Reusable code means that the same code is used in similar business scenarios.
Reusable code can reduce duplication of effort. There are many similar business scenarios in a software. Abstract these scenarios into reusable code. When developing new functions, reusing code can reduce duplication of effort.
Reusable code can reduce the number of changes and omissions caused by changes in requirements. Imagine that if you want to modify the color of the submit button of the whole station, if the whole station has 100 pages containing the submit button, and the button style of each page is not reused, there is a great risk of change momentum and omission. If it is made reusable, only one change is needed.
How to write reusable code
The more responsibilities a code block has, the harder it is to reuse. Writing reusable code is to identify and separate reusable parts.
Consider this scenario: the function of code block a is to obtain interface data and render the UI. The UI of code block B is the same as that of a, but the interface data obtained is different. Code block C gets the same data as a, but the UI is different from a. A. The code between B and C cannot be reused.
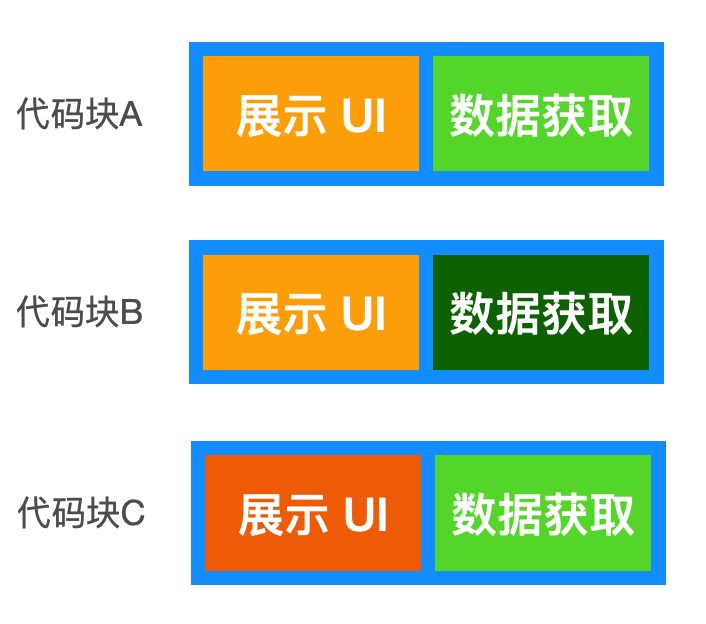
To change to reusable code is to separate the reusable UI and the code for obtaining interface data.
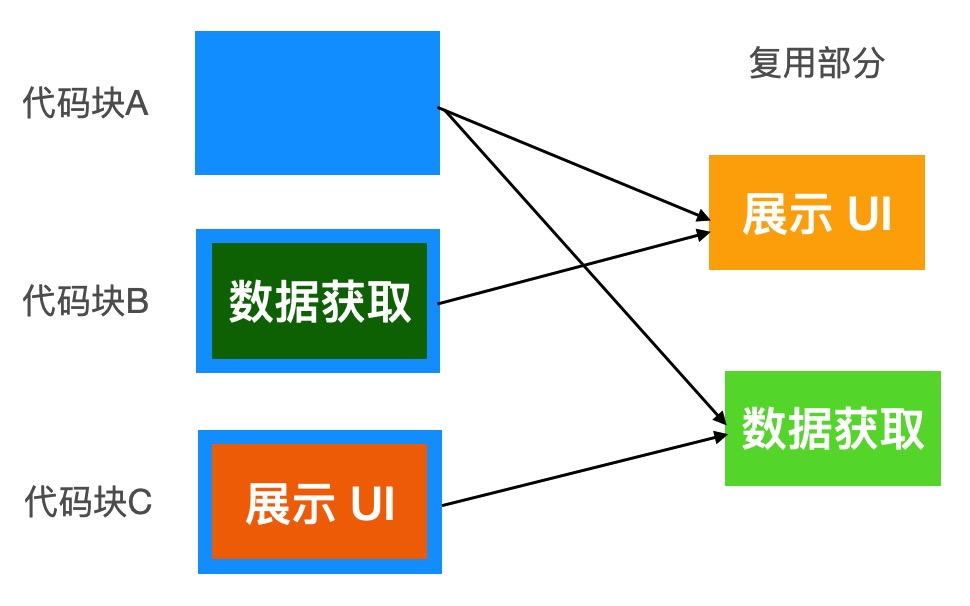
Next, let's look at some common reusable parts and reuse methods.
