Catalog
3. judge whether the channel is blocked
Conditional statement if
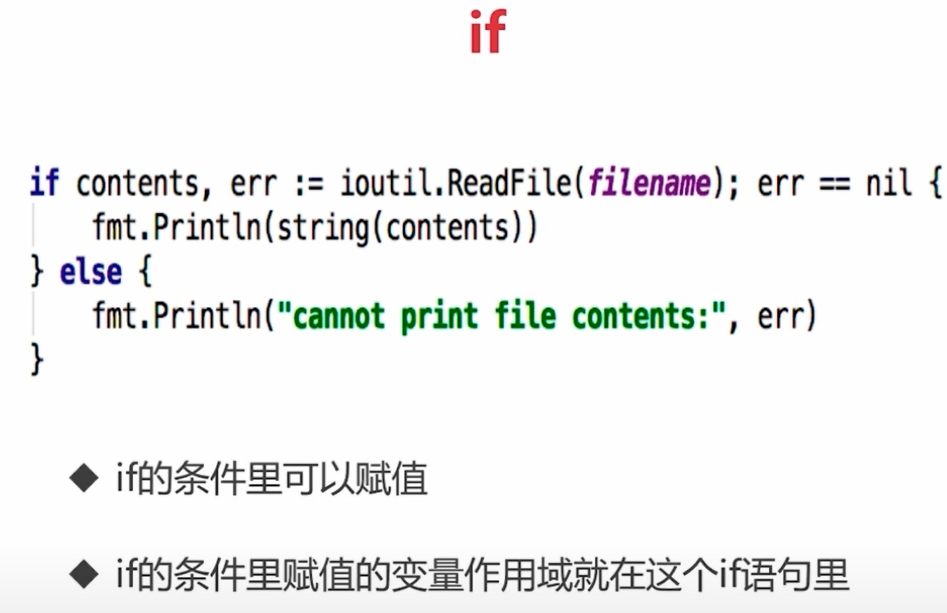
• conditional expression brackets may be omitted.
• with initialization statement, local variables of code block can be defined.
• the code block left parenthesis must be at the end of the conditional expression.
* ternary operator "a > b? A: B" is not supported.
Conditional statement switch
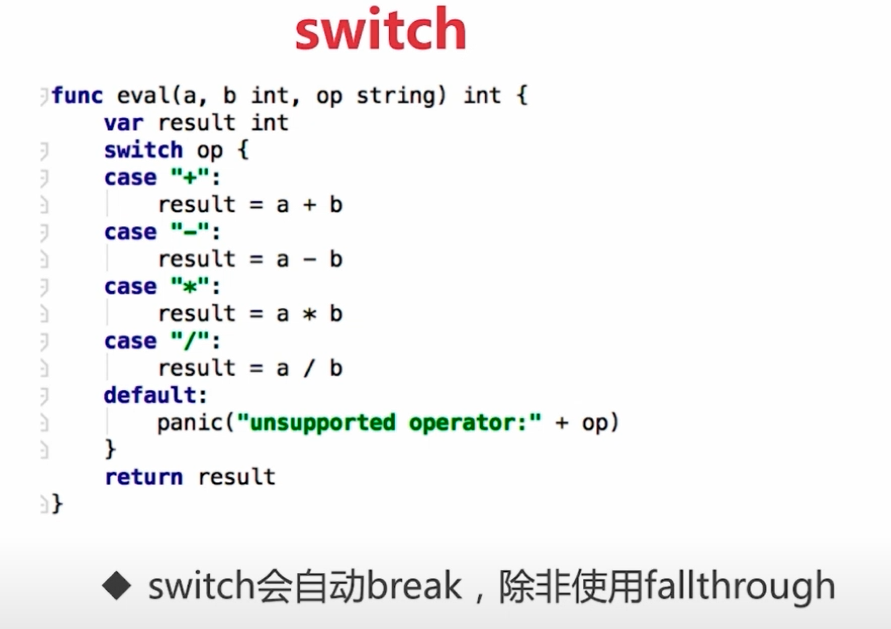
- The switch statement is used to perform different actions based on different conditions. Each case branch is unique. It is tested from top to bottom until it matches.
- The Golang switch branch expression can be of any type, not limited to constants. You can omit break, which is automatically terminated by default.
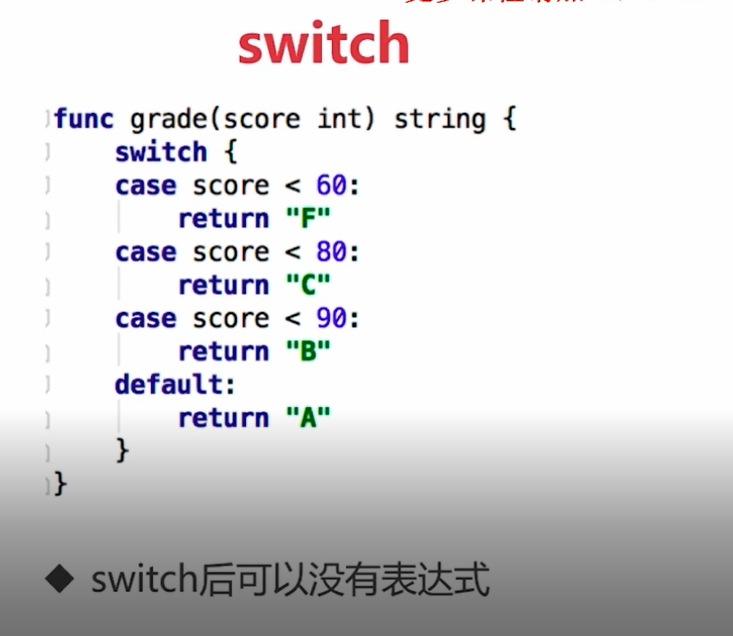
The type is not limited to constants or integers, but must be of the same type; or the final result must be an expression of the same type.
