catalogue
Sequential storage structure (static allocation)
Five operations in which top is an integer (when top points to the top element of the stack)
Sequential storage structure (dynamic allocation)
Five operations in which top is an integer (when top points to the top element of the stack)
5. Find the length of the stack
Basic operation of chain stack
Basic operation of circular queue
Judge whether the queue is empty
Stack
definition
1. Linear tables that can only be inserted and deleted at the top of the stack
2. Last in first out
Sequential storage structure (static allocation)
# define MaxSize 100 / / define the maximum length of a static sequential stack typedef int SElemType; typedef struct { SElemType base[MaxSize];//Define a one-dimensional array to store stack data elements int top; //Stack top pointer, which always points to the next position of the stack top element when the stack is not empty int StackSize;//Define a variable to store the current length of the sequence stack }SeqStack;
Five operations in which top is an integer (when top points to the next position of the top element of the stack)
1. Initialize S.top=0
2. Empty stack S.top==0
3. Element stack S.base[S.top++]=e (add after advanced)
4. Stack full s.top > = stacksize
5. Element out of stack e=S.base[--S.top] (subtract first and then out)
Five operations in which top is an integer (when top points to the top element of the stack)
1. Initialize S.top=-1
2. Empty stack S.top==-1
3. Element stack S.base[S.++top]=e (add first and then enter)
4. Stack full s.top > = stacksize-1
5. Element out of stack e=S.base[S.top --] (first out and then minus)
Sequential storage structure (dynamic allocation)
#define STACK_INIT_SIZE 10 / / initial allocation of storage space #define STACK_INCREMENT 2 / / storage space allocation increment typedef int SElemType; typedef struct SqStack { SElemType *base; //Stack bottom pointer, always pointing to the bottom of the stack int *top; //The stack top pointer can point to the next position of the stack top element or point to the stack top element int StackSize; //The maximum storage capacity in elements that can be used by the currently allocated stack }SqStack; //Stack order
Five operations where top is a pointer (when top points to the next position of the top element of the stack)
1. Initialize S.top=S.base
2. Empty stack S.top==S.base
3. Element stacking * S.top++=e (add after advanced)
4. Stack full s.top-s.base > = stacksize
5. Element out of stack e=*--S.top (subtract first and then out)
Five operations in which top is an integer (when top points to the top element of the stack)
1. Initialize S.top=S.base-1
2. Empty stack S.top==S.base-1
3. Element stack * + + S.top=e (add first and then enter)
4. Stack full s.top-s.base > = stacksize-1
5. Element out of stack e=*S.top -- (first out and then minus)
Basic operation of sequential stack (top is the pointer & when pointing to the next position of the top element of the stack)
1. Construct empty stack
//Construct an empty stack, and return 1 if successful; If it fails, return 0 int InitStack(SqStack *S) { S->base=(SElemType *)malloc(STACK_INIT_SIZE*sizeof(SElemType)); if (!S->base) return 0; //Storage allocation failed S->top=S->base; S->StackSize=STACK_INIT_SIZE; return 1; }
