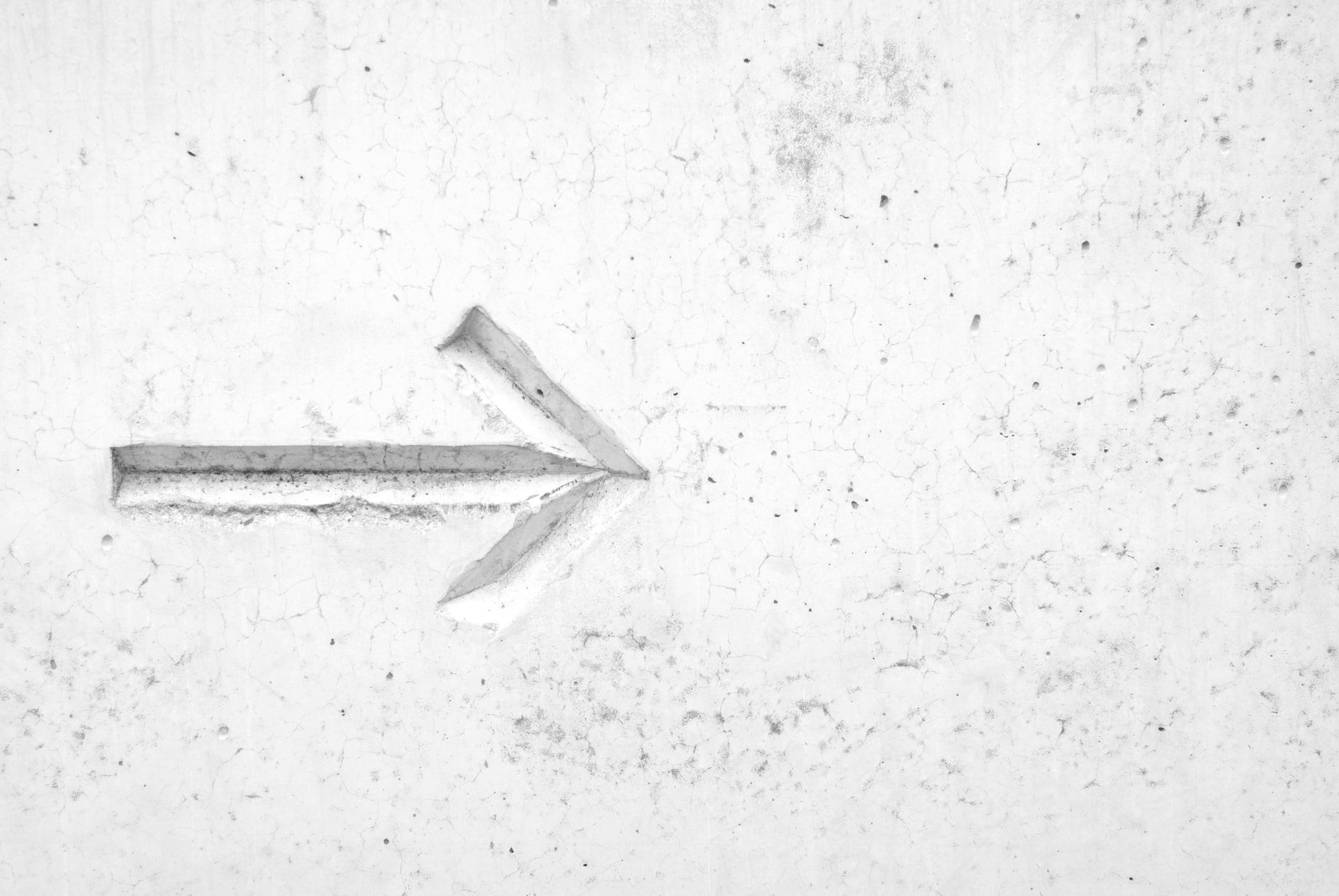
In the last article, FinClip Our engineers and we mainly compared FinClip with mPaaS and Unisdk. In this article, we will talk about how to introduce FinClip SDK into our own apps.
iOS terminal
It is very simple to introduce FinClip SDK into iOS project. Please refer to the following steps
Step 1: get SDK KEY and SDK SECRET
To use the SDK, you need to apply for SDK KEY and SDK SECRET. Only when you configure the correct SDK KEY and SDK SECRET during SDK initialization can you initialize successfully and use it normally.
