catalogue
variable
The Go language variable name consists of letters, numbers and underscores. The first character cannot be a number. Use the var keyword.
statement
var variable name 1 [, variable name 2...] type
Note: one or more variables can be declared, and the declared value is zero
Zero value: 0, "", false, nil, etc
code
var num int var num1,num2 int
initialization
General form
var variable name 1 [, variable name 2...] [type] = value1[,value2...]
Note: after initialization, it must also be used later. The type can be determined automatically without writing
Code
var num3 int8 = 1
Type derivation
It doesn't write type on it
var variable name 1 [, variable name 2...]= value1[,value2...]
Note: integer defaults to int and floating point defaults to float64
Code
var num4 = 2
Omit var
Variable name 1 [, variable name 2...]:= value1[,value2...]
I personally recommend this, similar to Python, without writing variable types.
Code
f1 := 5.0
There is another form, which is often used for global variable declarations
var (
Variable 1 = value1
Variable 2 = value2
)
code
var ( author = "lady_killer9" time = "now" )
use
Output, assignment and calculation are OK, and the exchange is simple
num1 = 1 num2 = 2 num1,num2 = num2,num1
Anonymous variable
_, Underline
This receiver can be used when the data is no longer used
all,_ := sumDiffer(num1,num2)
Scope
Note: variable names cannot be the same in the same scope
global variable
Function, lowercase package, uppercase cross package
Note: the form of omitting var cannot be used
//Global: = 1 error: Global unexpected var Global = 1
local variable
In function, in for/if
code
func sumDiffer(a int,b int) (int,int) { return a+b,a-b }
fmt.Println("author time:",author,time) //fmt.Println(a,b) a,b are local variables in sumdifference function and cannot be accessed
constant
A constant is an identifier of a simple value that will not be modified when the program runs.
The data types in constants can only be Boolean, numeric (integer, floating point and complex) and string.
Use the keyword const
const variable name [type] = value
const Pi = 3.1415926
All codes
package main import "fmt" var ( author = "lady_killer9" time = "now" ) //Global: = 1 error: Global unexpected var Global = 1 func sumDiffer(a int,b int) (int,int) { return a+b,a-b } func main() { //-------------Statement-------------- // Declare a single variable, num = 0 var num int // Declare multiple variables, num1 and num2 are 0 var num1,num2 int fmt.Println("num:",num) fmt.Println("num1 num2:",num1,num2) //-----------Initialize--------------- // Common form var num3 int8 = 1 //Type derivation var num4 = 2 // Omit var f1 := 5.0 fmt.Printf("num3:%v,num4 Type of:%T,num4:%v,f1 Type of:%T,f1:%v\n",num3,num4,num4,f1,f1) //-----------Variable use----------- num1 = 1 num2 = 2 num1,num2 = num2,num1 fmt.Println("num1 num2:",num1,num2) //------------Anonymous variable---------- all,_ := sumDiffer(num1,num2) fmt.Println("num1+num2:",all) //-----------Scope---------- fmt.Println("author time:",author,time) fmt.Println("Global:",Global) //fmt.Println(a,b) a,b are local variables in sumdifference function and cannot be accessed //-----------Constant------------ const Pi = 3.1415926 fmt.Println("Pi:",Pi) }
screenshot
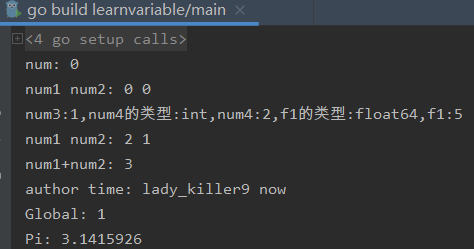
reference resources
More Go related content: ***
If you have any questions, please comment below. Please indicate the source and attach the link to the original text. Thank you! In case of infringement, please contact us in time. If you feel rewarded and volunteer to reward, you can choose Alipay 18833895206 (less than). Your support is my constant updating power.