Game Item Management System
@email: lovefyj616@foxmail.com
This management system can store the same kind of items, support the operation of selling, using, removing, purchasing, etc. In addition, it introduces the attributes of personal life value, manpower value, gold coin, attacking power to show the excellent data binding operation of this system.
For example, Apple's effect is "Mana + 10". When clicked on it, the person's corresponding life value attribute + 10 will be updated simultaneously with the red progress bar (blood bar) bound to that attribute. When you click on the purchase bomb, the system will first determine if the user has enough money to buy it and respond...
When you deploy this system, you need to import project dependencies (mentioned below), import sql files from the src directory into the database, and modify the database connection configuration file to work properly!
1. Project dependencies (packages) and structure
1.1 Project Dependency
javafx-sdk-11.0.2,mysql-connector-java-8.8.16,mybatis,log4j-1.2.17
1.2 Project Structure
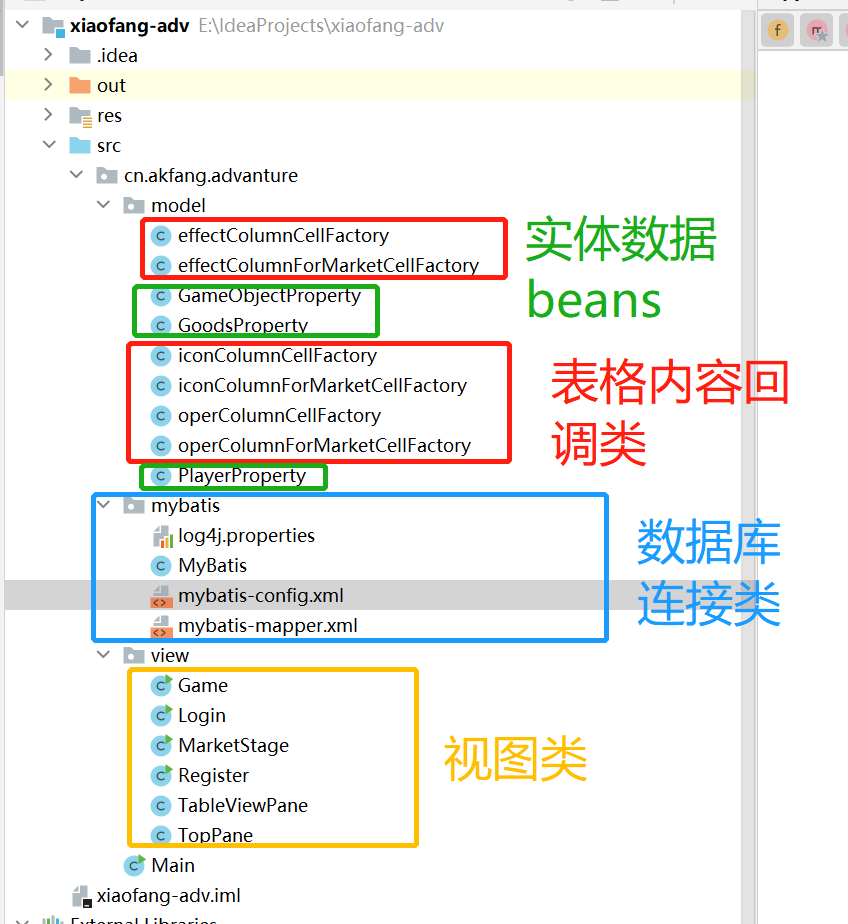
1.3 Database Structure

2. Class structure (UML diagram)
2.1 Data Beans
Storage used for data and dynamic binding between fx components
2.2 Main Interface
2.2.1 Game Main Class (Game.java)
2.2.2 Store Class (MarketStage.java)
2.2.3 Login (Login.java), Register class (Register.java)
2.2.4 Custom Panel Classes (TableViewPane.java, TopPane)
2.3 Tool Class (Connect to Database)
3. Storage, reading and query of data
All database operations are defined in MyBatis as static methods
3.2 Introducing MyBatis Factory singleton mode
3.2.1 Configure database address, user name, password
In project cn/akfang/advanture/mybatis/mybatis-config. In xml, configure database information
In the property label, fill in the labels with name url, username, password
The following code indicates that the database address is native (127.0.0.1), the database name is xiaofang, the user name is root, and the password is root
... <dataSource type="POOLED"> <property name="driver" value="com.mysql.cj.jdbc.Driver"/> <property name="url" value="jdbc:mysql://127.0.0.1/xiaofang?useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai"/> <property name="username" value="root"/> <property name="password" value="root"/> </dataSource> ...