You can test multiple values that may meet the criteria at the same time, and use commas to separate them, for example: case val1, val2, val3.
switch marks { case 90: grade = "A" case 80: grade = "B" case 50,60,70 : grade = "C" default: grade = "D" }
Type Switch
Switch statements can also be used for type switch to determine the type of variables actually stored in an interface variable.
The Type Switch syntax format is as follows:
switch x.(type){ case type: statement(s) case type: statement(s) /* You can define any number of case s */ default: /* Optional */ statement(s) }
Example:
package main import "fmt" func main() { var x interface{} //Writing one: switch i := x.(type) { // With initialization statement case nil: fmt.Printf(" x Types :%T\r\n", i) case int: fmt.Printf("x yes int type") case float64: fmt.Printf("x yes float64 type") case func(int) float64: fmt.Printf("x yes func(int) type") case bool, string: fmt.Printf("x yes bool or string type") default: fmt.Printf("Unknown type") } //Writing two var j = 0 switch j { case 0: case 1: fmt.Println("1") case 2: fmt.Println("2") default: fmt.Println("def") } //Writing three var k = 0 switch k { case 0: println("fallthrough") fallthrough /* Go The switch is very flexible. The expression does not have to be a constant or an integer. The process is from top to bottom until a match is found; If a switch has no expression, it matches true. Go In it, switch is equivalent to each case with break at the end, After the match is successful, it will not automatically execute other case s down, but jump out of the whole switch, But you can use fallthrough to enforce the following case code. */ case 1: fmt.Println("1") case 2: fmt.Println("2") default: fmt.Println("def") } //Writing three var m = 0 switch m { case 0, 1: fmt.Println("1") case 2: fmt.Println("2") default: fmt.Println("def") } //Writing four var n = 0 switch { //Omit the conditional expression when if...else if...else case n > 0 && n < 10: fmt.Println("i > 0 and i < 10") case n > 10 && n < 20: fmt.Println("i > 10 and i < 20") default: fmt.Println("def") } }
The above code execution result is:
x Types :<nil> fallthrough 1 1 def
Conditional statement select
A select statement is similar to a switch statement, but select randomly executes a runnable case.
If there is no case to run, it will block until there is a case to run.
Syntax of select statement:
select { case communication clause : statement(s); case communication clause : statement(s); /* You can define any number of case s */ default : /* Optional */ statement(s); }
The syntax of the select statement is described below:
- Each case must be a communication
- All channel expressions are evaluated
- All sent expressions are evaluated
- If any communication can be made, it is executed; the others are ignored.
- If more than one case can run, Select randomly and fairly selects an execution. Others will not be executed.
- Otherwise:
- If there is a default clause, the statement is executed.
- If there is no default, select will block until a communication can run; Go will not re evaluate the channel or value.
