DOM attributes of Jquery series
Posted by thefamouseric on Tue, 11 Jun 2019 23:06:49 +0200
This chapter will analyze and explain Jquery attributes in conjunction with JQuery's official API. It mainly talks about. addClass (),. attr (),. hasClass (),. HTML (),. prop (),. removeAtrr (),. removeClass (),. removeProp (),. toggleClass (),. val ().
2.1 .addClass()
Adds a style to the specified element.
(1)addClass(string className)
Add one or more style names for the style you have created
A. Add a style name to the specified element

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("#div_id").addClass("div_class");
12 })
13 })
14 </script>
15
16 </head>
17 <body>
18 <div id="div_id">by id="div_id"Of div Add a single class name:div_class</div>
19 <input id="btnJquery" type="button" value="Jquery" />
20 </body>
21 </html>
View Code
Test results:
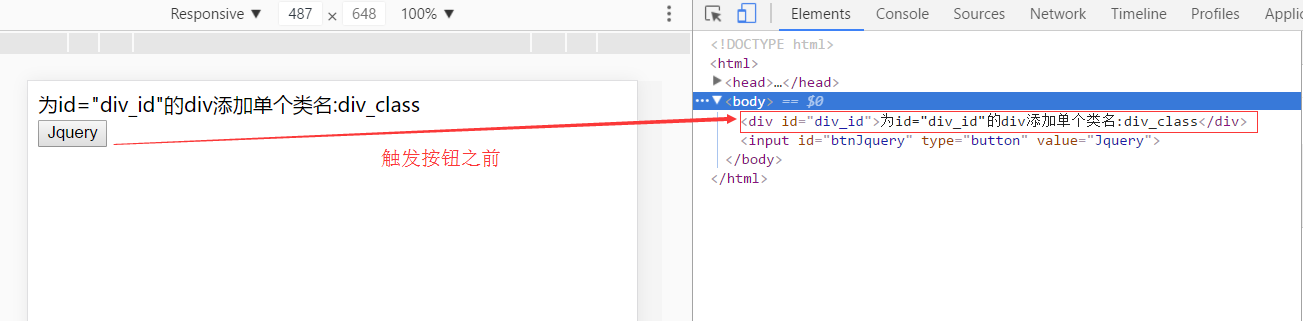
b. Adding multiple style names to multiple elements
Note: Multiple class names are separated by spaces.

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("#div_id").addClass("div_class div_class1 div_class2 div_class3");
12 })
13 })
14 </script>
15
16 </head>
17 <body>
18 <div id="div_id">by id="div_id"Of div Add a single class name:div_class</div>
19 <input id="btnJquery" type="button" value="Jquery" />
20 </body>
21 </html>
View Code
Test results:

(2)addClass(function(index){})
Note: index is the index position of matching elements

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("ul li").addClass(function (index) {
12 return "li-" + index;
13 })
14 })
15 })
16 </script>
17
18 </head>
19 <body>
20 <ul>
21 <li></li>
22 <li></li>
23 <li></li>
24 <li></li>
25 </ul>
26 <input id="btnJquery" type="button" value="Jquery"/>
27 </body>
28 </html>
View Code
Test results:

2.2 .attr()
Gets the attribute value of the first element in the set of matching elements or sets one or more attributes of each matching element.
(1)attr(string attributeName)
Gets the attribute value of the first element in the set of matched elements

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 var attrText = $("#div_idFirst").attr("id")
12 $("#div_idSecond").text(attrText);
13 })
14 })
15 </script>
16
17 </head>
18 <body>
19 <div id="div_idFirst">First div</div>
20 <div id="div_idSecond"></div>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:
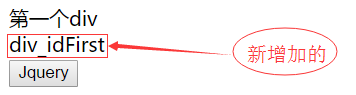
(2)attr(string attributeName,string value)
Setting a single property name and property value

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 //var attrText = $("#div_idFirst").attr("id")
12 $("#div_idSecond").attr("class","div_classSecond");
13 })
14 })
15 </script>
16
17 </head>
18 <body>
19 <div id="div_idFirst">First div</div>
20 <div id="div_idSecond"></div>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:

(3)attr(PlainObject attributes)
Set multiple attributes and attribute values in attribute-value format

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 //var attrText = $("#div_idFirst").attr("id")
12 $("#div_idSecond").attr({
13 "class": "div_classSecond",
14 "title":"divSecond"
15 });
16 })
17 })
18 </script>
19
20 </head>
21 <body>
22 <div id="div_idFirst">First div</div>
23 <div id="div_idSecond"></div>
24 <input id="btnJquery" type="button" value="Jquery" />
25 </body>
26 </html>
View Code

(4)attr(string attributeName,function(index,attr))
Setting attributes and attribute values in the form of functions

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("img").attr("src",function () {
12 return "Images/" + this.title;
13 });
14
15 })
16 })
17 </script>
18
19 </head>
20 <body>
21 <img src="~/BlogImgs/Jquery.png" title="Jquery"/>
22 <input id="btnJquery" type="button" value="Jquery" />
23 </body>
24 </html>
View Code
Test results:

2.3 .hasClass()
To determine whether any matching element is assigned a given class style, the element may have a style name. In HTML, spaces are used to separate multiple styles:
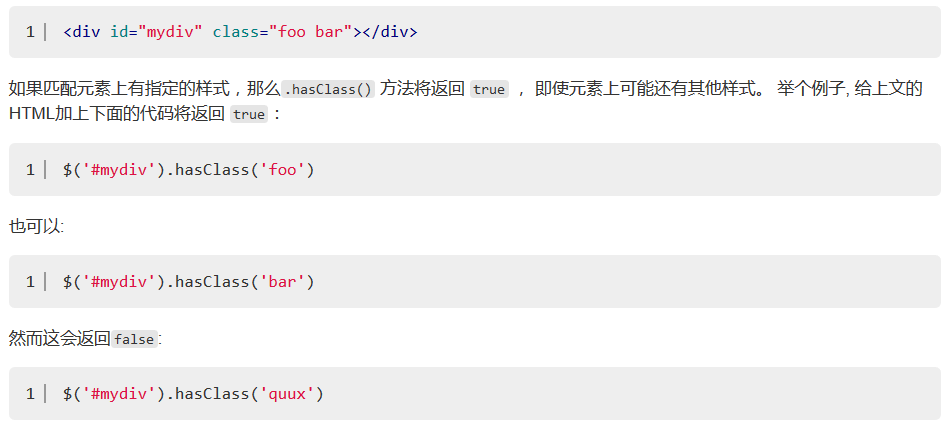
(1)hasClass(string className)

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 var div1=$("#div_first").hasClass("div1");
12 var div2 = $("#div_second").hasClass("div1");
13 })
14 })
15 </script>
16
17 </head>
18 <body>
19 <div id="div_first" class="div1">The first div is:</div>
20 <div id="div_second" class="div2">The second div is:</div>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:

2.4 .prop()
prop represents attributes, attr(). represents attributes, which is relatively simple and omitted.
2.5 .removeAtrr()
Skip over
2.6 .removeClass()
Skip over
2.7 . removeProp()
Skip over
2.8 .toggleClass()
Adding or deleting one or more style classes on each element of the matched set of elements depends on whether the style class exists or the value switching property. That is, if there is (does not exist) a class is deleted (added).
(1)toggleClass(string className)
One or more (spaced) style class names used to toggle on each element of the matched set of elements. The style class is removed if it exists on each element of the matched set of elements; if an element does not have the style class, the style class is added.

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("div").toggleClass("idDiv2");
12 })
13 })
14 </script>
15
16 </head>
17 <body>
18 <div class="idDiv1"></div>
19 <div class="idDiv1 idDiv2"></div>
20 <div class="idDiv1 idDiv2 idDiv3"></div>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:

The following will switch back and forth between the original style and the settings style

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 $("div").toggleClass("idDiv2 div4");
12 })
13 })
14 </script>
15
16 </head>
17 <body>
18 <div class="idDiv1"></div>
19 <div class="idDiv1 idDiv2"></div>
20 <div class="idDiv1 idDiv2 idDiv3"></div>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:

(2)toggleClass(string className,Boolean switch)
className is used to switch one or more style class names (separated by spaces) on each element of the matched set of elements; switch, a Boolean value, is used to determine whether the style should be added or removed.

(3)toggleClass(index,calss ,switch)
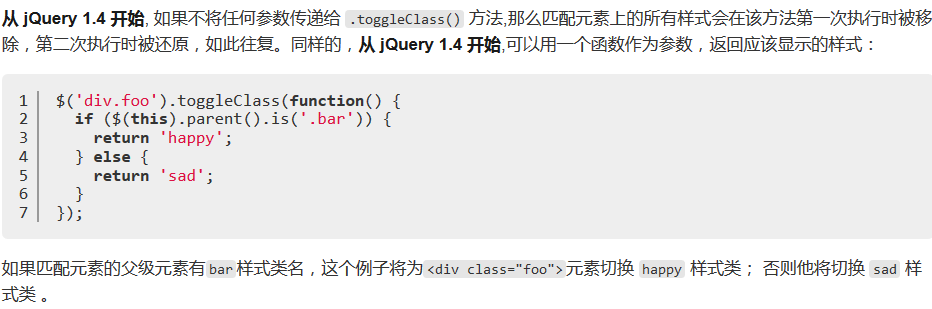
2.9 .val()
Get the current value of the first element in the set of matched elements or set the value of each element in the set of matched elements
(1)val()
To get the current value of the first element in the set of matching elements, the. val() method is mainly used to get the values of form elements, such as input, select and textarea. For the < select multiple= "multiple"> element, the. val() method returns an array containing each selection, and if no selection is selected, it returns null.

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 var valInput= $("#idInput").val();
12 var valSelect= $("#idSelect").val();
13 })
14 })
15 </script>
16
17 </head>
18 <body>
19 <input type="text" id="idInput"/>
20 <select id="idSelect">
21 <option>opt1</option>
22 <option>opt2</option>
23 <option>opt3</option>
24 </select>
25 <input id="btnJquery" type="button" value="Jquery" />
26 </body>
27 </html>
View Code
Test results:
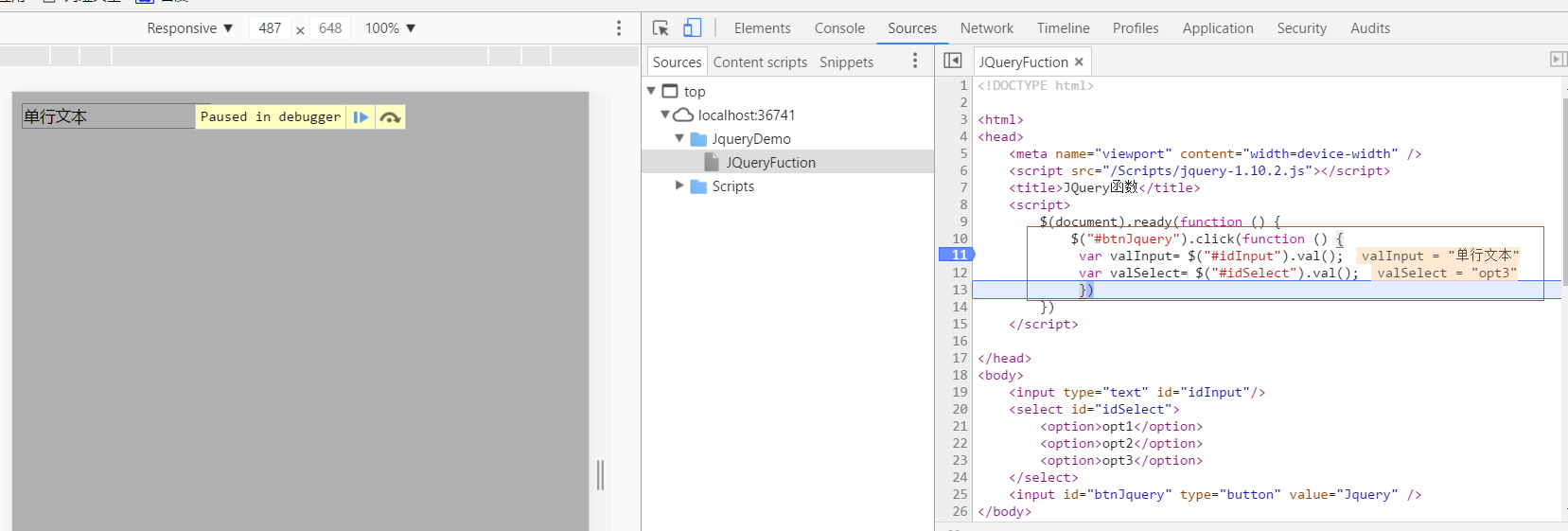
(2)val(string value) or val(Array value)
Set values for specified elements

1 <!DOCTYPE html>
2 <html>
3 <head>
4 <style>
5 button { margin:4px; cursor:pointer; }
6 input { margin:4px; color:blue; }
7 </style>
8 <script src="http://cdn.bootcss.com/jquery/1.11.2/jquery.min.js"></script>
9 </head>
10 <body>
11 <div>
12 <button>Feed</button>
13 <button>the</button>
14 <button>Input</button>
15 </div>
16 <input type="text" value="click a button" />
17 <script>
18 $("button").click(function () {
19 var text = $(this).text();
20 $("input").val(text);
21 });
22 </script>
23
24 </body>
25 </html>
View Code
Test results:

(3)val(function(index,value))
This method is usually used to set the value of the form field, index represents the index of the current element, value represents the value of the current element.

1 <!DOCTYPE html>
2
3 <html>
4 <head>
5 <meta name="viewport" content="width=device-width" />
6 <script src="~/Scripts/jquery-1.10.2.js"></script>
7 <title>JQuery function</title>
8 <script>
9 $(document).ready(function () {
10 $("#btnJquery").click(function () {
11 var valInput = $("#idInput").val(function (index,value) {
12 return value.toUpperCase().toString();
13 })
14 })
15 })
16 </script>
17
18 </head>
19 <body>
20 <input type="text" id="idInput"/>
21 <input id="btnJquery" type="button" value="Jquery" />
22 </body>
23 </html>
View Code
Test results:
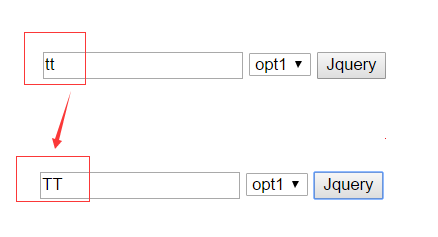
[01]http://www.jquery123.com/category/attributes/
[02]http://api.jqueryui.com
- Thank you for your reading. If there are any shortcomings, you are welcome to give advice, learn together and make progress together.
- The blogger's website is http://www.cnblogs.com/wangjiming/.
- Few articles are integrated by reading, reference, citation, plagiarism, copy and paste, most of which are original.
- If you like it, please recommend it; if you have any new ideas, you are welcome to submit them. Email: 2016177728@qq.com.
- The blog can be reprinted, but it must be a well-known source of the blog.
Topics:
Javascript
JQuery
Attribute