[TOC]
Gradle use
Gradle is a project automation construction tool based on the concepts of Apache Ant and Apache Maven. It uses a Groovy-based domain-specific language to declare project settings, rather than traditional XML. Wiki
- Automatically Processing Packet Dependency Relations - The Concept from Maven Repos
- Automated disposal of deployment problems - concepts from Ant
- Conditional Judgment Writing Intuition - Using Groovy Language
Note: In the use of Maven and Gradle, the problem is to introduce local jar packages, Gradle's one line of code is done, and Maven is quite troublesome, have to introduce one by one, did not find a way to introduce batch.
1. settings.gradle
Located in the project root directory to indicate which modules Gradle should include when building applications.
rootProject.name = 'java-toolkit' include 'toolkit-common' include 'toolkit-config' include 'toolkit-model'
2. build.gradle(parent)
In the project root directory, the configuration is applied to the whole project through all projects {}, and then to the whole sub-module through subprojects {}.
// General configuration for all projects allprojects { //Introducing idea Plug-in apply plugin: 'idea' //Specify project version number and group version = '2.0' group = 'gradle.multi' idea.module.iml { beforeMerged { module -> module.dependencies.clear() } whenMerged { module -> module.dependencies*.exported = true } } } // General configuration for all subprojects subprojects { //Introducing java plug-ins apply plugin: 'java' // JVM Version Number Requirements sourceCompatibility = 1.6 // When compiling java, the default state will fail because of Chinese characters, so we need to change it to utf8. [compileJava, compileTestJava]*.options*.encoding = 'UTF-8' repositories { //local repository(${user.home}/.m2/repository) mavenLocal() //Maven Central Library (http://repo1.maven.org/maven2) mavenCentral() } jar { manifest.attributes provider: 'gradle' } configurations { ... } dependencies { // Universal dependency compile( ... ) // Test dependence testCompile( ... ) } } //If the name in module contains web, add the following configure(subprojects.findAll {it.name.contains('web')}) { apply plugin: 'war' apply plugin: 'jetty' war { manifest { attributes("Implementation-Title": "Gradle") } } }
3. build.gradle(child module)
In the sub-module, the specific construction requirements are configurated separately.
// The name of jar or war archivesBaseName = 'module1' dependencies { compile ( ... ) }
4. gradle.properties
The configuration of some global parameters of Gradle or the variables used in the Gradle file can be customized, and the relevant values defined in the file can be accessed by using ${xxx} in the build script.
env=dev springVersion=4.3.16.RELEASE servletApiVersion = 3.1.0 jacksonVersion=2.8.6 junitVersion=4.12 log4jVersion=2.10.0 hibernateCoreVersion=5.2.1.Final hibernateEntityManagerVersion=5.2.1.Final hibernateValidatorVersion=5.2.4.Final hibernateJava8Version=5.2.1.Final
- Adding dependency packages
dependencies { // Batch introduction of local `jar'packages implementation fileTree(dir: 'libs', include: ['*.jar']) // Introduce locally specified `jar'packages implementation files('libs/foo.jar', 'libs/bar.jar') // Introducing remote packages implementation group: 'org.slf4j', name: 'slf4j-simple', version: '1.7.25' // Abbreviations are as follows //implementation 'org.slf4j:slf4j-simple:1.7.25' }
II. Multi-module Creation
Multi-module creation is a division of responsibilities for project code. Inheritance and aggregation are managed by gradle or maven between modules, which facilitates code decoupling and reuse, and module compilation and release.
