Global exception handling of SpringBoot (full version)
Posted by Mordred on Fri, 25 Oct 2019 22:01:07 +0200
1. Project structure
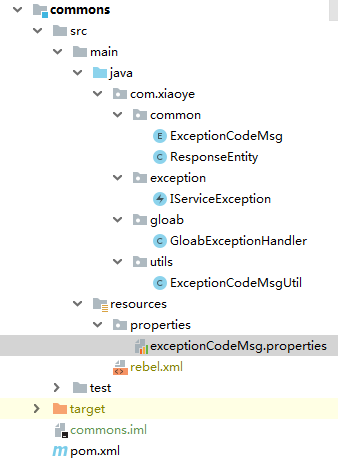
2. exceptionCodeMsg.properties configuration file
-1 = system exception, please try again later!
0=OK
200=SUCCESS
404 = request not found, please check whether the request address is correct
500 = server exception
3. ExceptionCodeMsgUtil configuration file tool class
package com.xiaoye.utils;
import java.io.IOException;
import java.util.Properties;
public class ExceptionCodeMsgUtil {
private static Properties prop = null;
static {
prop = new Properties();
try {
prop.load(ExceptionCodeMsgUtil.class.getClassLoader().getResourceAsStream("properties/exceptionCodeMsg.properties"));
} catch (IOException e) {
e.printStackTrace();
}
}
public static String getValue(String key) {
return prop.getProperty(key);
}
}
4. ExceptionCodeMsg error code / information entity
package com.xiaoye.common;
import com.xiaoye.utils.ExceptionCodeMsgUtil;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.Getter;
@Getter
public enum ExceptionCodeMsg {
ERROR(-1),
OK(0),
SUCCESS(200),
ERROR_404(404),
ERROR_500(500);
private Integer code;
private String msg;
private ExceptionCodeMsg(Integer code) {
this.code = code;
}
public String getMsg() {
return ExceptionCodeMsgUtil.getValue(this.code + "");
}
}
5. ResponseEntity return entity
package com.xiaoye.common;
import com.xiaoye.exception.IServiceException;
import lombok.AllArgsConstructor;
import lombok.Data;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import java.io.Serializable;
import java.util.Date;
@Data
@AllArgsConstructor
public class ResponseEntity<T extends Serializable> implements Serializable {
private static final Logger LOGGER = LoggerFactory.getLogger(ResponseEntity.class);
private Integer code;
private String msg;
private T data;
private Boolean success;
private Long date;
private Object exception;
private ResponseEntity() {
date = new Date().getTime();
}
public static <T extends Serializable> ResponseEntity<T> IS_SUCCESS(T data) {
ResponseEntity<T> responseEntity = new ResponseEntity<>();
responseEntity.setCode(ExceptionCodeMsg.SUCCESS.getCode());
responseEntity.setMsg(ExceptionCodeMsg.SUCCESS.getMsg());
responseEntity.setSuccess(true);
responseEntity.setData(data);
return responseEntity;
}
public static <T extends Serializable> ResponseEntity<T> IS_ERROR(ExceptionCodeMsg exceptionCodeMsg, Exception exception) {
ResponseEntity<T> responseEntity = new ResponseEntity<>();
responseEntity.setCode(exceptionCodeMsg.getCode());
responseEntity.setMsg(exceptionCodeMsg.getMsg());
responseEntity.setSuccess(false);
responseEntity.setException(exception.getClass().getName());
return responseEntity;
}
public static <T extends Serializable> ResponseEntity<T> IS_ERROR(IServiceException iServiceException) {
ResponseEntity<T> responseEntity = new ResponseEntity<>();
responseEntity.setCode(iServiceException.getExceptionCodeMsg().getCode());
responseEntity.setMsg(iServiceException.getExceptionCodeMsg().getMsg());
responseEntity.setSuccess(false);
responseEntity.setException(iServiceException.getClass().getName());
return responseEntity;
}
}
6. IServiceException custom exception
package com.xiaoye.exception;
import com.xiaoye.common.ExceptionCodeMsg;
import lombok.Getter;
import lombok.ToString;
@Getter
@ToString
public class IServiceException extends Exception {
private ExceptionCodeMsg exceptionCodeMsg;
public IServiceException(ExceptionCodeMsg exceptionCodeMsg) {
this.exceptionCodeMsg = exceptionCodeMsg;
}
}
7. GloabExceptionHandler global exception interception
package com.xiaoye.gloab;
import com.xiaoye.common.ExceptionCodeMsg;
import com.xiaoye.common.ResponseEntity;
import com.xiaoye.exception.IServiceException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class GloabExceptionHandler {
private static final Logger LOGGER = LoggerFactory.getLogger(GloabExceptionHandler.class);
@ExceptionHandler(IServiceException.class)
public ResponseEntity handIServiceException(IServiceException exception) {
exception.printStackTrace();
return ResponseEntity.IS_ERROR(exception);
}
@ExceptionHandler(Exception.class)
public ResponseEntity handException(Exception exception) {
exception.printStackTrace();
return ResponseEntity.IS_ERROR(ExceptionCodeMsg.ERROR, exception);
}
private void printConsole(Exception exception) {
exception.printStackTrace();
}
}
Topics:
Lombok
Java