UI presentation
UI display is the display of appearance, including HTML and CSS. It does not include data acquisition and event processing.
The reuse of UI display code can be realized with components. Such components are called presentation components. Data and event processing are passed in through properties. Ant Design The components in the component library are display components. The following is the news list implemented by React:
import React from 'react' import s from './style.scss' import Item, {IItem} from './item' export interface INewsListProps { list: IItem[], onItemClick: (id: number) => void } const NewsList: FC<INewsListProps> = ({ list, onItemClick }) => { return ( <div className={s.list}> { list.map(item => ( <Item key={item.id} onClick={onItemClick} payload={item} /> )) } </div> ) } export default React.memo(NewsList)
Interface call
Interface calls can be reused in two parts:
- Common processing of interface requests and responses.
- Call of specific interface.
Common processing of interface requests and responses
When calling an interface, you often have to do some general processing. For example:
- For websites with separate front and back ends, a token shall be added to the request header of the interface to identify the user.
- When the interface reports an error, the error code shall be converted into user-friendly error information.
use axios Do this:
// request interceptor axios.interceptors.request.use(...) // Response interceptor axios.interceptors.response.use(...)
Call of specific interface
Interface calling code is usually placed in a file, such as service js:
export const fetchList = ... export const fetchDetail = ... export const createItem = ... export const updateItem = ... export const deleteItem = ...
Interface call, as well as Loading state management, anti shake, throttling, error retry, caching and other scenarios. React works useRequest , Vue has a similar wheel.
operation flow
Many business processes are similar and can be reused. For example, the business processes for managing background list pages are similar to this:
- When entering the page, get the list data.
- Click the search button to obtain the list data according to the current query criteria.
- Click paging to get the list of specified pages.
Custom hooks (called in vue3) Combined API )Support internal state management and lifecycle. Therefore, hooks can be used to encapsulate business processes. The following is the implementation of encapsulating the list page of the management background with Vue3's combined API:
import { onMounted, reactive, ref, Ref } from 'vue' export interface Params { url: string searchConditions: Record<string, any> } interface Return<T> { searchConditions: Record<string, any> resetConditions: () => void pagination: Record<string, any> fetchList: (isReset: boolean) => void list: Ref<T[]> isLoading: Ref<boolean> } function useList<T extends Record<string, any>> ({ url, searchConditions: initCondition }: Params): Return<T> { const searchConditions = reactive({...initCondition}) const pagination = reactive({ pageSize: 10 }) const list = ref<T[]>([]) as Ref<T[]> const isLoading = ref(false) as Ref<boolean> // isReset is true to search the first page. const fetchList = (isReset: boolean = false): void => ... // Enter page onMounted(() => { fetchList() }) return { searchConditions, pagination, fetchList, list, isLoading, } } export default useList
Recommended hooks tool libraries: ahooks . Vue version: ahooks-vue.
data
Some data points are used in multiple places. Such as login user information and permission data.
You can use the state management library to manage this data. React status management general Redux,Mobx or Context API . Vue general Vuex.
Tool function
Tool functions are business independent. For example, format the date, generate a unique id, etc. Lodash and moment.js Contains a lot of tools and methods.
summary
To write reusable code, the essence is to identify and separate the reusable parts. The front end can find reusable parts from UI display, interface call, business process, data, tools and functions.
The next level of code quality is reconfigurable code. I will introduce it in the next article.