The Q & a module of csdn answers some questions, some of which are quite interesting. It jumps out for your reading and reference Leave a mark - answer before December 7, 2021
Article catalog
- 1. Accountants use Python to write codes for month end weighted average method, first in first out method and moving weighted average method
- 2. Score statistics and triangle printing
- 3. Interface request, parameter extraction from text, how to operate, and complete code
- 4. How to solve the problem that pycharm crawls data and stores it in mysql repeatedly?
- 5. Enter an integer and find the product of each digit
1. Accountants use Python to write codes for month end weighted average method, first in first out method and moving weighted average method
Title Description: Python writes three methods of cost calculation Please, guys! Only write the weighted average method once at the end of the month!
import numpy as np import pandas as pd # Generate test data df = pd.DataFrame([pd.date_range("2021-11-01","2021-11-30"),np.random.randint(30,50,size=30),np.random.randint(20,40,size=30),np.random.randint(10,20,size=30)]).T df.columns = ["Warehousing date","Purchase quantity","sales volumes","Purchase price"] df.head() # weighted average method # Assume inventory cost and quantity at the beginning of the month cost0 = 450 num0 = 50 # Inventory unit cost = [actual cost of inventory at the beginning of the month + ∑ (actual unit cost of each batch of purchase in the current month) × Purchase quantity of each batch in the current month)] / (sum of inventory quantity at the beginning of the month + purchase quantity of each batch in the current month) cost_ = (cost0+sum(df["Purchase quantity"]*df["Purchase price"]))/(num0+df["Purchase quantity"].sum()) #Month end inventory cost = month end inventory quantity × Inventory unit cost cost_end = (num0+df["Purchase quantity"].sum()-df["sales volumes"].sum())*cost_ print(cost_end) # First in first out method import queue #Generate a first in first out queue, transfer the price by quantity, and construct the actual price sequence Q=queue.PriorityQueue() for i in df.values: num_c,price = i[1],i[3] for j in range(num_c): Q.put(price) real_price_list = [] all_sale_num = df["sales volumes"].sum() # Take out by sales order for k in range(all_sale_num): real_price = Q.get() real_price_list.append(real_price) # Summary summation real_cost = sum(real_price_list) print(f"Actual cost of sales:{real_cost}")
2. Score statistics and triangle printing
Score statistics and triangle printing
Title Description: Take a look at questions two and four
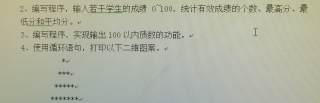
# Second question scores = input('Please enter several student grades to","separate:') real_score_list = [i for i in scores.split(",") if (int(i)>=0)&(int(i)<=100)] print(f"Number of valid scores:{len(real_score_list)}") print(f"Highest score:{max(real_score_list)}") print(f"Minimum score:{min(real_score_list)}") # Question 4 for i in range(1,8,2): for j in range(0, int((7 - i)/2)): print(end=" ") for k in range(i): print("*", end=" ") print("")
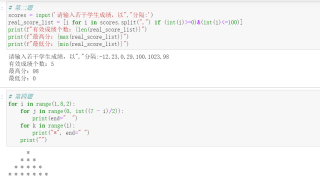
3. Interface request, parameter extraction from text, how to operate, and complete code
