Tutorial address: http://www.showmeai.tech/tutorials/56
Article address: http://www.showmeai.tech/article-detail/88
Notice: All Rights Reserved. Please contact the platform and the author for reprint and indicate the source
1.Python object oriented
Object Oriented Programming, called Object Oriented Programming in English, is a programming idea. OOP regards object as the basic unit of program. An object contains data and functions that operate data.
Python is a natural object-oriented programming language. In Python, all data types can be regarded as objects. Custom object data type is the concept of Class in object-oriented.
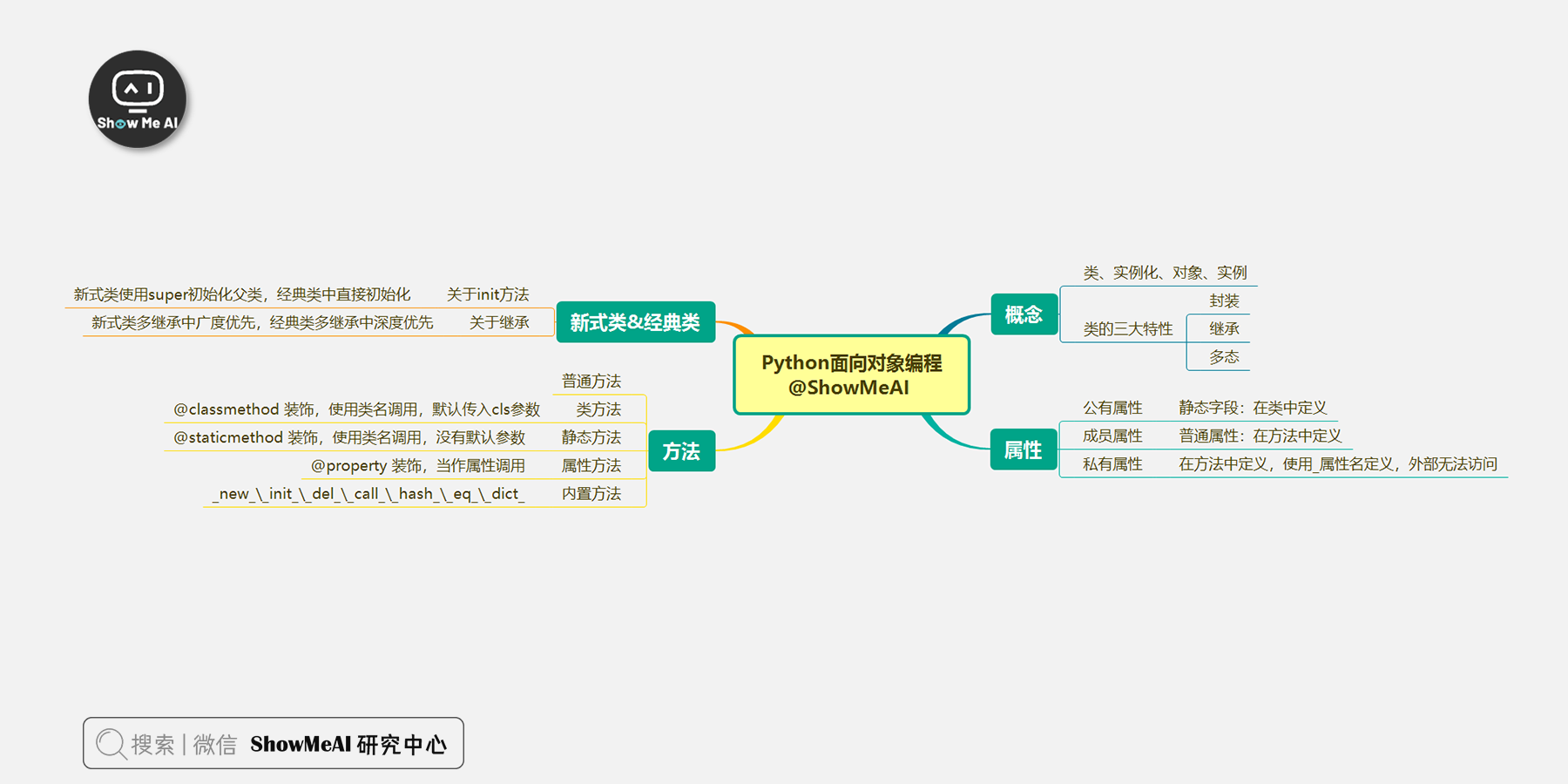
2. Object oriented concept
- Class: used to describe a collection of objects with the same properties and methods. It defines the properties and methods common to each object in the collection. An object is an instance of a class.
