preface
★ this is Xiao Leng's blog Current series: data structure series Source code git warehouse‘ Data structure code address Code Git warehouse address
Linked list
Introduction to linked list:
A linked list is an ordered list, but it is stored in memory as follows
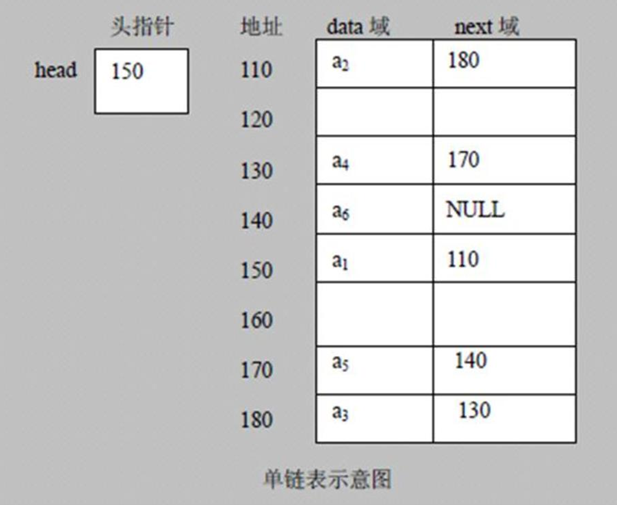
- Linked list is stored in the form of nodes, which is chain storage
- Each node contains data field and next field: points to the next node
- As shown in the figure: it is found that each node of the linked list is not necessarily stored continuously
- The linked list is divided into the linked list with the leading node and the linked list without the head node, which is determined according to the actual needs
Unidirectional linked list
One way linked list storage node diagram
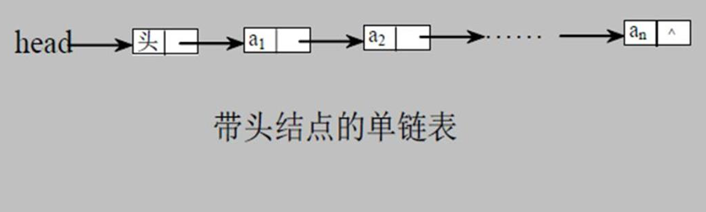
Application example of single linked list
Use the one-way linked list with head to realize the management of Water Margin hero ranking list, and complete the addition, deletion, modification and query of heroes
We all know that there are 108 heroes in the water margin
Let's just give four examples here. We want to put them into our hero list (one-way linked list) in the way of linked list
Ideas of adding, modifying and deleting
Add Hero:
Analysis diagram of the idea of inserting heroes into the specified position according to the ranking (if there is this ranking, the addition fails and a prompt is given):
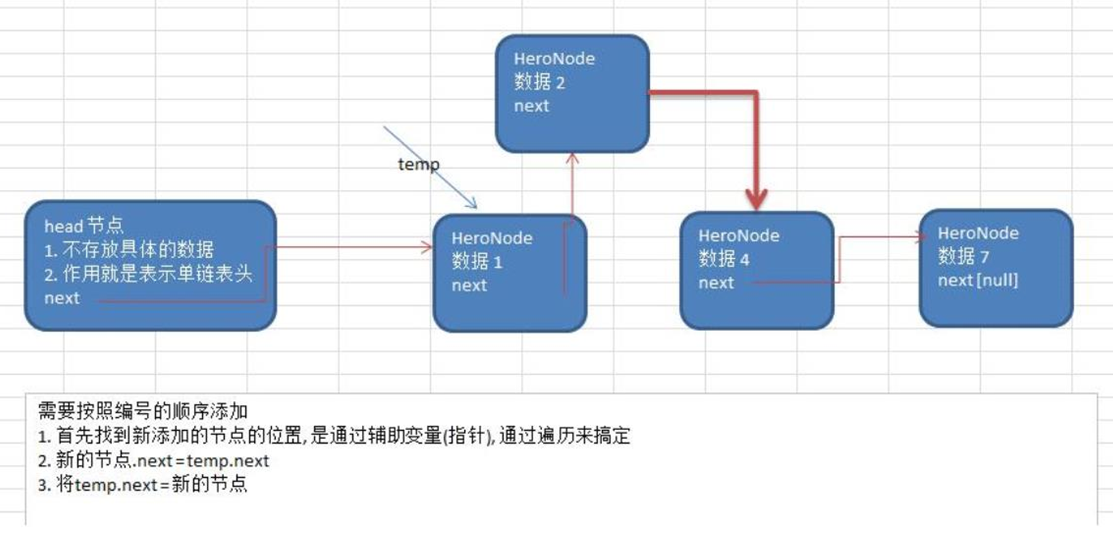
Modify node functions
In ancient times, people with martial arts like to fight for ranking, so we need to have the function of changing the ranking when making the hero list
The ranking is fixed, and his next name is also fixed. We only need to modify the current ranking person and nickname
- Find the node first, and through traversal,
- temp.name = newHeroNode.name ; temp.nickname= newHeroNode.nickname
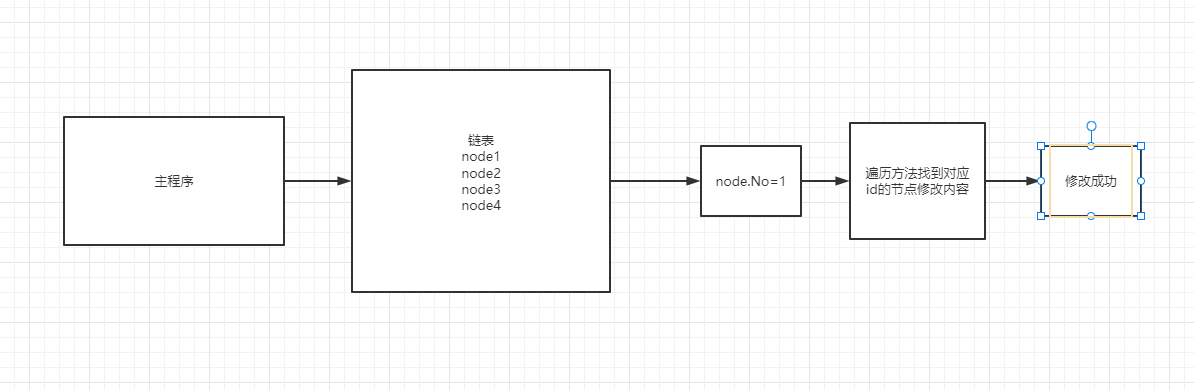
Delete node
This function can be understood as that some heroes don't want to compete for ranking. When they retire and go home to farm and enjoy life, we need to free up the positions occupied by heroes who don't need positions.
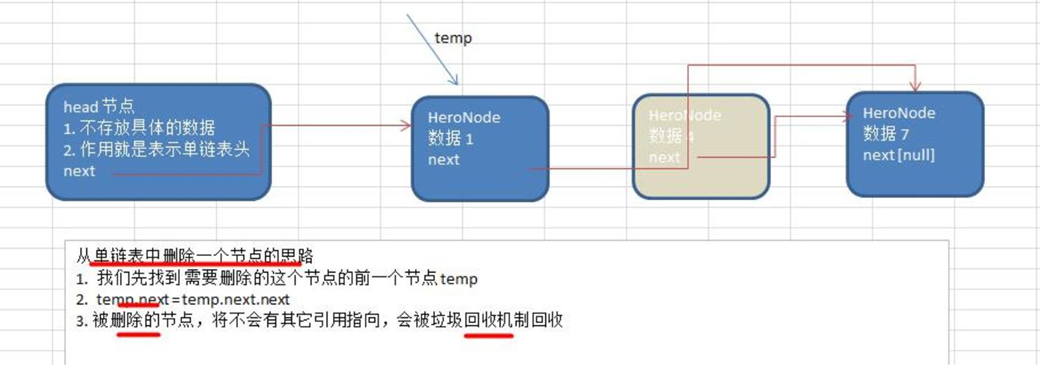
Addition, deletion, modification and query of one-way linked list