Interface request, parameter extraction from text, how to operate, and complete code
Title Description: Please give examples of how to operate post and get respectively url and request parameters, read TXT, and the txt name is 3 txt Twice between two paragraphs / n
txt content is
api.sssbrowser.com:443/v1/sec/policy [('device', '1'), ('vpn_user', '0'), ('sign', '1a309eb59366b4c1d50620e393f8e585031de595340c04415333314bb2eb1859'), ('mid', 'c28e53d37b50f100ffd14b0519b74a2b'), ('timestamp', '2021-10-28T10:21:37+08:00'), ('app_name', 'free.speedvpn.video.download'), ('timezone', 'Asia/Shanghai'), ('format', 'JSON'), ('campaign', 'default'), ('sign_version', '2.0.0'), ('sign_method', 'SHA256'), ('appver', '2.0.3.1020'), ('lang', 'zh-hans'), ('version', '20201105'), ('signnonce', '16353876973872634482'), ('channel', '0011'), ('imsi', ''), ('netop', ''), ('lang_region', 'zh-CN'), ('region', 'CN')] api.sssbrowser.com:443/video/videos/discover [('device', '1'), ('vpn_user', '0'), ('sign', '4c9619f3349b6663c9f818bd77210339448253eaf88024e15a2dd0d7da444ba2'), ('mid', 'c28e53d37b50f100ffd14b0519b74a2b'), ('timestamp', '2021-10-28T10:21:37+08:00'), ('app_name', 'free.speedvpn.video.download'), ('timezone', 'Asia/Shanghai'), ('format', 'JSON'), ('campaign', 'default'), ('sign_version', '2.0.0'), ('sign_method', 'SHA256'), ('appver', '2.0.3.1020'), ('lang', 'zh-hans'), ('version', '20201105'), ('signnonce', '16353876974719319961'), ('channel', '0011'), ('imsi', '')]
import json import requests def handle_txt(txt_path): f = open(txt_path) parm = f.readlines() parm.remove("\n") f.close() parm_dic = (dict(zip(parm[::2], parm[1::2]))) return parm_dic def post_get_url(base_url): for p in parm_dic: url = base_url + p.split(":")[1][:-1] parm_data = json.loads( parm_dic[p].replace("[", "{").replace("]", "}").replace("(", "").replace(",", ":").replace("):", ",").replace( ")", "").replace("'", '"')) # # get # response = requests.get(url, params=parm_data) # post response = requests.post(url, params=parm_data) print(response.text) parm_dic = handle_txt("3.txt") base_url = "xxxxxx" post_get_url(base_url)
4. How to solve the problem that pycharm crawls data and stores it in mysql repeatedly?
How to solve the problem that pycharm crawls data and stores it in mysql repeatedly?
Title Description: How can pycham avoid printing and storing mysql multiple times when writing code
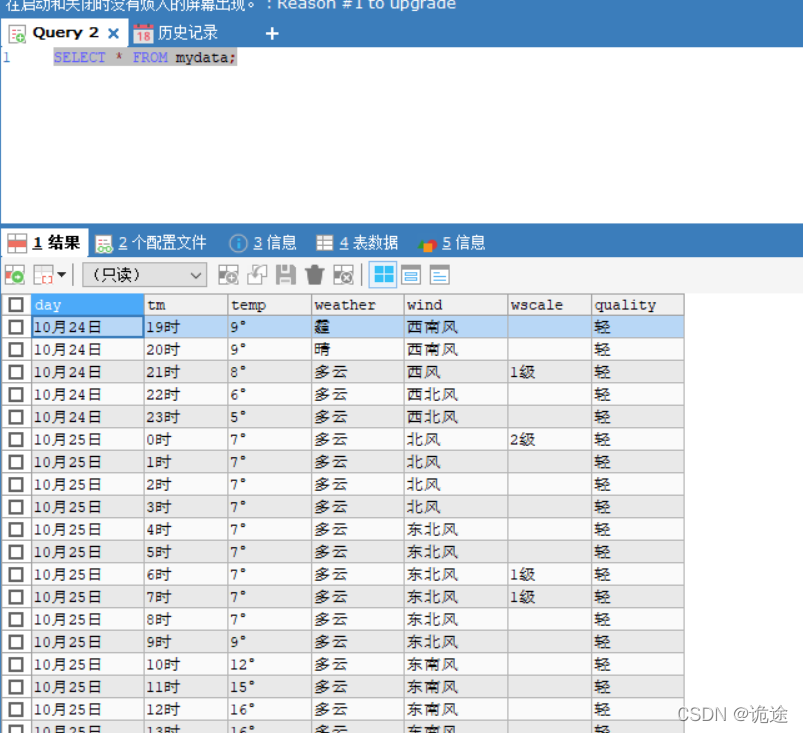
Here's the repetition,
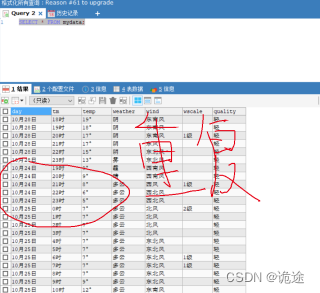
How to write code before saving it and climb it only once My code is the same as others. Why is it repeated? Didn't they find this problem?

It's all print. Why did you print it three times?
answer: 1. mysql sets the primary key, 2. De duplication before warehousing 1 is better. If there are duplicates in two documents, 2 cannot be avoided
5. Enter an integer and find the product of each digit
Enter an integer and find the product of each digit
Title Description Enter an integer and find the product of each digit For example: Input 123, output 6 Input 319, output 27 Input 110, output 0
# Extract each number from the for loop. Pay attention to the format under int conversion and numpy quadrature function prod import numpy as np a = input() print(np.prod( [int(i) for i in a]))