3.2.2 Singleton Mode
static { System.out.println("MyBatis factory Initialization ..."); // Static code block: Initialize SqlSessionFactory instance try{ String resource = "./cn/akfang/advanture/mybatis/mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); factory = new SqlSessionFactoryBuilder().build(inputStream); } catch(Exception e) { e.printStackTrace(); } }
3.3 in mapper. Define all operations in XML
This is a limited space, add, delete, and check one display for more details
<select id="checkUserExists" parameterType="hashmap" resultType="hashmap"> select * from `player_profile` where nick_name=#{nick_name}; </select>
<insert id="insertUser" parameterType="hashmap" useGeneratedKeys="true" keyProperty="player_id" > INSERT INTO `player_profile` (`player_id`,`nick_name`,`password`,`registered_date`) VALUES (#{player_id},#{nick_name},#{password},#{registered_date}); </insert>
<update id="updatePlayerBoxItem" parameterType="hashmap" > update `player_box` set `amount`=#{amount} where object_id=#{object_id} </update>
<delete id="removeNullItemInPlayBox" parameterType="hashmap"> delete from player_box where object_id=#{object_id} and owner_id=#{owner_id} </delete>
...
3.4 Packaging Sqlsession
All session s also exist as static methods in the MyBatis class
public static HashMap<Integer,HashMap<String,Object>> getObjectPropertyFromDatabase(){ HashMap<Integer,HashMap<String,Object>> objectProperty = new HashMap<>(); try (SqlSession session = MyBatis.factory.openSession()){ List<Map> resultList = session.selectList("getObjectClass"); resultList.forEach(item -> { int key = (int) item.get("class_id"); HashMap<String,Object> value = new HashMap<>(); value.put("name",item.get("name")); value.put("cost",item.get("cost")); String[] effect = ((String)item.get("effect")).split(","); for (String effects :effect){ String[] effectMap = effects.split(":"); value.put(effectMap[0],Integer.parseInt(effectMap[1])); } objectProperty.put(key,value); }); } return objectProperty; }
public static void updatePlayerConditionHp(int player_id,int hp){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionHp",new HashMap<>(){{ put("player_id",player_id); put("hp",hp); }}); session.commit(); } }
public static void updatePlayerConditionMoney(int player_id,int money){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionMoney",new HashMap<>(){{ put("player_id",player_id); put("money",money); }}); session.commit(); } }
...
4. Layout
4.1 Game.java
4.2 TopPane.java
4.3 TableViewPane.java
4.4 Market.java
5. Demonstration of some key operations
5.1 Login and Registration
5.1.1 Registration
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, submit an insert and commit the transaction
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.1.2 Login
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, a prompt box pops up
Conversely, a Map with player data is passed into Game.java, run the begin() method of the Game class
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.2 Item handling
5.2.1 Item Use
