Hello, everyone. Meet again. I'm Jun Quan.
1. Object creation mode
1.4 prototype mode
1.4.1 requirements
Create a new object by copying the original object.
1.4.2 structure
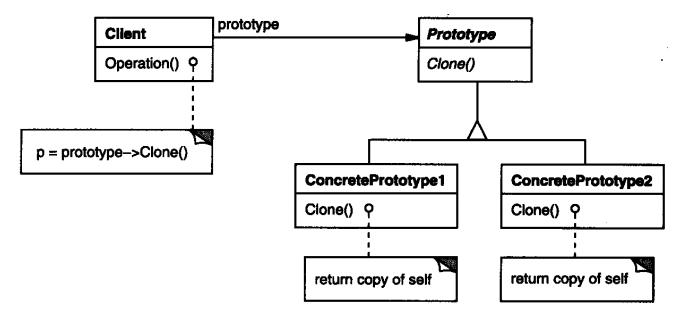
•P r o t o t y p e(Gr a p h i c)
- declare an interface to clone itself.
•C o n c r e t e P r o t o t y p e(S t a ff,W h o l e N o t e,H a l fN o t e)
- implement an operation to clone itself.
• C l i e n t(G r a p h i c To o l)
- let a prototype clone itself to create a new object.
1.4.3 example-C++
//Prototype.h #ifndef _PROTOTYPE_H_ #define _PROTOTYPE_H_ class Prototype { public: virtual ~Prototype(); virtual Prototype*Clone()const = 0; protected: Prototype(); private: }; class ConcretePrototype:public Prototype { public: ConcretePrototype(); ConcretePrototype(const ConcretePrototype& cp); ~ConcretePrototype(); Prototype* Clone() const; protected: private: }; #endif //~_PROTOTYPE_H_ Code snippet 2: Prototype.cpp //Prototype.cpp #include"Prototype.h" #include<iostream> using namespace std; Prototype::Prototype() { } Prototype::~Prototype() { } Prototype* Prototype::Clone() const { return 0; } ConcretePrototype::ConcretePrototype() { } ConcretePrototype::~ConcretePrototype() { } ConcretePrototype::ConcretePrototype(const ConcretePrototype& cp) { cout<<"ConcretePrototype copy..."<<endl; } Prototype* ConcretePrototype::Clone() const { return newConcretePrototype(*this); } //main.cpp #include"Prototype.h" #include<iostream> using namespace std; int main(int argc,char*argv[]) { Prototype* p= newConcretePrototype(); Prototype* p1=p->Clone(); return 0; }
Note: This is just a description of the concept and does not involve the common deep copy problems in C + +
1.4.4 example - JAVA
In Java, the prototype pattern can be implemented very easily. You can "clone" any object of the implementation class just by implementing the identifying interface clonable and overriding the clone() method in the interface.
class ConcretePrototype02 implements Cloneable{ private String name; private ArrayList<String> nameList = new ArrayList<String>(); public ConcretePrototype02(String name) { this.name = name; this.nameList.add(this.name); } //Objects added to nameList public void setName(String name) { this.nameList.add(name); } public ArrayList<String> getNameList() { return this.nameList; } //Override the clone() method in the Object base class, expand the access permission of this method, and return this type in detail public ConcretePrototype02 clone() { ConcretePrototype02self = null; try { self= (ConcretePrototype02) super.clone(); //The following sentence is the key to deep copy // self.nameList =(ArrayList<String>) this.nameList.clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return self; } } //Test class public class Client { public static void main(String[] args) { ConcretePrototype02prototype02 = new ConcretePrototype02("Ants... "); System.out.println(prototype02.getNameList()); //Get a copy through clone ConcretePrototype02fromClone02 = prototype02.clone(); fromClone02.setName("Little ant... "); System.out.println(fromClone02.getNameList()); System.out.println(prototype02.getNameList()); } }
Test results:
Copy the prototype before: [ant...] Copied object: [ant..., little ant...] Copied prototype: [ant... Little ant...] |
---|
After the copy is found, the nameList reference of ArrayList < string > type held by the original object will change as the copied fromClone object runs the setName() method. This is not the result we want, because it means that the prototype and the copied object share the same reference variable, which is thread unsafe. After removing the staring statement in the clone() method above, we test and get the following results, for example:
Copy the prototype before: [ant...] Copied object: [ant..., little ant...] Copied prototype: [ant...] |
---|
Using the Prototype pattern Prototype in Java is quite simple. Just remember a few points and you can easily implement the pattern.
Because using clone() method to copy an object is to read and write IO from the memory binary stream. Therefore, copying an object will not run the constructor of the corresponding class of the object.
Summary examples are as follows:
1. The constructor will not be run.
2. If there is a variable of reference type in the member variable of class (array is also an object). The default clone() does not copy it, but needs to provide its own deep copy;
Publisher: full stack programmer, stack length, please indicate the source for Reprint: https://javaforall.cn/116324.html Original link: https://javaforall.cn