1. First create an empty project:
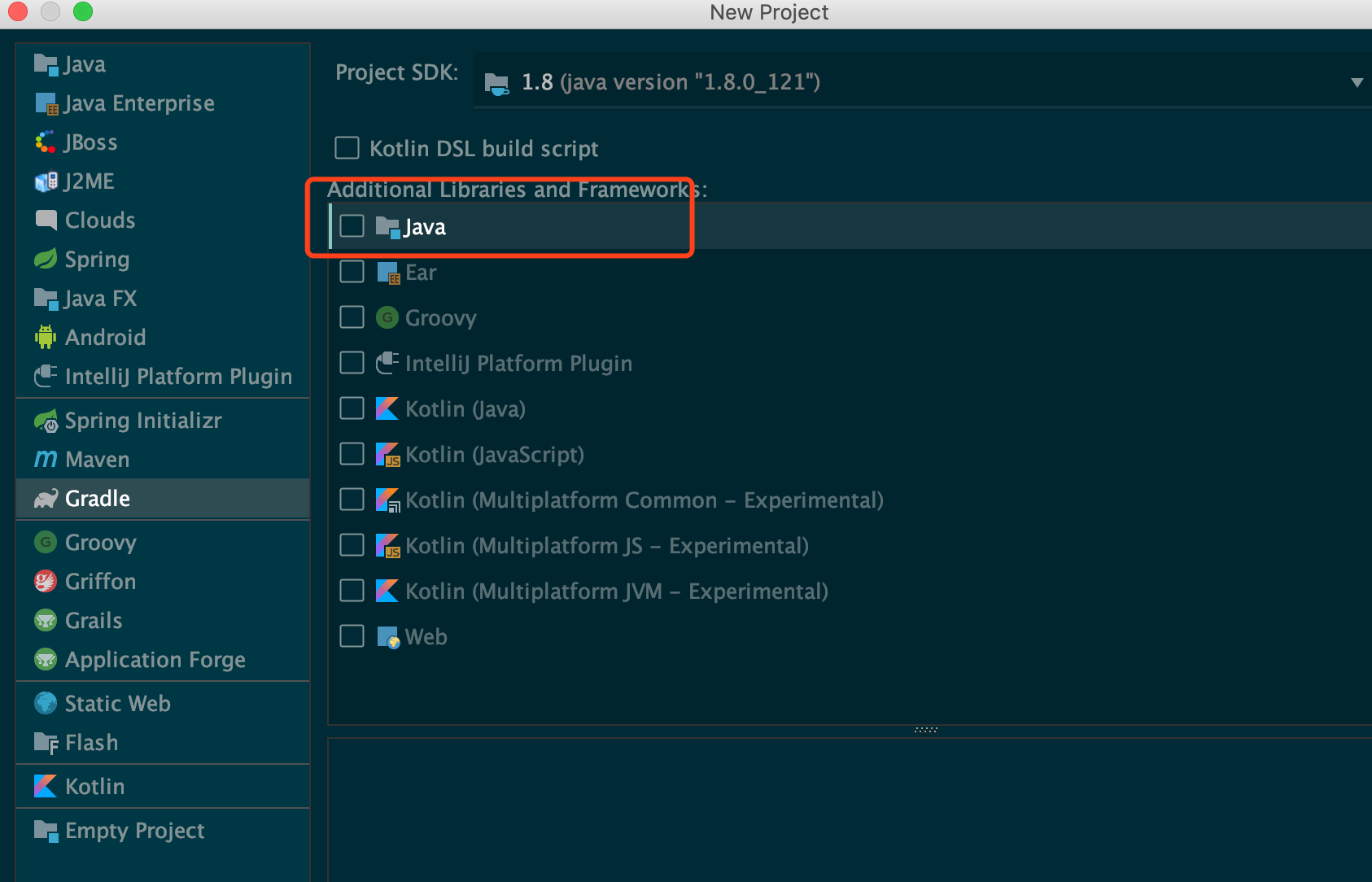
image.png
- GroupID Is the unique identifier of the project organization, which corresponds to the actual situation JAVA The structure of the package is main Directory java The first paragraph is domain, the second paragraph is company name, and the domain is divided into two parts. org,com,cn Wait a lot, among them org For non-profit organizations, com For business organizations. as
com.me.moduleName
.- ArtifactID Is the unique identifier of the project, the actual name of the corresponding project is the name of the project root directory.
