Token authentication
- In restful design, user authentication mode usually uses json web token instead of traditional HTTP Basic authentication (incoming account password)
The token authentication mode is as follows: add token to the request header
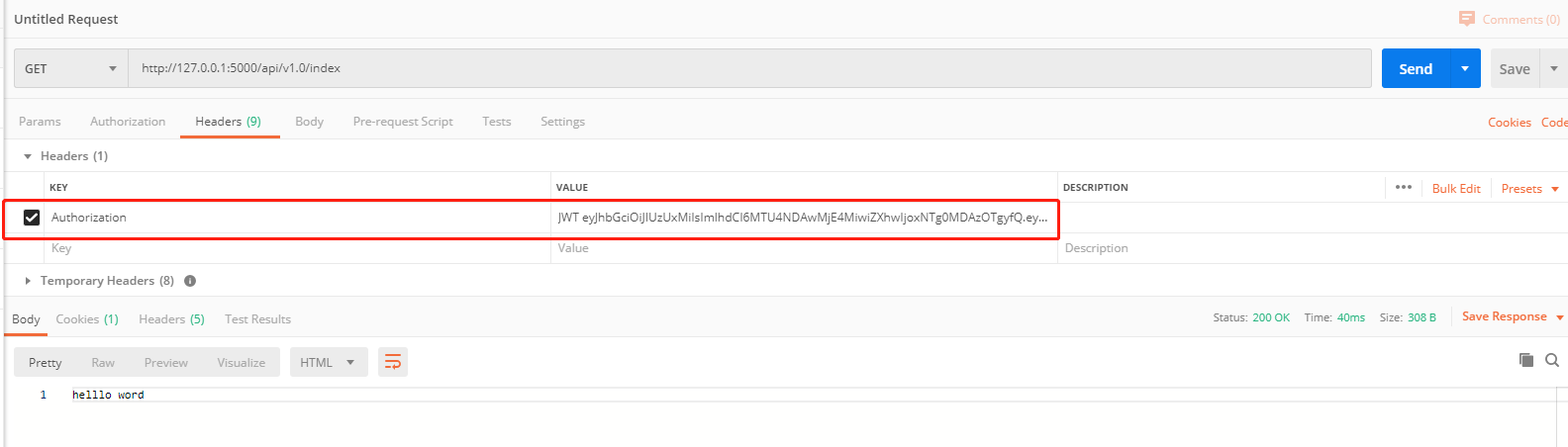
Implementation in Flask
Flask extension flask httpauth provides the authentication method
$ pip install flask-httpauth
Instantiate first
auth = HTTPTokenAuth(scheme='JWT')
Method of checking token (token_auth.py )
from flask_httpauth import HTTPTokenAuth
from itsdangerous import TimedJSONWebSignatureSerializer as Serializer, BadSignature, SignatureExpired
from config import Config
from home.libs.error_code import AuthFailed
auth = HTTPTokenAuth(scheme='JWT')
@auth.verify_token
def verify_token(token):
# Config.SECRET_KEY: internal private key, which is written in the configuration information here
s = Serializer(Config.SECRET_KEY)
try:
data = s.loads(token)
except BadSignature:
# AuthFailed custom exception type
raise AuthFailed(msg='token incorrect')
except SignatureExpired:
raise AuthFailed(msg='token be overdue')
# Verify pass returns True
return True
The method of generating token is put in modeles.user Generated in class
from config import Config
from home import db
from werkzeug.security import generate_password_hash, check_password_hash
from itsdangerous import TimedJSONWebSignatureSerializer as Serializer
class User(db.Model):
"""user"""
__tablename__ = 'user'
id = db.Column(db.Integer, primary_key=True, autoincrement=True)
username = db.Column(db.String(80), unique=True, nullable=False) # user name
hash_password = db.Column(db.String(120), nullable=False) # password
phone = db.Column(db.String(20), nullable=False) # cell-phone number
# Clear text password (read only)
@property
def password(self):
raise AttributeError('unreadable ')
# Write the password, calculate the hash value at the same time, and save it in the model
@password.setter
def password(self,value):
self.hash_password = generate_password_hash(value)
# Check if the password is correct
def check_password(self, password):
return check_password_hash(self.hash_password,password)
# Generate token
@staticmethod
def create_token(user_id):
"""
//Generate token
:param user_id: user id
:return:
"""
# The first parameter is the internal private key, which is written in the configuration information. If it is just a test, it can be written dead
# The second parameter is the validity period (seconds)
s = Serializer(Config.SECRET_KEY, expires_in=Config.TOKEN_EXPIRATION)
# Receive user id conversion and coding
token = s.dumps({"id": user_id}).decode('ascii')
return token
Using decorators
from flask import request, jsonify
from home.modles import User
@api.route('/login', methods=['POST'])
def login():
'''
//Accept the parameter and verify the parameter, return token
:return:
'''
# user = request.json['username']
# password = request.json['password']
# guest = User.query.filter_by(username=user).first()
# # Generate token
# z_token = User.create_token(guest.id)
#
# return jsonify(token=z_token)
pass
# Call the decorator to authenticate the token (import token_auth.py Auth: from in home.utils.token_ auth import auth)
@api.route('/index')
@auth.login_required
def index():
return 'helllo word'
- ps: step: do not use named file“ token.py ”, exception such as unable to import xxx class will be reported