Links to the original text: http://blog.csdn.net/kakashi8841/article/details/45478433
1. Demand for ashing
Often, when we play games, we encounter a situation. For example, a skill icon is normal color when it can be clicked, and grey when it cannot be clicked. Another example is a function that is normally colored when it is open and grey when it is not. More often, your QQ friends, for example, will turn gray if they are not online.
Then there is a need to turn a picture grey.
2. Talking about Ashing and Gray Level
First of all, you can't say let art produce two sets of pictures, one set of colors and one set of gray ones. This will increase resource usage.
Then we can only find a way to deal with it through procedures.
So let's first figure out what a grey picture looks like.
(colour)
(after ashing)
It's gray to see the words "Team Upgrade" and their background.
How to make a picture grey? First, the color is composed of RGB (you want to say CMYK, HSB, even index color, I won't argue with you about this, after all, I'm a accomplished person). What we see is so-called grey. That is, R, G and B all have the same values. That is to say, we need to calculate the original RGB value of the picture into a new RGB, the new color of his R=G=B.
Well, how can RGB be calculated as a gray value?
This involves an ashing formula, which is an empirical formula, that is to say, the formula is not fixed. As long as you figure it out, the effect is grey, that's OK.
For example, in the simplest case, you can use formulas:
k = (r + g + b) / 3
This is to average RGB.
There is also a psychological formula:
k = r*.222 + g*.707 + b*.071;
What does this formula mean? As you can see, 0.222 + 0.707 + 0.071 = 1
In fact, this formula means that the proportion of RGB is different, for example, R accounts for 0.222. Then he multiplied the RGB values by the corresponding proportions and got a new value. Formula 1, in fact, holds that RGB accounts for the same proportion.
These formulas end up with gray pictures (because you R=G=B=k), but look different. You can also try other weights yourself.
3. Practice of ashing NGUI
So much, let's start with a few lines of code.
The idea is to modify the Transparent Colored shader of NGUI. We deal with it by losing a color. Take (0, 0, 0) as the switch to enable gray level.
The Transparent Colored code is as follows:
- Shader "Unlit/Transparent Colored"
- {
- Properties
- {
- _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {}
- }
- SubShader
- {
- LOD 100
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Cull Off
- Lighting Off
- ZWrite Off
- Fog { Mode Off }
- Offset -1, -1
- Blend SrcAlpha OneMinusSrcAlpha
- Pass
- {
- CGPROGRAM
- #pragma vertex vert
- #pragma fragment frag
- #include "UnityCG.cginc"
- struct appdata_t
- {
- float4 vertex : POSITION;
- float2 texcoord : TEXCOORD0;
- fixed4 color : COLOR;
- };
- struct v2f
- {
- float4 vertex : SV_POSITION;
- half2 texcoord : TEXCOORD0;
- fixed4 color : COLOR;
- fixed gray : TEXCOORD1;
- };
- sampler2D _MainTex;
- float4 _MainTex_ST;
- v2f vert (appdata_t v)
- {
- v2f o;
- o.vertex = mul(UNITY_MATRIX_MVP, v.vertex);
- o.texcoord = v.texcoord;
- o.color = v.color;
- o.gray = dot(v.color, fixed4(1,1,1,0));
- return o;
- }
- fixed4 frag (v2f i) : COLOR
- {
- fixed4 col;
- col = tex2D(_MainTex, i.texcoord);
- if(i.gray == 0)
- {
- float grey = dot(col.rgb, float3(0.299, 0.587, 0.114));
- col.rgb = float3(grey, grey, grey);
- }
- else
- {
- col = col * i.color;
- }
- return col;
- }
- ENDCG
- }
- }
- SubShader
- {
- LOD 100
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Fog { Mode Off }
- Offset -1, -1
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- ColorMaterial AmbientAndDiffuse
- SetTexture [_MainTex]
- {
- Combine Texture * Primary
- }
- }
- }
- }
Shader "Unlit/Transparent Colored" { Properties { _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {} } SubShader { LOD 100 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Cull Off Lighting Off ZWrite Off Fog { Mode Off } Offset -1, -1 Blend SrcAlpha OneMinusSrcAlpha Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" struct appdata_t { float4 vertex : POSITION; float2 texcoord : TEXCOORD0; fixed4 color : COLOR; }; struct v2f { float4 vertex : SV_POSITION; half2 texcoord : TEXCOORD0; fixed4 color : COLOR; fixed gray : TEXCOORD1; }; sampler2D _MainTex; float4 _MainTex_ST; v2f vert (appdata_t v) { v2f o; o.vertex = mul(UNITY_MATRIX_MVP, v.vertex); o.texcoord = v.texcoord; o.color = v.color; o.gray = dot(v.color, fixed4(1,1,1,0)); return o; } fixed4 frag (v2f i) : COLOR { fixed4 col; col = tex2D(_MainTex, i.texcoord); if(i.gray == 0) { float grey = dot(col.rgb, float3(0.299, 0.587, 0.114)); col.rgb = float3(grey, grey, grey); } else { col = col * i.color; } return col; } ENDCG } } SubShader { LOD 100 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Fog { Mode Off } Offset -1, -1 ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha ColorMaterial AmbientAndDiffuse SetTexture [_MainTex] { Combine Texture * Primary } } } }We calculate in vert whether the set color (the place shown below) is all zero.
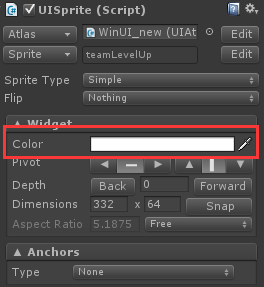
If all is 0, the ashing formula is applied to frag.
So the modification was completed.
4. Supporting softclip
To work with softclip, please modify it together
Transparent Colored 1.shader, Transparent Colored 2.shader, Transparent Colored 3.shader are the following codes:
Many articles on the Internet may not mention the above three shader modifications, resulting in many developers using ashing in softclip ineffective.
- Shader "HIDDEN/Unlit/Transparent Colored 1"
- {
- Properties
- {
- _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {}
- }
- SubShader
- {
- LOD 200
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Offset -1, -1
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- CGPROGRAM
- #pragma vertex vert
- #pragma fragment frag
- #include "UnityCG.cginc"
- sampler2D _MainTex;
- float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0);
- float2 _ClipArgs0 = float2(1000.0, 1000.0);
- struct appdata_t
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- };
- struct v2f
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- float2 worldPos : TEXCOORD1;
- };
- v2f vert (appdata_t v)
- {
- v2f o;
- o.vertex = mul(UNITY_MATRIX_MVP, v.vertex);
- o.color = v.color;
- o.texcoord = v.texcoord;
- o.worldPos = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy;
- return o;
- }
- half4 frag (v2f IN) : COLOR
- {
- // Softness factor
- float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos)) * _ClipArgs0;
- // Sample the texture
- half4 col = tex2D(_MainTex, IN.texcoord);
- if (dot(IN.color, fixed4(1,1,1,0)) == 0)
- {
- col = tex2D(_MainTex, IN.texcoord);
- col.rgb = dot(col.rgb, fixed3(.222,.707,.071));
- }else{
- col = col * IN.color;
- }
- col.a *= clamp( min(factor.x, factor.y), 0.0, 1.0);
- return col;
- }
- ENDCG
- }
- }
- SubShader
- {
- LOD 100
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- ColorMaterial AmbientAndDiffuse
- SetTexture [_MainTex]
- {
- Combine Texture * Primary
- }
- }
- }
- }
Shader "HIDDEN/Unlit/Transparent Colored 1" { Properties { _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {} } SubShader { LOD 200 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Offset -1, -1 Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" sampler2D _MainTex; float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0); float2 _ClipArgs0 = float2(1000.0, 1000.0); struct appdata_t { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; }; struct v2f { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; float2 worldPos : TEXCOORD1; }; v2f vert (appdata_t v) { v2f o; o.vertex = mul(UNITY_MATRIX_MVP, v.vertex); o.color = v.color; o.texcoord = v.texcoord; o.worldPos = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy; return o; } half4 frag (v2f IN) : COLOR { // Softness factor float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos)) * _ClipArgs0; // Sample the texture half4 col = tex2D(_MainTex, IN.texcoord); if (dot(IN.color, fixed4(1,1,1,0)) == 0) { col = tex2D(_MainTex, IN.texcoord); col.rgb = dot(col.rgb, fixed3(.222,.707,.071)); }else{ col = col * IN.color; } col.a *= clamp( min(factor.x, factor.y), 0.0, 1.0); return col; } ENDCG } } SubShader { LOD 100 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha ColorMaterial AmbientAndDiffuse SetTexture [_MainTex] { Combine Texture * Primary } } } }
- Shader "HIDDEN/Unlit/Transparent Colored 2"
- {
- Properties
- {
- _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {}
- }
- SubShader
- {
- LOD 200
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Offset -1, -1
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- CGPROGRAM
- #pragma vertex vert
- #pragma fragment frag
- #include "UnityCG.cginc"
- sampler2D _MainTex;
- float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0);
- float4 _ClipArgs0 = float4(1000.0, 1000.0, 0.0, 1.0);
- float4 _ClipRange1 = float4(0.0, 0.0, 1.0, 1.0);
- float4 _ClipArgs1 = float4(1000.0, 1000.0, 0.0, 1.0);
- struct appdata_t
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- };
- struct v2f
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- float4 worldPos : TEXCOORD1;
- };
- float2 Rotate (float2 v, float2 rot)
- {
- float2 ret;
- ret.x = v.x * rot.y - v.y * rot.x;
- ret.y = v.x * rot.x + v.y * rot.y;
- return ret;
- }
- v2f vert (appdata_t v)
- {
- v2f o;
- o.vertex = mul(UNITY_MATRIX_MVP, v.vertex);
- o.color = v.color;
- o.texcoord = v.texcoord;
- o.worldPos.xy = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy;
- o.worldPos.zw = Rotate(v.vertex.xy, _ClipArgs1.zw) * _ClipRange1.zw + _ClipRange1.xy;
- return o;
- }
- half4 frag (v2f IN) : COLOR
- {
- // First clip region
- float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos.xy)) * _ClipArgs0.xy;
- float f = min(factor.x, factor.y);
- // Second clip region
- factor = (float2(1.0, 1.0) - abs(IN.worldPos.zw)) * _ClipArgs1.xy;
- f = min(f, min(factor.x, factor.y));
- half4 col;
- col = tex2D(_MainTex, IN.texcoord);
- if (dot(IN.color, fixed4(1,1,1,0)) == 0)
- {
- col.rgb = dot(col.rgb, fixed3(.222,.707,.071));
- }else{
- col = col * IN.color;
- }
- col.a *= clamp(f, 0.0, 1.0);
- return col;
- }
- ENDCG
- }
- }
- SubShader
- {
- LOD 100
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- ColorMaterial AmbientAndDiffuse
- SetTexture [_MainTex]
- {
- Combine Texture * Primary
- }
- }
- }
- }
Shader "HIDDEN/Unlit/Transparent Colored 2" { Properties { _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {} } SubShader { LOD 200 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Offset -1, -1 Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" sampler2D _MainTex; float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0); float4 _ClipArgs0 = float4(1000.0, 1000.0, 0.0, 1.0); float4 _ClipRange1 = float4(0.0, 0.0, 1.0, 1.0); float4 _ClipArgs1 = float4(1000.0, 1000.0, 0.0, 1.0); struct appdata_t { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; }; struct v2f { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; float4 worldPos : TEXCOORD1; }; float2 Rotate (float2 v, float2 rot) { float2 ret; ret.x = v.x * rot.y - v.y * rot.x; ret.y = v.x * rot.x + v.y * rot.y; return ret; } v2f vert (appdata_t v) { v2f o; o.vertex = mul(UNITY_MATRIX_MVP, v.vertex); o.color = v.color; o.texcoord = v.texcoord; o.worldPos.xy = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy; o.worldPos.zw = Rotate(v.vertex.xy, _ClipArgs1.zw) * _ClipRange1.zw + _ClipRange1.xy; return o; } half4 frag (v2f IN) : COLOR { // First clip region float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos.xy)) * _ClipArgs0.xy; float f = min(factor.x, factor.y); // Second clip region factor = (float2(1.0, 1.0) - abs(IN.worldPos.zw)) * _ClipArgs1.xy; f = min(f, min(factor.x, factor.y)); half4 col; col = tex2D(_MainTex, IN.texcoord); if (dot(IN.color, fixed4(1,1,1,0)) == 0) { col.rgb = dot(col.rgb, fixed3(.222,.707,.071)); }else{ col = col * IN.color; } col.a *= clamp(f, 0.0, 1.0); return col; } ENDCG } } SubShader { LOD 100 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha ColorMaterial AmbientAndDiffuse SetTexture [_MainTex] { Combine Texture * Primary } } } }
- Shader "HIDDEN/Unlit/Transparent Colored 3"
- {
- Properties
- {
- _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {}
- }
- SubShader
- {
- LOD 200
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Offset -1, -1
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- CGPROGRAM
- #pragma vertex vert
- #pragma fragment frag
- #include "UnityCG.cginc"
- sampler2D _MainTex;
- float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0);
- float4 _ClipArgs0 = float4(1000.0, 1000.0, 0.0, 1.0);
- float4 _ClipRange1 = float4(0.0, 0.0, 1.0, 1.0);
- float4 _ClipArgs1 = float4(1000.0, 1000.0, 0.0, 1.0);
- float4 _ClipRange2 = float4(0.0, 0.0, 1.0, 1.0);
- float4 _ClipArgs2 = float4(1000.0, 1000.0, 0.0, 1.0);
- struct appdata_t
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- };
- struct v2f
- {
- float4 vertex : POSITION;
- half4 color : COLOR;
- float2 texcoord : TEXCOORD0;
- float4 worldPos : TEXCOORD1;
- float2 worldPos2 : TEXCOORD2;
- };
- float2 Rotate (float2 v, float2 rot)
- {
- float2 ret;
- ret.x = v.x * rot.y - v.y * rot.x;
- ret.y = v.x * rot.x + v.y * rot.y;
- return ret;
- }
- v2f vert (appdata_t v)
- {
- v2f o;
- o.vertex = mul(UNITY_MATRIX_MVP, v.vertex);
- o.color = v.color;
- o.texcoord = v.texcoord;
- o.worldPos.xy = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy;
- o.worldPos.zw = Rotate(v.vertex.xy, _ClipArgs1.zw) * _ClipRange1.zw + _ClipRange1.xy;
- o.worldPos2 = Rotate(v.vertex.xy, _ClipArgs2.zw) * _ClipRange2.zw + _ClipRange2.xy;
