Getting started with Python - day 4
Application of branch and loop structure
The importance of branch and loop structure in Python is self-evident. It is the basis of constructing program logic. We need to strengthen programming practice.
Exercise 1:
Find the number of daffodils between 100 and 999 (the cube sum of each number is just equal to the number itself)
153 = 1^3 + 5^3 +3^3
for i in range(100, 1000): a = i // 100 b = i // 10 % 10 c = i % 10 if a ** 3 + b ** 3 + c ** 3 == i: print(i)

Exercise 2:
Find the perfect number between 1 and 10000 (the sum of all factors except itself is equal to this number)
6= 1 +2 + 3
28 = 1 +2+4+7 +14
for num in range(2, 10001): total = 1 for i in range(2, int(num ** 0.5) + 1): if num % i == 0: total += i if i != num // i: total += num // i if num == total: print(num)
int(num ** 0.5) + 1 if i != num // i: total += num // i It is used to reduce computer calculation and increase running speed

import time start = time.time() end = time.time() print(f'Execution time:{end - start:.3f}second') Used to calculate the running time of the computer
Exercise 3:
Enter m and n (M > = n) to calculate the value of C(m,n) - > m/ (n! * (m - n)!)
from math import factorial as f m = int(input('m = ')) n = int(input('n = ')) print(f(m) / f(n) / f(m - n))

as - > alias - > alias
Exercise 4:
Enter a positive integer and judge whether it is a prime number (a number that can only be divided by 1 and itself)
num = int(input('Enter a positive integer:')) b = True for i in range(2, int(num ** 0.5) + 1): if num % i == 0: b = False break if b and num > 1: print(f'{num}Is a prime number') else: print(f'{num}Not prime')

Break keyword, which is used to end the loop in advance. It should be noted that break can only terminate the loop in which it is located.
Exercise 5:
Enter the length of the three sides of the triangle. If it can form a triangle, calculate the perimeter and area. If it cannot form a triangle, prompt the user to re-enter until it is correct
while True: a = float(input('a = ')) b = float(input('b = ')) c = float(input('c = ')) p = 0.5 * (a + b + c) if a + b > c and a + c > b and b + c > a: print(f'The perimeter of the triangle is:{a + b + c}') print(f'The triangle area is:{(p * (p - a) * (p -b) * (p -c)) ** 0.5}') break else: print('Please re-enter')

Exercise 6:
Enter a positive integer N to reverse N
n = int(input('N = ')) total = 0 while n != 0: a = n // 10 b = n % 10 total = total * 10 + b n = a print(total)
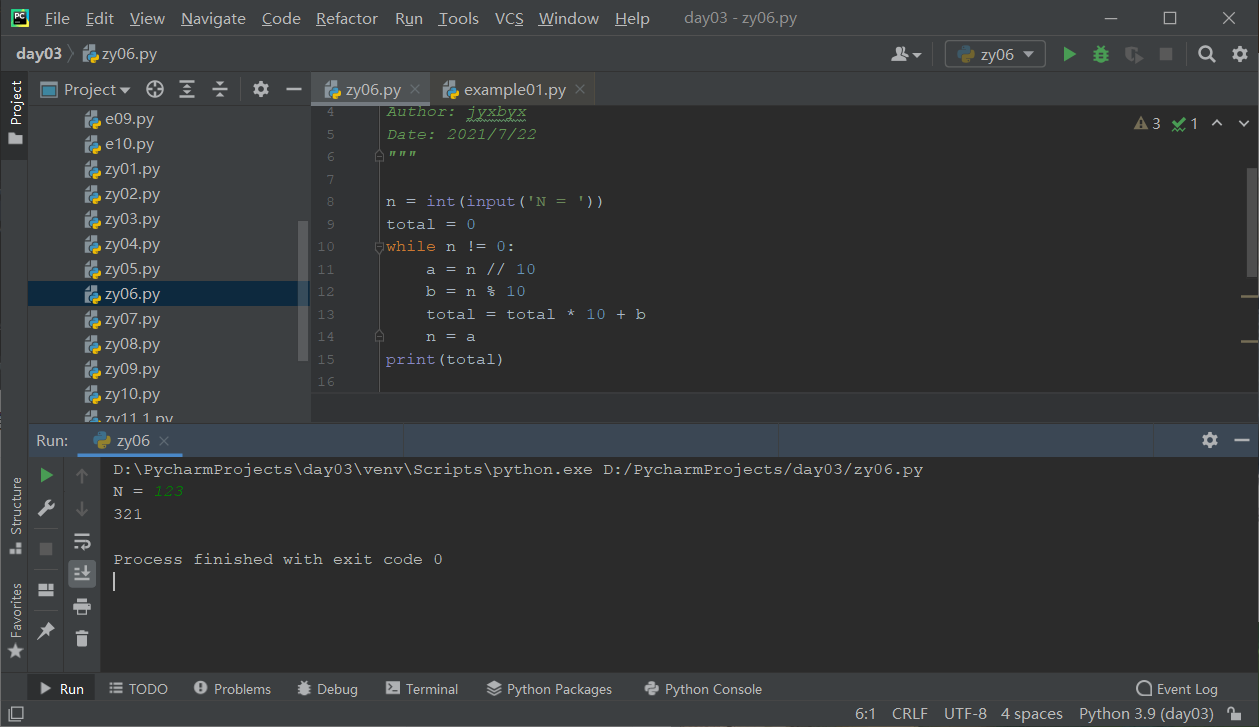
Exercise 7:
Print out prime numbers in the range of 1-100
for num in range(1, 101): b = True for i in range(2, num): if num % i == 0: b = False break if b and num > 1: print(num, end=' ')
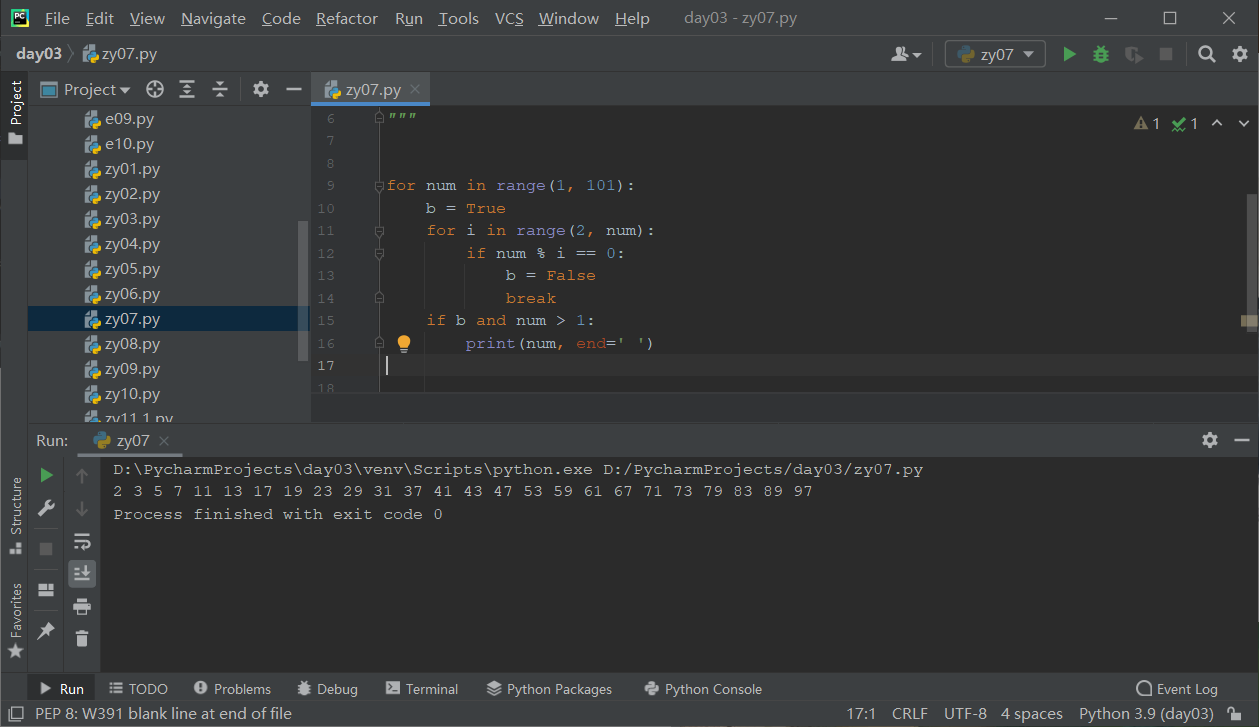
Exercise 8:
Print 99 multiplication table
for i in range(1, 10): for j in range(1, i + 1): print(f'{j} * {i} = {i * j}', end='\t') print()
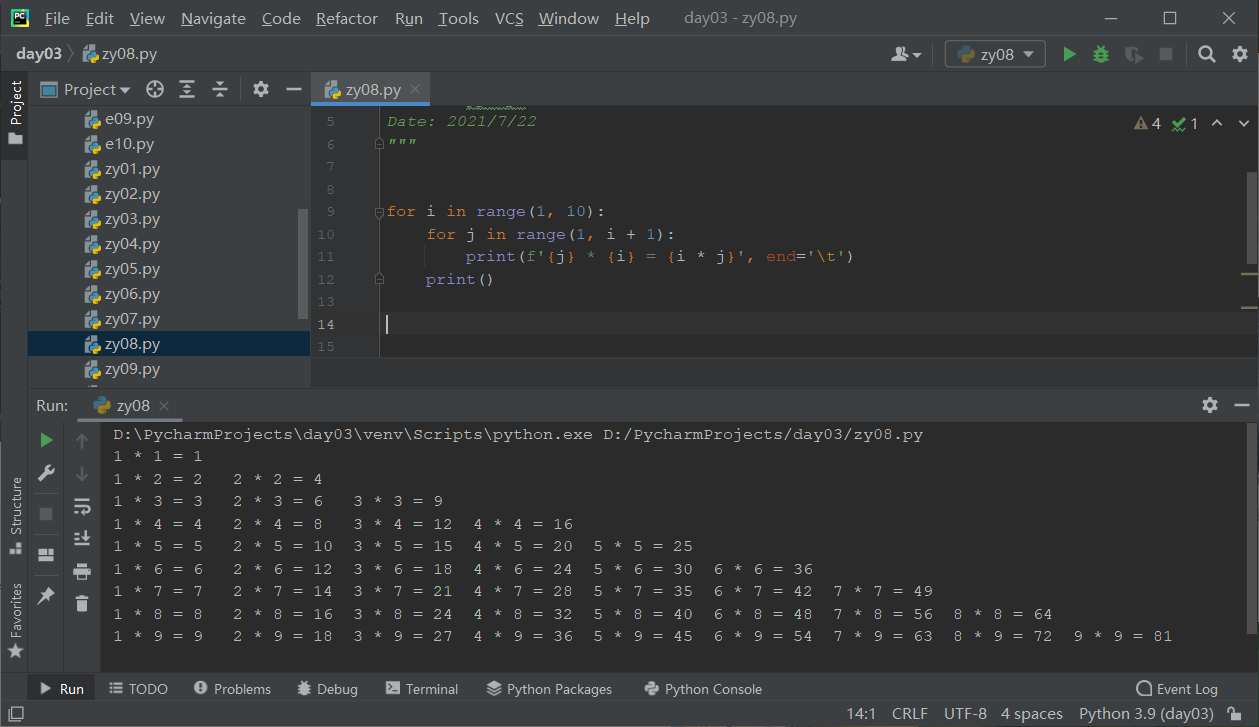
Exercise 9:
Input an N and output n fiboracci numbers
Fibonacci sequence is a very famous sequence in the field of mathematics. Its first items are 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89144 ···················. The biggest feature of this sequence is that each item is equal to the sum of its first two items. From the third number, the ratio of each number to the number behind it is very close to 0.618, which is the "golden section". Fibonacci sequence is also called "rabbit sequence" and golden section sequence. This seemingly simple sequence of numbers always appears in front of people.
n = int(input('n = ')) a = 1 b = 1 print(a, b, sep='\n') for _ in range(0, n-2): a, b = b, a + b print(b)
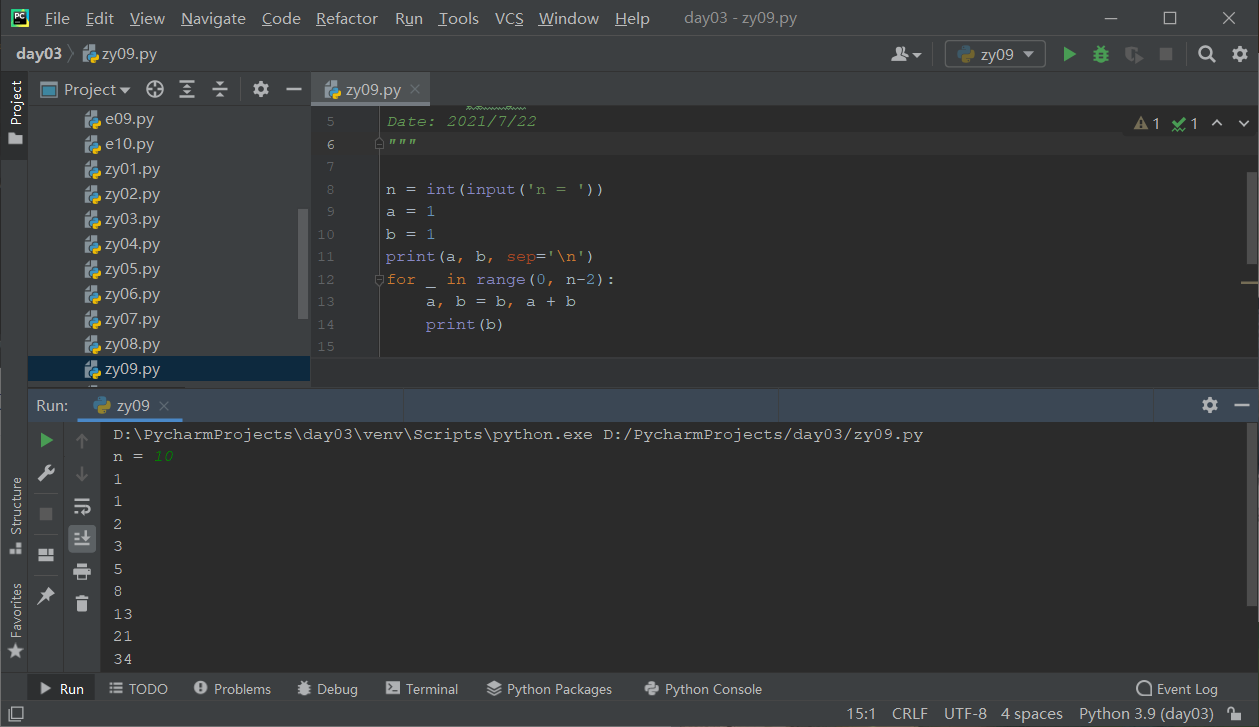
Exercise 10:
A hundred dollars and a hundred chickens
A hen is worth five, a hen is worth three, and a chick is worth three. If you buy a hundred chickens for a hundred dollars, ask the chicken owner, the chicken mother and the chicken chick?