2. Destroy stack
void DestroyStack(SeqStack *S) { if(S != NULL) { free(S->base); //Release stack S->top = S->base =NULL; S->StackSize=0; } }
3. Empty stack
void ClearStack(SqStack *S) { S->top=S->base; }
4. Empty stack
//If stack S is an empty stack, it returns 1; otherwise, it returns 0 int StackEmpty(SqStack S) { if(S.top==S.base) return 1; else return 0; }
5. Find the length of the stack
int LengthStack(SqStack S) { if((S.base !=NULL) &&(S.top!=NULL)) return(S.top-S.base); }
6. Get stack top element
//If the stack is not empty, use e to return the top element of S and return 1; Otherwise, return 0 int GetTop(SqStack S,SElemType *e) { if(S.top>S.base) { *e=*(S.top-1); return 1; } else return 0; }
7. Stack
//The inserted element e is a new stack top element. If the insertion is successful, it returns 1, otherwise it returns 0 int Push(SqStack *S,SElemType e) { if(S->top - S->base> = S->StackSize)//Stack full, additional storage space { S->base=(SElemType *) realloc(S->base,(S->StackSize+STACKINCREMENT)*sizeof(SElemType)); if(!(S->base) return 0; //Storage allocation failed S->top=S->base+S->StackSize;/*Modify stack top pointer*/ S->StackSize+=STACKINCREMENT; } *(S->top)++=e;//Put e on the stack and become a new top element return 1; }
8. Out of the stack
//If the stack is not empty, delete the top element of S, return its value with e, and return 1; Otherwise, return 0 int Pop(SqStack *S,SElemType *e) { //Stack empty if(S->top==S->base) return 0; //Assign the element at the bottom of the stack to e, and the pointer at the top of the stack moves down *e=*--S->top; return 1; }
9. Traverse the stack
void StackTraverse(SqStackS,void (*visit)(SElemType)) { while(S.top>S.base) visit(*S.base++); }
Basic operation of chain stack
Linked Storage Structure 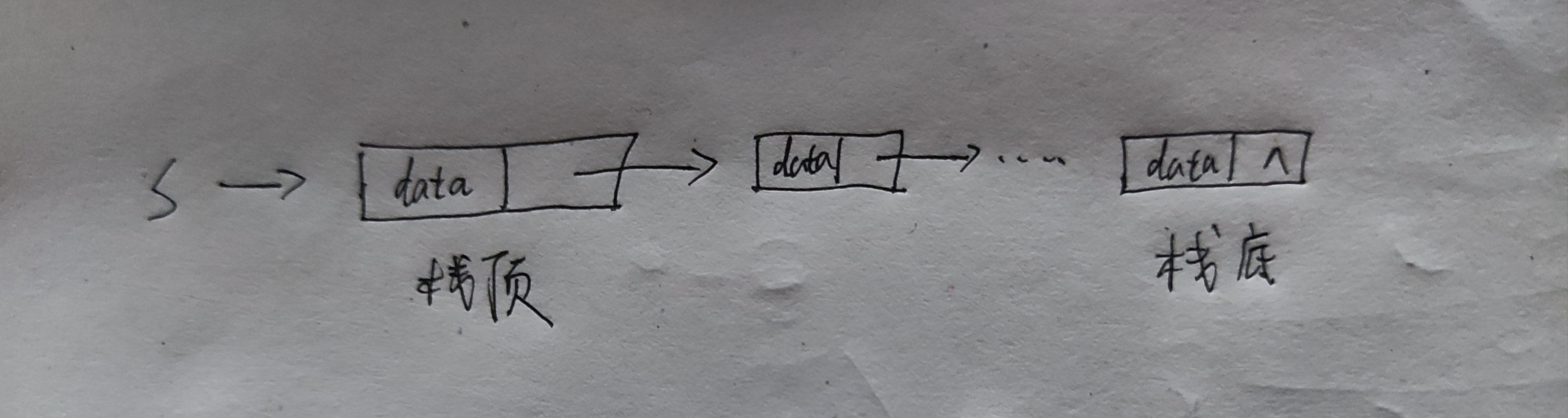
typedef struct node { elemtype data; struct node *next; }NODE,*NODEPTR #define LEN sizeof(NODE)
1. Construct empty stack
int InitStack() { NODEPTR top; top=(NODEPTR)malloc(LEN); if(top==NULL) { printf(""Failed"); return 0; } top->next=NULL; return 1; }
2. Stacking
int Push_Stack(NODEPTR *top,elemtype e) { NODEPTR p; p=(NODEPTR)malloc(LEN); if(p==NULL) { printf("The system failed to allocate space! "); return 0; } p->data=e; //Save new node element value p->next=top->next;//The new node is connected to the top of the original stack top->next=p; //New node as new stack top return 1; }
3. Out of the stack
int Pop_Stack(NODEPTR *top,elemtype *e) { NODEPTR p; p=top->next; if(p==NULL) { printf("Stack is empty, unable to operate! "); return0; } *e=p->data; //Get the element value of the top node of the stack top->next =p->next; //Move the stack top pointer backward, that is, delete the stack top node free(p); //Free the memory space occupied by the deleted node return 1; }
queue
definition
1. Rear, insert element, Front delete
2. First in first out
Sequential queue
structure