/** * @projectName: DataStructure * @package: com.hyc.DataStructure.LinkedList * @className: LinkedlistDemo * @author: Cold Huanyuan doorwatcher * @description: TODO * @date: 2021/12/17 16:24 * @version: 1.0 */ public class LinkedlistDemo { public static void main(String[] args) { // Set some hero objects HeroNode heroNode = new HeroNode(1, "Song Jiang", "Timely rain"); HeroNode heroNode1 = new HeroNode(2, "Lu Junyi", "Jade Kirin"); HeroNode heroNode2 = new HeroNode(3, "Wu Yong", "Zhiduoxing"); HeroNode heroNode3 = new HeroNode(4, "Lin Chong", "Leopard head"); // Declare a one-way linked list SingleLinkedlist linkedlist = new SingleLinkedlist(); //Join our hero node //linkedlist.add(heroNode); //linkedlist.add(heroNode1); //linkedlist.add(heroNode2); //linkedlist.add(heroNode3); //Add by number linkedlist.addByOrder(heroNode); linkedlist.addByOrder(heroNode3); linkedlist.addByOrder(heroNode2); linkedlist.addByOrder(heroNode1); //Output node information linkedlist.List(); System.out.println("After updating data"); linkedlist.updateNode(new HeroNode(1, "Leng Huanyuan", "Coding master")); //Output node information linkedlist.List(); System.out.println("Output after deleting data"); linkedlist.DeleteNode(1); linkedlist.List(); } } class SingleLinkedlist { //Create a header node HeroNode head = new HeroNode(0, "", ""); //Add linked list public void add(HeroNode node) { //The head node cannot be moved. Here we use a temporary pointer to record HeroNode temp = head; //Traversal linked list while (true) { //If the traversal is empty, the last node is found //If it is not empty, move back one bit and continue to find if (temp.next == null) { break; } //If it is not found, move back temp temp = temp.next; } //Jump out of while to prove that the last node is found, and add our new node to the next of the last node temp.next = node; } //The second method of adding nodes public void addByOrder(HeroNode node) { /* * Because the head node cannot move, we record the head node through the pointer to help find the added position *Because it is a single linked list, we are looking for Temp, which is the previous node at the addition location, otherwise it cannot be inserted * */ //Create a temporary variable HeroNode temp = head; //It is used to identify whether the hero exists. The default is not exists (false) boolean flag = false; while (true) { //Find the last empty location if (temp.next == null) { break; } //If the next no of temp is greater than the node we join, join to temp if (temp.next.No > node.No) { break; } //If the next node is equal to the No of the joining node, it means that a node already exists else if (temp.next.No == node.No) { //Change the flag to true to indicate that it already exists flag = true; break; } //If none of the above achievements are reached, it means that the current node position is wrong, and continue to traverse backward temp = temp.next; } // Judge the value of flag if (flag) { //If true, the