It means five yuan for a rooster, three yuan for a hen and three yuan for a chick. Now I want to buy 100 chickens with 100 yuan. How many roosters, hens and chicks are there?
for a in range(0, 21): for b in range(0, 34): # for c in range(0, 100, 3): c = 100 - a - b if c % 3 == 0 and 5 * a + 3 * b + c // 3 == 100: print(f'The cock has{a}Only, the hen has{b}Chicken, chicken{c}only')

Exhaustive method: exhaust all possibilities, then set conditions and find the solution to the problem - > violent cracking method
Exercise 11:
Five people (ABCDE) went fishing at night, caught countless fish, and then went to bed when they were tired.
The next day, A woke up first, divided the fish into five parts, threw away the extra one, and then took one of his own;
B the second woke up and thought the fish had not been divided. He divided the remaining fish into five parts, threw away the extra one and took one of his own;
C. D and E wake up and divide the fish in the same way. How many fish did they catch at least?
fish = 1 while True: is_enough = True total = fish for _ in range(5): if (total - 1) % 5 == 0: total = (total -1) // 5 * 4 else: is_enough = False break if is_enough: print(fish) break
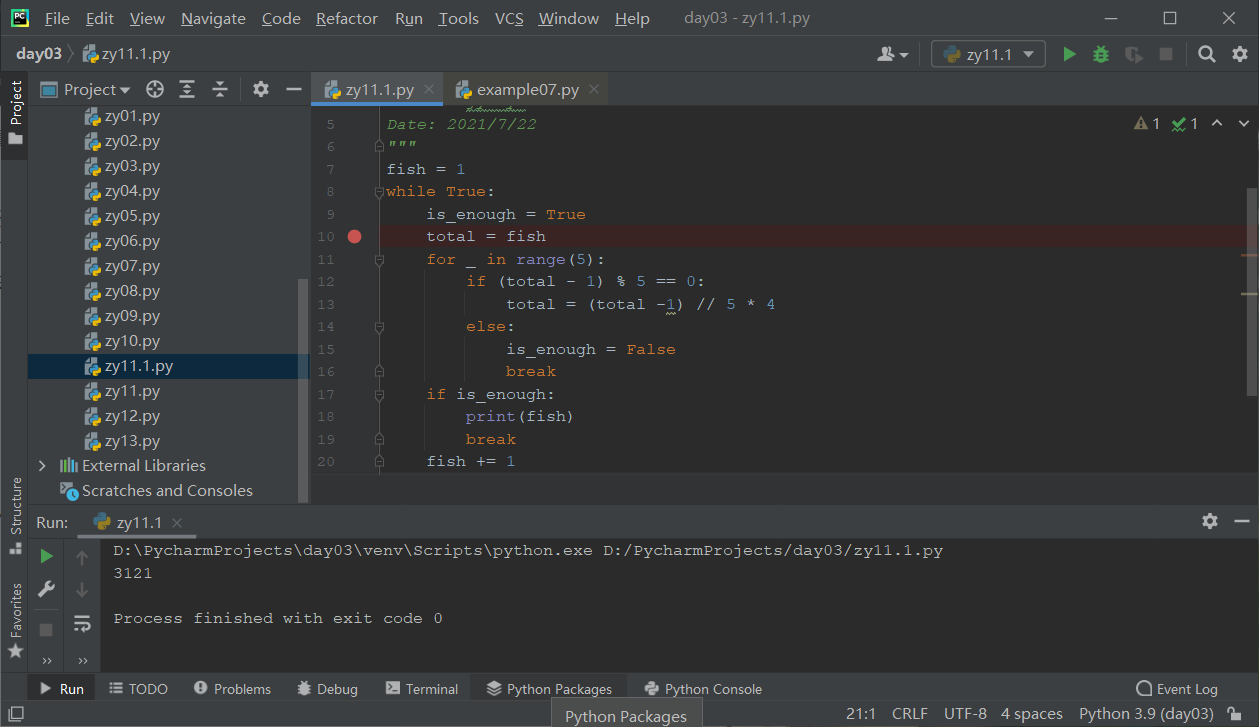
Exercise 12:
Figure guessing game
The computer generates a random number of 1-100, and people input the number they guess,
The computer gives the corresponding prompt "bigger", "smaller" or "Congratulations, you guessed right" until you got it.
