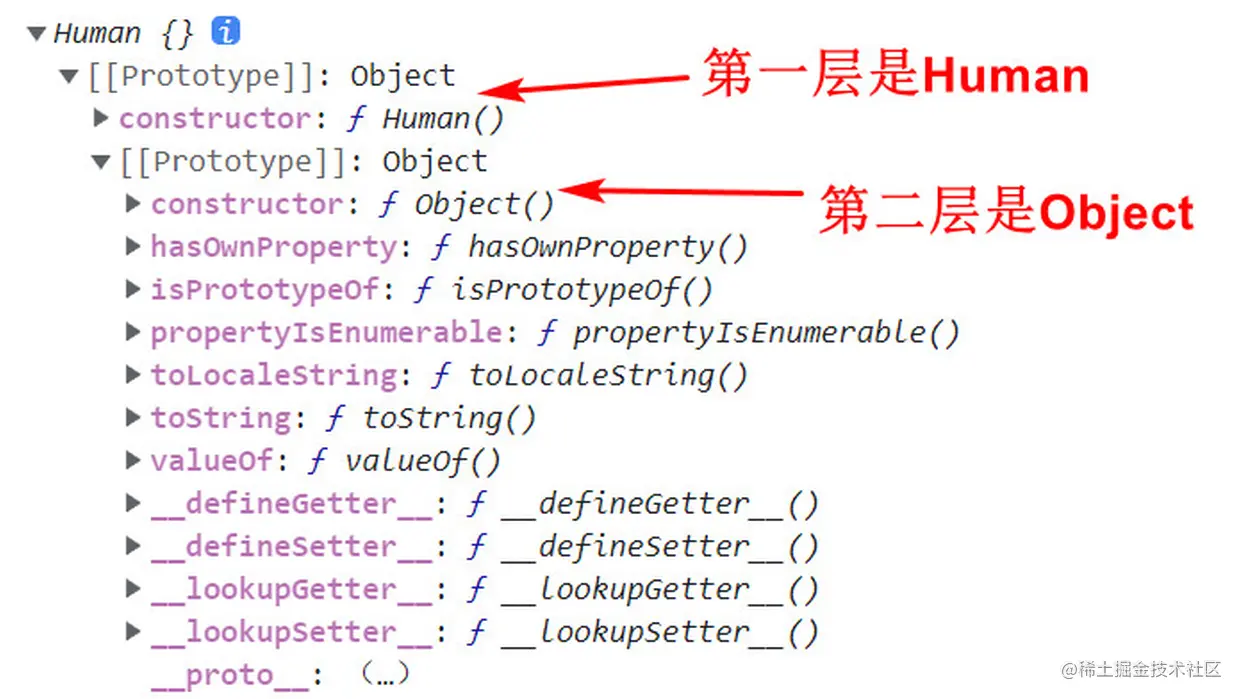
Detailed explanation of isPrototypeOf function in JavaScript
Sometimes when you look at some framework source code, you will encounter the isPrototypeOf() function. What is the function of this function?
isPrototypeOf()
isPrototypeOf() is the next method of the Object function (class), which is used to judge whether the current Object is the prototype of another Object. If yes, it returns true, otherwise it returns false.
The key to understanding this function is in the prototype chain, which is said to be one of the three mountains of JavaScript.
The principle will not be described in detail here. In short, there are three points:
1. Function objects are born with one prototype Prototype properties. 2. Each object is also born with an attribute__proto__Point to the function object that generated it prototype. 3. Function object prototype Also__proto__Point to the function object that generated it prototype. Copy code
Example 1: Object class instance:
let o = new Object(); console.log(Object.prototype.isPrototypeOf(o)); // true Copy code
Because the o Object is an instance of the Object, the prototype (_proto_) of the o Object points to the prototype of the Object, which will output true.
Example 2: define your own Human class:
function Human() {} let human = new Human(); console.log(Human.prototype.isPrototypeOf(human)); // true Copy code
This example is similar to the above example. Because the human object is an instance of human, the prototype of the human object (__) points to the prototype of human, and true will be output above.
In example 3, let's see if the prototype of Object is the prototype of human:
console.log(Object.prototype.isPrototypeOf(human)); // true Copy code
Why? It may be better explained by code. Please see the following derivation:
// Because the prototype (_proto_) in the Human prototype points to the prototype of the Object Human.prototype.__proto__ === Object.prototype // And because the _ prototype of human points to the prototype of human huamn.__proto__ === Human.prototype // Therefore, the prototype of the human Object (_proto_) points to the prototype of the Object huamn.__proto__.__proto__ === Object.prototype Copy code
If you look at the structure of human, it is easy to understand:
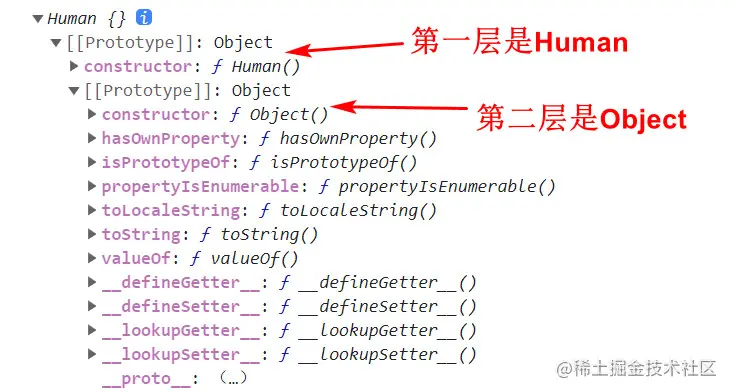
Is the prototype of an Object the prototype of a human Object? To be exact, the prototype of an Object is on the human prototype chain.
Example 4: is Object.prototype the prototype of a built-in class
The built-in classes Number, String, Boolean, Function and Array in JavaScript inherit Object, so the following output is true:
console.log(Object.prototype.isPrototypeOf(Number)); // true console.log(Object.prototype.isPrototypeOf(String)); // true console.log(Object.prototype.isPrototypeOf(Boolean)); // true console.log(Object.prototype.isPrototypeOf(Array)); // true console.log(Object.prototype.isPrototypeOf(Function)); // true Copy code
Naturally, Object.prototype is also the prototype of instances of Number, String, Boolean, Function and Array.
Example 5: Object is also a function (class):
In addition, it is worth mentioning that Function.prototype is also the prototype of Object, because Object is also a function (class) and they are generated from each other.
See the following output:
console.log(Object.prototype.isPrototypeOf(Function)); // true console.log(Function.prototype.isPrototypeOf(Object)); // true Copy code
And instanceof
instanceof is used to determine whether an object belongs to an instance of an object.
For example:
function Human() {} let human = new Human(); // Human is an instance of human, so the result outputs true console.log(human instanceof Human); // true // Because all classes inherit Object, the result also outputs true console.log(human instanceof Object); // true // Because the human object is not an array, the result is false console.log(human instanceof Array); // false Copy code
Here are some examples of built-in classes:
// [1,2,3] is an instance of Array, so it outputs true console.log([1, 2, 3] instanceof Array); // true // The method function() {} is an instance of Function, so it outputs true console.log(function(){} instanceof Function); Copy code
The principle of instanceof is to judge whether the prototype object of the class can be found in the prototype chain of the instance, and isprotypeof is to judge whether the prototype object of the class is on the prototype chain of the instance.
Therefore, I understand that the two expressions mean the same, that is, they are written differently. The following two outputs should be the same:
console.log(A instanceof B); console.log(B.prototype.isPrototypeOf(A)); Copy code
Summary
In fact, to understand the isPrototypeOf function, the key is to understand the prototype chain.