Git branch-a View all local and remote branches ()
View local branch git branch
View remote branch git branch -r
WePY Component Usage
components={ child:Child, anotherchild:Child };
props static pass value: parent passes constant data to son, only String string type can be passed
<child title="mytitle"></child>
<Component: Dynamic data='data Name in Parent data'> Data independent between father and child <Component: Dynamic data.sync='Data name in parent data'> <Component: twoWayTitle='Data name in parent data'> Cyclic rendering of WePY components requires <repeat> <repeat for="{{list}}" key="index" index="index" item="item"> <child :item="item"></child>
WeChat Applet Learning and the Use of wepy Framework
For example: index.js, index.wxml, index.wxss, index.json.
Files with the.js suffix are script files, files with the.json suffix are configuration files, and files with the.wxss suffix are style sheet files, which are equivalent to css files in html.
<image src="{{src}}"></image>
MVVM data rendering {{data}} like vue's design pattern
Determine wx:if, wx:else
Loop wx:for.
Applet event binding bindtap, catchtap
Get the loop number data-x
<!--stay wxml in--> <view class="contents"> <button catchtap="choose" wx:if="{{showBtn}}">Choice</button> <text wx:for="{{arrList}}" wx:key="{{index}}" data-i="{{index}}" data-type="{{item}}" bindtap="selectTab" >{{item}}</text> </view>
//In the corresponding js data:{ showBtn:true, arrList:['apple','pear','orange'] }, choose(){ this.setData({ showBtn:false }) }, selectTab(ev){ var getAttrType=ev.target.dataset.type; var index=ev.target.dataset.i; } onLoad(){ }, onShow(){ }
Local Picture Selection wx.chooseImage(OBJECT) Picture preview wx.previewImage(OBJECT) File upload wx.uploadFile(OBJECT) Data request wx.request()
wx.setStorageSync wx.getStorageSync wx.clearStorageSync
Corresponding local picture selection js
//Corresponding local picture selection js wx.chooseImage({ count: 3, // Default 9, if Spreadsheet sizeType: ['original', 'compressed'], // You can specify whether you want the original or the compressed image, both by default sourceType: ['album', 'camera'], // You can specify whether the source is an album or a camera, both by default success: function (res) { // Returns a list of local file paths for the selected photo, tempFilePath can display the picture as an src attribute of the img tag var tempFilePaths = res.tempFilePaths wx.previewImage({ //Corresponding picture preview current: temFilePaths[0], // Links to currently displayed pictures urls: tempFilePaths||[] // List of http links for pictures that need Preview }) wx.uploadFile({ //Picture upload url: 'http://example.weixin.qq.com/upload', //example only, unreal interface address filePath: tempFilePaths[0], name:"file", formData:{ //Upload with other parameters "user":"test" } success: function(res){ var data = res.data //do something } }) } })
//Data Request js wx.request({ url: 'test.php', //Example only, not actual interface address data: { //Note that the url parameter for uploading pictures here is not received in the background because there is a method for uploading pictures on it params1: '' , params2: '' }, header:{ "Content-Type":"application/json" }, success: function(res) { console.log(res.data) } })
//Data storage js wx.setStorageSync("key","value") //Set the key value to store locally wx.getStorageSync("key") //Get locally stored key wx.clearStorageSync("key")
Introduction to installation and use of wepy framework
Install or update the WePY command line tool globally npm install wepy-cli -g Generate Demo development projects in the Development Directory wepy init standard myproject cd myproject npm install Wepy build--watch (startup project) Component name suffix.wpy Component page structure is the same as vue structure
page Page Instance export default class MyPage extends wepy.page { } Component Component Instance export default class MyPage extends wepy.component { }
Repat Loop Component
<!--wepy structure--> <style type="scss"> </style> <template> <button bindtap="showFirstComponent">Button</button> <view v-if="show"> <DemoCom></DemoCom> <!--Using Components--> </view> <repeat for="{{arr}}" key="index"> <!--Circular Component--> <DemoCom :passdata="item" /> <!--Pass Value--> <repeat> </template> <script> import wepy from 'wepy' import DemoComponent from '../components/demoComponent' //For example, here the demoComponent component is introduced by path export default class pageDemo extends wepy.page { config = { 'navigationBarTitleText': 'Page Title', 'navigationBarTextStyle': '#FFFFFF', //Head Background Color 'navigationBarBackgroundColor': '#0386FF'//Header Font Color }; components = { //Register imported components DemoCom:DemoComponent }; data = { show:true, arr:[ {name:'aa',age:'16'}, {name:'bb',age:'17'}, {name:'cc',age:'c18'} ] }; methods = { showFirstComponent(){ //Events inside bindtap this.show=!this.show; //Here the settings for data data, get the same settings as inside vueJS, get the same } }; onLoad(){ }; onShow(){ } </script>
<style> .mainBgcolor{ /*Global Style Global*/ background:#ffffff; } </style> <script> import wepy from 'wepy' import 'wepy-async-function' import { aldstat } from './utils/ald-stat' export default class extends wepy.app { config={ pages: [ //All registered here are pages.wpy components built in pages, jumping between pages 'pages/index', 'pages/login', 'pages/companyDetailInfo', ], window: { backgroundTextStyle: 'light', navigationBarBackgroundColor: '#fff', navigationBarTitleText: 'WeChat', navigationBarTextStyle: 'black' } } globalData = { //Global data global use userInfo: null } </script>
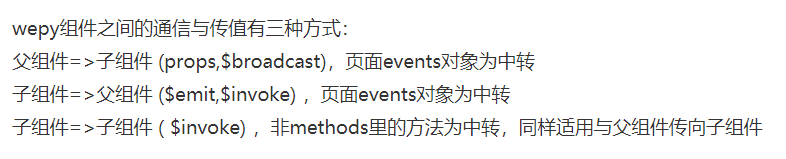
<child title="mytitle"></child> // child.wpy, static pass value props = { title: String }; onLoad () { console.log(this.title); // mytitle }
props dynamic value transfer refers to the parent component transferring dynamic data content to the child component, which is completely independent of each other.
