catalogue
Sequential reading and writing of files
Random reading and writing of files
- Program file
- data file
1, Document classification
Program file
- Source program file (. c);
- Object file (windows environment. obj), temporary file during compilation;
- Executable files (windows environment. exe), etc;
data file
- The content of the file is not necessarily the program, but the data read and written when the program runs;
- If the program runs, it needs to read the data from the file, or output the content of the file;
II. Documents
- A document shall have a unique document identification for user identification and reference;
file name
- File path
- File name backbone
- file extension
For example, C: \ code \ test txt
field name pointer
- In the buffered file system, the key concept is "file type pointer", which is called file pointer for short;
- Each used file opens up a corresponding file information area in the memory to store the relevant information of the file (such as file name, file status and current location of the file, etc.);
- This information is stored in a structure variable, which is declared by the structure type system and named FILE;
- In different C compilers, the contents of FILE type are not exactly the same, but they are similar;
- Whenever a FILE is opened, the system will automatically create a variable of FILE structure and fill in the information according to the FILE situation;
- Generally, the variables of the FILE structure are maintained through a FILE pointer, which is more convenient to use;
- Such as FILE* pf;
Opening and closing of files
- The file should be opened before reading and writing, and closed after use;
- When opening a FILE, a pointer variable of FILE * will be returned to point to the FILE;
- Open the file fopen and close the file fclose;
int main() { FILE* pf = fopen("F:\\VS\\Project1\\test.txt", "w"); if (pf == NULL) { perror("fopen"); return 0; } fclose(pf); pf = NULL; return 1; }
Note: document usage
Sequential reading and writing of files
//Input and output the current character int main() { FILE* pf = fopen("test.txt", "w+"); if (pf == NULL) { perror("fopen"); return 0; } //Output / write character fputc('b', pf); fputc('i', pf); fputc('t', pf); //Move the file pointer to the start position fseek(pf, 0L, SEEK_SET); //Enter / read characters printf("%c", fgetc(pf)); printf("%c", fgetc(pf)); printf("%c", fgetc(pf)); fclose(pf); pf = NULL; return 1; }
//Input and output a line of characters int main() { char str[] = "abcde"; FILE* pf = fopen("test.txt", "w+"); if (pf == NULL) { perror("fopen"); return 0; } //Output / write string fputs("bit", pf); //Move the file pointer to the start position fseek(pf, 0L, SEEK_SET); //Input / read string fgets(str, 2, pf); //2 characters contain '\ 0' printf("%s", str); fgets(str, 2, pf); //2 characters contain '\ 0' printf("%s", str); fclose(pf); pf = NULL; return 1; }
//Format data input and output struct S { char str[100]; int num; }; int main() { struct S s1 = { "bit",10 }; struct S s2 = { {0},0 }; FILE* pf = fopen("test.txt", "w+"); if (pf == NULL) { perror("fopen"); return 0; } //Output / write formatted data fprintf(pf, "%s ", s1.str); fprintf(pf, "%d ", s1.num); //Move the file pointer to the start position fseek(pf, 0L, SEEK_SET); //Enter / read formatted data up to spaces fscanf(pf, "%s", s2.str); fscanf(pf, "%d", &(s2.num)); printf("%s %d", s2.str, s2.num); fclose(pf); pf = NULL; return 1; }
//Binary input and output struct S { char str[100]; int num; }; int main() { struct S s1 = { "bit",10 }; struct S s2 = { {0},0 }; FILE* pf = fopen("test.txt", "w+"); if (pf == NULL) { perror("fopen"); return 0; } //Binary output / write fwrite(&s1,sizeof(struct S),1,pf); //Move the file pointer to the start position fseek(pf, 0L, SEEK_SET); //Binary input / read fread(&s2, sizeof(struct S), 1, pf); printf("%s %d", s2.str, s2.num); fclose(pf); pf = NULL; return 1; }
Memory and hard disk read / write:
Note: file read / write function
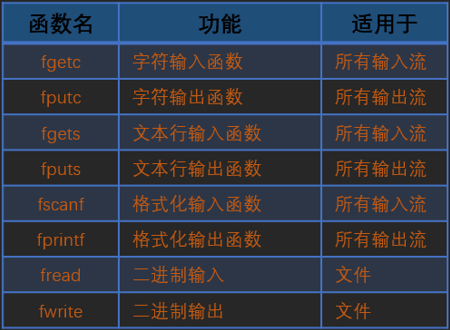
Flow probability:
- File stream;
- Standard input / output stream stdin/stdout;
Standard I / O stream read / write
- gets, get a line of string from stdio;
- Put, write a line of string to stdout;
remarks:
- scanf/printf, format input / output statements for standard input / output streams;
- fscanf/fprintf, format input / output statements for all input / output streams;