Create application
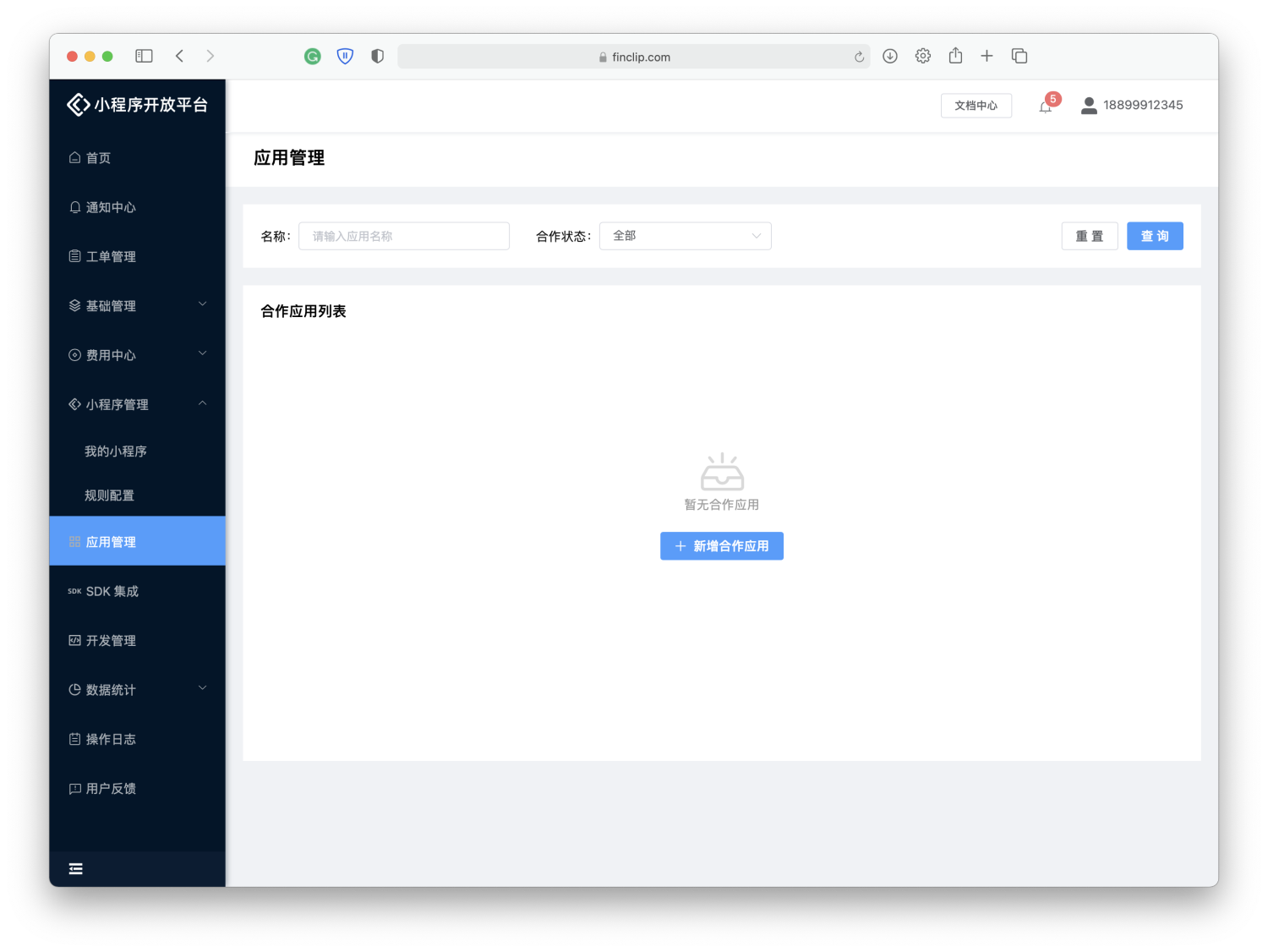
Get SDK KEY and SDK SECRET
After creating an application and adding a Bundle ID, if you need to export the corresponding SDK KEY and SDK SECRET, please select copy after the corresponding Bundle ID to paste through ctrl+v or command+v:
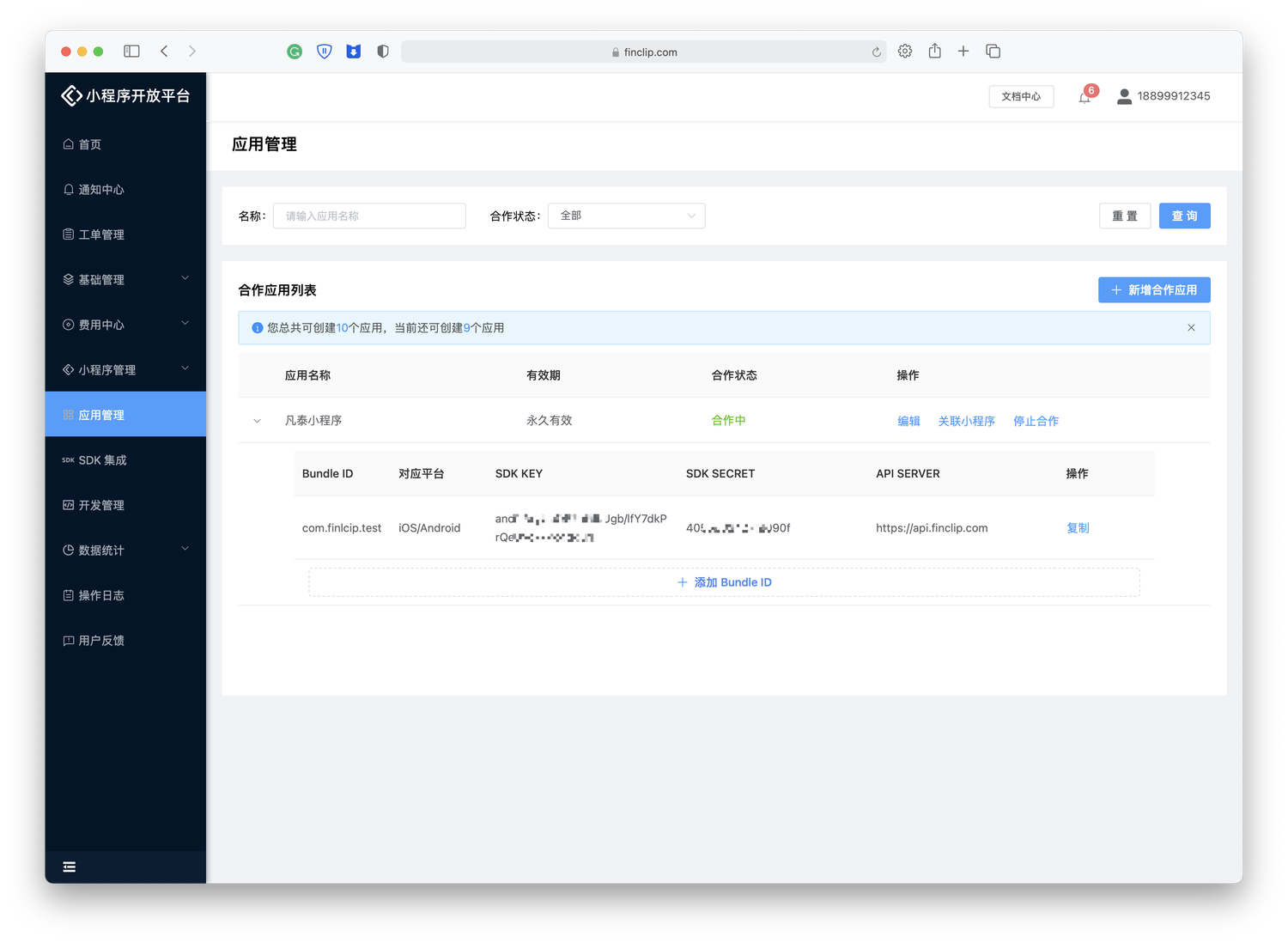
Please note that:
- SDK Key: the certificate that the cooperative application can use the applet SDK. If the SDK Key verification fails, all APIs of the SDK cannot be used.
- SDK secret: it is the security certificate for accessing the service and should not be given to a third party.
