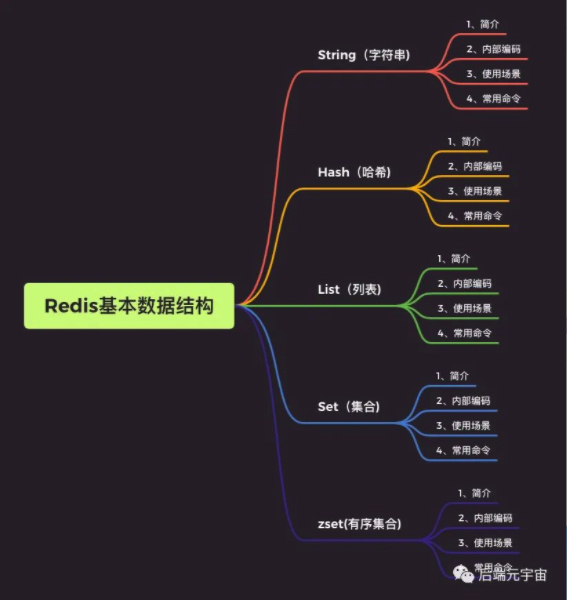
preface
If you are asked what data structures redis has, you can certainly name five basic data structures: string, hash, list, set and Zset
You may also know that it has three special data structure types: Geospatial, hyperlog and Bitmap.
But if you ask what data structures you use in your actual project. Do you think most of them just use the String data structure? Even if an object is cached, it is only through jsonobject Tojsonstring (object) turns it into String storage. The json String is converted into an object when fetching.
Since redis provides five basic data structures, there must be specific applications.
Next, the application scenarios in actual development will be demonstrated for five basic data types.
1, String (string)
1. Introduction
String type is the most basic and commonly used data type in Redis, and is even used by many players as the only data type in Redis. The string type is binary safe in Redis, which means that the string value cares about the binary string and does not care about the specific format. You can use it to store strings in json format or JPEG image format.
2. Internal coding
If the number is stored, it is encoded with int (8-byte long integer); If a non numeric string less than or equal to 39 bytes is stored, it is embstr encoded; If it is greater than 39 bytes, it is raw encoding.
The internal coding of redis data takes time to sort out an article and write it separately
3. Usage scenario
(1) Store some configuration data
In the separate development, although some data is stored in the database, there are few changes. For example, there is a national area table. After the current end initiates a request, if the background reads from the relational database every time, it will affect the overall performance of the website.
On the first visit, we can store all region information in the redis string, request again, directly read the json string of the region from the database and return it to the front end.
(2) Cache object
Turn the object into json storage, such as commodity information and user information.
(3) Data statistics
redis integer can be used to record website visits, downloads of a file, number of sign INS, video visits, etc. (self increasing and self decreasing)
(4) Limit the number of requests in time
For example, the logged in user requests the SMS verification code, and the verification code is valid within 5 minutes.
When the user requests the SMS interface for the first time, store the user id in the string that redis has sent the SMS, and set the expiration time to 5 minutes. When the user requests the SMS interface again and finds that the user's SMS sending record already exists, no SMS will be sent.
(5) Order number (globally unique)
Sometimes you need to generate a globally unique value through redis. Key command: incrby (atomic self increment).
SET order_no 2001 --Assume that the order number starts in 2001,here vlaue Must be int type INCRBY order_no 1 --Since the increase of 1, this time returns to 2002
(6) Distributed session
When we use nginx for load balancing, if each slave server stores its own session, the session information will be lost due to non sharing after switching the server. We have to consider a third application to store the session.
We use relational databases or non relational databases such as Redis. The storage and reading performance of relational databases cannot be compared with non relational databases such as Redis.
4. Common commands
--increase set mykey "test" --Set a new value for the key and overwrite the original value setex mykey 10 "hello" -- Setting assignment Key The expiration time for is 10 seconds,Available at survival time value mset key3 "stephen" key4 "liu" --Batch setting key --Delete del mykey --Delete existing keys --change incr mykey --Value increases by 1,If key non-existent,establish key,The initial value is set to 0,The result after increase is 1 decrby mykey 5 --Value reduced by 5 --check exists mykey --Judge whether the key exists, return 1 if it exists, otherwise return 0 get mykey --obtain Key Corresponding value mget key3 key4 --Batch get key
2, Hash (hash)
1. Introduction
The data structure of hash can be simply understood as map < String, map < String, String > in java. This structure is particularly suitable for storing objects. The above String type can also store objects, but each time a property in the object is modified, the whole json String must be taken out to modify the property and then re inserted, The interface feature of hash allows us to modify only one attribute of the object.
Hash data type has the advantages of being more flexible and faster than String type when storing the above types of data. Specifically, when using String type storage, it is necessary to convert and parse json format strings. Even if conversion is not required, hash still has an advantage in memory overhead.
2. Internal coding
If the number of hash type elements is less than 512 and all values are less than 64 bytes, use zip list encoding; otherwise, use hashtable encoding.
4. Usage scenario
(1) Redisson distributed lock
When Redisson implements distributed locks, the internal data used is hash instead of String. Because Redisson implements the reentrant locking mechanism. Therefore, the current thread ID is stored in the hash.
(2) Shopping cart list
The user id is the key, the commodity id is the field, and the commodity quantity is the value, which exactly constitutes the three elements of the shopping cart, as shown in the figure below.
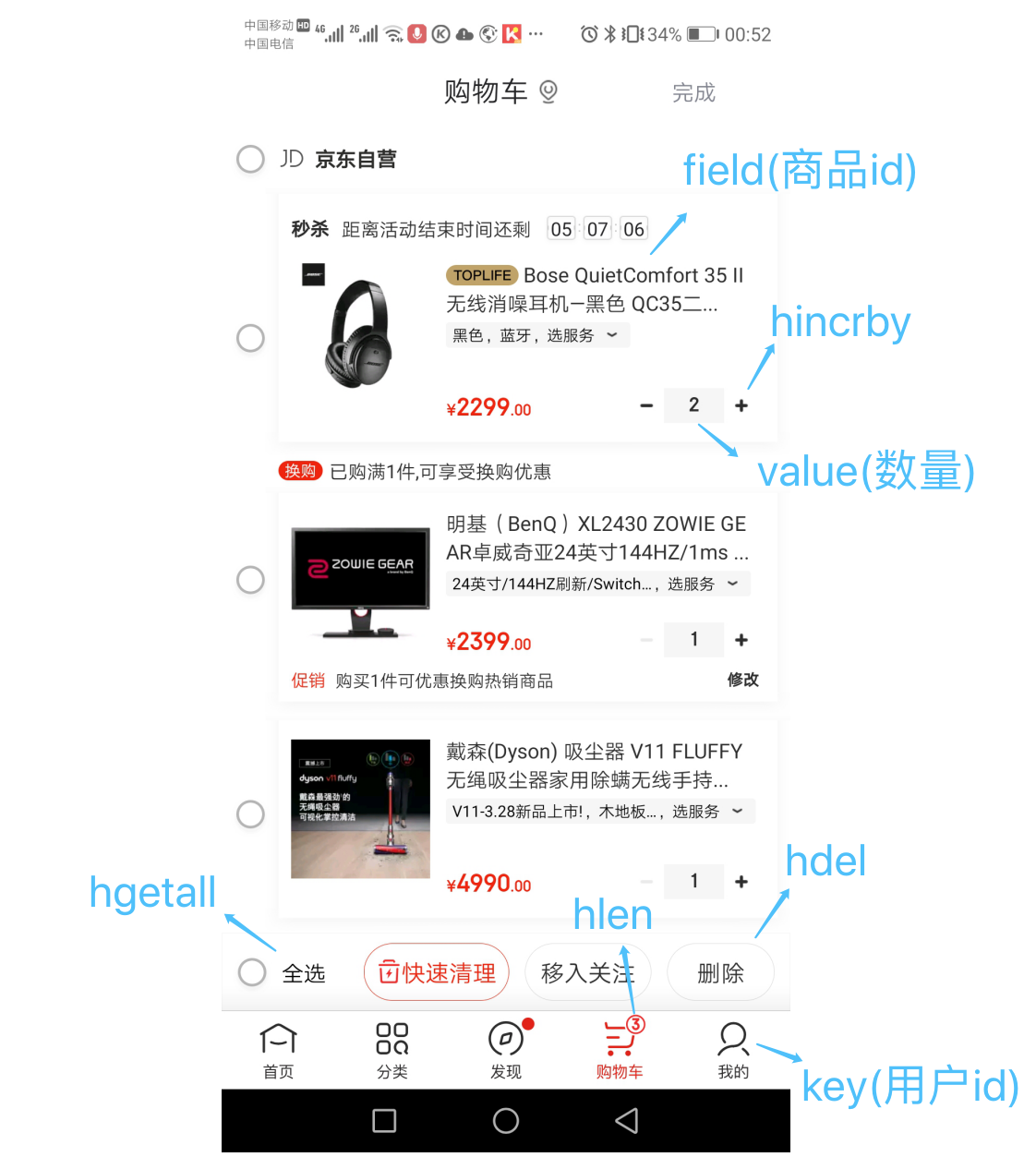
The commands involved here are as follows
hset cart:{user id} {commodity id} 1 # Add item hincrby cart:{user id} {commodity id} 1 # Increase quantity hlen cart:{user id} # Get the total number of items hdel cart:{user id} {commodity id} # Delete item hgetall cart:{user id} #Get all items in shopping cart
Note: at present, only the commodity ID is stored in Redis. When the specific information of the commodity is echoed, you also need to query the database with the commodity ID.