Next, click "Next" by default all the way until it is finished, and get the following project structure:
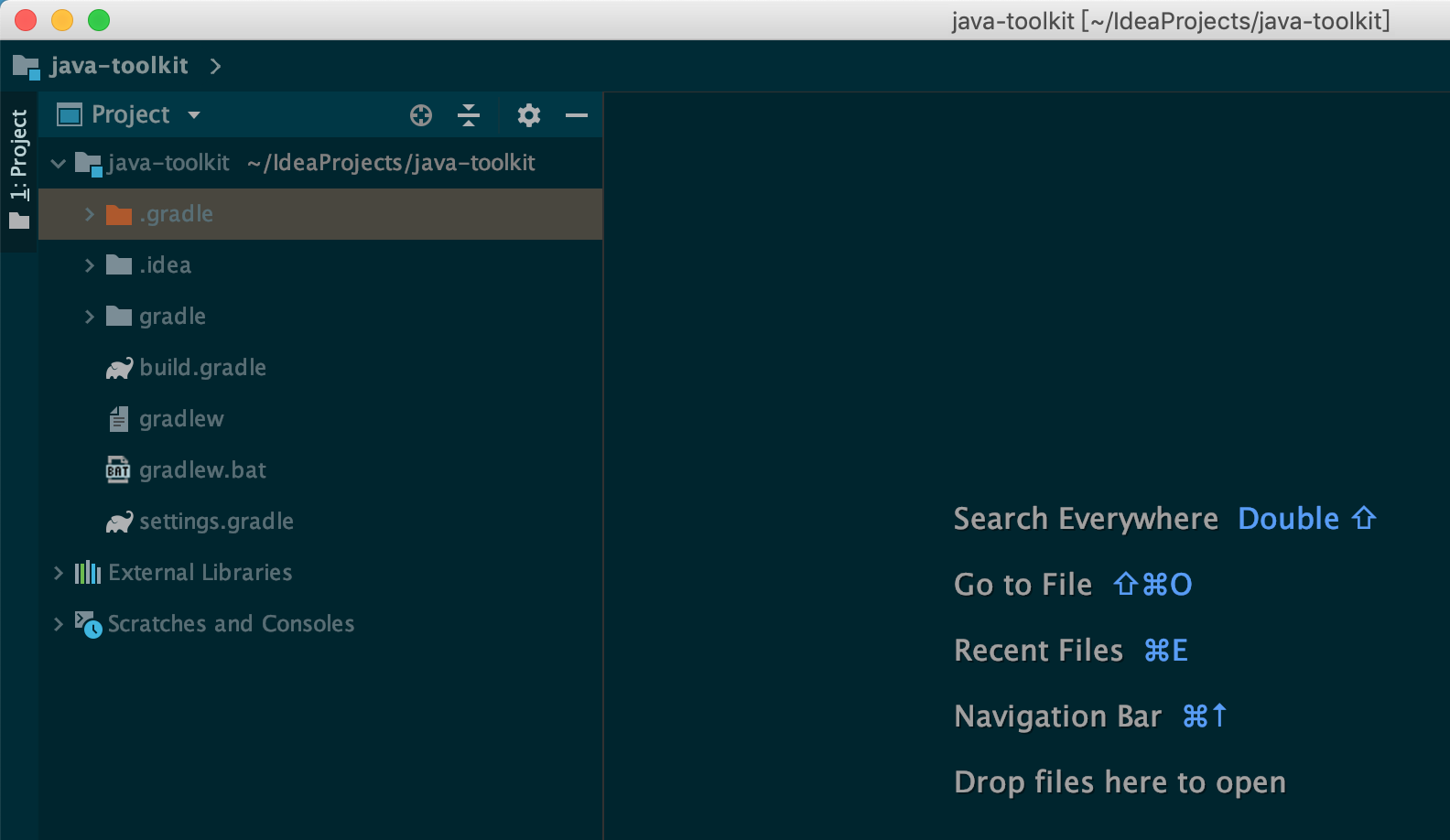
2. Create submodules
Follow these steps to create sub-modules toolkit-common, toolkit-config, toolkit-model in turn.
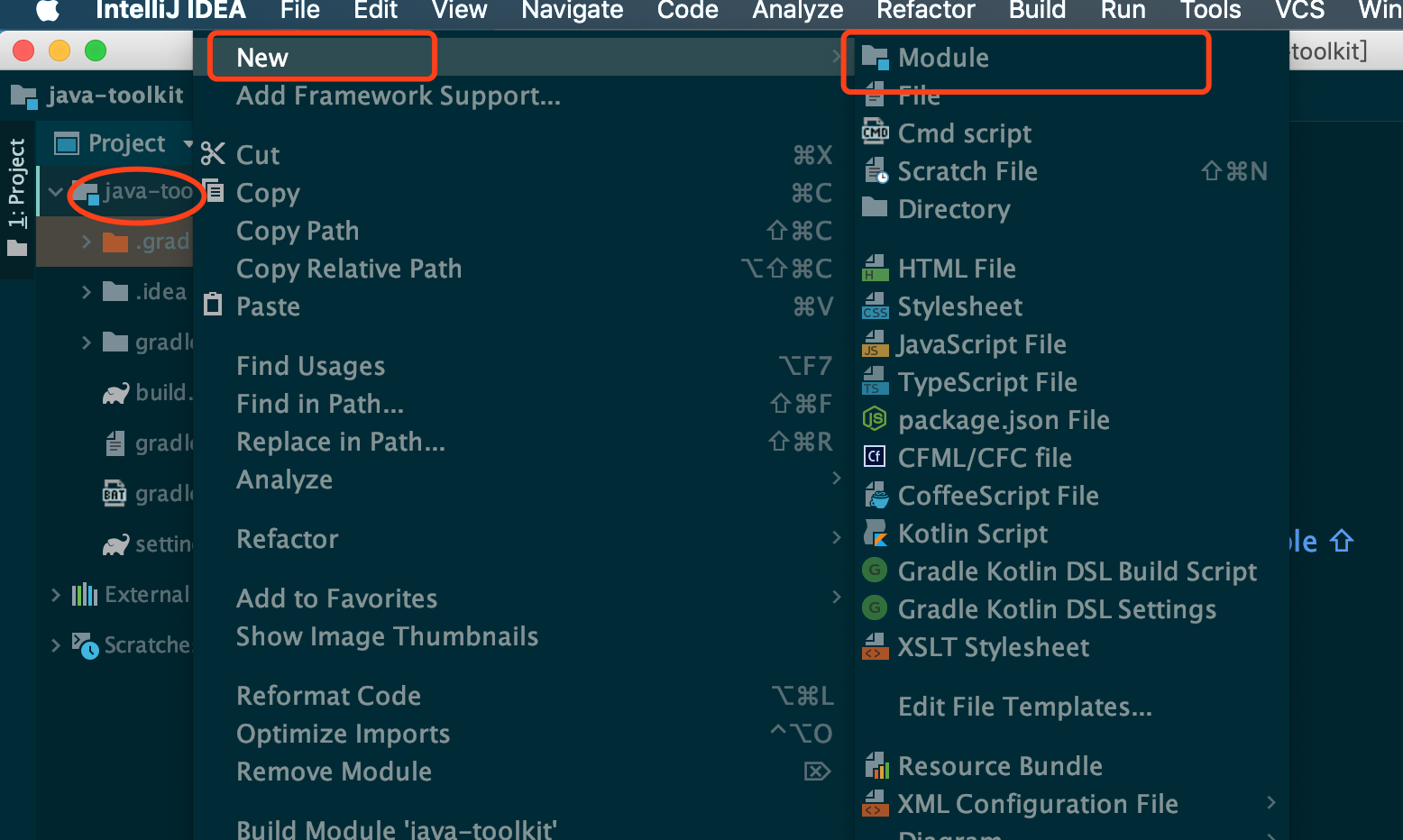
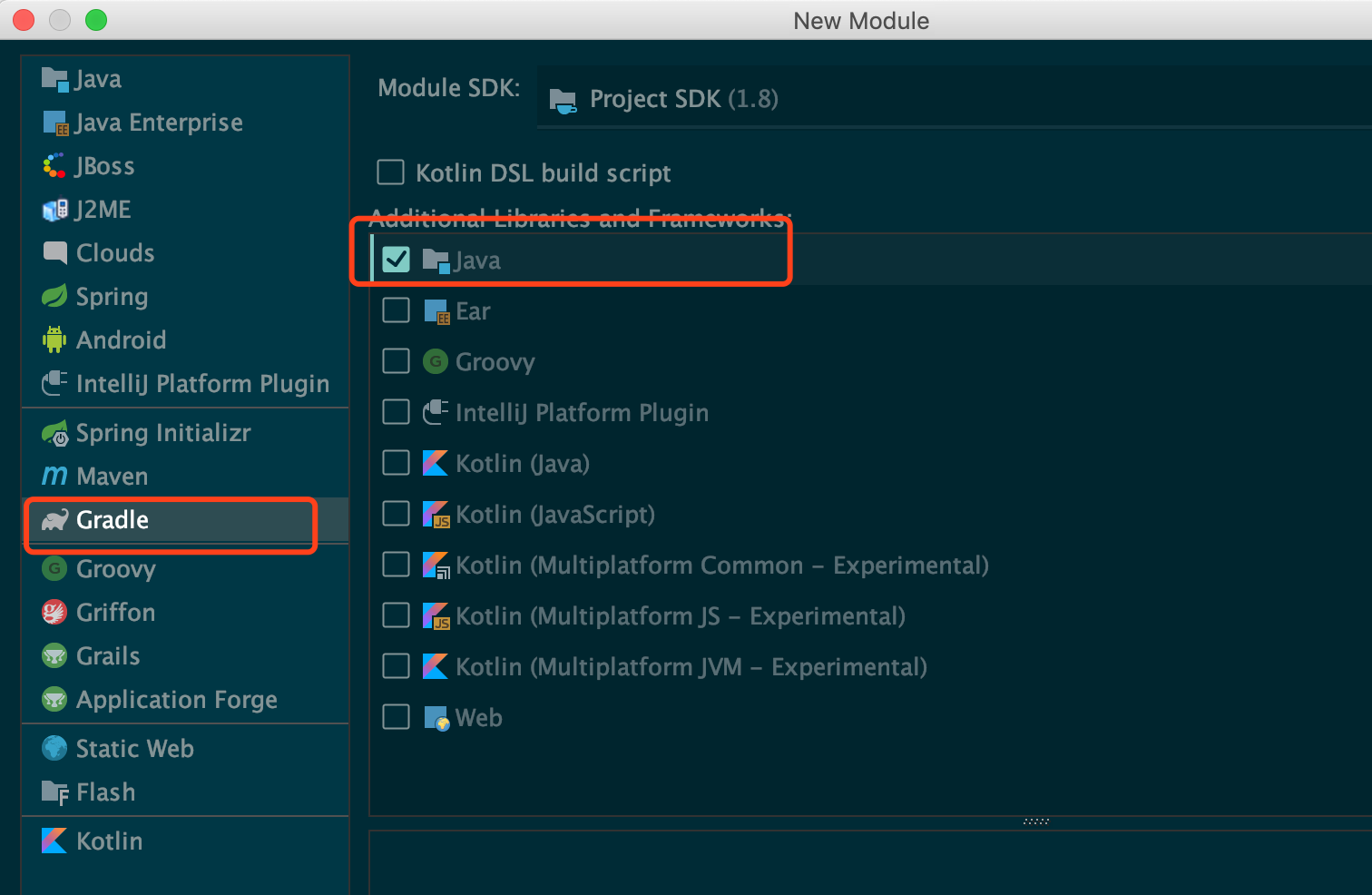
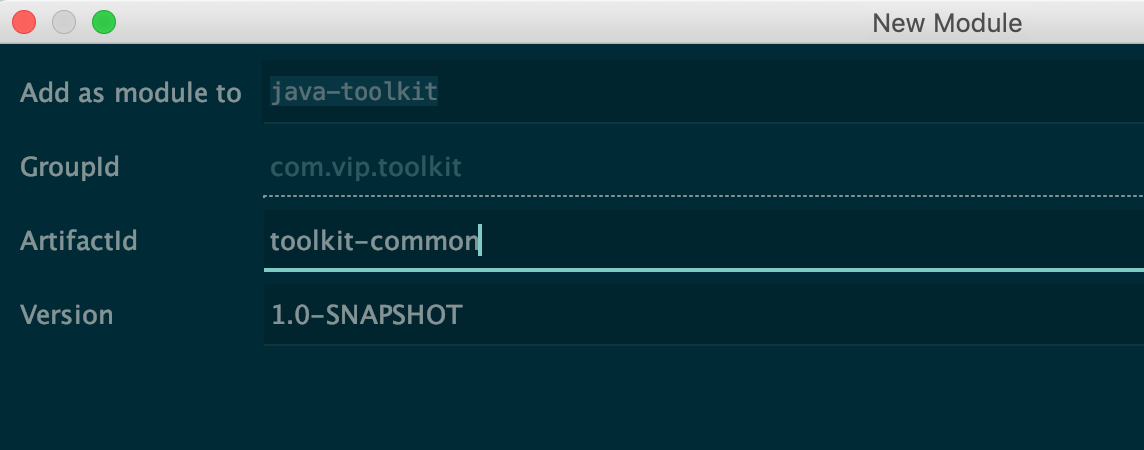
3. Final project structure
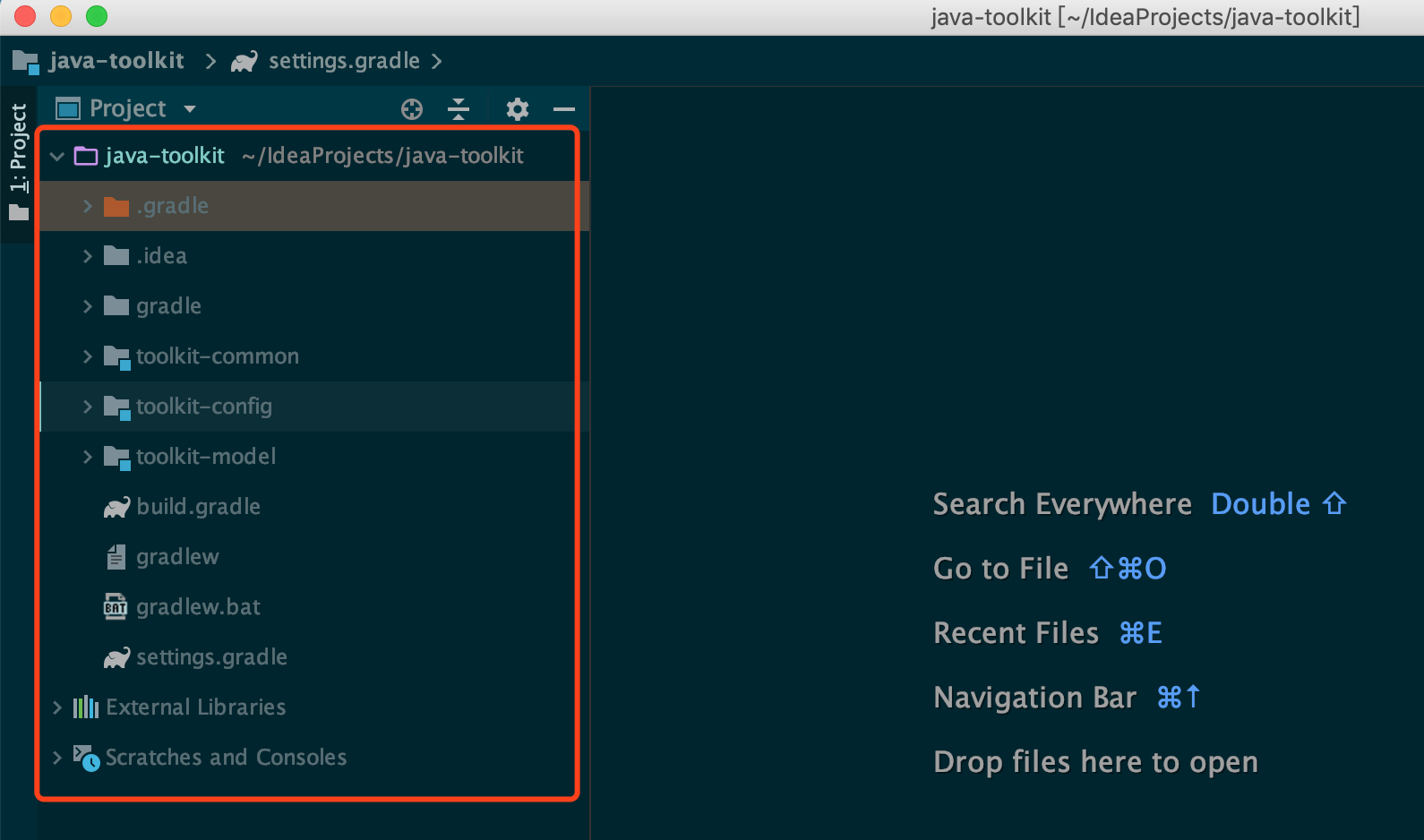
4. Question Records
Why did you write this documentation?