Typical use of select
1. Timeout judgment
//For example, in the following scenario, global respan is used to accept the response. If the time exceeds 3S and there is no data returned in respan, the second case will execute var resChan = make(chan int) // do request func test() { select { case data := <-resChan: doData(data) case <-time.After(time.Second * 3): fmt.Println("request time out") } } func doData(data int) { //... }
2. exit
//In the main thread (cooperation process), it is as follows: var shouldQuit=make(chan struct{}) fun main(){ { //loop } //...out of the loop select { case <-c.shouldQuit: cleanUp() return default: } //... } //In another cooperation process, if the operation encounters illegal operation or unhandled error, the data notification program is sent to shouldQuit to stop operation close(shouldQuit)
3. Judge whether the channel is blocked
//In some cases, there is a requirement that you do not want the channel cache to be full ch := make (chan int, 5) //... data: =0 select { case ch <- data: default: //Do something like discard data. Depending on demand }
Loop statement for
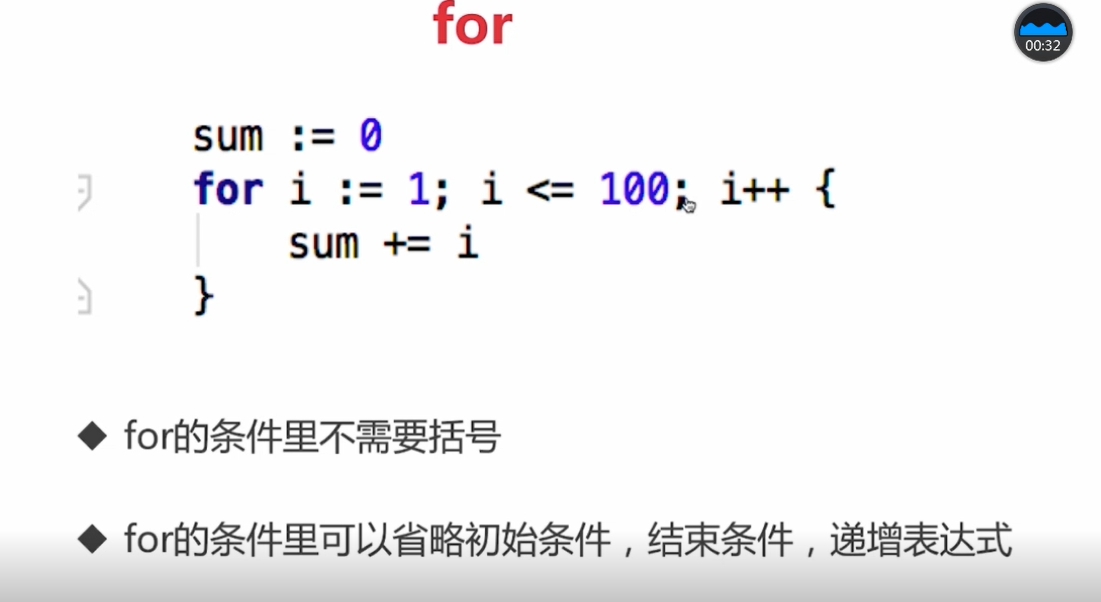
The For loop of the Go language has three forms, only one of which uses semicolons.
- for init; condition; post { }
- for condition { }
- for { }
init: Generally, it is an assignment expression to assign initial value to the control variable;
condition: Relation expression or logic expression, cycle control condition;
post: Generally, it is an assignment expression, which increments or decrements the control variable.
- for The statement is executed as follows:
- ①Expression first init Initial value;
- ②Discriminant assignment expression init Whether the given condition Condition, if the value is true and the loop condition is met, execute the statement in the loop body, and then execute post,Enter the second cycle, and judge again condition;Otherwise judgment condition If the condition is not met, it will terminate for Loop, execute out of loop statements.
s := "abc" for i, n := 0, len(s); i < n; i++ { // The common for loop supports initialization statements. println(s[i]) } n := len(s) for n > 0 { // Replace while (n > 0) {} println(s[n]) // Replace for (; n > 0;) {} n-- } for { // Replace while (true) {} println(s) // Replace for (;;) {} }
Don't expect the compiler to understand you. It's a good idea to calculate all the results in the initialization statement.
package main func length(s string) int { println("call length.") return len(s) } func main() { s := "abcd" for i, n := 0, length(s); i < n; i++ { // Avoid calling the length function multiple times. println(i, s[i]) } }
Output:
call length. 0 97 1 98 2 99 3 100
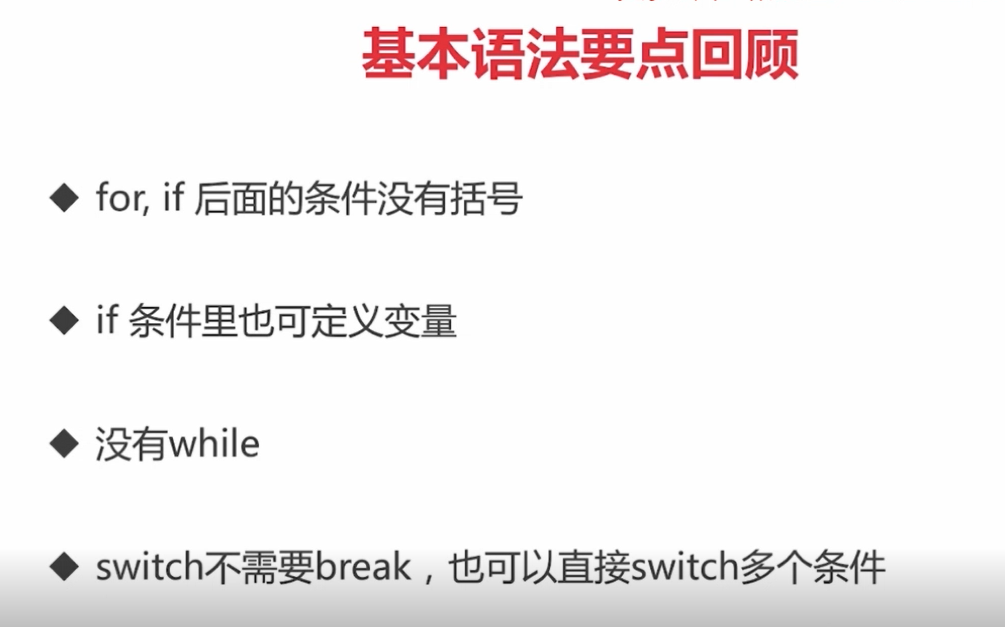
Reference link: