keyboard entry
Get user input dynamically
//Guide Package import java.util.Sccanner; public static void main(String[] args){ Scanner input = new Scanner(System.in); // Enter an integer data int num = input.nextInt(); //Enter a string data String name = input.next(); //Enter a double data double money = input.nextDouble(); }
import java.util.Scanner; public class IpuptDemo{ public static void main(String[] args){ Scanner input = new Scanner(System.in); System.out.println("Please enter an integer"); int number = input.nextInt(); System.out.println("What you entered is"+ number); } }
operator
Assignment Operators
assignment |
---|
= |
A very basic but very common operator. Its function is to assign the data on the right of the equal sign to the variables on the left
public static void main(String[] args){ int m = 10; int k = 20; }
Note: the assignment data must be consistent with the type (excluding automatic type promotion)
Arithmetic operator
addition | subtraction | multiplication | division | Modular operation |
---|---|---|---|---|
+ | - | * | / | % |
public static void main(String[] args){ int m = 90; int k = 5; System.out.println( m+k ); System.out.println( m-k ); System.out.println( m*k ); System.out.println( m/k ); System.out.println( m%k ); }
Note: it is used for arithmetic and mathematical operations, following the mixed algorithm, and the function of% is to find the remainder. Multiplier use*
Compound Assignment
Add etc | Subtraction | Multiply, etc | Divide and so on | Module, etc |
---|---|---|---|---|
+= | -= | *= | /= | %= |
public static void main(String[] args){ int m = 10; int k = 20; int n = 105; System.out.println( m+=10 ); System.out.println( m-=20 ); System.out.println( k*=2 ); System.out.println( m/=5 ); System.out.println( m%=5 ); }
Self increasing and self decreasing operator
The operation symbols of + 1 or - 1 on their own basis are divided into pre + +, post + + and pre -, post -
class PlusDemo{ public static void main(String[] args){ int age = 30; age--; --age; //Whether + + -- before or after, the influence on the variable itself is to determine + 1 or - 1 System.out.println(age);// 30 System.out.println("-------------------------------------"); int money = 100; System.out.println(--money); // 99 System.out.println(money--); // 99 System.out.println(money); // 98 // For + + -- expressions, the position of + + -- is influential, with immediate computability before and delay computability after. } }
Note: the pre + + (pre –) is calculated by itself first, and then the value is given; Post + + (post –) give the value first, and then calculate by yourself
