0x00 overview
This paper deals with the Collection of Java knowledge points, including Collection collection, List Collection, array and queue structure, and the implementation class of List Collection
0x01 Collection
1.1 collection architecture
- Characteristics of set classes
A storage model with variable storage space is provided, and the stored data content can be changed at any time
- System diagram of collection class
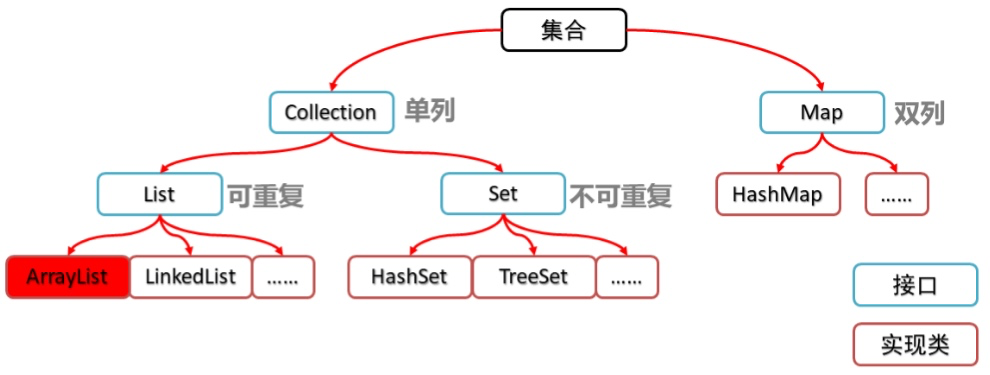
1.2 Collection collection overview and basic usage
- Collection collection overview
Is the top-level interface of a singleton Collection. It represents a set of objects, which are also called Collection elements
JDK does not provide any direct implementation of this interface. It provides more specific implementation of sub interfaces (such as Set and List)
- Collection basic usage
package com.CollectionTest1; import java.util.ArrayList; import java.util.Collection; public class CollectionDemo1 { public static void main(String[] args) { // Create an object for the Collection Collection<String> c = new ArrayList<String>(); // Add element: Boolean add (E) c.add("hello"); c.add("world"); c.add("java"); // Output collection object System.out.println(c); } }
1.3 common methods of collection

1.4 traversal of collection
- Introduction to iterators
Iterator, special traversal mode of collection
Iterator iterator(): returns the iterator of the elements in this collection, which is obtained through the iterator() method of the collection
The iterator is obtained through the iterator() method of the collection, so they say it depends on the collection
- Traversal of Collection
package com.CollectionTest1; import java.util.ArrayList; import java.util.Collection; import java.util.Iterator; public class IteratorDemo { public static void main(String[] args) { // Create collection object Collection<String> c = new ArrayList<>(); // Add element c.add("hello"); c.add("world"); c.add("java"); c.add("javaee"); // Iterator < E > Iterator(): returns the iterator of the elements in this collection, which is obtained through the Iterator() method of the collection Iterator<String> it = c.iterator(); // Using while loop to improve the judgment and acquisition of elements while (it.hasNext()) { String s = it.next(); System.out.println(s); } } }
1.5 illustration of collective use steps
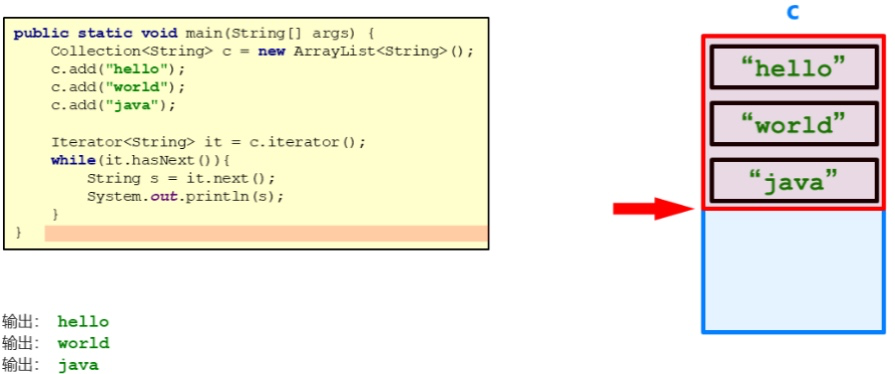
1.6 Collection case - Collection storage learning
Requirements: create a collection for storing student objects, store 3 student objects, and use the program to traverse the collection on the console
Example
package com.CollectionTest2; public class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
