DOM:
Concept: Document Object Model document object model. Function: to operate marked documents (xml and html documents learned so far)
Dom classification
-
Core DOM (basic syntax of ECMA specification)
The api of core Dom operates XML and HTML
-
Dom of xml
Function: to manipulate xml documents
-
Dom of html
Function: to manipulate html documents
-
Summary:
Most APIs of core Dom, xml Dom and HTML Dom are generic
Dom's api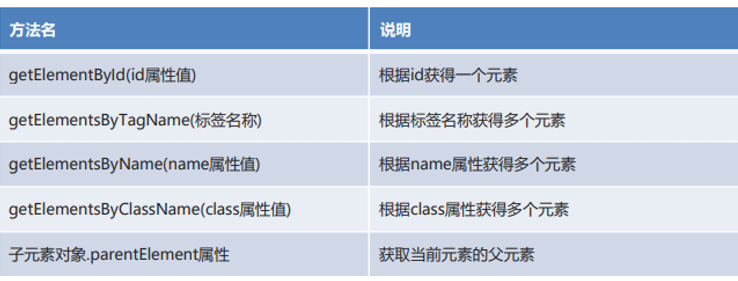
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> password:<input type="password" name="psw" /><br/> user name:<input type="text" name="username" id="uname" class="s" /><br/> Gender:<input type="radio" name="sex" class="s" value="man"/>male <input type="radio" name="sex" class="s" value="woman"/>female<br/> <script type="text/javascript"> var username_tag=document.getElementById("uname"); document.write(username_tag.name+"<hr/>"); var input_tag=document.getElementsByTagName("input"); document.write(input_tag.length+"<hr/>"); var sex_objects=document.getElementsByName("sex"); document.write(sex_objects); var classname=document.getElementsByClassName("s"); document.write(classname); </script> </body> </html>
Dom operation text
Get text: innerhtml
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <div id="d1"> I'm a bird </div> <script type="text/javascript"> var div_tag=document.getElementById("d1"); document.write(div_tag.innerHTML); div_tag.innerHTML="I'm a little tiger" document.write(div_tag.innerHTML); </script> </body> </html>
event
Binding of events to event sources
Method 1: direct binding (define the event attribute directly in the tag and bind the corresponding js code)
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <input type="button" value="Normal button 1" onclick="top1()"/> <script type="text/javascript"> function top1(){ console.log("11111"); } </script> </body> </html>
Method 2: indirect binding (event attributes are not defined in the tag, but bound when used)
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <button type="button">Normal button 2</button> <script type="text/javascript"> var tags=document.getElementsByTagName("button"); var singalTag=tags[0]; singalTag.onclick=function(){ console.log(2222); } </script> </body> </html>
Common events:
onchange: this event is triggered when the content changes. It is often used together with select
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Directly bind the content switch in the drop-down selection box</title> </head> <body> <select onchange="changeContest()"> <option value="">plase choose</option> <option value="">Henan Province</option> <option value="">Hebei Province</option> </select> <script type="text/javascript"> num=0; function changeContest(){ console.log(num); num++; } </script> </body> </html>
onload: after the html page is fully loaded, the js code in the event is executed, so the code can be placed in the head
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> <script type="text/javascript"> function t2(){ var divTag=document.getElementById("p"); var div_text=divTag.innerHTML; console.log(div_text); } </script> </head> <body onload="t2()"> <div id="p"> Mutual progress </div> </body> </html>
BOM:
Concept:
BOM: browser object module, browser object model (a series of objects, forming a model), which operates the browser
Five objects contained in BOM
Window: represents the window object, which is also the top-level object of bom (the other four bom objects can be obtained through the window object)
location: represents the address bar object and operates the address of the address bar (obtain the address of the address bar or change the address of the address bar)
History: refers to the history before and after the user accessed, and the history accessed by the operation user
Screen: indicates the size of the web page under the screen band of the web page (resolution, such as 1366 * 768)
navigator: represents the browser object and obtains the relevant parameter information of the browser (for example, which browser is used and what is the version number)
Common API s:
open() and close() of window
<input type="button" value="Open a new window" onclick="openW();"/> <input type="button" value="Close a new window" onclick="closeW();"/> <script> //1 open a new window function openW(){ //wid: represents a new window object window.open("http://www.baidu.com"); } //2. Close a new window function closeW(){ //Open who, close who window.close(); } </script>
Location: location href
<body> <input type="button" value="Gets the address of the window" onclick="getPath();" /> <input type="button" value="Change the address of the window" onclick="changePath();" /> <script type="text/javascript"> //1. Get address function getPath(){ //var loc = window.location; //var path = loc.href; var path = location.href; document.write(path); } //2. Change address function changePath(){ //location.href="https://www.baidu.com"; //location.href="index.html"; location.href="http://127.0.0.1:8020/javascript_high1/index.html"; } </script> </body>
Exercise: provincial and municipal linkage:
Demand: click the provincial data to display the urban data
An error occurred: value = "" should be removed when option value = ""
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> province:<select id="p"> <option>Please select</option> <option value="1">Shanxi Province</option> <option value="2">Shaanxi Province</option> </select> city:<select id="c"> <option>Please select</option> </select> <script type="text/javascript"> document.getElementById("p").onchange=function(){ var province=this.value; var city=document.getElementById("c"); if(province==1){ var data="<option>Please select</option>"+ "<option>Taiyuan</option>"+ "<option>da tong</option>"; city.innerHTML=data; }else if(province==2){ var data="<option>Please select</option>"+ "<option>Xi'an</option>"+ "<option>Anxi</option>"; city.innerHTML=data; } } </script> </body> </html>
Common built-in objects
Global object
Global object: javascript built-in object (the name of this object is global: only name, no "real person")
Features: when calling a method, the global object is omitted and not written
Common methods:
eval() : evaluates the JavaScript string and executes it as script code.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var a="document.write(1111)"; eval(a); </script> </body> </html>
isNaN() : check whether a value is a number. Not a number returns false
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var a=10; document.write(isNaN(a)); </script> </body> </html>
parseFloat() : parses a string and returns a floating point number
parseInt() : parses a string and returns an integer.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var num="da101"; document.write(typeof(num)); document.write(num); var num1=parseInt(num); document.write(typeof(num1)); document.write(num1); </script> </body> </html>
Math object
common method
floor(); "Floor method" rounded down, for example: 5.9 – > rounded down 5
ceil(); "Ceiling method" rounded up, for example: 5.01 – > rounded up 6
round(); Rounding method
random(); Random number, range [0,1); including 0, excluding 1, decimals can be given randomly
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var n1=Math.random()*6; document.write(n1); </script> </body> </html>
JSON object
Concept: JSON(JavaScript Object Notation): it is a lightweight data exchange format.
Features: 1 Small amount of data 2 More intuitive
Function: exchange data between browser and server
Object definition and traversal:
The first: a single json object
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var user={"id":1,"name":"jack"}; document.write(user.id+":"+user.name) </script> </body> </html>
Second: json array object
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var user=[{"id":1,"name":"jack"},{"id":2,"name":"rose"},{"id":3,"name":"ship"},] for (var i = 0; i < user.length; i++) { var p=user[i]; document.write(p.id+":"+p.name); } </script> </body> </html>
Complex json objects
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title></title> </head> <body> <script type="text/javascript"> var user={"Horse cow force":{"age":1,"sex":"nan"}}; var casUser=user.Horse cow force; var casUserId=user.Horse cow force.age; document.write(casUserId); </script> </body> </html>