- Method: a function defined in a class.
- Class variables: class variables are common to the entire instantiated object. Class variables are defined in the class and outside the function body. Class variables are usually not used as instance variables.
- Data members: class variables or instance variables are used to process data related to classes and their instance objects.
- Method Rewriting: if the method inherited from the parent class cannot meet the needs of the child class, it can be rewritten. This process is called method override, also known as method rewriting.
- Local variable: the variable defined in the method, which only works on the class of the current instance.
- Instance variable: in the declaration of a class, attributes are represented by variables, which are called instance variables. Instance variables are variables decorated with self.
- Inheritance: that is, a derived class inherits the fields and methods of the base class. Inheritance also allows the object of a derived class to be treated as a base class object. For example, there is such a design: an object of Dog type is derived from the Animal class, which simulates the "is-a" relationship (for example, Dog is an Animal).
- Instantiation: create an instance of a class and the specific object of the class.
- Object: an instance of a data structure defined by a class. The object includes two data members (class variables and instance variables) and methods.
Compared with other programming languages, Python's class mechanism is very simple. Classes in Python provide all the basic functions of object-oriented programming
- The inheritance mechanism of class allows multiple base classes
- A derived class can override any method in the base class
- Method can call a method with the same name in the base class
Objects can contain any number and type of data.
3. Class definition
The syntax format is as follows:
class ClassName: <statement-1> . . . <statement-N>
After a class is instantiated, its properties can be used. In fact, after a class is created, its properties can be accessed through the class name.
4. Class object
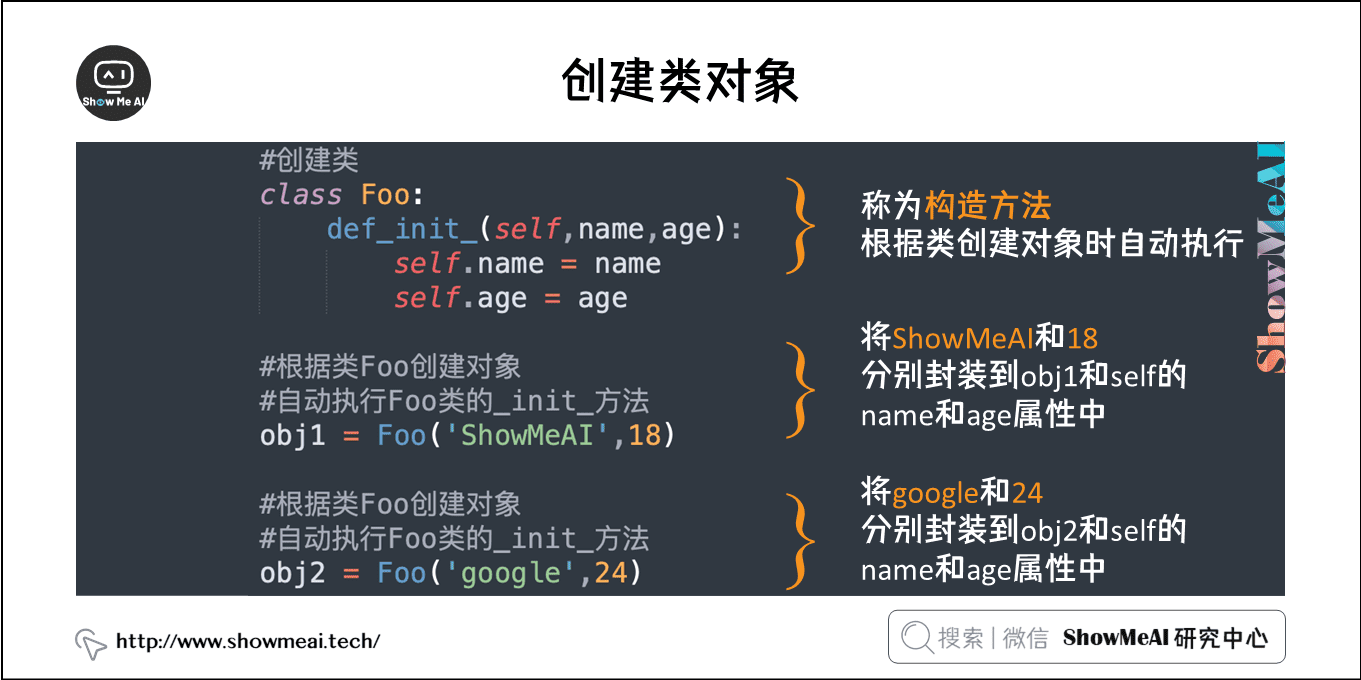
(1) Attribute reference and instantiation
Class objects support two operations: attribute reference and instantiation.