package com.CollectionTest2; import java.util.ArrayList; import java.util.Collection; import java.util.Iterator; public class CollectionDemo2 { public static void main(String[] args) { // Create Collection collection object Collection<Student> c = new ArrayList<Student>(); // Create student object Student s1 = new Student("Alice", 12); Student s2 = new Student("Bob", 13); Student s3 = new Student("Charlie", 14); // Add students to collection c.add(s1); c.add(s2); c.add(s3); // Traversal collection (iterator mode) Iterator<Student> it = c.iterator(); while (it.hasNext()) { Student s = it.next(); System.out.println(s.getName() + ", " + s.getAge()); } } }
0x02 List set
2.1 List set overview and features
- List collection overview
Ordered set (also known as sequence), users can accurately control the insertion position of each element in the list. Users can access elements through integer index and search for elements in the list
Unlike Set collections, lists usually allow duplicate elements
- List set features
Indexed
Duplicate elements can be stored
Element access order
2.2 unique methods of list set
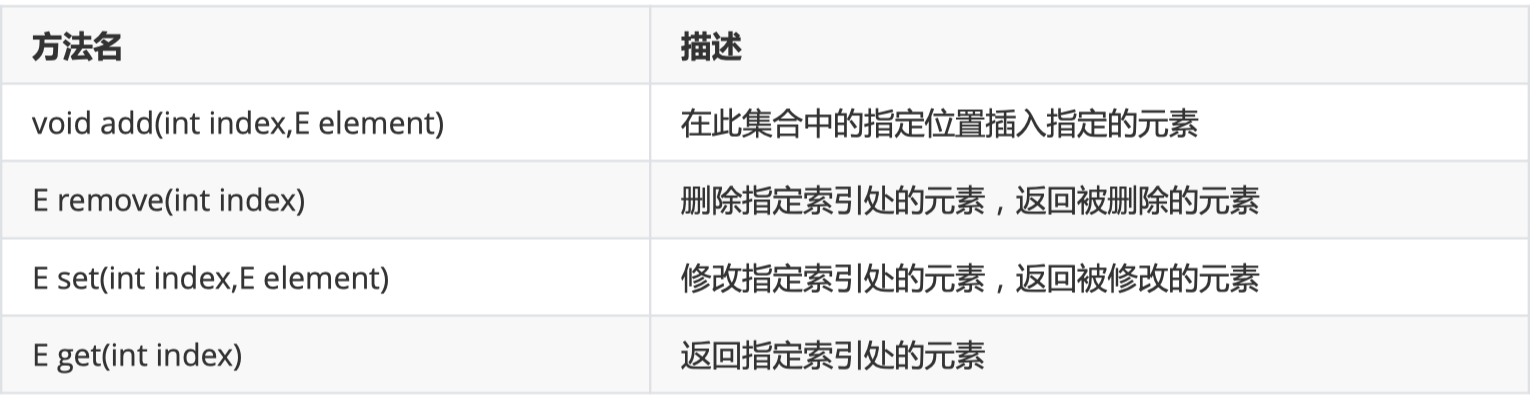
2.3 case of the set - the List set stores the student object and traverses it
Requirements: create a collection for storing student objects, store 3 student objects, and use the program to traverse the collection on the console
Example
package com.ListTest1; public class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
package com.ListTest1; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class ListDemo { public static void main(String[] args) { // Create a List collection object List<Student> list = new ArrayList<Student>(); // Create student object Student s1 = new Student("Alice", 13); Student s2 = new Student("Bob", 14); Student s3 = new Student("Charlie", 13); // Add students to collection list.add(s1); list.add(s2); list.add(s3); // Iterator pattern Iterator<Student> it = list.iterator(); while (it.hasNext()) { Student s = it.next(); System.out.println(s.getName() + ", " + s.getAge()); } System.out.println("------------"); for (int i = 0; i < list.size(); i++) { Student s = list.get(i); System.out.println(s.getName() + ", " + s.getAge()); } } }
2.4 concurrent modification exception
- Cause of occurrence
In the process of iterator traversal, the elements in the collection are modified by combining objects, resulting in the inconsistency between the expected modified value and the actual modified value judged by the iterator in the obtained elements, and a ConcurrentModificationException will appear
- Solution
Use for to traverse the loop, and then use the collection object to do the corresponding operation
Example