node already exists System.out.printf("Current node%d Already exists, cannot join\n", node.No); } else { // If false, it means that the insertion conditions are met and there is no link with the current linked list node.next = temp.next; temp.next = node; } } //Modify node information public void updateNode(HeroNode newHeroNode) { if (head.next == null) { System.out.println("The linked list is empty"); } //This is the header node and cannot be modified. I use temp to point to the header node HeroNode temp = head.next; // Did you find the ID of no boolean flag = false; while (true) { //Find the last one if (temp == null) { break; } if (temp.No == newHeroNode.No) { //If equal to, it means that it can be modified flag = true; break; } temp = temp.next; } if (flag) { // true modify information temp.NickName = newHeroNode.NickName; temp.Name = newHeroNode.Name; } else { System.out.printf("Can't find %d This node", newHeroNode.No); } } //Delete node information public void DeleteNode(int no) { HeroNode temp = head; // Used to mark whether it can be deleted boolean flag = false; while (true) { if (temp.next == null) { break; } if (temp.next.No == no) { flag = true; break; } temp = temp.next; } //Delete Node according to flag if (flag) { //If found, delete it. Here we just need to point to the empty GC and recycle it temp.next = temp.next.next; } else { System.out.printf("To delete %d Can't find", no); } } //Traversal linked list public void List() { if (head.next == null) { System.out.println("The linked list is empty"); return; } //The head node cannot be moved. Here we use a temporary pointer to record HeroNode temp = head.next; while (true) { //If the traversal is empty, the last node is found if (temp == null) { break; } //Output node System.out.println(temp); //Move back one bit temp = temp.next; } } } /** * Write the water margin hero node * Used to store the attributes of each hero * */ class HeroNode { //ranking public int No; // full name public String Name; //nickname public String NickName; // Who's next public HeroNode next; public HeroNode(int hNo, String hName, String hNickName) { this.No = hNo; this.Name = hName; this.NickName = hNickName; } //Convenient output statement output @Override public String toString() { return "HeroNode{" + "No=" + No + ", Name='" + Name + '\'' + ", NickName='" + NickName + '\'' + '}'; } }
Use effect of linked list
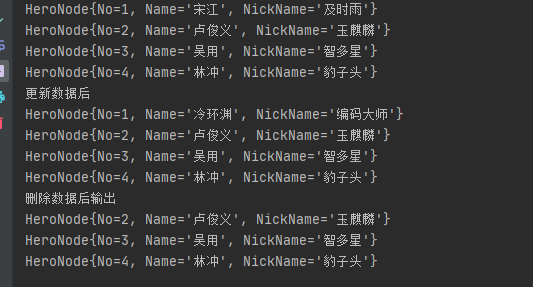
summary
We made our own hero list (one-way linked list) this time. What did we receive?
- Idea of connection between nodes
- Gradually adapt to some ideas of data structure
- Hands on