Relational operator
greater than | less than | Less than or equal to | Greater than or equal to | equal | Unequal |
---|---|---|---|---|---|
> | < | <= | >= | == | != |
public static void main(String[] args){ int m = 10; int k = 20; System.out.println( m>k ); System.out.println( m<k ); System.out.println( m>=k ); System.out.println( m<=k ); System.out.println( m==k ); System.out.println( m!=k ); }
Note: it is used to compare the size relationship of data, and the operation result is Boolean; Note the difference between relational operators and assignment operators
Logical operation
And (short circuit) | Or (short circuit) | wrong | And (without open circuit) | Or (no short circuit) |
---|---|---|---|---|
&& | || | ! | & | | |
public static void main(String[] args){ int m = 10; int k = 20; System.out.println( m>0 && k>0 ); }
Note: it is used for multi condition logic judgment, and the operation result is Boolean; Pay attention to the short circuit
ternary operator
Three eyes |
---|
? : |
public static void main(String[] args){ int m = 10; int k = 20; int t = 1>0?m:k; }
Note: it can be used for logical judgment and assign values to variables according to the judgment results
Bitwise Operators
Bitwise AND | Bitwise OR | Bitwise XOR | Bitwise inversion | Shift left | Shift right | unsigned right shift |
---|---|---|---|---|---|---|
& | | | ^ | ~ | << | >> | >>> |
Same as 1 | If there is one, there is one | The difference is 1 and the same is 0 | 0, 1 interchange | Move left | Move right | Move to the right without retaining the negative sign |
public static void main(String[] args){ int m = 10; int k = 20; System.out.println( m&k ); }
Application of bit operation
- Exchange of two numbers (whether it can be used or not)
public static void main(String[] args){ int m = 10; int k = 20; m ^= k; k ^= m; m ^= k; }
- Check whether the two IP addresses are in the same network segment
Precedence and associativity of operators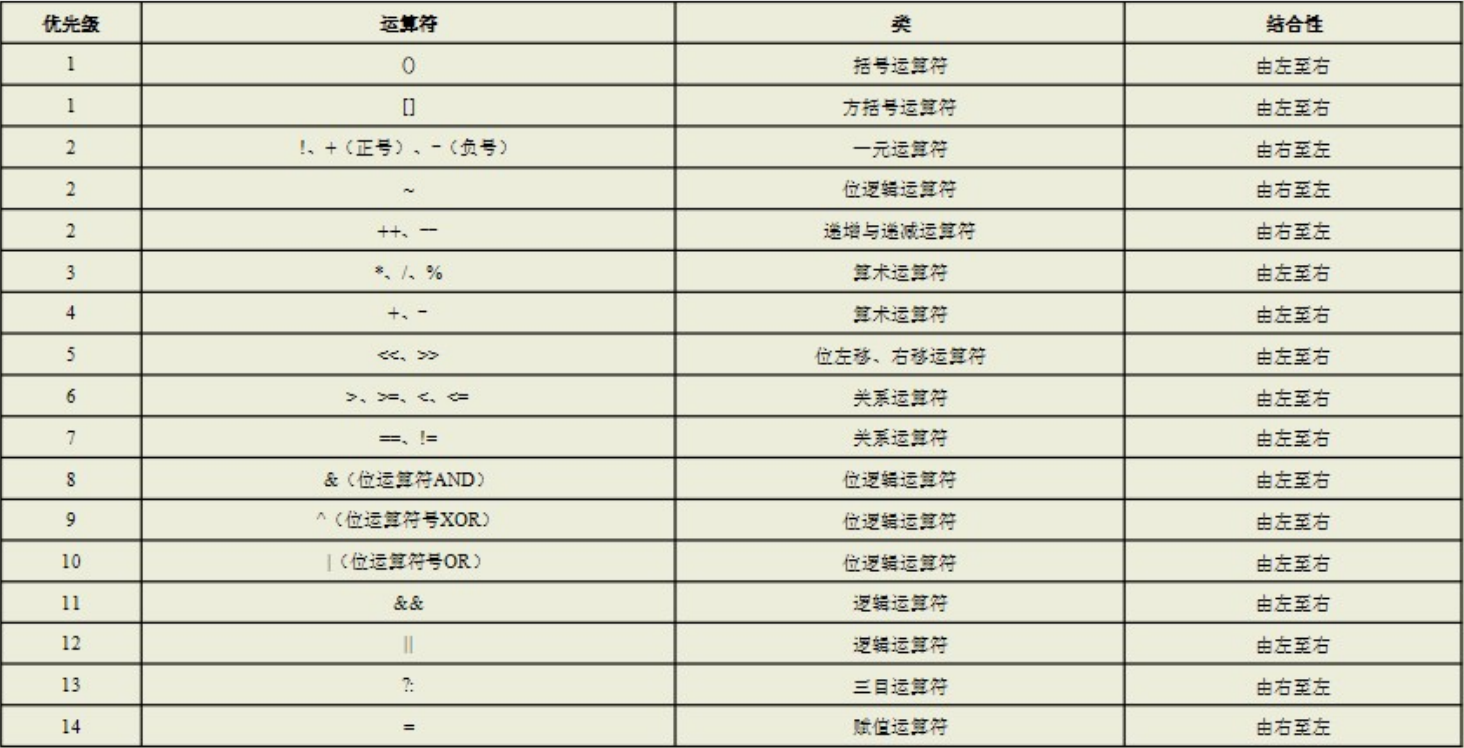
- What is the priority of operators?
The order in which operators are processed when the operation is completed - What is associativity?
Operator deals with the order of operands, which is divided into left associativity and right associativity - Multiple operators with the same priority may appear in an expression. At this time, the operator must be evaluated first through associativity judgment
Note: priority shall be considered before combination
Example: ab+a + +?
+The lowest priority, left associativity, first calculate ab, then a + +, and finally calculate+