Because I didn't create an empty project when I first created it, when I checked java in the first step, the generated project would have an src structure, such as
java-toolkit - src - - main
In order to create the final effect of the project structure, I manually deleted the src of the parent directory, and then the problem arises. Every time a new sub-module is created, the src of the parent directory is automatically generated.
The solution is to modify the default configuration of build.gradle in the parent directory. The original data is as follows:
plugins { id 'java' } group 'com.vip.toolkit' version '1.0-SNAPSHOT' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { testCompile group: 'junit', name: 'junit', version: '4.12' }
Amend as follows:
allprojects { group 'com.vip.toolkit' version '1.0-SNAPSHOT' repositories { // local repository(${user.home}/.m2/repository) mavenLocal() // Maven Central Library (http://repo1.maven.org/maven2) mavenCentral() // Specify the location of the Maven Library maven { url "http://repo.maven.apache.org/maven2" } } } subprojects { // Introducing java plug-ins apply plugin: 'java' // Specify jvm version sourceCompatibility = 1.8 targetCompatibility = 1.8 // When compiling java, the default state will fail because of Chinese characters, so we need to change it to utf8. [compileJava, compileTestJava]*.options*.encoding = 'UTF-8' dependencies { testCompile group: 'junit', name: 'junit', version: '4.12' } }
Reference resources: https://michaelliuyang.github.io/projectsupport/2014/11/04/gradle-multi-project.html
https://developer.android.com/studio/build/dependencies