(3) Cache object
The structure of hash type (key, field, value) is similar to that of object (object id, attribute, value). It can also be used to store objects.
When introducing the application scenarios of String type, String + json is also a way to store objects. When storing objects, do you use String + json or hash?
The comparison of the two storage methods is shown in the table below.
string + json | hash | |
---|---|---|
efficiency | Very high | high |
capacity | low | low |
flexibility | low | high |
serialize | simple | complex |
Generally, objects are stored in string + json. Some frequently changing attributes in objects can be extracted and stored in hash.
3. Common commands
--increase hset key field1 "s" --If field field1 non-existent,Create the key and its associated Hash, Hash in,key by field1 ,Parallel setting value by s ,If field field1 existence,Then cover hmset key field1 "hello" field2 "world" -- Set multiple fields at once --Delete hdel key field1 --delete key The field name in the key is field1 Field of del key -- Delete key --change hincrby key field 1 --to field Add 1 to the value of --check hget key field1 --Get key value is key,Field is field1 Value of hlen key --obtain key Number of fields for the key hmget key field1 field2 field3 --Get multiple fields at once hgetall key --return key All keys field Value and value value hkeys key --obtain key Of all fields in the key field value hvals key --obtain key Of all fields in the key value value
3, List
1. Introduction
List type is a string linked list sorted by insertion order. A list can store up to 2 ^ 32-1 elements. We can simply understand that it is equivalent to LinkesdList in java.
Like the ordinary linked list in the data structure, we can add new elements to its left and right. During insertion, if the key does not exist, Redis will create a new linked list for the key. On the contrary, if all the elements in the linked list are removed, the key will also be deleted from the database.
2. Internal coding
If the number of elements in the list is less than 512 and the value of each element in the list is less than 64 bytes (the default), zip list encoding is used; otherwise, linkedlist encoding is used.
After Redis 3.2, the hybrid structure of ziplist + linked list is used, which is called QuickList (quick linked list).
2. Usage scenario
Some people will consider using the list data structure to make some likes list, comment list and ranking list of the circle of friends. It's not impossible, but I personally think it would be more appropriate to use set or zset for these functions. Specific examples will be given below.
(1) Message queue
lpop and rpush (or vice versa) can implement the function of queues.
However, if so, you find that redis as a message queue is unsafe. It cannot be consumed repeatedly. Once consumed, it will be deleted. At the same time, it is troublesome to do consumer confirmation ACK. Therefore, generally, redis message queue is rarely used in actual development, because there are mature message queues such as Kafka, NSQ and RabbitMQ, and their functions are more perfect.