If you guess more than 7 times, the computer warm prompt "IQ balance is obviously insufficient".
import random answer = random.randrange(1, 101) i = 1 while True: num = int(input('Please enter a number:')) i += 1 if num > answer: print('smaller one') if num < answer: print('A little bigger') if num == answer: print('You guessed right') break if i > 7: print('The IQ balance is obviously insufficient') break
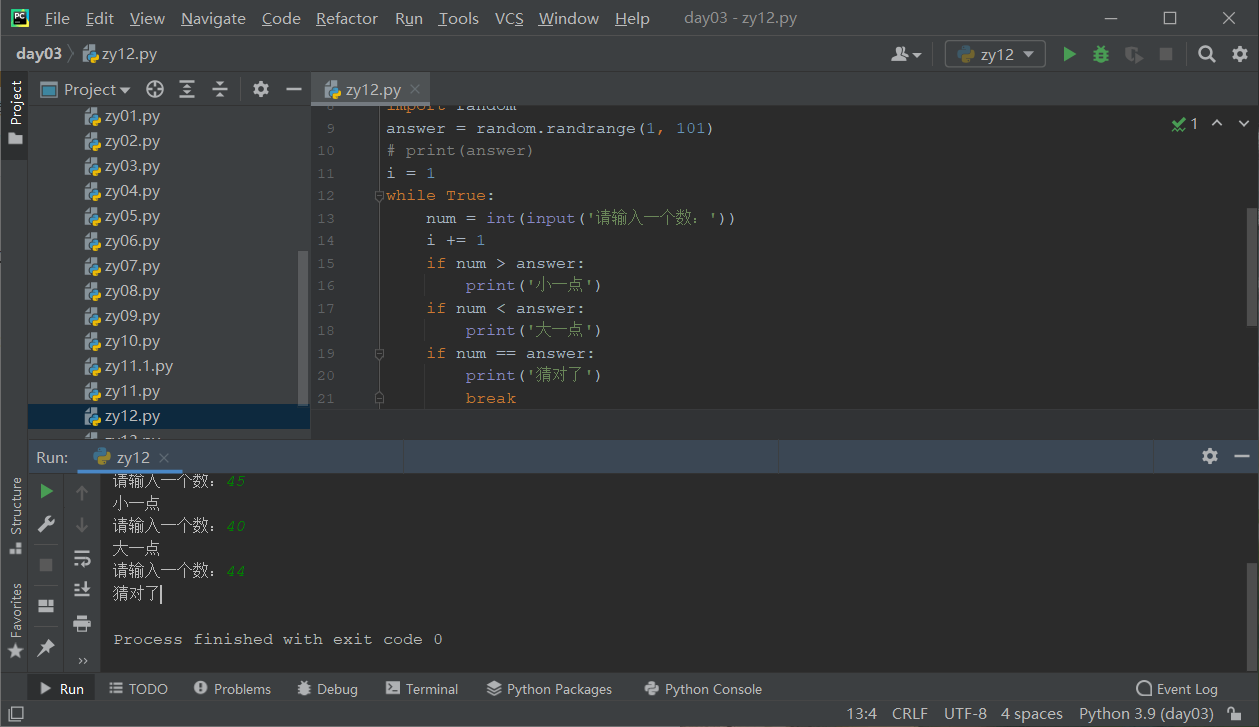
Exercise 13:
Enter 10 integers 1-99, calculate the average value, and find the maximum and minimum values
total, counter= 0, 0 max_value, min_value = 0, 100 while counter < 10: temp = int(input('Please enter:')) if temp < 1 or temp >= 100: print('invalid input') continue counter += 1 total += temp if temp > max_value: max_value = temp if min_value > temp: min_value = temp print(f'Average:{total / 10}') print(f'max:{max_value}') print(f'min:{min_value}')
continue - > it can only be used in a loop, allowing the loop to go directly to the next round, ignoring the subsequent codes of the current round
