Reprint of this article is prohibited!
At the weekend, Mr. Huang will sort out more dry goods for you and try to sort out some articles you haven't read.
In fact, I have summarized it for you Python system keyword "super complete summary" and its "meaning" . Today, I will list an example of each keyword for your reference, learning and memory.
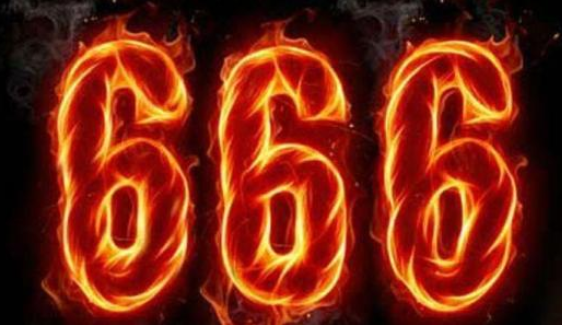
1 and,or,not
The and, or and not keywords are all logical operators. Their usage is as follows:
- and: if both statements return True, the return value will only be True, otherwise it will return False.
- or: if one of the statements returns True, the return value is True, otherwise it returns False.
- Not: if the statement is not True, the return value is True; otherwise, it returns False.
x1 = (5 > 3 and 5 < 10) x1 x2 = (5 > 3 or 5 > 10) x2 x3 = False not x3
The results are as follows:
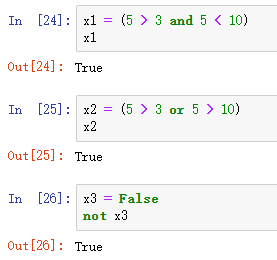
2 if,elif,else
if, elif and else are mainly used for conditional statements. Their usage is as follows:
- If: used to create conditional statements (if statements), and if code blocks are allowed to execute only when the condition is True.
- elif: used in conditional statements (if statements). It is the abbreviation of else if.
- else: used in conditional statements (if statements) and determines the code to execute when the if condition is False.
def func(x): if x < 18: print("under age") elif x < 30: print("youth") else: print("Middle aged and elderly") func(25)
The results are as follows:
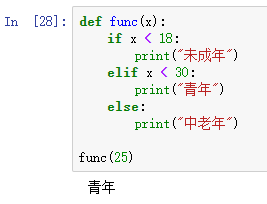
The else keyword is also used in the try... except block. See the following example.
def func1(x): try: 100//x except: print("ZeroDivisionError: division by zero(Divisor cannot be 0)") else: print(f"The program calculation result is{str(100//x)}") func1(10) func1(0)
The results are as follows:
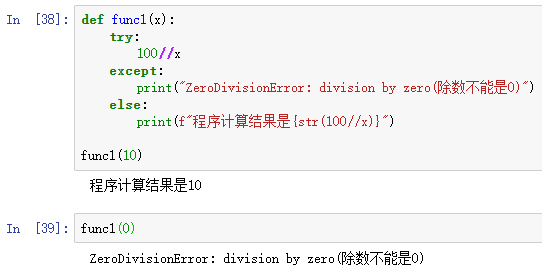
3 for,while
for and while are mainly used to define a loop. The usage is as follows:
- For: used to create a for loop, which can be used to traverse sequences, such as lists, tuples, etc.
- While: used to define a while loop. The while loop will continue until the while condition is False.
name_list = ["Zhang San","Li Si","Wang Wu"] for name in name_list: print(name)
The results are as follows:
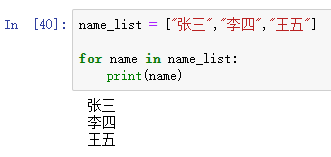
x = 0 while x<9: print(x) x += 1
The results are as follows:
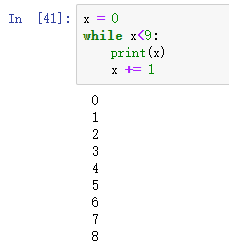
4 True,False
True and False are the results returned by the comparison operation. The usage is as follows:
- True: the keyword true is the same as 1.
- False: the keyword false is the same as 0.
print(9 > 6) print(6 in [11,6,33]) print(5 is 5) print(5 == 5) print(5 == 5 and 7 == 7) print(5 == 5 or 6 == 7) print(not(5 == 7))
The results are as follows:
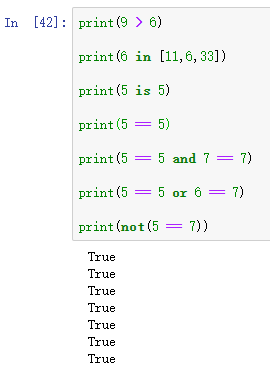
print(9 < 6) print(6 in [11,88,33]) print(15 is 5) print(5 != 5) print(5 < 5 and 7 == 7) print(5 <= 3 or 6 >= 7) print(not(5 == 5))
The results are as follows:
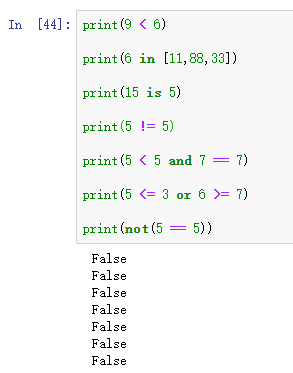
5 continue,break
continue and break are mainly used in for loops and while loops. The usage is as follows:
- Continue: the continue keyword is used to end the current iteration in a for loop (or while loop) and continue to the next iteration.
