This article has included the series of Java common interview questions. Gitee open source address: https://gitee.com/mydb/interview
List de duplication refers to the process of deleting duplicate elements in a list. This topic examines the ability to understand and flexibly use the new features in list iterator, Set set and JDK 8.
There are three implementation ideas for List de duplication:
- The user-defined method de duplication determines whether there are multiple current elements by cycling. If there are multiple elements, delete the duplicate item. The final result of cycling the whole collection is a List without duplicate elements;
- Use Set set to remove duplicate, and use the feature of Set set's own de duplication function to realize the de duplication of List;
- Use the de duplication function of Stream stream in JDK 8.
1. Custom de duplication
There are two ways to implement custom de duplication. First, we can create a new set and judge whether the elements of the cycle already exist in the new set by cycling the original set. If they do not exist, they will be inserted, otherwise they will be ignored. After the cycle is completed, the final new set is a set without duplicate elements. The specific implementation code is as follows:
import lombok.Data; import java.util.ArrayList; import java.util.List; public class DistinctExample { public static void main(String[] args) { // Create and assign a value to the List List<Person> list = new ArrayList<>(); list.add(new Person("Li Si", "123456", 20)); list.add(new Person("Zhang San", "123456", 18)); list.add(new Person("Wang Wu", "123456", 22)); list.add(new Person("Zhang San", "123456", 18)); // De duplication operation List<Person> newList = new ArrayList<>(list.size()); list.forEach(i -> { if (!newList.contains(i)) { // Insert if it does not exist in the new collection newList.add(i); } }); // Print set newList.forEach(p -> System.out.println(p)); } } @Data class Person { private String name; private String password; private int age; public Person(String name, String password, int age) { this.name = name; this.password = password; this.age = age; } }
The results of the above procedures are shown in the figure below:
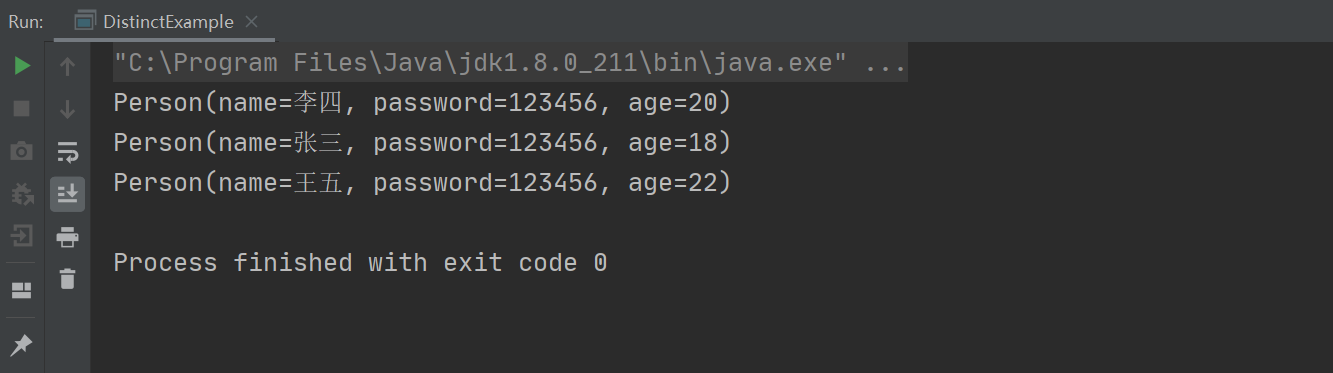
Implementation method 2 of user-defined de duplication function: use iterator loop to judge whether the first occurrence position (indexOf) of the current element is equal to the last occurrence position (lastIndexOf). If not, it indicates that the element is a duplicate element, and delete the current element. In this way, a set without duplicate elements can be obtained after the cycle. The implementation code is as follows:
import lombok.Data; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class DistinctExample { public static void main(String[] args) { // Create and assign a value to the List List<Person> list = new ArrayList<>(); list.add(new Person("Li Si", "123456", 20)); list.add(new Person("Zhang San", "123456", 18)); list.add(new Person("Wang Wu", "123456", 22)); list.add(new Person("Zhang San", "123456", 18)); // De duplication operation Iterator<Person> iterator = list.iterator(); while (iterator.hasNext()) { // Gets the value of the loop Person item = iterator.next(); // If two identical values exist if (list.indexOf(item) != list.lastIndexOf(item)) { // Remove the same value iterator.remove(); } } // Print collection information list.forEach(p -> System.out.println(p)); } } @Data class Person { private String name; private String password; private int age; public Person(String name, String password, int age) { this.name = name; this.password = password; this.age = age; } }
The results of the above procedures are shown in the figure below:
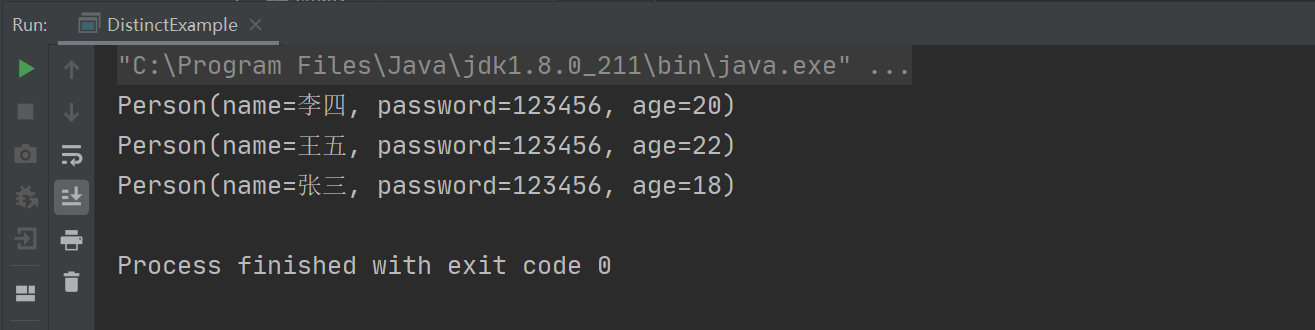
2. Use Set to remove duplication
The Set set is inherently de duplicated. When creating the Set set, a List Set can be passed, so that the functions of data transfer and de duplication can be realized. The specific implementation code is as follows:
import lombok.Data; import java.util.ArrayList; import java.util.HashSet; import java.util.List; public class DistinctExample { public static void main(String[] args) { // Create and assign a value to the List List<Person> list = new ArrayList<>(); list.add(new Person("Li Si", "123456", 20)); list.add(new Person("Zhang San", "123456", 18)); list.add(new Person("Wang Wu", "123456", 22)); list.add(new Person("Zhang San", "123456", 18)); // De duplication operation HashSet<Person> set = new HashSet<>(list); // Print collection information set.forEach(p -> System.out.println(p)); } } @Data class Person { private String name; private String password; private int age; public Person(String name, String password, int age) { this.name = name; this.password = password; this.age = age; } }
The results of the above procedures are shown in the figure below:
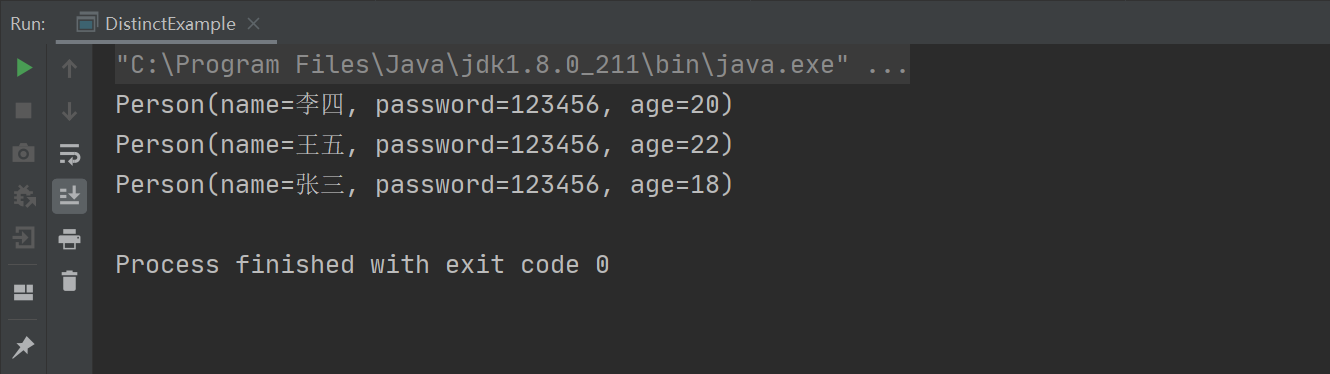
Through the above results, we found a problem. After using HashSet de duplication, the order of elements has changed. To solve this problem, we can use LinkedHashSet to implement the de duplication function. The specific implementation code is as follows:
import lombok.Data; import java.util.ArrayList; import java.util.LinkedHashSet; import java.util.List; public class DistinctExample { public static void main(String[] args) { // Create and assign a value to the List List<Person> list = new ArrayList<>(); list.add(new Person("Li Si", "123456", 20)); list.add(new Person("Zhang San", "123456", 18)); list.add(new Person("Wang Wu", "123456", 22)); list.add(new Person("Zhang San", "123456", 18)); // De duplication operation LinkedHashSet<Person> set = new LinkedHashSet<>(list); // Print collection information set.forEach(p -> System.out.println(p)); } } @Data class Person { private String name; private String password; private int age; public Person(String name, String password, int age) { this.name = name; this.password = password; this.age = age; } }
The results of the above procedures are shown in the figure below:
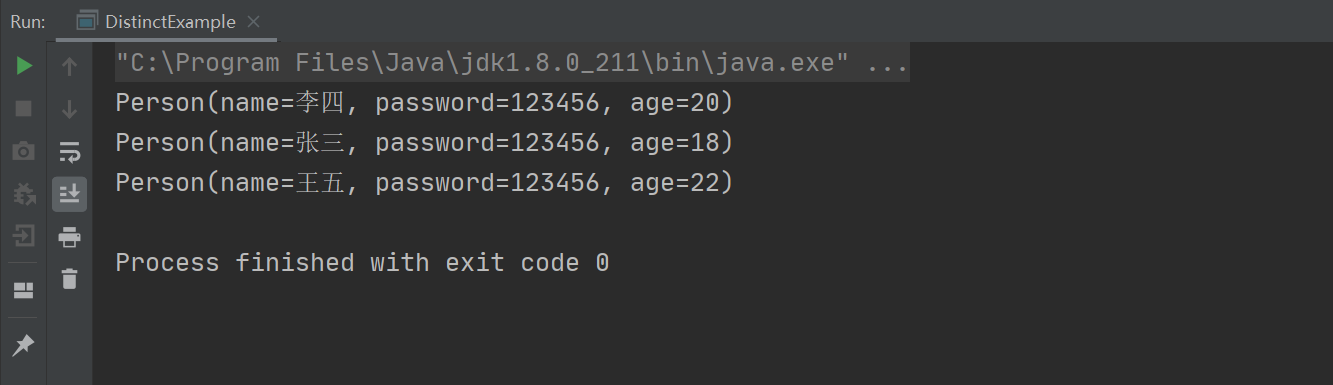
3. Use Stream for weight removal
The last and simplest way of de duplication is to use the Stream provided in JDK 8. The Stream contains a de duplication method: distinct, which can directly realize the de duplication function of the collection. The specific implementation code is as follows:
import lombok.Data; import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class DistinctExample { public static void main(String[] args) { // Create and assign a value to the List List<Person> list = new ArrayList<>(); list.add(new Person("Li Si", "123456", 20)); list.add(new Person("Zhang San", "123456", 18)); list.add(new Person("Wang Wu", "123456", 22)); list.add(new Person("Zhang San", "123456", 18)); // De duplication operation list = list.stream().distinct().collect(Collectors.toList()); // Print collection information list.forEach(p -> System.out.println(p)); } } @Data class Person { private String name; private String password; private int age; public Person(String name, String password, int age) { this.name = name; this.password = password; this.age = age; } }
The results of the above procedures are shown in the figure below:
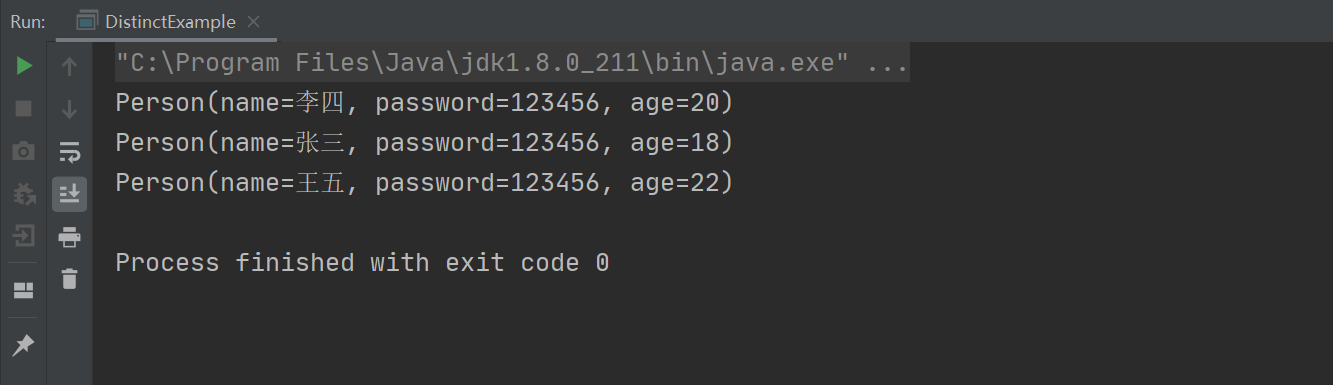
summary
This paper introduces three implementation ideas of List Set de duplication, in which the implementation of custom de duplication function is relatively cumbersome, while Set set can easily realize the de duplication function by relying on its own de duplication characteristics, and LinkedHashSet can be used to remove the duplication while ensuring that the location of elements is not changed. The last de duplication method is added in JDK 8. It uses the distinct method in Stream to realize de duplication. Its advantage is that it is not only simple to write, but also does not need to create a new Set. It is the preferred method to realize the de duplication function.
Right and wrong are judged by ourselves, bad reputation is heard by others, and the number of gains and losses is safe. Blogger introduction: post-80s programmers have "insisted" on blogging for 12 years. Hobbies: reading, jogging and badminton.