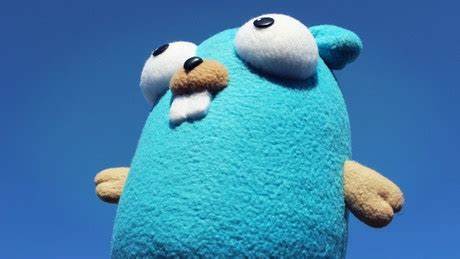
Json processing
Now most of the data we get from the server is in json format, so the data processing of json format is very important in web application development. I think you can't be more familiar with json. To put it simply, json is a lightweight data exchange language. Although json is a subset of javascript, it is language independent text format.
{ "data":[ { "title":"angular base tut", "author":"zidea" } ] }
Define the structure Tut and TutReponse corresponding to the above json data to write one letter less. json array corresponds to slice in go language.
Here, the fields of struct need to be capitalized. If there are more json fields than the struct, the fields that cannot be found will be ignored.
type Tut struct{ Title string Author string } type TutReponse struct{ Data []Tut }
package main import( "encoding/json" "fmt" ) type Tut struct{ Title string Author string } type TutReponse struct{ Data []Tut } func main() { var tuts TutReponse str := `{"data":[{"title":"angular base tut","author":"zidea"}]}` json.Unmarshal([]byte(str),&tuts) fmt.Println(tuts) }
json.Unmarshal([]byte(str),&tuts)
This is a function that parses json, accepts [] byte and represents any type of interface as parameters, so our json needs to be converted to [] byte for use.
func Unmarshal(data []byte, v interface{}) error
Because interface {} can represent any type of object, which is positive and resolves the unknown data structure of JSON. In the JSON package, map[string]interface {} and [] interface {} structures are used to store any JSON object and array
- bool stands for JSON boolean
b := []byte (`{"name":"zidea","age":30,"lang":["java","javascript","go","cpp","rust"]}`) var f interface{} err := json.Unmarshal(b, &f) if err != nil{ log.Fatal("json encoding:",err) } fmt.Println(f)
map[name:zidea age:30 lang:[java javascript go cpp rust]]
Use the previous knowledge about map and interface type inference to parse the json manually
Basis of golang (16) map
golang basic (20) interface
m := f.(map[string]interface{}) for k, v := range m{ switch vv := v.(type){ case string: fmt.Println(k, "is string", vv) case int: fmt.Println(k," is int ",vv) case []interface{}: fmt.Println(k," is an arry:") for i, u := range vv{ fmt.Println(i,u) } default: fmt.Println(k," is of a type ") } }
map[age:30 lang:[java javascript go cpp rust] name:zidea] name is string zidea age is of a type lang is an arry: 0 java 1 javascript 2 go 3 cpp 4 rust
Generate Json
package main import( "encoding/json" "fmt" ) type Tut struct{ Title string Author string } type TutResponse struct{ Data []Tut } func main() { var tutResponse TutResponse tutResponse.Data = append(tutResponse.Data, Tut{Title:"vuejs basic tut",Author:"zidea"}) b, err := json.Marshal(tutResponse) if err != nil { fmt.Println("json err:", err) } fmt.Println(string(b)) }
b, err := json.Marshal(tutResponse)
Enter a structure to return an array of bytes.
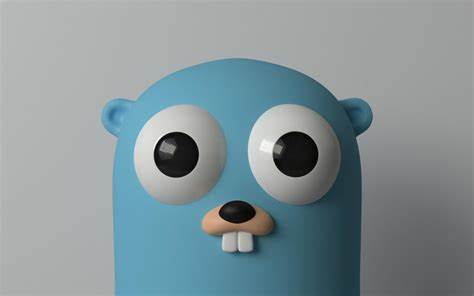