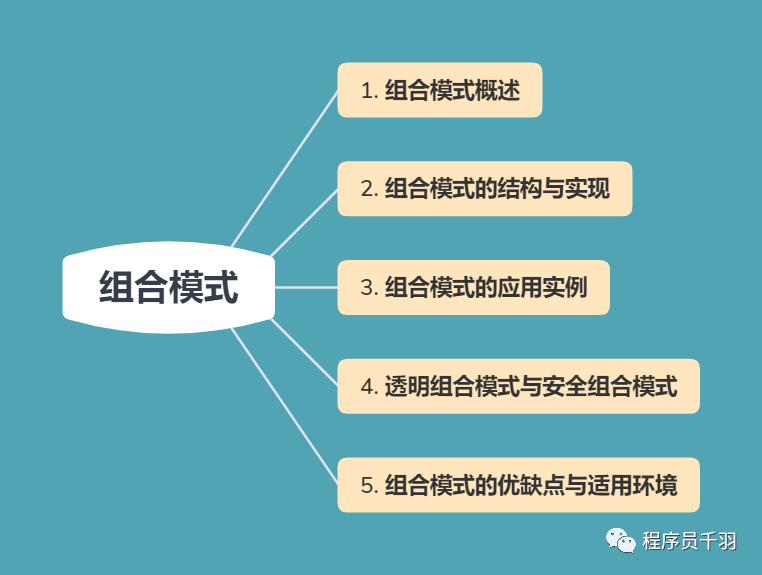
"Github: https://github.com/nateshao/design-demo/tree/main/JavaDesignPatterns/10-bridge
1. Overview of combination mode
Windows operating system directory structure
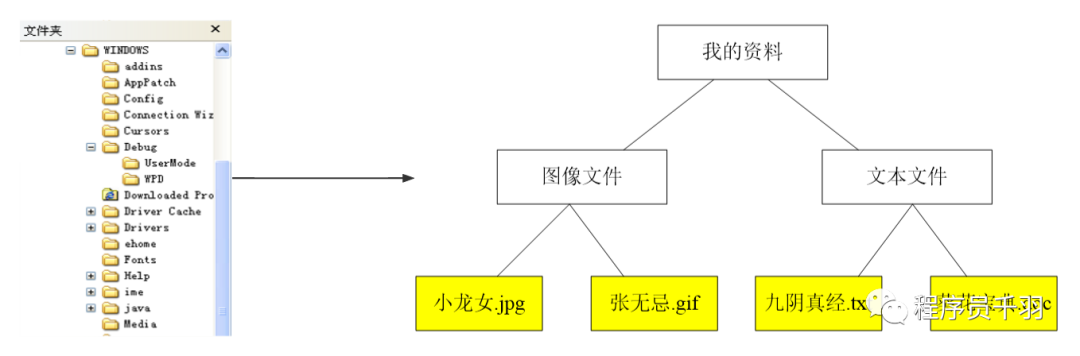
analysis
- In the tree directory structure, there are two different elements: files and folders
- You can include files in a folder and continue to include subfolders
- You can no longer include sub files or sub folders in a file
- Folder < --- > container
- File < --- > leaf
analysis
- When a method of a container object is called, it will traverse the whole tree structure, find the member object that also contains this method, call and execute it, and move a hundred. The recursive call mechanism is used to process the whole structure
- Due to the functional differences between container objects and leaf objects, container objects and leaf objects must be treated differently in the code using these objects. In fact, in most cases, the client wants to deal with them consistently, because the differential treatment of these objects will make the program very complex
How are container objects and leaf objects treated consistently?
"Combination mode enables users to consistently handle the whole tree structure or part of the tree structure through an ingenious design scheme. It describes how to recursively combine container objects and leaf objects, so that users can treat container objects and leaf objects consistently without distinguishing them.
definition
"Composite mode: multiple objects are combined to form a tree structure to represent a hierarchical structure with part whole relationship. Composite mode allows clients to treat single objects and composite objects uniformly.
Object structured pattern
- Also known as "part whole" mode
- Organizing objects into a tree structure can be used to describe the relationship between whole and part
Structure of combination mode
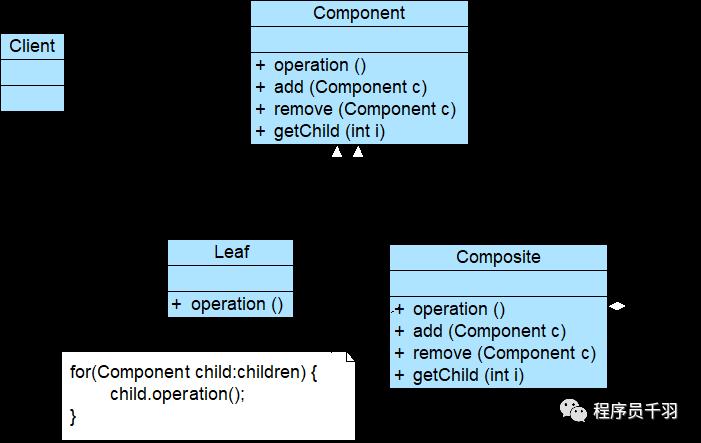
The composite mode contains the following three roles:
- Component (abstract component)
- Leaf (leaf component)
- Composite (container component)
2. Structure and implementation of combination mode
Typical code of abstract component role:
AbstractFile.java
package com.nateshao.composite; /** * @date Created by Shao Tongjie on 2021/10/20 20:34 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public abstract class AbstractFile { public abstract void add(AbstractFile file); public abstract void remove(AbstractFile file); public abstract AbstractFile getChild(int i); public abstract void killVirus(); }
VideoFile.java
package com.nateshao.composite; /** * @date Created by Shao Tongjie on 2021/10/20 20:34 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class VideoFile extends AbstractFile { private String name; public VideoFile(String name) { this.name = name; } public void add(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public void remove(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public AbstractFile getChild(int i) { System.out.println("Sorry, this method is not supported!"); return null; } public void killVirus() { //Simulated antivirus System.out.println("----For video files'" + name + "'Kill virus"); } }
TextFile.java
package com.nateshao.composite; /** * @date Created by Shao Tongjie on 2021/10/20 20:35 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class TextFile extends AbstractFile{ private String name; public TextFile(String name) { this.name = name; } public void add(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public void remove(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public AbstractFile getChild(int i) { System.out.println("Sorry, this method is not supported!"); return null; } public void killVirus() { //Simulated antivirus System.out.println("----For text files'" + name + "'Kill virus"); } }
ImageFile.java
package com.nateshao.composite; /** * @date Created by Shao Tongjie on 2021/10/20 20:37 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class ImageFile extends AbstractFile { private String name; public ImageFile(String name) { this.name = name; } public void add(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public void remove(AbstractFile file) { System.out.println("Sorry, this method is not supported!"); } public AbstractFile getChild(int i) { System.out.println("Sorry, this method is not supported!"); return null; } public void killVirus() { //Simulated antivirus System.out.println("----For image files'" + name + "'Kill virus"); } }
Folder.java
package com.nateshao.composite; import java.util.ArrayList; /** * @date Created by Shao Tongjie on 2021/10/20 20:38 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class Folder extends AbstractFile { //Define a collection fileList to store members of AbstractFile type private ArrayList<AbstractFile> fileList = new ArrayList<AbstractFile>(); private String name; public Folder(String name) { this.name = name; } public void add(AbstractFile file) { fileList.add(file); } public void remove(AbstractFile file) { fileList.remove(file); } public AbstractFile getChild(int i) { return (AbstractFile) fileList.get(i); } public void killVirus() { System.out.println("****To folder'" + name + "'Kill virus"); //Simulated antivirus //Recursively call the killVirus() method of the member component for (Object obj : fileList) { ((AbstractFile) obj).killVirus(); } } }
Client.java
package com.nateshao.composite; /** * @date Created by Shao Tongjie on 2021/10/20 20:39 * @WeChat official account programmers * @Personal website www.nateshao.com cn * @Blog https://nateshao.gitee.io * @GitHub https://github.com/nateshao * @Gitee https://gitee.com/nateshao * Description: */ public class Client { public static void main(String args[]) { //Programming for abstract components AbstractFile file1, file2, file3, file4, file5, folder1, folder2, folder3, folder4; folder1 = new Folder("Sunny Information"); folder2 = new Folder("image file"); folder3 = new Folder("text file"); folder4 = new Folder("video file "); file1 = new ImageFile("little dragon maiden.jpg"); file2 = new ImageFile("zhang wuji.gif"); file3 = new TextFile("The nine Yin manual.txt"); file4 = new TextFile("Sunflower collection.doc"); file5 = new VideoFile("Xiaoao Jianghu.rmvb"); folder2.add(file1); folder2.add(file2); folder3.add(file3); folder3.add(file4); folder4.add(file5); folder1.add(folder2); folder1.add(folder3); folder1.add(folder4); //Start the anti-virus operation from the "Sunny's data" node folder1.killVirus(); } }
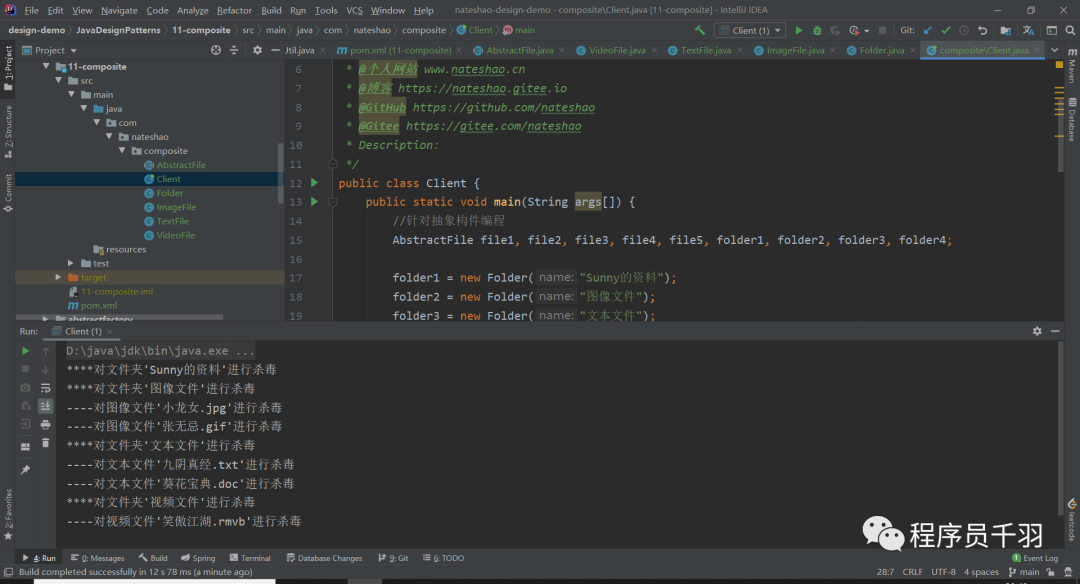
3. Application examples of combination mode
Example description
"A software company wants to develop an anti-virus software, which can kill both a folder and a specified file. The anti-virus software can also provide different anti-virus methods for different types of files according to the characteristics of various files, such as image file and text file The way of anti-virus is different. Now we use the combination mode to design the overall framework of the anti-virus software.
Instance class diagram:
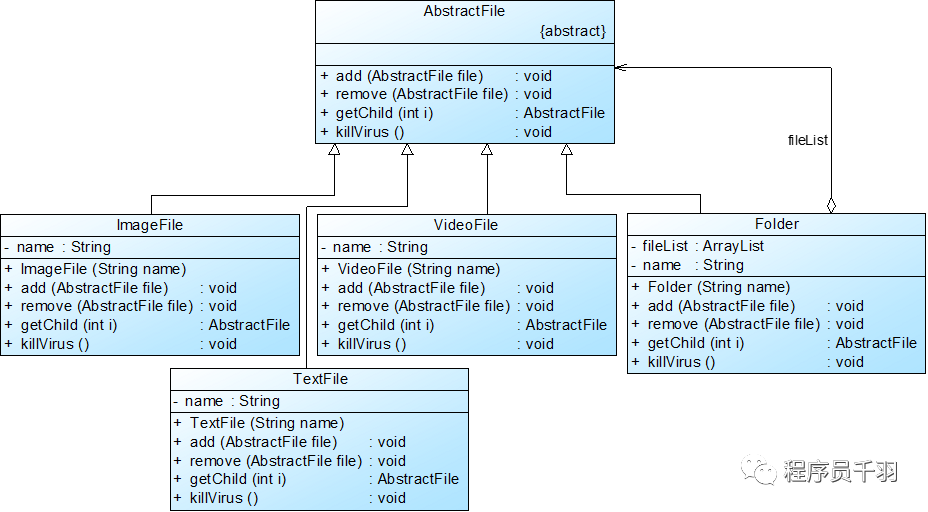
Anti virus software framework design structure diagram
Example code
- AbstractFile: Abstract file class, which acts as an abstract component class
- ImageFile: an image file class that acts as a leaf component class
- TextFile: a text file class that acts as a leaf component class
- VideoFile: a video file class that acts as a leaf component class
- Folder: a folder class that acts as a container component class
- Client: client test class
Results and analysis
If the operation node needs to be replaced, for example, only the "text file" of the folder is antivirus, the client code only needs to be modified by one line, for example:
folder1.killVirus();
Replace with:
folder3.killVirus();
In the specific implementation, a graphical interface can be created for users to select the root node of the required operation without modifying the source code, which conforms to the opening and closing principle
4. Transparent combination mode and security combination mode
Transparent combination mode
- The abstract Component component declares all methods for managing member objects, including add(), remove(), and getChild()
- In the client's view, the methods provided by the leaf object and the container object are consistent, and the client can treat all objects consistently
- The disadvantage is that it is not safe enough, because leaf objects and container objects are different in nature
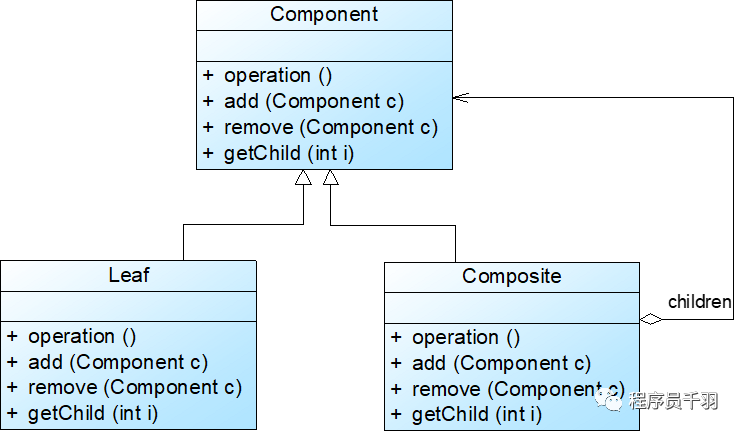
Safe combination mode
- The abstract Component component does not declare any methods for managing member objects, but declares and implements these methods in the Composite class
- For leaf objects, it is impossible for the client to call these methods
- The disadvantage is that it is not transparent enough, and the client can not program completely for abstraction. Leaf components and container components must be treated differently
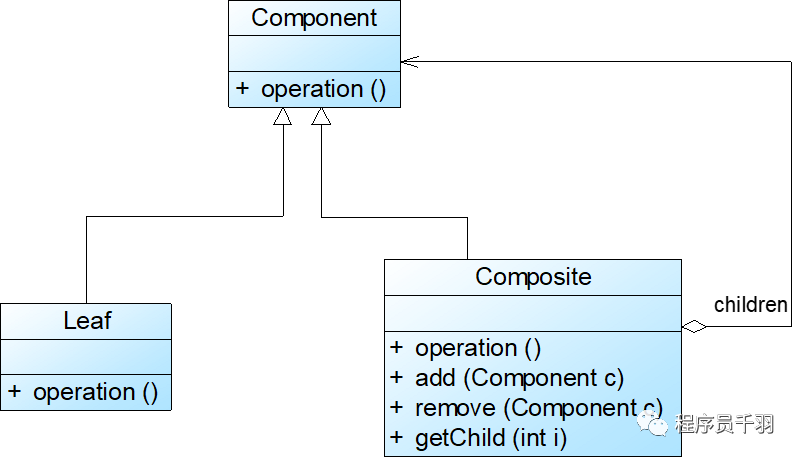
Component tree in Java AWT

5. Advantages, disadvantages and applicable environment of combination mode
Mode advantages
- Hierarchical complex objects can be clearly defined to represent all or part of the hierarchy of the object, so that the client can ignore the hierarchy differences and facilitate the control of the whole hierarchy
- The client can consistently use a composite structure or a single object in it, regardless of whether it is dealing with a single object or the whole composite structure, which simplifies the client code
- It is convenient to add new container components and leaf components, which conforms to the opening and closing principle
- It provides a flexible solution for the object-oriented implementation of tree structure
Mode disadvantages
When adding new components, it is difficult to limit the type of components in the container
Mode applicable environment
- In a hierarchy with whole and part, you want to ignore the differences between the whole and part in a way that clients can treat them consistently
- In a system developed with object-oriented language, we need to deal with a tree structure
- Leaf objects and container objects can be separated in a system, and their types are not fixed, so some new types need to be added