- Break: the break keyword is used to break a for loop or a while loop.
for i in range(10): if i <= 5: continue print(i)
The results are as follows:
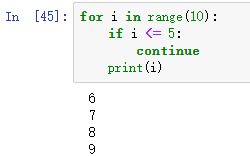
x = 0 while x < 10: if x == 5: break x += 1 print(x)
The results are as follows:
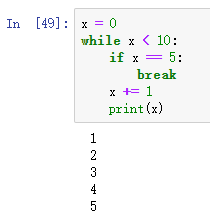
6 pass
The pass statement is used as a placeholder for future code. When the pass statement is executed, it will not have any impact, but the placeholder represents blank code. However, if you don't write anything, you will report an error. If empty code is not allowed in loop, function definition, class definition or if statement, pass can be used.
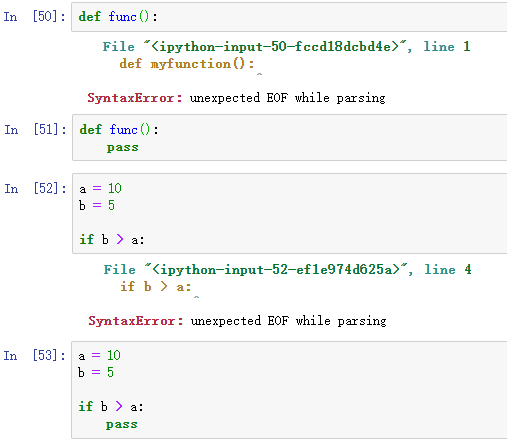
7 try,except,finally,raise
try, except, finally and raise are keywords related to exceptions. Their usage is as follows:
- Try: used in the try... except block, which defines a code block and executes the block without problem. If you include any errors, you can define different blocks for different error types.
- except: used in the try... except block. If the try block raises an error, execute the corresponding code block if there is a problem.
- Finally: used in the try... except block. It defines a code block that will run when the try... except... else block ends. The finally code block is executed whether or not the try block raises an error.
- Raise: raise keyword is used to throw an exception. It can define what kind of error is thrown and display error information to the user.
def func(x): try: 100 // x except: print("ZeroDivisionError: division by zero(Divisor cannot be 0)") else: print(f"The result is:{str(100 // x)}") finally: print("In any case, it will be implemented!") func(10) func(0)
The results are as follows:
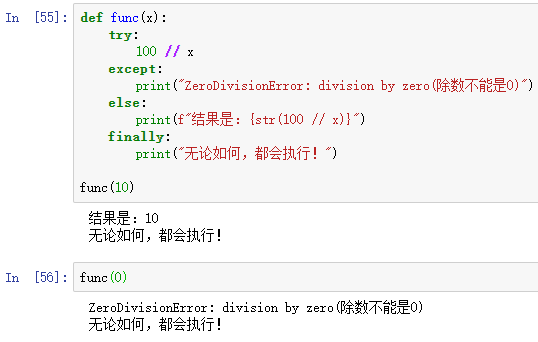
x = 15 if x < 18: raise Exception("I'm sorry, you're under 18, underage")
The results are as follows:
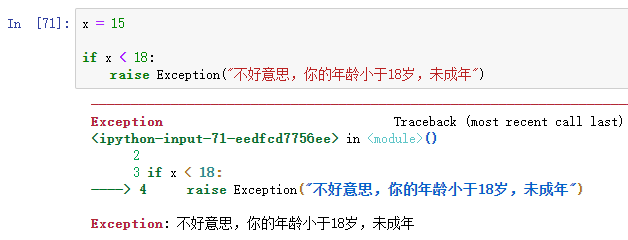
8 import,from,as
Import, from and as are related to module import. The usage is as follows:
- Import: used to import modules.
- From: used to import the specified part from the module, and import the specified subclass or function as needed to reduce unnecessary waste of resources.
- as: used to create aliases.
import openpyxl import pandas as pd from openpyxl import load_workbook()
9 def,return
def and return are keywords related to functions. Their usage is as follows:
- def: used to create (or define) a function.