#define MaxSize 10 / / maximum capacity of sequential queue typedef typedef int ElemType; struct { ElemType *data;//Defines the storage space for queue elements int front,rear; //Define queue head and tail pointers }SqQueue; SqQueue *sq;//Defines a pointer variable to the queue sq->data=(ElemType *)malloc(MaxSize*sizeof(ElemType));//Storage space of application sequence queue
Basic operations (front is the current position of the queue head element, and rear is the next position of the queue tail element)
1.Empty queue sq->front=sq->rear=0; 2.Join the team sq->data[sq->rear]=a; //Add new element a to the team sq->rear++; 3.Out of the team *e=sq->data[sq->front];//Remove team head element a from the team sq-> front++;
Basic operations (front is the previous position of the queue head element, and rear is the current position of the queue tail element)
1.Empty queue sq->front=sq->rear=-1; 2.Join the team sq->rear++; sq->data[sq->rear]=a; //Add new element a to the team 3.Out of the team sq-> front++; *e=sq->data[sq->front];//Remove team head element a from the team
Basic operation of circular queue
1.Empty queue Q.rear= Q. front 2.Join the team sq->rear=(sq->rear+1)%MaxSize; sq->data[sq->rear]=a; //Add new element a to the team 3.Out of the team sq->front=(sq->front+1)%MaxSize; *e=sq->data[sq->front];//Remove team head element a from the team 4.Judge whether the circular queue is full (Q.rear+1)%MaxSize==Q.front 5.Length of elements in the queue (Q.rear-Q.front+MaxSize) %MaxSize
Chain queue
structure
typedef struct QNode { QElemType data; struct QNode *next; }QNode,*QueuePtr; typedef struct { QueuePtr front; //Queue head pointer QueuePtr rear; //Tail pointer }LinkQueue;
Establish empty chain queue
//Initialize a queue void InitQueue(LinkQueue*Q) { Q->front=Q->rear=(QueuePtr)malloc(sizeof(QNode)); if(!Q->front) exit(OVERFLOW);//Failed to generate header node Q->front->next=NULL; }
Judge whether the queue is empty
Status QueueEmpty(LinkQueue Q) { if(Q.front->next==NULL) return TRUE; else return FALSE; }
Join the team
//The inserted element e is the new tail element of queue Q void EnQueue(LinkQueue *Q,QElemType e) { QueuePtr p; p=(QueuePtr)malloc(sizeof(QNode));//Dynamically generate new nodes if(!p) exit(OVERFLOW); p->data=e;//Assign the value of e to the new node p->next=NULL;//The pointer to the new node is null Q->rear->next=p;//The pointer of the original tail node points to the new node Q->rear=p;//The tail pointer points to the new node }
Out of the team
//If the queue is not empty, delete the queue header element of Q and return its value with e Status DeQueue(LinkQueue *Q,QElemType *e) { QueuePtr p; if(Q->front==Q->rear) return ERROR;//Queue is empty p=Q->front->next; //p points to the queue head node *e=p->data; //Team leader element assigned to e Q->front->next=p->next;//The head node points to the next node if(Q->rear==p)//If you delete a queue tail node Q->rear=Q->front;//Modify the tail pointer to point to the head node free(p); return OK; }
Get team head element
//If the queue is not empty, use e to return the queue header element Status GetHead(LinkQueue Q,QElemType *e) { QueuePtr p; if(Q.front==Q.rear) return ERROR; p=Q.front->next;//p points to the queue head node * / *e=p->data;//Assign the value of the team head element to e return OK; }