Data structure and algorithm (Java language implementation) -- linear table
- 1, Review and study of Java array
- 2, Writing variable length arrays using OOP
- 2.0 preparation
- 2.1 implement add to dynamically add an element
- 2.2 implement delete to delete elements at any position
- 2.3 implement the size method to obtain the length of the current array
- 2.4 implement get to obtain the element of the specified subscript
- 2.5 insert to insert an element at any position
- 2.6 implement set to replace any element
- 3, Stack implementation (stack)
- 4, Implementation of Queue
1, Review and study of Java array
When learning the basics of Java, we often use arrays to store the same type of data. Let's briefly review the simple use of Java arrays. Students who really forget how to use Java arrays can view this article Use of Java arrays
import java.util.Arrays; public class TestArray { public static void main(String[] args) { // Create an array int[] arr = new int[5]; // Get array length int len = arr.length; // Loop assignment to array for (int i=0;i<len;i++) { arr[i] = i*i; } // Traversal array for (int i=0; i<len; i++) System.out.println(arr[i]); // Displays all elements in the array System.out.println(Arrays.toString(arr)); } }
The above is the array api provided by the system. Based on this, we can complete many conventional operations. But the built-in array sometimes brings great limitations?
- The array is of fixed length. We need to modify the data dynamically. How can we solve it?
- For example, adding an element to an array? You can use existing data structures such as List ArrayList
- How to delete an element?
- Modifying an element is very simple. You can directly replace the value of the corresponding subscript
- What happens when you insert an element anywhere?
However, if a variable length array can appear, these problems can be easily solved.
2, Writing variable length arrays using OOP
Note: if we want to pass in any data type, we can change the int array to object
2.0 preparation
Define a Java class of MyArray, then add a private array attribute, and initialize the array to an array with length 0 using the parameterless constructor
/** * Encapsulated array * */ public class MyArray { private int[] elements;// An array that stores data. If other types are stored, it is declared as Object public MyArray() { elements=new int[0]; } }
2.1 implement add to dynamically add an element
When we use a fixed length array, we need to add an element to the array. At this time, one way we think of is to create a new array to expand the capacity. Follow this idea and think about it. Is it much easier?
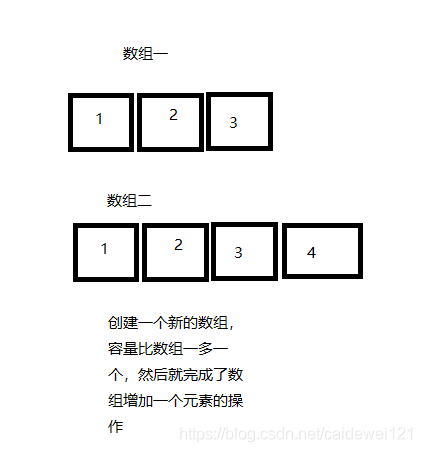
When we have the idea, we will implement it in code
// We want to add an element, so we need to pass a parameter to add an element public void add(int element) { // Create an array space with one more element than the capacity of the element group int[] newArr = new int[elements.length+1]; // Assign a value to a new array using a loop for(int i=0;i<elements.length;i++) { newArr[i] = elements[i]; } // After the assignment, put the new element in the last position of the array, and fill in elements.length here. You can carefully think about the positional relationship between the following subscripts and length newArr[elements.length] = element; // Just update the array elements = newArr; }
2.2 implement delete to delete elements at any position
Deleting an element can be divided into two cases:
- One is to delete the end element
- One is to delete the middle element
It's easy to delete the last element. Just subtract the length of the array by one, and then re assign the remaining elements to a new element
To delete an element in the middle, consider whether it is out of bounds. If it is not out of bounds, re assign the elements in front of the deleted element, and the elements behind the deleted element are directly assigned to the new array
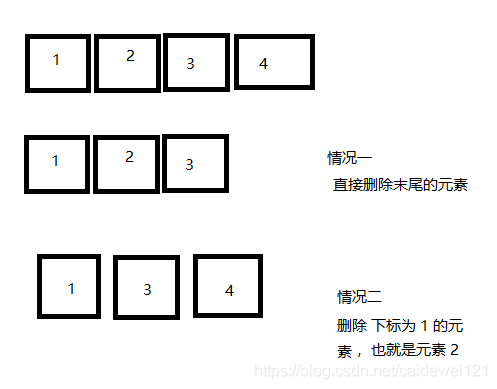
// Delete elements in array public void delete(int index) { // Judge whether the subscript is out of bounds if (index<0 || index>elements.length-1) { throw new RuntimeException("Subscript out of bounds"); } int newArr[] = new int[elements.length-1]; // Copy original data to new array for (int i=0; i<newArr.length; i++) { if (i<index) { newArr[i] = elements[i]; } else { // Want to delete the following element newArr[i] = elements[i+1]; } } // Replace array with new array elements = newArr; }
2.3 implement the size method to obtain the length of the current array
Directly return the length of the current array
public int size() { return elements.length; }
2.4 implement get to obtain the element of the specified subscript
The user passes in a subscript value, and then judges whether the passed in value is reasonable. If it is unreasonable, an exception is returned. Otherwise, the data will be returned normally
public int get(int index) { if (index<0 || index >elements.length) throw new RuntimeException("Subscript out of bounds"); return elements[index]; }
2.5 insert to insert an element at any position
It's the same as deleting an element, but adding an element anywhere
// Inserts an element into a specified location public void insert(int index, int element) { // Create a new array int[] newArr = new int[elements.length + 1]; for (int i=0;i<newArr.length;i++) { // The previous data are consistent if (i<index) { newArr[i] = elements[i]; } else { // The following data is left at the insertion position newArr[i+1] = elements[i]; } } // Implement insert operation newArr[index] = element; // Array replacement elements = newArr; }
2.6 implement set to replace any element
The replacement only requires the user to pass in the subscript value and the element to be replaced
// Replace the element at the specified location public void set(int index, int element) { elements[index] = element; }
3, Stack implementation (stack)
3.1 basic characteristics of stack
Stack is a single in single out data structure. The stack can only be in at one end and out at one end. Now draw a picture to deepen your impression
- The stack has two parts: the bottom of the stack and the top of the stack
- Every time you enter and exit the stack, you enter and exit from the top of the stack
- The first element in the stack will be pressed directly into the bottom of the stack, and then slowly piled to the top of the stack until the stack is full
- Stack has the characteristics of first in, last out and last in and out
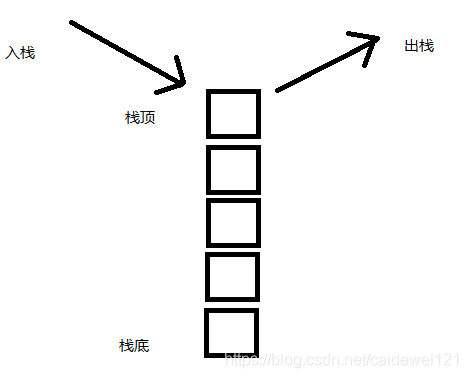
3.2 preparation for stack implementation
I use dynamic arrays to complete stack operations. Of course, the space of a stack should be specified at the beginning.
/** * Stack, using arrays to store data * Every time you add an element to the top of the stack * */ public class MyStack { int[] elements; // Stack initialization public MyStack() { elements = new int[0]; } }
3.3 push of stack implementation
// push on the stack, and put the data at the end of the stack public void push(int element) { int newArr[] = new int[elements.length+1]; for (int i=0;i<elements.length-1;i++) { newArr[i] = elements[i]; } // newArr[elements.length] = element; // Update array elements = newArr; }
3.4 stack out of stack (pop)
// pop out of the stack and take the top element of the stack public int pop() { if (elements.length == 0) { throw new RuntimeException("tack is empty!"); } // Go to the last element int element = elements[elements.length-1]; // Create a new array int newArr[] = new int[elements.length-1]; for (int i=0;i<elements.length-1;i++) { newArr[i] = elements[i]; } elements = newArr; // Return stack top element return element; }
3.5 stack implementation view stack top element (peek)
//View stack top element public int peek() { if (elements.length == 0) { throw new RuntimeException("stack is empty!"); } return elements[elements.length-1]; }
3.6 stack implementation judgment stack is empty (isEmpty)
// Determine whether the stack is empty public boolean isEmpty() { return elements.length == 0; }
4, Implementation of Queue
4.1 basic characteristics of queue
- The queue has two ends, one is the head of the team, and the other is the tail
- Queue entry elements generally enter from the head of the queue
- Queue out elements are generally out from the end of the queue
- The characteristics of the queue are first in first out, last in and last out
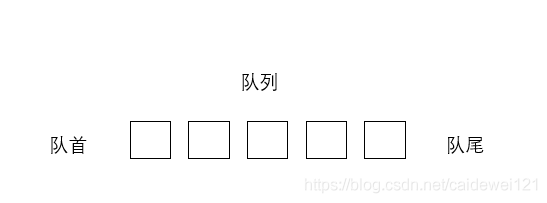
4.2 queue entry (add)
The first time with PPT animation
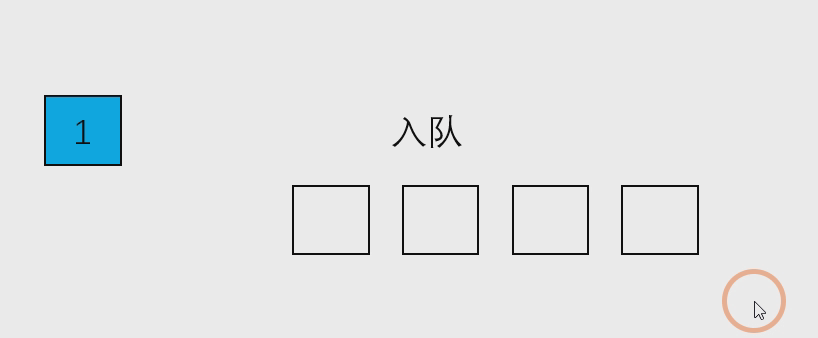
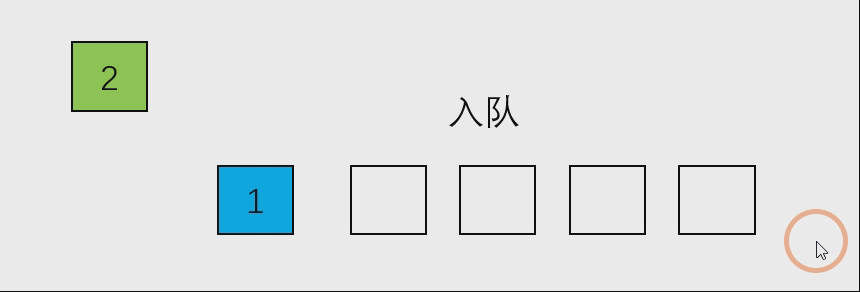
// Join the team public void add(int element) { int newArr[] = new int[elements.length + 1]; // Array copy for (int i=0;i<elements.length;i++) { newArr[i] = elements[i]; } newArr[elements.length] = element; this.elements = newArr; }
4.3 pull out of queue
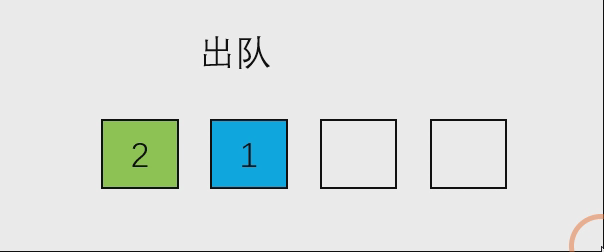
// Out of the team public int pull() { int element = elements[0]; // Take out the first number int newArr[] = new int[elements.length-1]; // Here, the length of the new array is used to copy for (int i=0;i<newArr.length;i++) { newArr[i] = elements[i+1]; } this.elements = newArr; return element; }
4.4 judging whether the queue is empty (isEmpty)
// Determine whether the queue is empty public boolean isEmpty() { return this.elements.length == 0; }