- return o;
- }
- half4 frag (v2f IN) : COLOR
- {
- // First clip region
- float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos.xy)) * _ClipArgs0.xy;
- float f = min(factor.x, factor.y);
- // Second clip region
- factor = (float2(1.0, 1.0) - abs(IN.worldPos.zw)) * _ClipArgs1.xy;
- f = min(f, min(factor.x, factor.y));
- // Third clip region
- factor = (float2(1.0, 1.0) - abs(IN.worldPos2)) * _ClipArgs2.xy;
- f = min(f, min(factor.x, factor.y));
- // Sample the texture
- half4 col = tex2D(_MainTex, IN.texcoord);
- if (dot(IN.color, fixed4(1,1,1,0)) == 0)
- {
- col.rgb = dot(col.rgb, fixed3(.222,.707,.071));
- }else{
- col = col * IN.color;
- }
- col.a *= clamp(f, 0.0, 1.0);
- return col;
- }
- ENDCG
- }
- }
- SubShader
- {
- LOD 100
- Tags
- {
- "Queue" = "Transparent"
- "IgnoreProjector" = "True"
- "RenderType" = "Transparent"
- }
- Pass
- {
- Cull Off
- Lighting Off
- ZWrite Off
- Fog { Mode Off }
- ColorMask RGB
- AlphaTest Greater .01
- Blend SrcAlpha OneMinusSrcAlpha
- ColorMaterial AmbientAndDiffuse
- SetTexture [_MainTex]
- {
- Combine Texture * Primary
- }
- }
- }
- }
Shader "HIDDEN/Unlit/Transparent Colored 3" { Properties { _MainTex ("Base (RGB), Alpha (A)", 2D) = "black" {} } SubShader { LOD 200 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Offset -1, -1 Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" sampler2D _MainTex; float4 _ClipRange0 = float4(0.0, 0.0, 1.0, 1.0); float4 _ClipArgs0 = float4(1000.0, 1000.0, 0.0, 1.0); float4 _ClipRange1 = float4(0.0, 0.0, 1.0, 1.0); float4 _ClipArgs1 = float4(1000.0, 1000.0, 0.0, 1.0); float4 _ClipRange2 = float4(0.0, 0.0, 1.0, 1.0); float4 _ClipArgs2 = float4(1000.0, 1000.0, 0.0, 1.0); struct appdata_t { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; }; struct v2f { float4 vertex : POSITION; half4 color : COLOR; float2 texcoord : TEXCOORD0; float4 worldPos : TEXCOORD1; float2 worldPos2 : TEXCOORD2; }; float2 Rotate (float2 v, float2 rot) { float2 ret; ret.x = v.x * rot.y - v.y * rot.x; ret.y = v.x * rot.x + v.y * rot.y; return ret; } v2f vert (appdata_t v) { v2f o; o.vertex = mul(UNITY_MATRIX_MVP, v.vertex); o.color = v.color; o.texcoord = v.texcoord; o.worldPos.xy = v.vertex.xy * _ClipRange0.zw + _ClipRange0.xy; o.worldPos.zw = Rotate(v.vertex.xy, _ClipArgs1.zw) * _ClipRange1.zw + _ClipRange1.xy; o.worldPos2 = Rotate(v.vertex.xy, _ClipArgs2.zw) * _ClipRange2.zw + _ClipRange2.xy; return o; } half4 frag (v2f IN) : COLOR { // First clip region float2 factor = (float2(1.0, 1.0) - abs(IN.worldPos.xy)) * _ClipArgs0.xy; float f = min(factor.x, factor.y); // Second clip region factor = (float2(1.0, 1.0) - abs(IN.worldPos.zw)) * _ClipArgs1.xy; f = min(f, min(factor.x, factor.y)); // Third clip region factor = (float2(1.0, 1.0) - abs(IN.worldPos2)) * _ClipArgs2.xy; f = min(f, min(factor.x, factor.y)); // Sample the texture half4 col = tex2D(_MainTex, IN.texcoord); if (dot(IN.color, fixed4(1,1,1,0)) == 0) { col.rgb = dot(col.rgb, fixed3(.222,.707,.071)); }else{ col = col * IN.color; } col.a *= clamp(f, 0.0, 1.0); return col; } ENDCG } } SubShader { LOD 100 Tags { "Queue" = "Transparent" "IgnoreProjector" = "True" "RenderType" = "Transparent" } Pass { Cull Off Lighting Off ZWrite Off Fog { Mode Off } ColorMask RGB AlphaTest Greater .01 Blend SrcAlpha OneMinusSrcAlpha ColorMaterial AmbientAndDiffuse SetTexture [_MainTex] { Combine Texture * Primary } } } }
OK, that's all. The test project will be uploaded later.