// parent.wpy <child :title="parentTitle" :syncTitle.sync="parentTitle" :twoWayTitle="parentTitle"></child> data = { parentTitle: 'p-title' }; // child.wpy props = { // Static value transfer title: String, // One-way dynamic value transfer from parent to child syncTitle: { type: String, default: 'null' }, twoWayTitle: { type: String, default: 'nothing', twoWay: true } }; onLoad () { console.log(this.title); // p-title console.log(this.syncTitle); // p-title console.log(this.twoWayTitle); // p-title this.title = 'c-title'; console.log(this.$parent.parentTitle); // p-title. this.twoWayTitle = 'two-way-title'; this.$apply(); console.log(this.$parent.parentTitle); // When two-way-title. --- twoWay is true, when the property value in the props of the child component changes, it also changes the value corresponding to the parent component. this.$parent.parentTitle = 'p-title-changed'; this.$parent.$apply(); console.log(this.title); // 'c-title'; console.log(this.syncTitle); // 'p-title-changed'- The value of the props property with the.sync modifier that changes in the parent component simultaneously changes the value of the child component. }
mini-program
Exploration-WeChat applet
app.json: Global Configuration - (Applet Public Settings) app.js: Global Configuration - (Applet Logic) app.wxss: Global Configuration - (Applet Common Style) pages: Page array - (Applets can have their own configuration, style, logical file, and a page structure file)
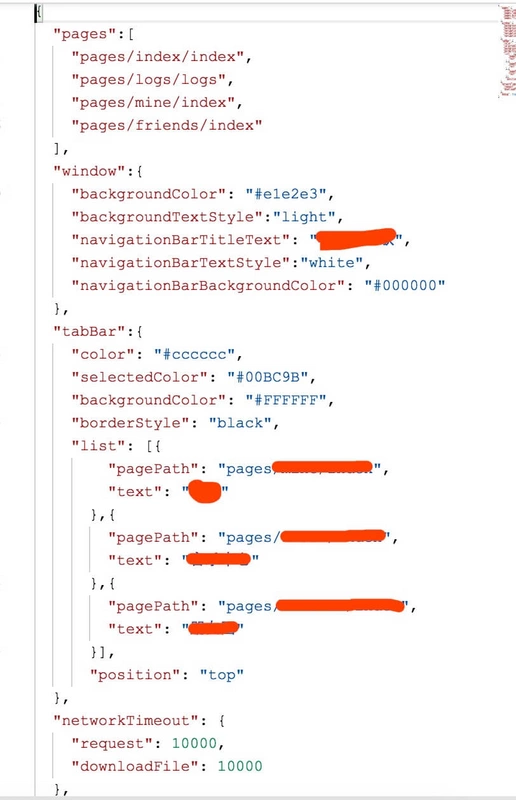
wx:key specifies the unique identifier of the item in the list.
import references the template defined by the current file
The bind event binding does not prevent the bubble event from bubbling up, while the catch event binding prevents the bubble event from bubbling up.
wepy Framework Notes for WeChat Applet
// index.wpy <template> <view> <panel> <h1 slot="title"></h1> </panel> <counter1 :num="myNum"></counter1> <counter2 :num.sync="syncNum"></counter2> <list :item="items"></list> </view> </template> <script> import wepy from 'wepy'; import List from '../components/list'; import Panel from '../components/panel'; import Counter from '../components/counter'; export default class Index extends wepy.page { config = { "navigationBarTitleText": "test" }; components = { panel: Panel, counter1: Counter, counter2: Counter, list: List }; data = { myNum: 50, syncNum: 100, items: [1, 2, 3, 4] } } </script>
project ├── pages | ├── index | | ├── index.json index Page Configuration | | ├── index.js index Page logic | | ├── index.wxml index Page structure | | └── index.wxss index Page Style Sheet | └── log | ├── log.json log Page Configuration | ├── log.wxml log Page logic | ├── log.js log Page structure | └── log.wxss log Page Style Sheet ├── app.js Applet logic ├── app.json Applet Public Settings └── app.wxss Applet Common Style Sheet
project └── src ├── pages | ├── index.wpy index Page configuration, structure, style, logic | └── log.wpy log Page configuration, structure, style, logic └──app.wpy Applet Configuration Items (Global Style Configuration, Claim Hook, etc.)
Directory specified by dist WeChat developer tool
├── node_modules - Directory for src code writing |- Compoonents components Folder (Incomplete Page) | |- com_a.wpy reusable component A | | - com_b.wpy reusable component B |- pages folder (full page) | |- index.wpy page index | | - page.wpy page |- app.wpy applet configuration item (global style configuration, declaration hook, etc.) - Package.json package configuration
props, data, computed, components, methods, watch (watcher in wepy) are supported, but methods in wepy can only be used for page event binding, other custom methods should be placed on the outer layer, and all methods in VUE should be placed under methods
props delivery in wepy requires a.sync modifier
this.$apply() method must be executed after props to update
wepy supports data two-way binding
Subcomponent modifies parent component data by adding twoway:true property value when defining props
EvetBus is recommended for component communication, whereas broadcast, emit, $invoke are three methods for component communication in wepy
wepy only supports the life cycle of applets: onLoad, onReady, and so on
lang determines its code compilation process, src determines whether to outline code, inline code is ignored when src attributes are present and valid
Common Component Reference
<template> <!-- with`<script>`Components declared in the script section ID Name the custom label for the name so that it can be used in the`<template>`Insert component in template section --> <child></child> </template> <script> import wepy from 'wepy'; //Introducing component files import Child from '../components/child'; export default class Index extends wepy.component { //Declare component, assign component id to child components = { child: Child }; } </script>
Cyclic rendering of components
<template> <!--Note that the for attribute is used instead of the wx:for attribute--> <repeat for="{{list}}" key="index" index="index" item="item"> <!--Insert the child component declared in the <script>script section and pass in item --> <child :item="item"></child> </repeat> </template>
<template> <repeat for="{{banners}}"> <child :item="item"></child> <repeat> </template>
computed calculation properties
watcher listener
data = { a: 1 } // Calculate the attribute aPlus, which can be referenced in scripts and interpolated in templates by {{aPlus}} computed = { aPlus () { return this.a + 1 } }
data = { num: 1 } // The listener function name must have the same name as the numeric attribute num in the data object that needs to be listened on. // The newValue in its parameter is the new value after the change of the numeric attribute, and the oldValue is the old value before the change. watch = { num (newValue, oldValue) { console.log(`num value: ${oldValue} -> ${newValue}`) } } // The corresponding listener function num() with the same name is automatically called and executed once each time the listened numeric property num is changed onLoad () { setInterval(() => { this.num++; this.$apply(); }, 1000) }
Emi emit is the opposite of broadcast in that all ancestor components of the event initiation component receive emit events in turn.
For example, you want to call a method of the component ComA in Page_Index: this.$invoke('ComA', 'someMethod', 'someArgs'); 1 If you want to call a method of the component ComG in the component ComA: this.$invoke('./../ComB/ComG', 'someMethod', 'someArgs');
.default: Bind applet bubble events, such as bindtap,.Default suffix can be omitted from writing; .stop: Bind applets to capture things, such as catchtap; .user: Bind user-defined component events, triggered by $emit.
// index.wpy <template> <child @childFn.user="parentFn"></child> </template> <script> import wepy from 'wepy' import Child from '../components/child' export default class Index extends wepy.page { components = { child: Child } methods = { parentFn (num, evt) { console.log('parent received emit event, number is: ' + num) } } } </script> // child.wpy <template> <view @tap="tap">Click me</view> </template> <script> import wepy from 'wepy' export default class Child extends wepy.component { methods = { tap () { console.log('child is clicked') this.$emit('childFn', 100) } } } </script>
// mixins/test.js import wepy from 'wepy'; export default class TestMixin extends wepy.mixin { data = { foo: 'foo defined by page', bar: 'bar defined by testMix' }; methods: { tap () { console.log('mix tap'); } } } // pages/index.wpy import wepy from 'wepy'; import TestMixin from './mixins/test'; export default class Index extends wepy.page { data = { foo: 'foo defined by index' }; mixins = [TestMixin ]; onShow() { console.log(this.foo); // foo defined by index. console.log(this.bar); // foo defined by testMix. } }
Please compliment!Because your encouragement is my greatest motivation to write!
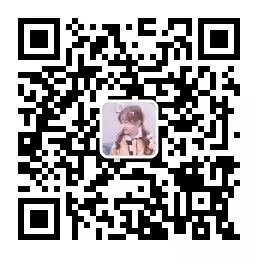
Blow the crowd: 711613774
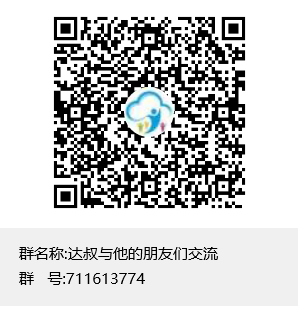