Example code cloud address
https://gitee.com/zhang-xin-1/springcloud1
1, Create Eureka project module
Create eureka project
Create a spring Lnitializr project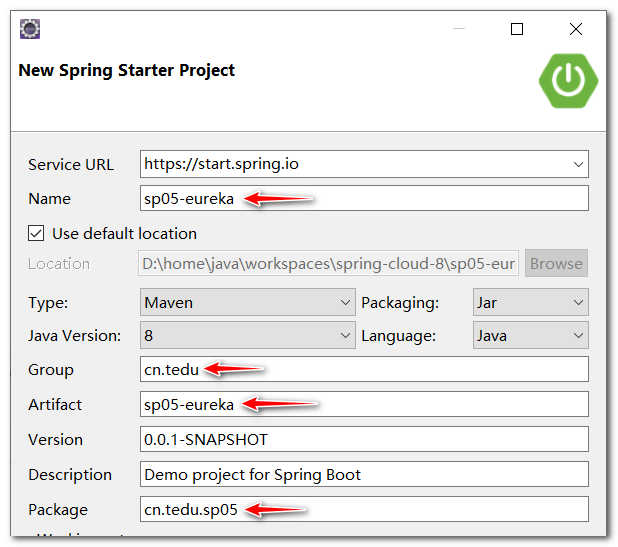
Direct search import Eureka dependency
Configuration dependency pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>cn.tedu</groupId> <artifactId>sp05-eureka</artifactId> <version>0.0.1-SNAPSHOT</version> <name>sp05-eureka</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> <spring-cloud.version>Hoxton.SR12</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Configure application.yml
spring: application: name: eureka-server # 8001 8101 8201 # 2001 3001 4001 5001 6001 server: port: 2001 eureka: server: # Disable self-protection mode enable-self-preservation: false instance: # host name hostname: eureka1 client: # For a single server, do not register with yourself or pull from yourself register-with-eureka: false fetch-registry: false
Detailed explanation of eureka configuration file
eureka cluster servers are distinguished by hostname
eureka.server.enable-self-preservation
eureka's self-protection status: does the rate of heartbeat failure exceed 85% within 15 minutes? If it exceeds, Eureka Server will protect the current instance registration information and prompt a warning. Once in the protection mode, Eureka Server will try to protect the information in its service registry and will not delete the data in the service registry. That is, no micro services will be logged off
eureka.client.register-with-eureka=false
Do not register with yourself
eureka.client.fetch-registry=false
Do not pull registration information from itself
eureka.instance.lease-expiration-duration-in-seconds
After the last heartbeat, how long is the interval between determining that the microservice is unavailable? 90 by default
Add notes to the main program
add to @ EnableEurekaServer
package cn.tedu.sp05; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @EnableEurekaServer @SpringBootApplication public class Sp05EurekaApplication { public static void main(String[] args) { SpringApplication.run(Sp05EurekaApplication.class, args); } }
Modify the hosts file and add eureka domain name mapping
hosts file address:
C:\Windows\System32\drivers\etc\hosts
127.0.0.1 eureka1
127.0.0.1 eureka2
Start and access the test
2, service provider (service registration eureka client)
Modify the item service, user service and order service to register the microservice with the eureka server
pom.xml add eureka dependency (add)
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency>
application.yml add eureka registration configuration (add)
eureka: client: service-url: defaultZone: http://eureka1:2001/eureka
eureka.instance.lease-renewal-interval-in-seconds
Heartbeat interval, 30 seconds by default
defaultZone, the default location, can be modified to a specific geographic location, such as beiJing, shangHai, shenZhen, etc. it indicates the deployment location of eureka server, which needs to be provided by ECs
eureka.client.registry-fetch-interval-seconds
The interval between pulling registration information is 30 seconds by default
The main program enables the service registration discovery client (@ EnableDiscoveryClient)
Modify item service, user service and order service,
Main program addition @ EnableDiscoveryClient annotation
package cn.tedu.sp05; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @EnableEurekaServer @SpringBootApplication public class Sp05EurekaApplication { public static void main(String[] args) { SpringApplication.run(Sp05EurekaApplication.class, args); } }
Start and visit eureka to view the registration information
3, High availability of Eureka and service providers
Item service high availability
startup parameter -- server.port You can override the port configuration in yml
Configure startup parameters
--server.port=8001
Start another port service
Copy springboot project
--server.port=8002
Start test
Visit eureka to view the item service registration information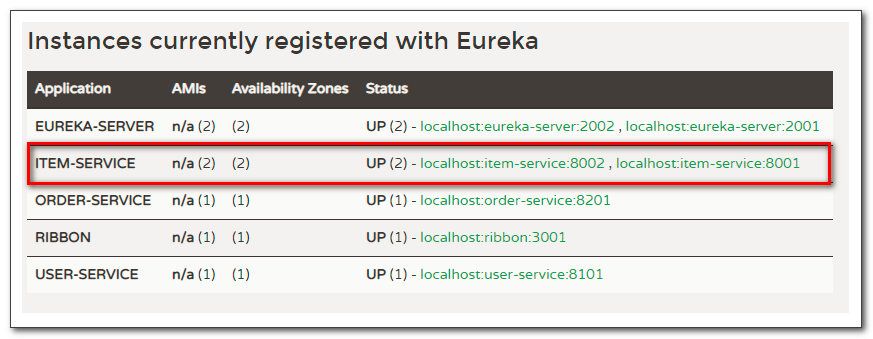
Access two port tests
http://localhost:8001/35
http://localhost:8002/35
eureka high availability (profile Profile knowledge points)
Add profile profiles for both servers
application-eureka1.yml(profile configuration file naming format application-xxxxx.yml)
# application-eureka1.yml eureka: instance: # host name hostname: eureka1 client: # Cluster servers register and pull each other register-with-eureka: true fetch-registry: true service-url: defaultZone: http://eureka2:2002/eureka
application-eureka2.yml
# application-eureka2.yml eureka: instance: # host name hostname: eureka2 client: # Cluster servers register and pull each other register-with-eureka: true fetch-registry: true service-url: defaultZone: http://eureka1:2001/eureka
Configure startup parameters -- spring.profiles.active and -- server.port
eureka1 startup parameters:
--spring.profiles.active=eureka1 --server.port=2001
eureka2 startup parameters:
If running on the command line, you can add parameters to the command line:
java -jar xxx.jar --spring.profiles.active=eureka1 --server.port=2001
Visit the eureka server to view the registration information
When the eureka client registers, it registers with two servers
Modify the following microservices
sp02-itemservice
sp03-userservice
sp04-orderservice
eureka: client: service-url: defaultZone: http://eureka1:2001/eureka, http://eureka2:2002/eureka
When one eureka service goes down, another eureka service can still be connected