4. Common commands
--increase lpush mykey a b --if key non-existent,Create the key and its associated List,Insert in sequence a ,b, if List Type key existence,Then insert value in rpush mykey a b --Insert at the end of the linked list b,Insert in a(lpush list a b So when you read it b,a The order of, and rpush How do you put it? How do you read it --Delete del mykey --Delete existing keys --change lset mykey 1 e --Start from scratch, Set the element value with index 1,Set to new value e,If the index is out of bounds,An error message is returned --check lrange mykey 0 -1 --Take all the elements in the linked list, where 0 represents the first element,-1 Represents the last element. lrange mykey 0 2 --Start from scratch,Fetch index to 0,1,2 Element of lpop mykey --Get header element,And pop up the header element,Out of stack
4, Set
1. Introduction
The set in Redis is somewhat similar to the HashSet in Java. Its internal key value pairs are unordered and unique. Its internal implementation is equivalent to a special dictionary. All values in the dictionary are NULL. When the last element in the collection is removed, the data structure is automatically deleted and memory is reclaimed.
2. Code
If all elements in the set are integers and the number of elements is less than 512, use intset encoding; otherwise, use hashtable encoding.
Application scenario
Since the characteristics of set are unordered and unique. So let's consider using it in some unique scenarios.
(1) Lucky draw
Store the winning user ID in an activity. Because of the de duplication function, it can ensure that the same user will not win the prize twice.
sadd user 1 2 3 4 5 --Put all employees(Name or number)Put it in the lucky draw box srandmember user 1 -- Draw a first prize(Employees can participate in the lucky draw repeatedly) spop user 1 -- Draw a first prize(Employees cannot participate in the lucky draw repeatedly) srandmember user 3 --Draw three second prizes smembers user --View all employees participating in the current lucky draw box scard user --View the number of participants in the current lucky draw box
(2) Like
Ensure that a user can only click one like. key can be the article id of XX article and wechat circle of friends
sadd key userId --give the thumbs-up(/Collection) srem key userId --Cancel like(/(Collection) smembers key -- Get all likes(/Collection)user card key -- Number of users getting likes sismember key userId --Judge whether to like(/Collection)
(3) Friends and contacts
The key can be a user id
sadd userId1 1 2 3 4 5 sadd userId2 4 5 6 7 8 --Some user My friends id Put into collection sinter userId1 userId2 --Get common friends sdiff userId1 userId2 --to user2 recommend user1 My friends sismember userId1 5 sismember userId2 5 --Verify that a user is simultaneously user1 and user2 follow
4. Common commands
--increase sadd myset a b c --if key non-existent,Create the key and its associated set,Insert in sequence a ,b,c. if key existence,Then insert value in,if a stay myset Already exists in,Is inserted b and c Two new members. --Delete spop myset --Caudal b Removed,in fact b Is not the first or last member previously inserted srem myset a d f --if f non-existent, Remove a,d ,And return 2 --change smove myset myset2 a --take a from myset Move to myset2, --check sismember myset a --judge a Whether it already exists. A return value of 1 indicates it exists. smembers myset --see set Content in scard myset --obtain Set Number of elements in the collection srandmember myset --Return a member randomly
5, Zset (ordered set)
1. Introduction
Each member in sorted sets will have a score associated with it. Redis uses the score to sort the members in the set from small to large. Members are unique, but scores can be repeated.
2. Data coding
When the number of elements in the ordered set is less than 128 and the value of each element is less than 64 bytes, zip list encoding is used; otherwise, skip list encoding is used
3. Application scenario
Since it is an orderly and non repeatable list, you can do some ranking related scenes.
- Ranking list (product sales, video score, user game score)
- News hot search.
4. Common commands
--increase zadd key 2 "two" 3 "three" --Add two members whose scores are 2 and 3 respectively --Delete zrem key one two --Delete multiple member variables,Returns the number of deleted items --change zincrby key 2 one --Will member one The score of the member is increased by 2, and the updated score of the member is returned (corresponding to its score after the score is changed) index Will also change) --check zrange key 0 -1 WITHSCORES --Returns all members and scores,No WITHSCORES,Return only members zrange key start stop --Returns the value from the smallest to the largest according to the score of the element start reach stop All elements between zscore key three --Get member three Score of zrangebyscore key 1 2 --The score obtained satisfies expression 1 < score <= 2 Members of zcard key --obtain myzset Number of members in key zcount key 1 2 --The score obtained satisfies expression 1 <= score <= 2 Number of members zrank key member --Get the ranking of elements, from small to large zrevrank key member --Get the ranking of elements, from large to small
This article will be written here first. For the internal coding of redis data, I'll take the time to write a separate article.
Pay attention to the official account: the backend meta universe. Continue to output high-quality good articles