- Return: used to end the defined function and return the value.
def func1(): print("Focus on official account: beauty of data analysis and statistics") func1()
The results are as follows:
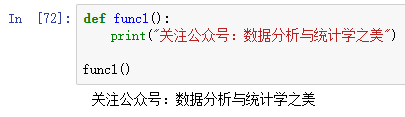
def func2(x,y): return x + y func2(x=2,y=8)
The results are as follows:
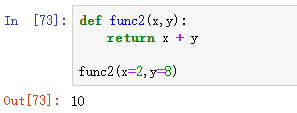
10 class
The class keyword is used to create (or define) a class.
class Person: name = "Zhang San" age = 18 p = Person() p.name,p.age
The results are as follows:
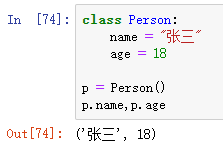
11 lambda
The lambda keyword is used to create an anonymous function.
x = lambda a: a + 8 x(2) y = lambda a,b: a + b y(1,1) z = lambda a,b,c: a * c + b z(2,5,5)
The results are as follows:
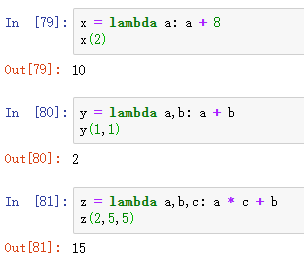
12 del
In Python, everything is an object. del keyword is mainly used to delete objects. It can also be used to delete variables, lists or parts of lists.
x = 1 del x print(x)
The results are as follows:
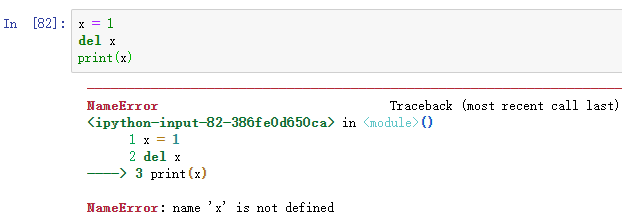
x = ["Zhang San","Li Si","Wang Wu"] del x[0] print(x)
The results are as follows:
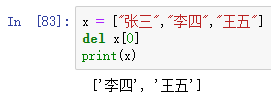
13 global,nonlocal
The global keyword is used to create a global variable. Nonlocal keyword is used to declare a nonlocal variable, which is used to identify the variable of external scope.
# Define a function: def func(): global x x = "Variables in functions" # Execute function: func() # X is defined in the function. Normally, printing x here will report an error. Let's have a look print(x)
The results are as follows:

14 in,is
The two keywords in and is must be distinguished. Their usage is as follows:
- In: on the one hand, it can be used to check whether there is a value in the sequence (list, range, string, etc.). It can also be used to traverse sequences in a for loop.
- is: used to judge whether two variables are the same object. If two objects are the same object, it returns True; otherwise, it returns False. To distinguish from = =, use the = = operator to determine whether two variables are equal.
x = ["Zhang San","Li Si","Wang Wu"] "Zhang San" in x # ------------------------- for i in range(3): print(i)
The results are as follows:
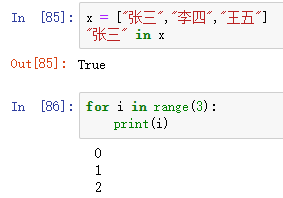
x = 2.0 y = 2.0 x is y x == y
The results are as follows:
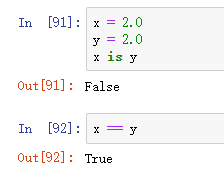
15 None
None keyword is used to define a null value (no value at all), which is different from 0, False or empty string. None is its own data type (NoneType), which can only be none.
x = None print(x) if x: print("Hee hee") else: print("ha-ha")
The results are as follows:
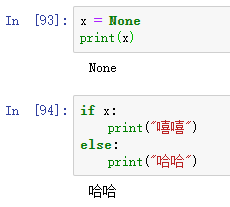
16 assert
When debugging code, use the assert keyword. It is mainly used to test whether the condition in the code is True. If False, AssertionError will be raised.
x = 666 assert x == 666 assert x == 888,"x It should be equal to 666. Your input is wrong!"
The results are as follows:
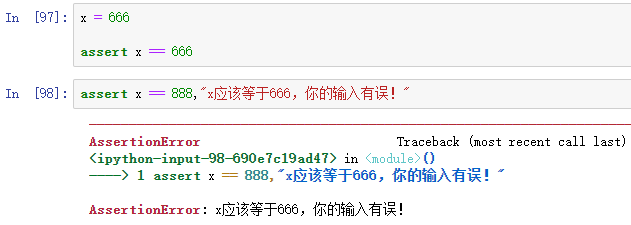
17 with
With is often used with open to read or write files.
with open("ha-ha.txt","r") as f: print(f.read())
The results are as follows:
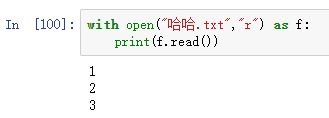
18 yield
yield keyword ends a function and returns a generator that returns values from the function in turn.
def f(): yield 5 f() next(f())
The results are as follows:
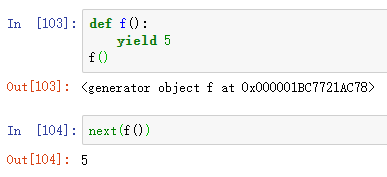