package com.ListDemo1; import java.util.ArrayList; import java.util.List; public class ListDemo { public static void main(String[] args) { // create object List<String> list = new ArrayList<String>(); // Add element list.add("hello"); list.add("world"); list.add("java"); // Traverse the collection to get each element. See if there is a "world" element. If so, add a Java EE. Please write code to implement it /* Iterator<String> it = list.iterator(); while(it.hasNext()) { String s = it.next(); if(s.equals("world")) { list.add("javaee"); } } */ for (int i = 0; i < list.size(); i++) { String s = list.get(i); if (s.equals("world")) { list.add("javaee"); } } } }
2.5 list iterator
- Introduction to ListIterator
It is obtained through the listIterator method of the List collection, so it is a unique iterator of the List collection
A list iterator that allows the programmer to traverse in either direction, modify the list during the iteration, and get the current position of the iterator in the list
Example
package com.ListDemo1; import java.util.ArrayList; import java.util.List; import java.util.ListIterator; public class ListIteratorDemo { public static void main(String[] args) { // create object List<String> list = new ArrayList<String>(); // Add element list.add("hello"); list.add("world"); list.add("java"); // Get list iterator ListIterator<String> lit = list.listIterator(); while(lit.hasNext()) { String s = lit.next(); if(s.equals("world")) { lit.add("javaee"); } } System.out.println(list); } }
2.6 enhanced for loop
Define format
for(Element data type variable name: array/Collection object name) { Circulatory body; }
Example
package com.ListDemo1; import java.util.ArrayList; import java.util.List; public class ForDemo { public static void main(String[] args) { int[] arr = {1, 2, 3, 4}; for (int i : arr) { System.out.print(i); } System.out.println("---------"); String[] strArray = {"hello", "world", "javaee"}; for (String s : strArray) { System.out.println(s); } System.out.println("---------"); List<String> list = new ArrayList<String>(); list.add("hellp"); list.add("world"); list.add("javaee"); for (String s : list) { System.out.println(s); } System.out.println("---------"); } }
2.7 set of cases - the List set stores three ways for students to traverse
Requirements: create a collection of student objects, store 3 student objects, and use the program to traverse the collection on the console
Example
package com.ListDemo2; public class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
package com.ListDemo2; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class ListDemo { public static void main(String[] args) { // Create a List collection object List<Student> list = new ArrayList<Student>(); // Create student object Student s1 = new Student("Alice", 13); Student s2 = new Student("Bob", 14); Student s3 = new Student("Charlie", 14); // Add students to collection list.add(s1); list.add(s2); list.add(s3); // Iterators: Collection specific traversal Iterator<Student> it = list.iterator(); while (it.hasNext()) { Student s = it.next(); System.out.println(s.getName() + ", " + s.getAge()); } System.out.println("---------"); // Normal for: traversal with index for (int i = 0; i < list.size(); i++) { Student s = list.get(i); System.out.println(s.getName() + ", " + s.getAge()); } // Enhanced for: the most convenient traversal method for (Student s : list) { System.out.println(s.getName() + ", " + s.getAge()); } } }
0x03 data structure
3.1 stack and queue of data structure
- Stack structure
In and out
- Queue structure
First in first out
3.2 array and linked list of data structure
- Array structure
Quick query, full additions and deletions
- Queue structure
Slow query, fast addition and deletion
0x04 implementation class of list collection
4.1 characteristics of list collection subclass
- ArrayList collection
The bottom layer is the implementation of data structure, with fast query and slow addition and deletion
- LinkedList collection
The bottom layer is the implementation of linked list structure, with slow query and fast addition and deletion
4.2 case of collection - the ArrayList collection stores student objects in three ways
package com.ArrayList01; public class Student { private String name; private int age; public Student() { } public Student(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
package com.ArrayList01; import java.util.ArrayList; import java.util.Iterator; public class ArrayListDemo { public static void main(String[] args) { // Create an ArrayList collection object ArrayList<Student> array = new ArrayList<Student>(); // Create student object Student s1 = new Student("Alice", 12); Student s2 = new Student("Bob", 13); Student s3 = new Student("Charlie", 14); // Add students to collection array.add(s1); array.add(s2); array.add(s3); // Iterators: Collection specific traversal Iterator<Student> it = array.iterator(); while (it.hasNext()) { Student s = it.next(); System.out.println(s.getName() + ", " + s.getAge()); } System.out.println("----------"); // Normal for: traversal with index for (int i = 0; i < array.size(); i++) { Student s = array.get(i); System.out.println(s.getName() + ", " + s.getAge()); } System.out.println("----------"); // Enhanced for: the most convenient traversal method for (Student s : array) { System.out.println(s.getName() + ", " + s.getAge()); } } }
4.3 unique functions of LinkedList set
Unique methods:
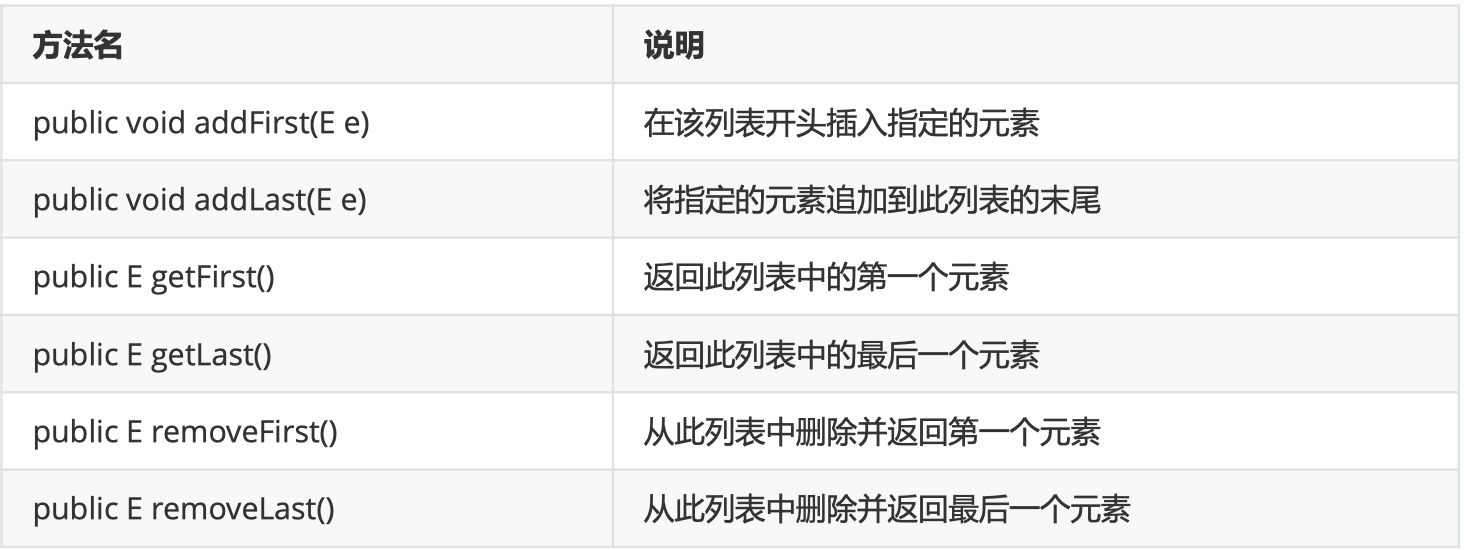