Attribute references use the same standard syntax as all attribute references in Python: obj name.
After a class object is created, all names in the class namespace are valid attribute names. So if the class definition is like this:
class NewClass: """A simple class instance""" num = 123456 def f(self): return 'hello ShowMeAI' # Instantiation class x = NewClass() # Access the properties and methods of the class print("NewClass Properties of class num For:", x.num) print("NewClass Class method f Output is:", x.f())
The above creates a new class instance and assigns the object to the object whose local variable x is empty.
After executing the above procedure, the output result is:
NewClass Properties of class num For: 123456 NewClass Class method f Output is: hello ShowMeAI
(2) Constructor
Class has a name__ init__ (), which will be called automatically when the class is instantiated, as follows:
def __init__(self): self.data = []
Class defines__ init__ () method, and the instantiation operation of the class will be called automatically__ init__ () method. Instantiate the class NewClass as follows, corresponding to__ init__ () method will be called:
x = NewClass()
Of course__ init__ () the method can have parameters, which can be passed__ init__ () passed to the instantiation operation of the class. For example (the code can be in Online Python 3 environment Running in:
class MyComplex: def __init__(self, real_part, imag_part): self.r = real_part self.i = imag_part x = MyComplex(5.0, -3.4) print(x.r, x.i) # Output result: 5.0 - 3.4
(3) self represents an instance of a class, not a class
Class methods differ only in one way from ordinary functions -- they must have an additional first parameter name, which is conventionally called self.
class Test: def prt(self): print(self) print(self.__class__) t = Test() t.prt()
The execution result of the above example is:
<__main__.Test instance at 0x100724179> __main__.Test
Through the execution results, we can see that self represents the instance of the class (including the address information of the current object), and self Class points to class.
Note that self here is not a python keyword. It can be executed normally by changing it to another name, such as showmeai (the code can be in Online Python 3 environment Running in:
class Test: def prt(showmeai): print(showmeai) print(showmeai.__class__) t = Test() t.prt()
The execution result of the above example is:
<__main__.Test instance at 0x100724179> __main__.Test
5. Method of class
Inside the class, we can use the def keyword to define the class method. The class method must contain the parameter self, which is the first parameter. Self represents the instance of the class. For example (the code can be in Online Python 3 environment (running in)
class Person: #Define basic properties name = '' age = 0 #Define private attributes, which cannot be accessed directly outside the class __weight = 0 #Define construction method def __init__(self,n,a,w): self.name = n self.age = a self.__weight = w def talk(self): print("%s What is your age %d Years old." %(self.name,self.age)) # Instantiation class p = Person('ShowMeAI',30,30) p.talk()
After executing the above procedure, the output result is:
ShowMeAI My age is 30.
6. Succession
Python also supports class inheritance. The definition of derived class is as follows:
class DerivedClass(BaseClass): <statement-1> . . . <statement-N>
Subclasses (derived class / DerivedClass) inherit the properties and methods of the parent class (base class / BaseClass).
BaseClassName (the base class name in the instance) must be defined in the same scope as the derived class. In addition to classes, expressions can also be used. This is very useful when the base class is defined in another module:
class DerivedClassName(modname.BaseClassName):
#Class definition class person: #Define basic properties name = '' age = 0 #Define private attributes, which cannot be accessed directly outside the class __weight = 0 #Define construction method def __init__(self,n,a,w): self.name = n self.age = a self.__weight = w def talk(self): print("%s What is your age %d Years old." %(self.name,self.age)) #Single inheritance example class student(person): grade = '' def __init__(self,n,a,w,g): #Call the constructor of the parent class people.__init__(self,n,a,w) self.grade = g #Override method of parent class def talk(self): print("%s What is your age %d Years old, currently reading %d grade"%(self.name,self.age,self.grade)) s = student('Small Show',12,60,5) s.talk()
After executing the above procedure, the output result is:
Small Show My age is 12 years old and I am currently in Grade 5
7. Multiple inheritance
Python also supports multiple inheritance forms. The class definition of multiple inheritance is as follows:
class DerivedClassName(Base1, Base2, Base3): <statement-1> . . . <statement-N>
Note the order of parent classes in parentheses. If the parent class has the same method name and is not specified when the child class is used, python searches from left to right, that is, if the method is not found in the child class, it searches from left to right whether the parent class contains the method.
