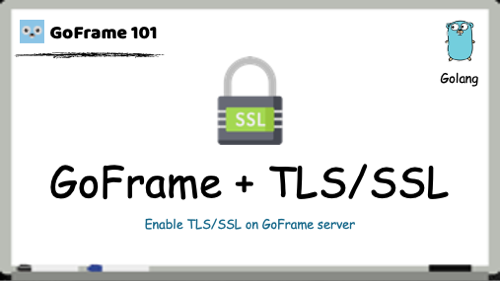
introduce
Through a complete example, in gogf/gf Open TLS/SSL in the framework. I'm what we often call https.
We will use rk-boot To start gogf/gf Microservices.
Please visit the following address for a complete tutorial:
Generate self signed certificate
Users can purchase certificates from major cloud manufacturers or use cfssl Create a custom certificate.
We describe how to generate a certificate locally.
1. Download cfssl & cfssljson command line
It is recommended to use the rk command line to download.
$ go get -u github.com/rookie-ninja/rk/cmd/rk $ rk install cfssl $ rk install cfssljson
Download from the official website
$ go get github.com/cloudflare/cfssl/cmd/cfssl $ go get github.com/cloudflare/cfssl/cmd/cfssljson
2. Generate CA
$ cfssl print-defaults config > ca-config.json $ cfssl print-defaults csr > ca-csr.json
Modify CA config. As needed JSON and Ca CSR json.
$ cfssl gencert -initca ca-csr.json | cfssljson -bare ca -
3. Generate server certificate
server.csr,server.pem and server key PEM will be generated.
$ cfssl gencert -config ca-config.json -ca ca.pem -ca-key ca-key.pem -profile www ca-csr.json | cfssljson -bare server
install
go get github.com/rookie-ninja/rk-boot/gf
Quick start
rk-boot The following methods are supported: gogf/gf Get certificate from service.
- Local file system
- Remote file system
- Consul
- ETCD
Let's first look at how to get a certificate locally and start.
1. Create boot yaml
In this example, we only start the server certificate. locale is used to distinguish cert in different environments.
Please refer to the previous article for details:
--- cert: - name: "local-cert" # Required provider: "localFs" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS gf: - name: greeter port: 8080 enabled: true enableReflection: true cert: ref: "local-cert" # Enable grpc TLS
2. Create main go
// Copyright (c) 2021 rookie-ninja // // Use of this source code is governed by an Apache-style // license that can be found in the LICENSE file. package main import ( "context" "github.com/gogf/gf/v2/net/ghttp" "github.com/rookie-ninja/rk-boot" "github.com/rookie-ninja/rk-boot/gf" "net/http" ) // @title Swagger Example API // @version 1.0 // @description This is a sample rk-demo server. // @termsOfService http://swagger.io/terms/ // @securityDefinitions.basic BasicAuth // @contact.name API Support // @contact.url http://www.swagger.io/support // @contact.email support@swagger.io // @license.name Apache 2.0 // @license.url http://www.apache.org/licenses/LICENSE-2.0.html func main() { // Create a new boot instance. boot := rkboot.NewBoot() // Register handler entry := rkbootgf.GetGfEntry("greeter") entry.Server.BindHandler("/v1/hello", hello) // Bootstrap boot.Bootstrap(context.TODO()) boot.WaitForShutdownSig(context.TODO()) } // @Summary Hello // @Id 1 // @Tags Hello // @version 1.0 // @produce application/json // @Success 200 string string // @Router /v1/hello [get] func hello(ctx *ghttp.Request) { ctx.Response.WriteHeader(http.StatusOK) ctx.Response.WriteJson(map[string]string{ "message": "hello!", }) }
