Because of working reasons, I have been doing some things with Cocos Creator recently. Personally, it can be said that Cocos Creator is the game gospel of front-end developers. Front-end developers can use their front-end knowledge + Cocos Creator to customize a game of their own.
Just used Cocos Creator for more than a month, I have sorted out some commonly used techniques for your reference.
global variable
When you start creating, you start thinking about how to define and use global variables, and whether they are the same as in JS.
In creator, global variables can be defined without using var to define variables directly, but this method is not advocated by the creator team. Official website provides a definition method:
// Globals.js window.Globals = { name : w, age : 18 }
Creating such a Globals.js script allows you to use Globals.name anywhere to access the value of the defined name.
Module access
If you don't want to imagine too many global variables (which may cause global pollution), you can access them through modules.
//In the test script, it is defined as follows [module.exports is the key word] module.exports= { name:"wgq", age:18 }; //It can be accessed through require in other scripts var test= require("test"); test.age = 20; console.log(test.name)//wgq console.log(test.age)//20
In this way, you can easily set up access to the properties you want!
Plug-in
I believe you will have your own plug-in library when you write js code, so how to use your own plug-in in creator?
It is not enough to create a plug-in script. You must check the import as a plug-in option in the script property checker.
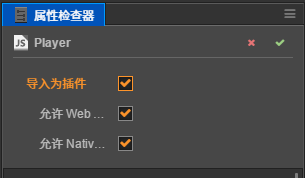
In this way, functions in the plug-in can be referenced anywhere without any declaration.
Get Dom in Creator
In the web, dom can be acquired by using js, and components and nodes can be acquired by using JS in Creator.
Getting Nodes
1. By dragging
You can declare a test attribute in a node's script with code type cc.Node.
//wgq.js properties: { test:{ default:null, type:cc.Node } },
This will result in an empty node in the place where the script is mounted.
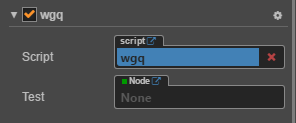
Then you can drag any node on the hierarchical manager to the test control and get it through the code this.test to perform a series of operations.
2. Through code
Global lookup is available
cc.find("Canvas/Menu/Back")
When cc.find passes in only the first parameter, it will start to look up step by step from the scene root node.
Search can be done layer by layer through child nodes.
var son1 = this.node.getChildByName("son1"); var son2 = son1.getChildByName("son2");
Here, son1 is the child node under this.node, and son2 is the child node under son1, which can be searched layer by layer through getChildByName function.
You can also search by serial number without name.
For example, there are many son2 in the following son1
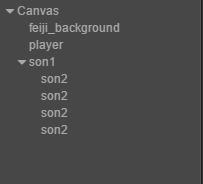
Each son2 can be obtained through the following loops:
var son2 = []; for(var i=0;i<son1.childrenCount;i++){ var son2[i] = son1.chindren[i] }
Getting components
In Creator, a node can mount multiple components, as follows:
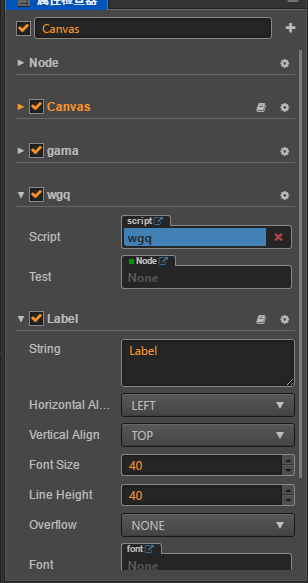
Canvas nodes have Canvas components, gama and wgq script components, Label rendering components and so on.
Take the son node as an example:
var son = this.node.getChildByName("son"); var label = son.getComponent(cc.Label); //In this way, the label component under the son node can be obtained, and then some operations can be performed on the component.
Node attributes
Nodes have some attributes. In development, we always use js to dynamically change the attributes of nodes.
var son = this.node.getChildByName("son"); //The following code son is the acquired node son.active = true or false //Display Hiding of Control Node son son.parent = father or ... //Change the parent node of the son node son.children //Returns an array of child nodes under the son node son.childrenCount //Returns the number of child nodes under the sending node son.x or son.y = 10//Setting x, y axis coordinates of son node son.rotation = 90(Degrees)//Setting the rotation angle of son node son.scaleX = 2 (Default 1)//Setting the zoom of son node son.width or son.height //Changing the Length and Width of the son Node son.color //Set the color (default is white) son.opacity = 200(0~255,The default is 255)//Setting Transparency
Dynamic addition of components for nodes
Sometimes we need to add components dynamically for nodes
var myLabel = new cc.Node().addComponent(cc.Label); myLabel.string = "test"; myLable.node.parent = son;//Setting Nodes myLable.node.setPosition(300,200);//Location