Previous Automation Framework Construction The article mentions object management (or element management), and Web Driver provides us with two ideas of object management: PageObject and PageFactory. Today I will compare the similarities and differences between the two methods.~
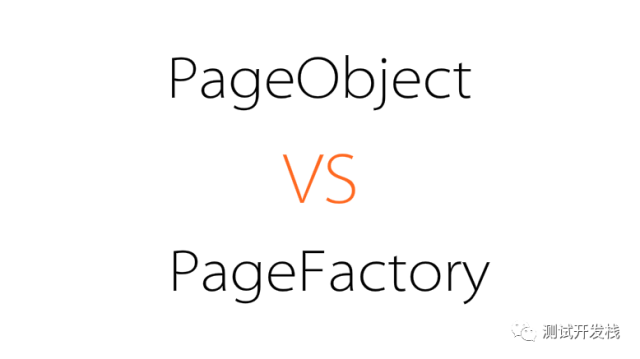
On PageObject
Considering a page or a class of elements as a page object, a page object corresponds to a class, and the acquisition of element objects is defined in this class. (Explanations always seem so pale. Look at the code.)
Mode 1:
public class HomePage { protected WebDriver driver public HomePage(WebDriver driver) { this.driver= driver; } public WebElement getUserName() { By by = By.xpath("//span[@class='name']"); return driver.findElement(by); } public List<WebElement> getLinks() { By by = By.xpath("//a"); return driver.findElements(by); } public WebElement getHomeLink() { By by = By.xpath("//a[contains(text(),'home page')] "; return driver.findElement(by); } }
Mode 2:
public class HomePage { protected WebDriver driver public HomePage(WebDriver driver) { this.driver= driver; } public WebElement getUserName() { By by = By.xpath("//span[@class='name']"); return isElementExist(by) ? driver.findElement(by) : null; } public List<WebElement> getLinks() { By by = By.xpath("//a"); return isElementsExist(by) ? driver.findElement(by) : null; } public WebElement getHomeLink() { By by = By.xpath("//a[contains(text(),'home page')] "; return isElementExist(by) ? driver.findElement(by) : null; } }
The method returns findElement() method all the time, and throws NoSuchElementException exception when the element is not located, thus interrupting the test. So I give mode 2 (recommendation mode 2). Here I give isElementExist() method:
public static boolean isElementExist(By selector){ try { driver.findElement(selector); return true; } catch (NoSuchElementException e) { return false; } }
About PageFactory
The whole idea, like PageObject, is to define element objects in the form of annotations (@FindBy) and see the code: **
Mode 1:
public class HomePage { @FindBy(xpath = "//span[@class='name']") @CacheLookup public static WebElement userName; @FindBy(xpath = "//a") @CacheLookup public static List<WebElement> links; @FindBy(xpath = "//a[contains(text(),'home page')]" @CacheLookup public static WebElement homeLink; }
Classes need to be initialized when using objects:
PageFactory.initElements(driver ,HomePage.class); HomePage.homeLink.click();
Mode 2:
public class HomePage extends BasePage{ public HomePage(WebDriver driver) { super(driver); } public HomePage(WebDriver driver, String titile) { super(driver, titile); } @FindBy(xpath = "//span[@class='name']") @CacheLookup public WebElement userName; @FindBy(xpath = "//a") @CacheLookup public List<WebElement> links; @FindBy(xpath = "//a[contains(text(),'home page')]" @CacheLookup public WebElement homeLink; } public class BasePage { public static final String MAIN_TITLE = "XXX platform"; public WebDriver driver; public String title; private final int TIMEOUT = 10; public BasePage() {} public BasePage(WebDriver driver) { this.driver = driver; PageFactory.initElements(new AjaxElementLocatorFactory(driver, TIMEOUT) , this); } public BasePage(WebDriver driver, final String title) { this.driver = driver; this.title = title; try{ new WebDriverWait(driver,TIMEOUT).until(new ExpectedCondition<Boolean>(){ @Override public Boolean apply(WebDriver arg0) { // TODO Auto-generated method stub String acttitle = arg0.getTitle(); return acttitle.equals(title); }}); }catch(TimeoutException te) { throw new IllegalStateException("Current page title(" + driver.getTitle() + ")With expectations("+ title +")Atypism"); } PageFactory.initElements(new AjaxElementLocatorFactory(driver, TIMEOUT) , this); } }
Mode 2 (recommended) increases the waiting time of pages and elements, which is more robust than mode 1 and clearer when using objects:
HomePage home = new HomePage(driver,"XXX"); home.homeLink.click();
summary
The difference between PageObject and PageFactory is given in the form of code above, and two recommended ways of using object management ideas are given in the way of code optimization. I usually use both ways and sometimes mix them together. Generally speaking, it can't say who is good or bad, each has its own merits and demerits. I think PageObject is more convenient in element judgment. The idea that null is the absence of elements is straightforward; besides using annotations to make the code cleaner, PageFactory can also set the waiting time for page and element loading. Overall, PageFactory is recommended.
The original text is from the public number below. Please contact the author for reprinting, and be sure to keep the source.
If you want to see more original technology articles and materials for the first time, please pay attention to the public number: test development stack
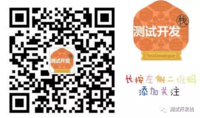