3. Folder structure
. ├── boot.yaml ├── cert │ ├── server-key.pem │ └── server.pem ├── go.mod ├── go.sum └── main.go 1 directory, 6 files
4. Start main go
$ go run main.go
5. Verification
$ curl -X GET --insecure https://localhost:8080/v1/hello {"message":"hello!"}
framework
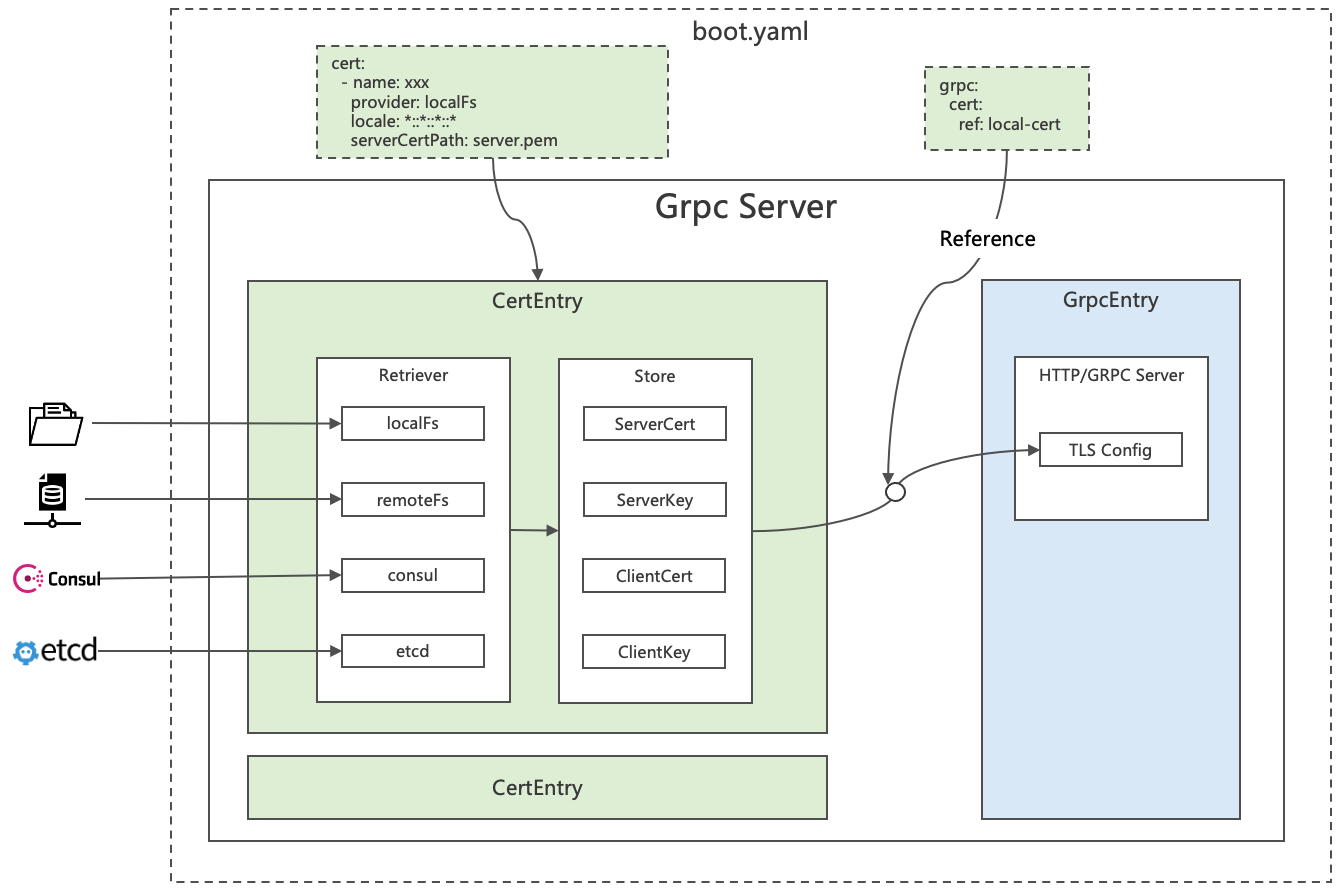
Parameter introduction
1. Read the certificate locally
Configuration item | details | need | Default value |
---|---|---|---|
cert.localFs.name | Local file system getter name | yes | "" |
cert.localFs.locale | Follow locale: \ < realm >:: \ < region >: \ < AZ >: \ < domain > | yes | "" |
cert.localFs.serverCertPath | Server certificate path | no | "" |
cert.localFs.serverKeyPath | Server certificate key path | no | "" |
cert.localFs.clientCertPath | Client certificate path | no | "" |
cert.localFs.clientCertPath | Client certificate key path | no | "" |
- example
--- cert: - name: "local-cert" # Required description: "Description of entry" # Optional provider: "localFs" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS gf: - name: greeter port: 8080 enabled: true enableReflection: true cert: ref: "local-cert" # Enable grpc TLS
2. Read the certificate from the remote file service
Configuration item | details | need | Default value |
---|---|---|---|
cert.remoteFs.name | Remote file service getter name | yes | "" |
cert.remoteFs.locale | Follow locale: \ < realm >:: \ < region >: \ < AZ >: \ < domain > | yes | "" |
cert.remoteFs.endpoint | Remote address: http://x.x.x.x Or x.x.x.x | yes | N/A |
cert.remoteFs.basicAuth | Basic auth: <user:pass>. | no | "" |
cert.remoteFs.serverCertPath | Server certificate path | no | "" |
cert.remoteFs.serverKeyPath | Server certificate key path | no | "" |
cert.remoteFs.clientCertPath | Client certificate path | no | "" |
cert.remoteFs.clientCertPath | Client certificate key path | no | "" |
- example
--- cert: - name: "remote-cert" # Required description: "Description of entry" # Optional provider: "remoteFs" # Required, etcd, consul, localFs, remoteFs are supported options endpoint: "localhost:8081" # Required, both http://x.x.x.x or x.x.x.x are acceptable locale: "*::*::*::*" # Required, default: "" serverCertPath: "cert/server.pem" # Optional, default: "", path of certificate on local FS serverKeyPath: "cert/server-key.pem" # Optional, default: "", path of certificate on local FS gf: - name: greeter port: 8080 enabled: true cert: ref: "remote-cert" # Enable grpc TLS
3. Read the certificate from Consul
Configuration item | details | need | Default value |
---|---|---|---|
cert.consul.name | Consul getter name | yes | "" |
cert.consul.locale | Follow locale: \ < realm >:: \ < region >: \ < AZ >: \ < domain > | yes | "" |
cert.consul.endpoint | Consul address: http://x.x.x.x or x.x.x.x | yes | N/A |
cert.consul.datacenter | Consul data center | yes | "" |
cert.consul.token | Consul access key | no | "" |
cert.consul.basicAuth | Consult basic auth, format: < user: pass > | no | "" |
cert.consul.serverCertPath | Server certificate path | no | "" |
cert.consul.serverKeyPath | Server certificate key path | no | "" |
cert.consul.clientCertPath | Server certificate key path | no | "" |
cert.consul.clientCertPath | Server certificate key path | no | "" |
- example
--- cert: - name: "consul-cert" # Required provider: "consul" # Required, etcd, consul, localFS, remoteFs are supported options description: "Description of entry" # Optional locale: "*::*::*::*" # Required, "" endpoint: "localhost:8500" # Required, http://x.x.x.x or x.x.x.x both acceptable. datacenter: "dc1" # Optional, default: "", consul datacenter serverCertPath: "server.pem" # Optional, default: "", key of value in consul serverKeyPath: "server-key.pem" # Optional, default: "", key of value in consul gf: - name: greeter port: 8080 enabled: true cert: ref: "consul-cert" # Enable grpc TLS
4. Read the certificate from ETCD
Configuration item | details | need | Default value |
---|---|---|---|
cert.etcd.name | ETCD collector name | yes | "" |
cert.etcd.locale | Follow locale: \ < realm >:: \ < region >: \ < AZ >: \ < domain > | yes | "" |
cert.etcd.endpoint | ETCD address: http://x.x.x.x or x.x.x.x | yes | N/A |
cert.etcd.basicAuth | ETCD basic auth, format: < user: pass > | no | "" |
cert.etcd.serverCertPath | Server certificate path | no | "" |
cert.etcd.serverKeyPath | Server certificate path | no | "" |
cert.etcd.clientCertPath | Client certificate path | no | "" |
cert.etcd.clientCertPath | Client certificate key path | no | "" |
- example
--- cert: - name: "etcd-cert" # Required description: "Description of entry" # Optional provider: "etcd" # Required, etcd, consul, localFs, remoteFs are supported options locale: "*::*::*::*" # Required, default: "" endpoint: "localhost:2379" # Required, http://x.x.x.x or x.x.x.x both acceptable. serverCertPath: "server.pem" # Optional, default: "", key of value in etcd serverKeyPath: "server-key.pem" # Optional, default: "", key of value in etcd gf: - name: greeter port: 8080 enabled: true cert: ref: "etcd-cert" # Enable grpc TLS