#Class definition class person: #Define basic properties name = '' age = 0 #Define private attributes, which cannot be accessed directly outside the class __weight = 0 #Define construction method def __init__(self,n,a,w): self.name = n self.age = a self.__weight = w def speak(self): print("%s What is your age %d Years old." %(self.name,self.age)) #Single inheritance example class student(person): grade = '' def __init__(self,n,a,w,g): #Call the constructor of the parent class people.__init__(self,n,a,w) self.grade = g #Override method of parent class def talk(self): print("%s What is your age %d Years old, currently reading %d grade"%(self.name,self.age,self.grade)) #Another class, preparation before multiple inheritance class speaker(): topic = '' name = '' def __init__(self,n,t): self.name = n self.topic = t def talk(self): print("%s I'm a speaker today ta The theme of the speech is %s"%(self.name,self.topic)) #multiple inheritance class sample(speaker,student): a ='' def __init__(self,n,a,w,g,t): student.__init__(self,n,a,w,g) speaker.__init__(self,n,t) test = sample("ShowMeAI",25,80,4,"Python") test.talk() #The method name is the same. By default, it calls the method of the parent class listed in parentheses
After executing the above procedure, the output result is:
ShowMeAI I'm a speaker today ta The theme of the speech is Python
8. Method rewriting
If the function of your parent class method cannot meet your needs, you can override the method of your parent class in the subclass. Examples are as follows:
class Parent: # Define parent class def my_method(self): print ('Call parent method') class Child(Parent): # Define subclasses def my_method(self): print ('Call subclass method') c = Child() # Subclass instance c.my_method() # Subclass call override method super(Child,c).my_method() #Call a method whose parent class has been overridden with a subclass object
The super() function is a method used to call the parent class (superclass).
After executing the above procedure, the output result is:
Call subclass method Call parent method
9. Class attributes and methods
(1) Private properties of class
__ private_attrs: it starts with two underscores and is declared as a private attribute. It cannot be used or accessed directly outside the class. It can be used in the methods inside the class, and the use method is self__ private_attrs.
(2) Class method
The member method defined inside the class must contain the parameter self, which is the first parameter. Self represents the instance of the class.
The name of self is not specified. You can also use this, but it is recommended to use self as agreed.
(3) Private method of class
__ private_method: it starts with two underscores and is declared as a private method. It can only be called inside the class. The method used is self__ private_ methods.
The example code of private attribute of class is as follows:
class NewCounter: __secret_count = 0 # private variable public_count = 0 # Public variable def count(self): self.__secret_count += 1 self.public_count += 1 print (self.__secret_count) counter = NewCounter() counter.count() counter.count() print (counter.public_count) print (counter.__secret_count) # An error is reported. The instance cannot access private variables
After executing the above procedure, the output result is:
1 2 2 Traceback (most recent call last): File "test.py", line 16, in <module> print (counter.__secret_count) # An error is reported. The instance cannot access private variables AttributeError: 'NewCounter' object has no attribute '__secret_count'
Examples of private methods of class are as follows:
class WebSite: def __init__(self, name, url): self.name = name # public self.__url = url # private def who(self): print('name : ', self.name) print('url : ', self.__url) def __foo(self): # Private method print('This is a private method') def foo(self): # Public method print('This is a public method') self.__foo() x = WebSite('ShowMeAI Knowledge community', 'www.showmeai.tech') x.who() # Normal output x.foo() # Normal output x.__foo() # report errors
(4) Class specific methods:
- __ init__: Constructor, called when the object is generated
- __ del__: Destructor, used when releasing an object
- __ repr__: Print, convert
- __ setitem__: Assignment by index
- __ getitem__: Get value by index
- __ len__: Get length
- __ cmp__: Comparison operation
- __ call__: function call
- __ add__: Addition operation
- __ sub__: Subtraction operation
- __ mul__: Multiplication operation
- __ truediv__: Division operation
- __ mod__: Remainder operation
- __ pow__: Power
(5) Operator overloading
Python also supports operator overloading. We can overload the proprietary methods of classes. Examples are as follows:
class MyVector: def __init__(self, a, b): self.a = a self.b = b def __str__(self): return 'Vector (%d, %d)' % (self.a, self.b) def __add__(self,other): return Vector(self.a + other.a, self.b + other.b) v1 = MyVector(2,10) v2 = MyVector(5,-2) print(v1 + v2)
The execution results of the above code are as follows:
Vector(7,8)
10. Video tutorial
Please click to station B to view the version of [bilingual subtitles]
https://www.bilibili.com/video/BV1yg411c7Nw
Data and code download
The code for this tutorial series can be found in github corresponding to ShowMeAI Download in, you can run in the local python environment. Babies who can visit foreign websites can also directly use Google lab to run and learn through interactive operation!
The Python quick look-up table involved in this tutorial series can be downloaded and obtained at the following address: