catalogue
3.1 tail insertion of sequence table
3.2 # sequential header insertion
3.3 # insert data at the specified location
3.4 delete the end of the sequence table
3.5 deletion of sequence header
3.6 delete data at the specified location
1. Linear table
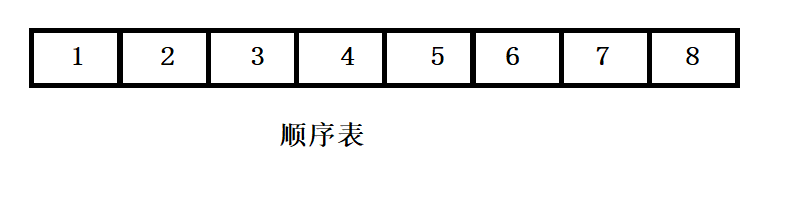
2. Sequence table
2.1 concept of sequence table
// Requirements: the stored data shall be stored continuously from 0 // Static sequence table // Problem: it's too small to use. It's too big. There's waste. #define N 10000 struct SeqList { int a[N]; int size; // Record how many pieces of data are stored };
Dynamic order table: use dynamic array storage.
Obviously, if the space is not enough, the capacity can be increased (flexible and practical)
2.2 interface
// Dynamic sequence table typedef struct SeqList { SLDataType* a; int size; // Number of stored data int capacity; // Storage space size }SeqList; // Basic addition, deletion, query and modification interface // Sequence table initialization void SeqListInit(SeqList* psl, size_t capacity); // Check the space. If it is full, increase the capacity void CheckCapacity(SeqList* psl); // End of sequence table void SeqListPushBack(SeqList* psl, SLDataType x); // Sequential tail deletion void SeqListPopBack(SeqList* psl); // Sequential header insertion void SeqListPushFront(SeqList* psl, SLDataType x); // Sequential header deletion void SeqListPopFront(SeqList* psl); // Sequential table lookup int SeqListFind(SeqList* psl, SLDataType x); // Insert x in pos position in sequence table void SeqListInsert(SeqList* psl, size_t pos, SLDataType x); // The sequence table deletes the value of pos position void SeqListErase(SeqList* psl, size_t pos); // Sequence table destruction void SeqListDestory(SeqList* psl); // Sequence table printing void SeqListPrint(SeqList* psl);
3. Interface implementation
3.1 tail insertion of sequence table
void SeqListPushBack(SeqList* psl, SLDataType x) { assert(psl); /*SeqListCheckCapacity(psl); psl->a[psl->size] = x; psl->size++;*/ SeqListInsert(psl, psl->size, x); }
Note: for tailoring data, first consider whether the space is enough. If not, increase the capacity. Insert data after capacity increase.
Capacity increasing function:
void SeqListCheckCapacity(SeqList* psl) { assert(psl); // If it's full, we'll expand it if (psl->size == psl->capacity) { size_t newCapacity = psl->capacity == 0 ? 4 : psl->capacity * 2; SLDataType* tmp = realloc(psl->a, sizeof(SLDataType) * newCapacity); if (tmp == NULL) { printf("realloc fail\n"); exit(-1); } else { psl->a = tmp; psl->capacity = newCapacity; } } }
3.2 # sequential header insertion
void SeqListPushFront(SeqList* psl, SLDataType x) { assert(psl); //SeqListCheckCapacity(psl); Move the data to make room for your head //int end = psl->size - 1; //while (end >= 0) //{ // psl->a[end + 1] = psl->a[end]; // --end; //} //psl->a[0] = x; //psl->size++; SeqListInsert(psl, 0, x); }
Note: the head must be moved forward from the back when inserting.
3.3 # insert data at the specified location
void SeqListInsert(SeqList* psl, size_t pos, SLDataType x) { // Violence check assert(psl); // Mild examination if (pos > psl->size) { printf("pos Cross border:%d\n", pos); return; //exit(-1); } // Violence check //assert(pos <= psl->size); SeqListCheckCapacity(psl); //int end = psl->size - 1; //while (end >= (int)pos) //{ // psl->a[end + 1] = psl->a[end]; // --end; //} size_t end = psl->size; while (end > pos) { psl->a[end] = psl->a[end - 1]; --end; } psl->a[pos] = x; psl->size++; }
be careful:
1: The coverage is still from back to front.
2: The value of pos must be less than size, otherwise it will cross the boundary (because it is an unsigned integer of size_t, it must be greater than 0)
3: When pos is equal to size, the function is equivalent to tail insertion, and when pos is equal to 0, the function is equivalent to head insertion.
3.4 delete the end of the sequence table
void SeqListPopBack(SeqList* psl) { assert(psl); //psl->a[psl->size - 1] = 0; /*if (psl->size > 0) { psl->size--; }*/ SeqListErase(psl, psl->size - 1); }
You can delete the tail directly.
3.5 deletion of sequence header
void SeqListPopFront(SeqList* psl) { assert(psl); // Move data overwrite delete /*if (psl->size > 0) { int begin = 1; while (begin < psl->size) { psl->a[begin - 1] = psl->a[begin]; ++begin; } --psl->size; }*/ SeqListErase(psl, 0); }
Note: header deletion is from front to back
3.6 delete data at the specified location
void SeqListErase(SeqList* psl, size_t pos) { assert(psl); assert(pos < psl->size); size_t begin = pos + 1; while (begin < psl->size) { psl->a[begin - 1] = psl->a[begin]; ++begin; } psl->size--; }
be careful:
1. pos must be less than the value of size (since size_t does not consider less than zero).
2. begin is defined in the next bit of pos, right front, backward, and forward coverage.
3. When pos equals 0, the function is equivalent to header deletion; when pos equals size-1, the function is equivalent to tail deletion.
3.7 finding data
int SeqListFind(SeqList* psl, SLDataType x) { assert(psl); for (int i = 0; i < psl->size; ++i) { if (psl->a[i] == x) { return i; } } return -1; }
The returned subscript is found, but - 1 is not found.
Full code: 2022_ 03_ 09_ Table 2022_ 03_ 09_ Li · gitee - China open source data table
demonstration:
4. Menu
#define _CRT_SECURE_NO_WARNINGS #include "SeqList.h" void menu() { printf("***********************************************\n"); printf(" 1.Tail interpolation data \n"); printf(" 2.Header data \n"); printf(" 3.Insert data at the specified location \n"); printf(" 4.Tail deletion data \n"); printf(" 5.Header deletion data \n"); printf(" 6.Delete data at the specified location \n"); printf(" 7.print data \n"); printf(" 8.Find data \n"); printf(" -1.sign out \n"); printf("***********************************************\n"); } int main() { //TestSeqList5(); SeqList s; SeqListInit(&s); int option = -1; do { menu(); printf("Please select your operation:>"); scanf("%d", &option); if (option == 1) { printf("Please enter the data you want to insert:>"); //int val = 0; SLDataType val = 0; scanf("%d", &val); SeqListPushBack(&s, val); } else if (option == 2) { printf("Please enter the data you want to insert:>"); SLDataType val = 0; scanf("%d", &val); SeqListPushFront(&s, val); } else if (option == 3) { printf("Please enter the location you want to insert pos And data x:>"); SLDataType val = 0; int x = 0; scanf("%d %d", &val, &x); SeqListInsert(&s, val - 1, x); } else if (option == 4) { SeqListPopBack(&s); printf("Tail deletion succeeded!\n"); } else if (option == 5) { SeqListPopFront(&s); printf("Header deleted successfully!\n"); } else if (option == 6) { printf("Please enter the location you want to delete pos:>"); int x = 0; scanf("%d", &x); SeqListErase(&s, x - 1); } else if (option == 8) { printf("Please enter the data you are looking for x:>"); int x = 0; scanf("%d", &x); int i = SeqListFind(&s, x); if (i == -1) { printf("The data does not exist\n"); } else { printf("The subscript of this data is%d\n", i); } } else if (option == 7) { SeqListPrint(&s); } } while (option != -1); SeqListDestroy(&s); return 0; }
(end of this chapter)