bt[0].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } HashMap<String, Object> usage = Game.objectProperty.get(thisItem.getClassId()); for (String key : usage.keySet()) { if (key.equals("attack")) player.setAttack(player.getAttack() + (Integer) usage.get("attack")); if (key.equals("hp")) { int after = player.getHp() + (Integer) usage.get("hp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Life Value!").show(); return; } player.setHp(after); } if (key.equals("mp")) { int after = player.getMp() + (Integer) usage.get("mp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Mandatory Value!").show(); return; } player.setMp(player.getMp() + (Integer) usage.get("mp")); } } thisItem.setAmount(thisItem.getAmount() - 1); //System.out.println(player.mpProperty().get()); });
5.2.2 Item Sale
bt[1].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } thisItem.setAmount(thisItem.getAmount()-1); player.setMoney(player.getMoney()+thisItem.getCost()); });
5.2.4 Item Removal
Button bt33 = new Button("remove"); this.setGraphic(bt33); bt33.setOnAction(event->{ MyBatis.deleteNullItemInPlayerBox(thisItem.getId(),player.getPlayer_id()); data.remove(getIndex()); });
5.2.5 Item Purchase
buyButton.setOnAction(event->{ int money = player.getMoney(); if(money<thisItem.getCost()) return; player.setMoney(player.getMoney()-thisItem.getCost()); GameObjectProperty result = checkPlayerBoxExists(thisItem.getClass_id()); if(result!=null){ result.setAmount(result.getAmount()+1); //update } else { int feeback_id = MyBatis.insertItemInPlayerBox(player.getPlayer_id(),thisItem.getClass_id(),1); //System.out.println(feeback_id); playerbox.add(new GameObjectProperty(feeback_id,thisItem.getClass_id(),thisItem.getName(),thisItem.getCost(),1)); } });
5.3 Attribute increase or decrease
5.3.1 Blood Strip Increase or Decrease
public void setHp(int hp) { if(hp<0) return; MyBatis.updatePlayerConditionHp(getPlayer_id(),hp); this.hp.set(hp); }
5.3.2 Increase or decrease of blue bars
public void setMp(int mp) { if(mp<0) return; MyBatis.updatePlayerConditionMp(getPlayer_id(),mp); this.mp.set(mp); }
5.3.3 Money increase or decrease
public void setMoney(int money) { if(money<0) return; MyBatis.updatePlayerConditionMoney(getPlayer_id(),money); this.money.set(money); }
Game Item Management System
@author: Class 20 Accounting Class 3 Fang Yujie 2020122104559
@email: lovefyj616@foxmail.com
This management system can store the same kind of items, support the operation of selling, using, removing, purchasing, etc. In addition, it introduces the attributes of personal life value, manpower value, gold coin, attacking power to show the excellent data binding operation of this system.
For example, Apple's effect is "Mana + 10". When clicked on it, the person's corresponding life value attribute + 10 will be updated simultaneously with the red progress bar (blood bar) bound to that attribute. When you click on the purchase bomb, the system will first determine if the user has enough money to buy it and respond...
When you deploy this system, you need to import project dependencies (mentioned below), import sql files from the src directory into the database, and modify the database connection configuration file to work properly!
1. Project dependencies (packages) and structure
1.1 Project Dependency
javafx-sdk-11.0.2,mysql-connector-java-8.8.16,mybatis,log4j-1.2.17
1.2 Project Structure
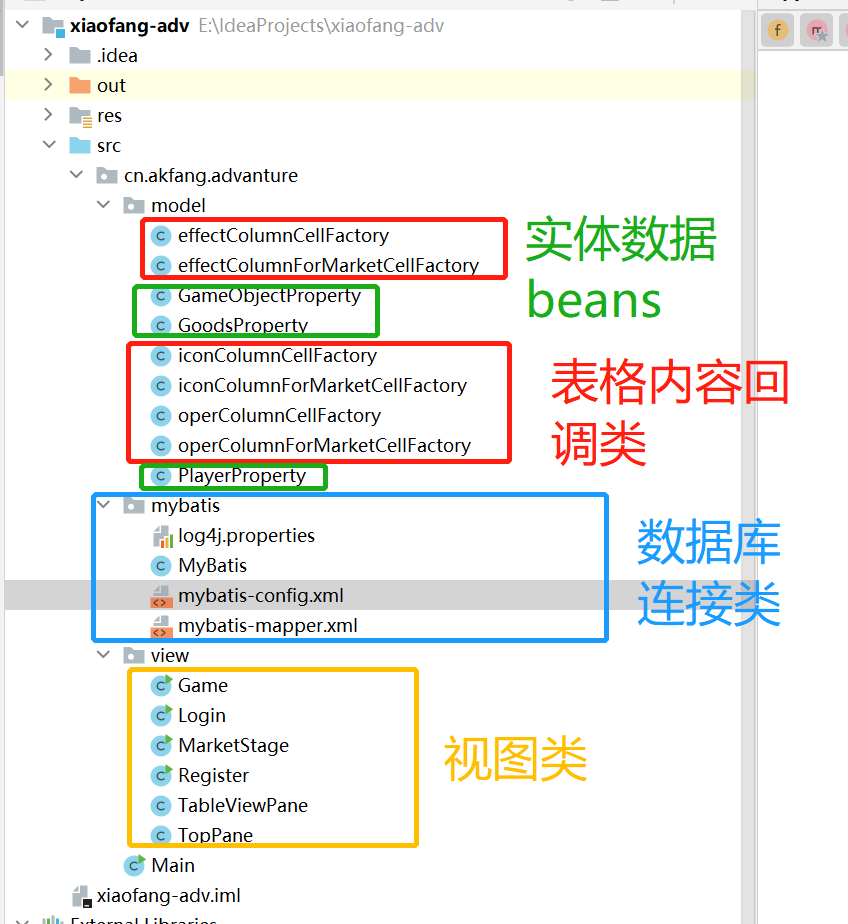
1.3 Database Structure

2. Class structure (UML diagram)
2.1 Data Beans
Storage used for data and dynamic binding between fx components
2.2 Main Interface
2.2.1 Game Main Class (Game.java)
2.2.2 Store Class (MarketStage.java)
2.2.3 Login (Login.java), Register class (Register.java)