- sscanf/sprintf, sscanf reads the formatted data from the string, and sprintf outputs the formatted data to the string;
struct S { char name[10]; int num; float score; }; int main() { struct S s1 = { "lisi",20,99.5f }; char str[100] = { 0 }; //Format the data from s1 and output it to str sprintf(str, "%s %d %f", s1.name, s1.num, s1.score); printf("%s\n", str); struct S s2 = { 0 }; //Read the formatted data from str to s2 sscanf(str, "%s %d %f", s2.name, &(s2.num), &(s2.score)); printf("%s %d %f", s2.name, s2.num, s2.score); return 0; }
Random reading and writing of files
- fseek, locate the file pointer according to the position and offset of the file pointer;
- origin, need to be seek_ Cur (current position), seek_ End, seek_ Set (file start location);
- ftell, returns the offset of the file pointer relative to the starting position;
- rewind to return the position of the file pointer to the starting position of the file;
int main() { FILE* pf = fopen("test.txt", "w+"); if (pf == NULL) { perror("fopen"); return 0; } //Output / write character fputc('b', pf); fputc('i', pf); fputc('t', pf); //Move the file pointer to the start position fseek(pf, 0L, SEEK_SET); printf("%c\n", fgetc(pf)); //The file pointer moves to the end position fseek(pf, -2L, SEEK_END); printf("%c\n", fgetc(pf)); //The file pointer moves to the current location fseek(pf, 0L, SEEK_CUR); printf("%c\n", fgetc(pf)); //Returns the current pointer offset printf("%d\n", ftell(pf)); //Move the file pointer to the start position rewind(pf); printf("%c\n", fgetc(pf)); fclose(pf); pf = NULL; return 1; }
Text and binary files
- The organization form of more data is classified into text file and binary file;
- Data stored in binary form in memory and output to external memory without conversion is binary file;
- Data is stored in binary form in memory, converted into ASCII code and output to external memory, that is, text file;
How data is stored in a file
- All characters are stored in ASCII code;
- Numerical data can be stored in ASCII code or binary form;
End judgment of documents
feof
- It is often used incorrectly to determine the end of the file;
- In the process of file reading, the return value of feof function can not be used to directly judge whether the file ends;
- It should be used to judge whether the reading fails or the end of the file is encountered when the file reading ends;
End of text file reading
- fgetc, EOF is returned at the end of reading, which is normally read as character ASCII code value;
- fgets, NULL is returned at the end of reading, and the normal reading is the starting position of the string;
Whether binary file reading is over
- fread, normally returns the actual number of elements. If it is less than the actual number to be read, the file reading is completed;
//The file test1 Txt, copy to test2 txt int main() { FILE* pfr = fopen("test1.txt", "r"); if (pfr == NULL) { perror("pfr"); return 0; } FILE* pfw = fopen("test2.txt", "w"); if (pfw == NULL) { perror("pfw"); return 0; } char c = 0; //EOF is returned when reading fails or the file ends while ((c = fgetc(pfr)) != EOF) { fputc(c, pfw); } if (ferror(pfr)) puts("I/0,error when reading!"); else if (feof(pfr)) puts("End of File!"); fclose(pfr); pfr = NULL; fclose(pfw); pfw = NULL; return 1; }
file buffer
- ANSIC standard adopts "buffer file system" to process data files;
- Buffer file system means that the system automatically opens up a "file buffer" for each file being used in the program in memory;
- The data output from the memory to the disk will be sent to the buffer in the memory first, and then sent to the disk together after the buffer is filled;
- When reading data from the disk to the computer, read the data from the disk file and input it into the memory buffer. After filling the buffer, send the data to the program data area one by one;
- The size of the buffer is determined by the C compiler system;
#include <stdio.h> #include <Windows.h> int main() { FILE* pf = fopen("test.txt", "w"); if (pf == NULL) { perror("pf"); return 0; } //Write code to output buffer fputc('a', pf); //Sleep for 10s, no content is written in the file Sleep(10000); //Refresh cache fflush(pf); //Sleep for 10s, contents written in the file Sleep(10000); //Closing the file also flushes the cache fclose(pf); pf = NULL; return 1; }
case
- Address book (use file to store the entered information)