input statement
(1) Programming program, input the lengths a and b of the two right angles of the right triangle, and calculate the length of the hypotenuse c.
import math a=float(input('Enter right triangle side a:')) b=float(input('Enter right triangle side b:')) c=math.sqrt(a*a+b*b) print('The length of the third side is:',c)
(2) Programming program, used to realize the exchange of two numbers.
a=float(input('Enter number a Value of:')) b=float(input('Enter number b Value of:')) a,b=b,a print(a,b)
(3) The programming program calculates the average value and sum according to the input scores of the three subjects
a=float(input('Please enter your language score:')) b=float(input('Please enter your math grade:')) c=float(input('Please enter your English score:')) list1=[a,b,c] b=sum(list1) avg=b/3 print(avg,b)
(4) The programming program outputs the area of the triangle according to the length of the three sides of the input triangle
(tip: use Helen formula, import math, and call math.sqrt() function)
from math import * a=float(input('Please enter one side of triangle a:')) b=float(input('Please enter one side of triangle b:')) c=float(input('Please enter one side of triangle c:')) d=(a+b+c)/2 e=sqrt(d*(d-a)*(d-b)*(d-c)) print("The triangle area is:",e)
Comprehensive operation
1. The user inputs a three digit natural number, calculates and outputs its hundreds, tens and tens of digits.
a=int(input('Please enter a three digit integer:')) b=a//100 c=a%100//10 d=a%10 print('Hundred is:',b) print('Ten for:',c) print('Bits are:',d)
2. Write a program to input any large natural number and output the sum of each number.
a=int(input('Please enter a natural number:')) summ=0 while a>0: b=a%10 summ+=b a=a//10 print('The sum of the figures is:',summ)
3. Write a program, input two sets setA and setB, and output their intersection, union and difference setA-setB respectively.
a=input('Input set A:') b=input('Input set B:') seta=a.split(' ')#The parameters of the split("") function can be any delimiter, including (a,b,c..; 1,2,3...;%,!, *, space) setb=b.split(' ')#Split ('), the input is separated by spaces, and split (', ') is separated by commas seta=[int(seta[i])for i in range(len(seta))]#A for loop that converts each character to an int value setb=[int(setb[i])for i in range(len(setb))]#Convert input values to a list setA=set(seta) setB=set(setb)#Convert list to collection m=setA&setB n=setA|setB s=setA-setB print('Intersection is:',m) print('Union as:',n) print('Difference set is:',s)
4. Write a program, input a natural number and output its binary, octal and hexadecimal representations. Tip: the corresponding formula in the value type in ppt
a=eval(input('Enter a natural number:')) b=bin(a) c=oct(a) d=hex(a) print('The binary of natural number is:',b) print('The octal of a natural number is:',c) print('The hexadecimal of a natural number is:',d)
5. As shown in the figure below, complete the programming of the following two topics, input the principal, annual interest rate and year, and calculate the compound interest (keep two decimal places)
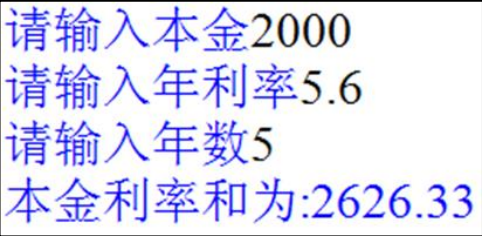
Tip: the user can use the statement form of "print(str.format(" sum of principal and interest rate: {0:2.2f}", amount))" to output the program running effect (the result is decimal).
a=eval(input('Please enter the principal:')) b=eval(input('Please enter annual interest rate:')) c=eval(input('Please enter the number of years:')) d=a*(1+b/100)**c print(str.format('The principal and interest rates are:{0:2.2f}',d))
6 .
Programming
,
Enter the radius of the ball
,
Calculate the surface area and volume of the ball
(
Results to two decimal places)
Tips
:
(1)
The formula for calculating the surface area of the ball is
4*
π
*r
2
,
The formula for calculating the volume of the ball is
4/3*
π
*r
3
(2)
Users can use
" print(str.format("
The surface area of the ball is
:{0:2.2f},
Volume: {1:2.2f} ", area, volume)"
Output the running effect of the program in the form of statements
from math import * r=eval(input('Please enter the radius of the ball:')) S=4*pi*r**2 C=(4*pi*r**3)/3 print(str.format("The surface area of the ball is:{0:2.2f},Volume is:{1:2.2f}",S,C))
7.
Programming
,
Enter principal according to
b
. annual interest rate
r
And years
n
Calculate final receipt
v,v=b(1+r)";
Then write the test code
,
Prompt for principal, annual interest rate and number of years
,
Display most
Final income
(
Keep two decimal places
)
.
b=eval(input('Please enter the principal:')) r=eval(input('Please enter annual interest rate:')) n=eval(input('Please enter the number of years:')) v=b*(1+r) print(str.format('The final income is:{0:2.2f}',v))
8.
Programming
,
Solving quadratic equation of one variable
x
2
-10x+16=0,
See the figure below for the operation effect.

from math import * print('It is known that the form of binary quadratic equation is a*x**2+bx+c=0') a=eval(input('Please enter a value:')) b=eval(input('Please enter b value:')) c=eval(input('Please enter c value:')) m=b**2-4*a*c if m==0 : x=(-b)/2*a print('Solution of equation:',x) elif m>0 : x1=(-b+sqrt(b**2-4*a*c))/2*a x2=(-b-sqrt(b**2-4*a*c))/2*a print('Solution of equation:',x1,x2) elif m<0 : print('The equation has no solution')
9.
Programming
,
Prompt for name and year of birth
,
Output name and age
,
Operation effect see
Tips
:
(1)
Users can use
datetime.date. today().year
Returns the year value of the current year.
(2)
Users can use
" print("
Hello!
!{0}
You
{1}
Years old.
". format (sname, age)" is used to output the program running effect
from datetime import * now=date.today().year SName=input('Please enter your name:') age=input('Please enter age:') print('Current year is:',now) print('Hello!{0}. you{1}Years old.'.format(SName,age))
10.
Enter the two right angle sides of a right triangle, calculate the perimeter and area of the triangle, and two
An acute angle. The results are kept to one decimal place. The effect is shown in the figure below.
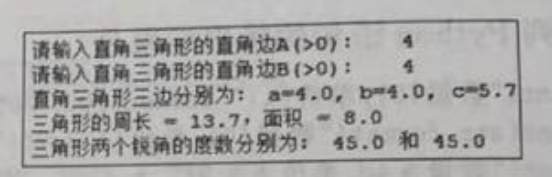
Tips:
(1)math.asin()
Function returns the radian whose sine is the specified number
;math.acos()
Function returns radians whose cosine value is a specified number.
(2)
The formula for converting radians to angles is angle
=
radian
*180/
π
(3)
have access to
" round(asin(sinA)*180/pi,0)"
Find acute angle in the form of statement
A
Degree of
(4)
Can use
"print(str. format("
Perimeter of triangle
={0:1.1f},
Area = {1:1.1f}",p,area)" and output the perimeter and area of the triangle according to the requirements of the topic.
from math import * a=float(input('Please enter right triangle right edge a:')) b=float(input('Please enter right triangle right edge b:')) c=sqrt(a*a+b*b) f=a+b+c d=f/2 area=sqrt(d*(d-a)*(d-b)*(d-c)) sinA=a/b A=round(asin(sinA)*180/pi,0) C=90-A print(str.format("Area of triangle={0:1.1f}, the measure of area={1:1.1f}",f,area)) print('Please output acute angle A Degree of:',A) print('Please output acute angle A Degree of:',C)