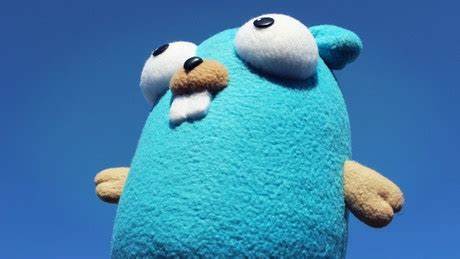
Beginners tend to ignore exception handling in the program, thinking that the program is always running in a cared-for environment, without any wind or rain, because we are the most knowledgeable people of our program. So we know what he likes, what he can eat and what he can't eat. But one day he returned to nature. This is the basic condition for him to survive in the fiercely competitive market to adapt to the external environment. This ability to adapt to harsh environments is his ability to deal with exceptions or errors.
The standard error handling model and error interface are introduced into go language. As long as the data type of the error interface is implemented, we can consider it an error type.
type error interface { Error() string }
Prove the above conclusion by code, define the errorString structure, and then let it implement the Error method. As long as we implement the method of interface, we think that this structure implements the error interface. This is the ingenuity of go language interface design.
type errorString struct{ s string } type (e *errorString) Error() string{ return e.string }
Previous exposure to IO or network operation functions will return an error in multiple return values, the error type is usually located in the last return value. Although this is just a habit, I hope you will follow the custom in designing the function. Good specifications lead to elegant code.
type Tut struct { Title string Courses int }
var db map[string]Tut func addTut(t Tut) error{ if t.Courses == 0 { return // If Only title does not have the number of courses, we throw a mistake. } //If the added course already exists in db, we throw item duplication // Preserving data db[t.Title] = t return nil }
We are adding the course addTut method to return an error, which happens as follows
- Zero Course Number for Additional Courses
- The course record already exists in the database.
Below is a concrete example, you can try it on your own.
ype Tut struct { Title string Courses int } var db map[string]Tut func addTut(t Tut) error{ if t.Courses == 0 { return errors.New("Courses should not be 0 ") } _, ok := db[t.Title] if !ok { return errors.New("Duplicate record") } db[t.Title] = t return nil } func main() { db = make(map[string]Tut) angularTut := Tut{ Title:"angular", Courses: 0} err := addTut(angularTut) if err != nil{ fmt.Println("error handling: " + err.Error()) } //Handling errors angularTwoTut := Tut{ Title:"angular", Courses: 0} err3 := addTut(angularTwoTut) if err3 != nil{ } angularDuplicated := Tut{Title:"angular",Courses:10} err2 := addTut(angularDuplicated) if err2 != nil{ fmt.Println("error handling" + err2.Error()) } }
Error Handling: Course cannot be 0 Error handling duplicate records
In addTut, we judge the two situations we thought were wrong before. If we think it was wrong, we throw Error, and we understand the cause of the error by defining the compiler of different output content.
var ( ErrNoErrors = errors.New("Number of courses is 0 ") ErrDuplicateEntry = errors.New("Duplicate record") )
func addTut(t Tut) error{ if t.Courses < 1 { return ErrNoErrors } _, ok := db[t.Title] if !ok { return ErrDuplicateEntry } db[t.Title] = t return nil }
We can refactor the code to optimize the code, and judge the error according to the type of error defined before to output.
func addTut(t Tut) error{ if t.Courses < 1 { return ErrNoErrors } _, ok := db[t.Title] if !ok { return ErrDuplicateEntry } db[t.Title] = t return nil } func main() { db = make(map[string]Tut) angularTut := Tut{ Title:"angular", Courses: 0} err := addTut(angularTut) switch err { case ErrNoErrors: fmt.Println("error handling: The number of courses should not be zero ") case ErrDuplicateEntry: fmt.Println("error handling: The course already exists and cannot be added repeatedly.") } //Handling errors angularTwoTut := Tut{ Title:"angular", Courses: 0} err3 := addTut(angularTwoTut) if err3 != nil{ } angularDuplicated := Tut{Title:"angular",Courses:10} err2 := addTut(angularDuplicated) switch err2 { case ErrNoErrors: fmt.Println("error handling: The number of courses should not be zero ") case ErrDuplicateEntry: fmt.Println("error handling: The course already exists and cannot be added repeatedly.") } }
import( "fmt" "errors" ) func main() { const fn = "temp.cpp" var ln = 19 text := fmt.Sprintf("compile problem with %q: %d",fn,ln) err := errors.New(text) if err != nil{ fmt.Println(err) } }
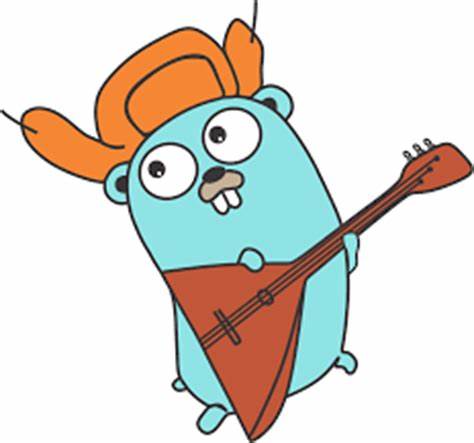