2.2.4 Custom Panel Classes (TableViewPane.java, TopPane)
2.3 Tool Class (Connect to Database)
3. Storage, reading and query of data
All database operations are defined in MyBatis as static methods
3.2 Introducing MyBatis Factory singleton mode
3.2.1 Configure database address, user name, password
In project cn/akfang/advanture/mybatis/mybatis-config. In xml, configure database information
In the property label, fill in the labels with name url, username, password
The following code indicates that the database address is native (127.0.0.1), the database name is xiaofang, the user name is root, and the password is root
... <dataSource type="POOLED"> <property name="driver" value="com.mysql.cj.jdbc.Driver"/> <property name="url" value="jdbc:mysql://127.0.0.1/xiaofang?useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai"/> <property name="username" value="root"/> <property name="password" value="root"/> </dataSource> ...
3.2.2 Singleton Mode
static { System.out.println("MyBatis factory Initialization ..."); // Static code block: Initialize SqlSessionFactory instance try{ String resource = "./cn/akfang/advanture/mybatis/mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); factory = new SqlSessionFactoryBuilder().build(inputStream); } catch(Exception e) { e.printStackTrace(); } }
3.3 in mapper. Define all operations in XML
This is a limited space, add, delete, and check one display for more details
<select id="checkUserExists" parameterType="hashmap" resultType="hashmap"> select * from `player_profile` where nick_name=#{nick_name}; </select>
<insert id="insertUser" parameterType="hashmap" useGeneratedKeys="true" keyProperty="player_id" > INSERT INTO `player_profile` (`player_id`,`nick_name`,`password`,`registered_date`) VALUES (#{player_id},#{nick_name},#{password},#{registered_date}); </insert>
<update id="updatePlayerBoxItem" parameterType="hashmap" > update `player_box` set `amount`=#{amount} where object_id=#{object_id} </update>
<delete id="removeNullItemInPlayBox" parameterType="hashmap"> delete from player_box where object_id=#{object_id} and owner_id=#{owner_id} </delete>
...
3.4 Packaging Sqlsession
All session s also exist as static methods in the MyBatis class
public static HashMap<Integer,HashMap<String,Object>> getObjectPropertyFromDatabase(){ HashMap<Integer,HashMap<String,Object>> objectProperty = new HashMap<>(); try (SqlSession session = MyBatis.factory.openSession()){ List<Map> resultList = session.selectList("getObjectClass"); resultList.forEach(item -> { int key = (int) item.get("class_id"); HashMap<String,Object> value = new HashMap<>(); value.put("name",item.get("name")); value.put("cost",item.get("cost")); String[] effect = ((String)item.get("effect")).split(","); for (String effects :effect){ String[] effectMap = effects.split(":"); value.put(effectMap[0],Integer.parseInt(effectMap[1])); } objectProperty.put(key,value); }); } return objectProperty; }
public static void updatePlayerConditionHp(int player_id,int hp){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionHp",new HashMap<>(){{ put("player_id",player_id); put("hp",hp); }}); session.commit(); } }
public static void updatePlayerConditionMoney(int player_id,int money){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionMoney",new HashMap<>(){{ put("player_id",player_id); put("money",money); }}); session.commit(); } }
...
4. Layout
4.1 Game.java
4.2 TopPane.java
4.3 TableViewPane.java
4.4 Market.java
5. Demonstration of some key operations
5.1 Login and Registration
5.1.1 Registration
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, submit an insert and commit the transaction
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.1.2 Login
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, a prompt box pops up
Conversely, a Map with player data is passed into Game.java, run the begin() method of the Game class
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.2 Item handling
5.2.1 Item Use
bt[0].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } HashMap<String, Object> usage = Game.objectProperty.get(thisItem.getClassId()); for (String key : usage.keySet()) { if (key.equals("attack")) player.setAttack(player.getAttack() + (Integer) usage.get("attack")); if (key.equals("hp")) { int after = player.getHp() + (Integer) usage.get("hp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Life Value!").show(); return; } player.setHp(after); } if (key.equals("mp")) { int after = player.getMp() + (Integer) usage.get("mp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Mandatory Value!").show(); return; } player.setMp(player.getMp() + (Integer) usage.get("mp")); } } thisItem.setAmount(thisItem.getAmount() - 1); //System.out.println(player.mpProperty().get()); });
5.2.2 Item Sale
bt[1].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } thisItem.setAmount(thisItem.getAmount()-1); player.setMoney(player.getMoney()+thisItem.getCost()); });
5.2.4 Item Removal
Button bt33 = new Button("remove"); this.setGraphic(bt33); bt33.setOnAction(event->{ MyBatis.deleteNullItemInPlayerBox(thisItem.getId(),player.getPlayer_id()); data.remove(getIndex()); });
5.2.5 Item Purchase
buyButton.setOnAction(event->{ int money = player.getMoney(); if(money<thisItem.getCost()) return; player.setMoney(player.getMoney()-thisItem.getCost()); GameObjectProperty result = checkPlayerBoxExists(thisItem.getClass_id()); if(result!=null){ result.setAmount(result.getAmount()+1); //update } else { int feeback_id = MyBatis.insertItemInPlayerBox(player.getPlayer_id(),thisItem.getClass_id(),1); //System.out.println(feeback_id); playerbox.add(new GameObjectProperty(feeback_id,thisItem.getClass_id(),thisItem.getName(),thisItem.getCost(),1)); } });
5.3 Attribute increase or decrease
5.3.1 Blood Strip Increase or Decrease
public void setHp(int hp) { if(hp<0) return; MyBatis.updatePlayerConditionHp(getPlayer_id(),hp); this.hp.set(hp); }
5.3.2 Increase or decrease of blue bars
public void setMp(int mp) { if(mp<0) return; MyBatis.updatePlayerConditionMp(getPlayer_id(),mp); this.mp.set(mp); }
5.3.3 Money increase or decrease
public void setMoney(int money) { if(money<0) return; MyBatis.updatePlayerConditionMoney(getPlayer_id(),money); this.money.set(money); }
Game Item Management System
@author: Class 20 Accounting Class 3 Fang Yujie 2020122104559
@email: lovefyj616@foxmail.com
This management system can store the same kind of items, support the operation of selling, using, removing, purchasing, etc. In addition, it introduces the attributes of personal life value, manpower value, gold coin, attacking power to show the excellent data binding operation of this system.
For example, Apple's effect is "Mana + 10". When clicked on it, the person's corresponding life value attribute + 10 will be updated simultaneously with the red progress bar (blood bar) bound to that attribute. When you click on the purchase bomb, the system will first determine if the user has enough money to buy it and respond...
When you deploy this system, you need to import project dependencies (mentioned below), import sql files from the src directory into the database, and modify the database connection configuration file to work properly!
1. Project dependencies (packages) and structure
1.1 Project Dependency
javafx-sdk-11.0.2,mysql-connector-java-8.8.16,mybatis,log4j-1.2.17
1.2 Project Structure
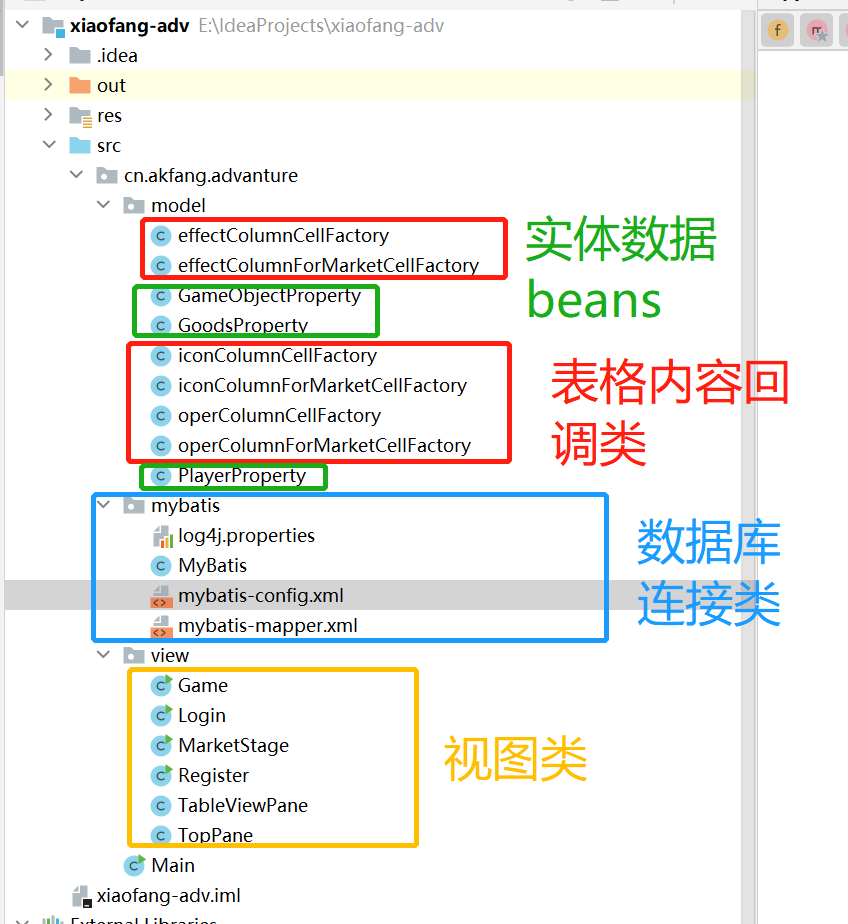
1.3 Database Structure

2. Class structure (UML diagram)
2.1 Data Beans
Storage used for data and dynamic binding between fx components
2.2 Main Interface
2.2.1 Game Main Class (Game.java)
2.2.2 Store Class (MarketStage.java)
2.2.3 Login (Login.java), Register class (Register.java)
2.2.4 Custom Panel Classes (TableViewPane.java, TopPane)
2.3 Tool Class (Connect to Database)
3. Storage, reading and query of data
All database operations are defined in MyBatis as static methods
3.2 Introducing MyBatis Factory singleton mode
3.2.1 Configure database address, user name, password
In project cn/akfang/advanture/mybatis/mybatis-config. In xml, configure database information
In the property label, fill in the labels with name url, username, password
The following code indicates that the database address is native (127.0.0.1), the database name is xiaofang, the user name is root, and the password is root
... <dataSource type="POOLED"> <property name="driver" value="com.mysql.cj.jdbc.Driver"/> <property name="url" value="jdbc:mysql://127.0.0.1/xiaofang?useUnicode=true&characterEncoding=UTF-8&serverTimezone=Asia/Shanghai"/> <property name="username" value="root"/> <property name="password" value="root"/> </dataSource> ...
3.2.2 Singleton Mode
static { System.out.println("MyBatis factory Initialization ..."); // Static code block: Initialize SqlSessionFactory instance try{ String resource = "./cn/akfang/advanture/mybatis/mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); factory = new SqlSessionFactoryBuilder().build(inputStream); } catch(Exception e) { e.printStackTrace(); } }
3.3 in mapper. Define all operations in XML
This is a limited space, add, delete, and check one display for more details
<select id="checkUserExists" parameterType="hashmap" resultType="hashmap"> select * from `player_profile` where nick_name=#{nick_name}; </select>
<insert id="insertUser" parameterType="hashmap" useGeneratedKeys="true" keyProperty="player_id" > INSERT INTO `player_profile` (`player_id`,`nick_name`,`password`,`registered_date`) VALUES (#{player_id},#{nick_name},#{password},#{registered_date}); </insert>
<update id="updatePlayerBoxItem" parameterType="hashmap" > update `player_box` set `amount`=#{amount} where object_id=#{object_id} </update>
<delete id="removeNullItemInPlayBox" parameterType="hashmap"> delete from player_box where object_id=#{object_id} and owner_id=#{owner_id} </delete>
...
3.4 Packaging Sqlsession
All session s also exist as static methods in the MyBatis class
public static HashMap<Integer,HashMap<String,Object>> getObjectPropertyFromDatabase(){ HashMap<Integer,HashMap<String,Object>> objectProperty = new HashMap<>(); try (SqlSession session = MyBatis.factory.openSession()){ List<Map> resultList = session.selectList("getObjectClass"); resultList.forEach(item -> { int key = (int) item.get("class_id"); HashMap<String,Object> value = new HashMap<>(); value.put("name",item.get("name")); value.put("cost",item.get("cost")); String[] effect = ((String)item.get("effect")).split(","); for (String effects :effect){ String[] effectMap = effects.split(":"); value.put(effectMap[0],Integer.parseInt(effectMap[1])); } objectProperty.put(key,value); }); } return objectProperty; }
public static void updatePlayerConditionHp(int player_id,int hp){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionHp",new HashMap<>(){{ put("player_id",player_id); put("hp",hp); }}); session.commit(); } }
public static void updatePlayerConditionMoney(int player_id,int money){ try (SqlSession session = MyBatis.factory.openSession()){ session.update("updatePlayerConditionMoney",new HashMap<>(){{ put("player_id",player_id); put("money",money); }}); session.commit(); } }
...
4. Layout
4.1 Game.java
4.2 TopPane.java
4.3 TableViewPane.java
4.4 Market.java
5. Demonstration of some key operations
5.1 Login and Registration
5.1.1 Registration
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, submit an insert and commit the transaction
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.1.2 Login
After clicking the Register button, a select is submitted to the database
try (SqlSession session = MyBatis.factory.openSession()){ List<Map> result = session.selectList( "reg.checkUserExists", new HashMap<>(){{ put("nick_name",tf[0].getText()); }} ); ... }
If there is no account with this nickname in the database, a prompt box pops up
Conversely, a Map with player data is passed into Game.java, run the begin() method of the Game class
if(result.size()!=1){ new Alert(Alert.AlertType.WARNING,"No corresponding user name found").show(); } else { String truePassword = (String) result.get(0).get("password"); if(truePassword.equals(pf.getText())){ thisStage.close(); new Game().begin(this.thisStage,result.get(0)); } else { new Alert(Alert.AlertType.WARNING,"Wrong password!").show(); } }
5.2 Item handling
5.2.1 Item Use
bt[0].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } HashMap<String, Object> usage = Game.objectProperty.get(thisItem.getClassId()); for (String key : usage.keySet()) { if (key.equals("attack")) player.setAttack(player.getAttack() + (Integer) usage.get("attack")); if (key.equals("hp")) { int after = player.getHp() + (Integer) usage.get("hp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Life Value!").show(); return; } player.setHp(after); } if (key.equals("mp")) { int after = player.getMp() + (Integer) usage.get("mp"); if (after < 0) { new Alert(Alert.AlertType.WARNING, "Insufficient Mandatory Value!").show(); return; } player.setMp(player.getMp() + (Integer) usage.get("mp")); } } thisItem.setAmount(thisItem.getAmount() - 1); //System.out.println(player.mpProperty().get()); });
5.2.2 Item Sale
bt[1].setOnAction(event->{ if(thisItem.getAmount()==0){ return; } thisItem.setAmount(thisItem.getAmount()-1); player.setMoney(player.getMoney()+thisItem.getCost()); });
5.2.4 Item Removal
Button bt33 = new Button("remove"); this.setGraphic(bt33); bt33.setOnAction(event->{ MyBatis.deleteNullItemInPlayerBox(thisItem.getId(),player.getPlayer_id()); data.remove(getIndex()); });
5.2.5 Item Purchase
buyButton.setOnAction(event->{ int money = player.getMoney(); if(money<thisItem.getCost()) return; player.setMoney(player.getMoney()-thisItem.getCost()); GameObjectProperty result = checkPlayerBoxExists(thisItem.getClass_id()); if(result!=null){ result.setAmount(result.getAmount()+1); //update } else { int feeback_id = MyBatis.insertItemInPlayerBox(player.getPlayer_id(),thisItem.getClass_id(),1); //System.out.println(feeback_id); playerbox.add(new GameObjectProperty(feeback_id,thisItem.getClass_id(),thisItem.getName(),thisItem.getCost(),1)); } });
5.3 Attribute increase or decrease
5.3.1 Blood Strip Increase or Decrease
public void setHp(int hp) { if(hp<0) return; MyBatis.updatePlayerConditionHp(getPlayer_id(),hp); this.hp.set(hp); }
5.3.2 Increase or decrease of blue bars
public void setMp(int mp) { if(mp<0) return; MyBatis.updatePlayerConditionMp(getPlayer_id(),mp); this.mp.set(mp); }
5.3.3 Money increase or decrease
public void setMoney(int money) { if(money<0) return; MyBatis.updatePlayerConditionMoney(getPlayer_id(),money); this.money.set(money); }