For details on creating applications and obtaining SDK keys and SDK SECRET, see "Introduction - operation guidelines - enterprise operation guidelines - 7. Associated mobile applications" A section.
Step 2: integrate SDK
This step has been introduced in the previous article for reference How does iOS introduce an SDK This article, to introduce FinApplet.framework and FinAppletExt.framework.
Step 3: add SDK header file
Where the FinClip applet SDK needs to be used, add the following code:
#import <FinApplet/FinApplet.h>
If the extension SDK is also integrated, you need to add the following code to call the api in the extension SDK:
#import <FinAppletExt/FinAppletExt.h>
Of course, the most convenient way is to add the above code to the pch file, so that there is no need to refer to it where it is used.
Step 4: initialize SDK
FATConfig *config = [FATConfig configWithAppSecret:@"SDK KEY" appKey:@"SDK SECRET"]; config.apiServer = @"https://www.finclip.com"; [[FATClient sharedClient] initWithConfig:config error:nil];
Step 5: open the applet
[[FATClient sharedClient] startRemoteApplet:@"app id" startParams:nil InParentViewController:self completion:^(BOOL result, NSError *error) { NSLog(@"result:%d---error:%@", result, error); }];
If you want to get the sample DEMO of all projects, you can click below to download.
Android terminal
We Android developers should all know the slogan of Java, write once, run anywhere, and the applet platform can write once, run any miniprogram. The applet container platform can carry tens of millions of kinds of applets. Today, let's study how to make our App a container and run all kinds of applets. Here, this function is completed with the help of FinClip platform.
Android FinClip SDK integration
Before integrating the SDK, you need to apply for the SDK Key and Secret from the platform, and upload your applet code package to the platform to obtain the applet appID. The client needs to drive the applet through this information. Just refer to the above documents for operation, which will not be repeated here.
Before FinClip SDK integration, you need to get the following information
- SDK Key
- SDK Secret
- apiURL / / the url of the applet platform
- appId / / appId of the applet
SDK integration mainly consists of the following steps
- Get the latest SDK version
- Add dependency
- Modify the obfuscation configuration and add the obfuscation configuration of FinClip
- Initialize FinClip SDK
- Start applet
Step 1: add dependency
In the build of root project Add Maven warehouse in gradle file
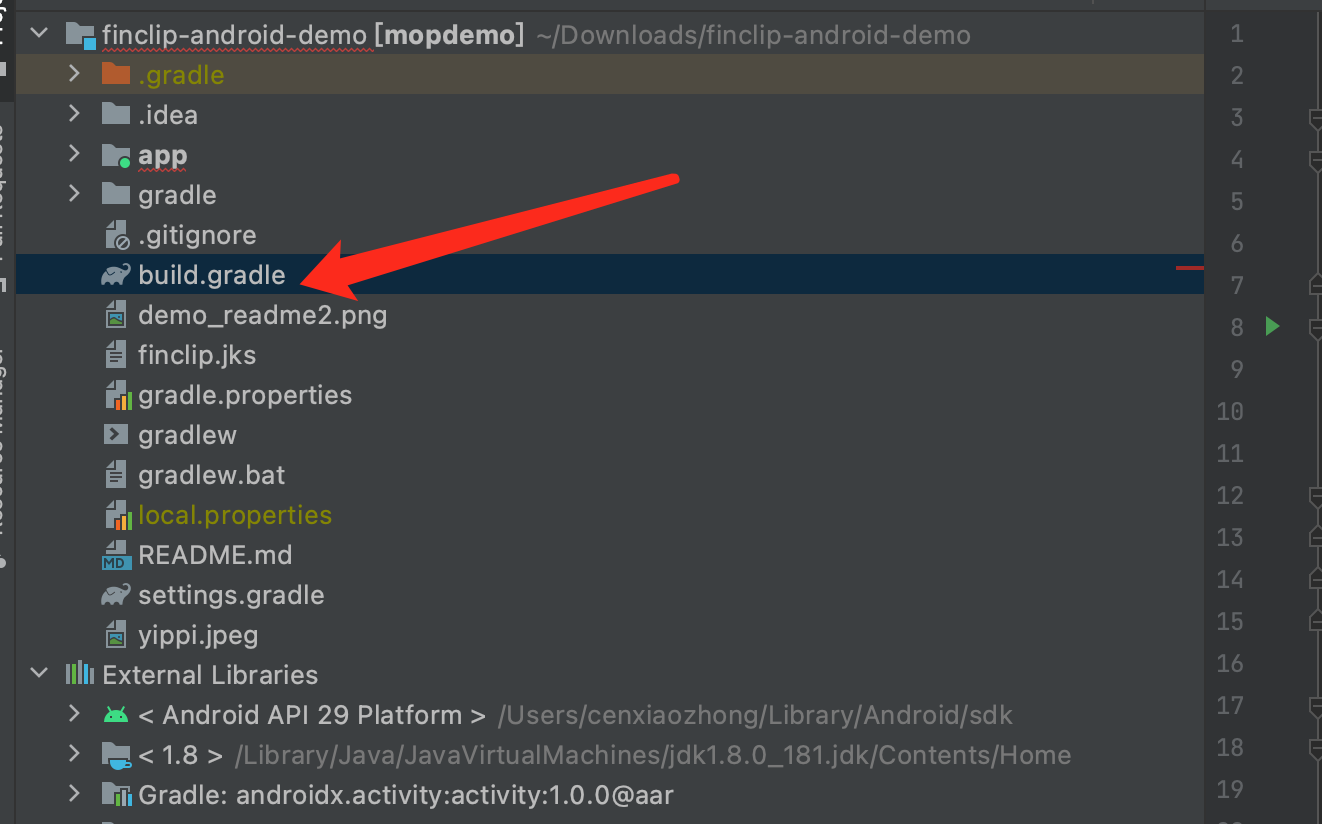
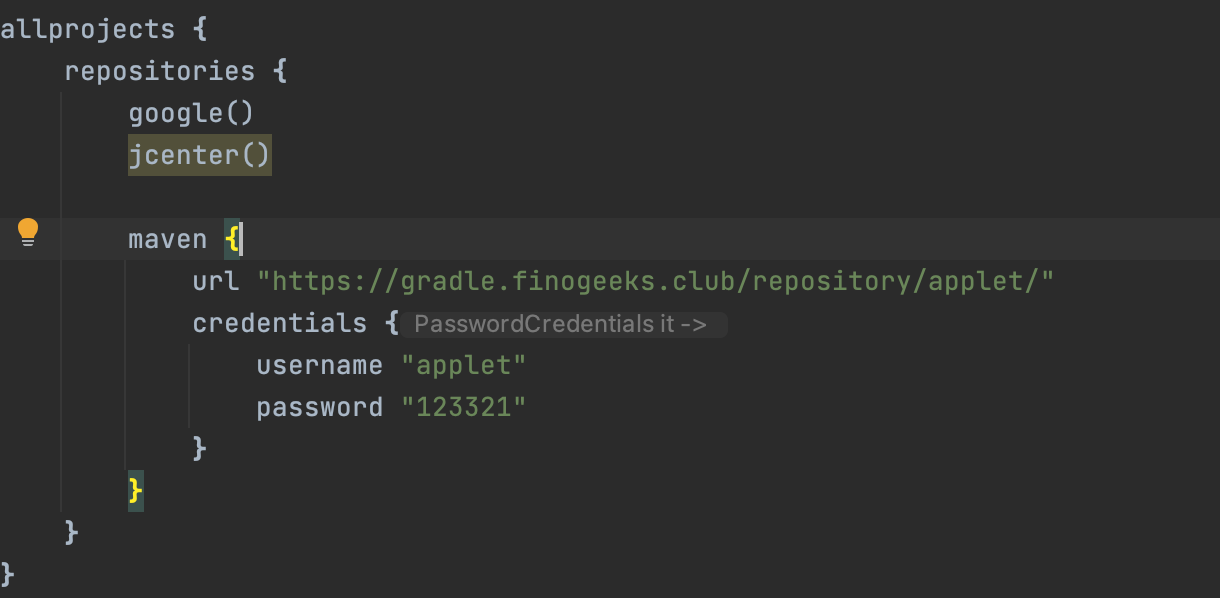
maven { url "https://gradle.finogeeks.club/repository/applet/" credentials { username "applet" password "123321" } }
Then, in app module build Add dependency to gradle file
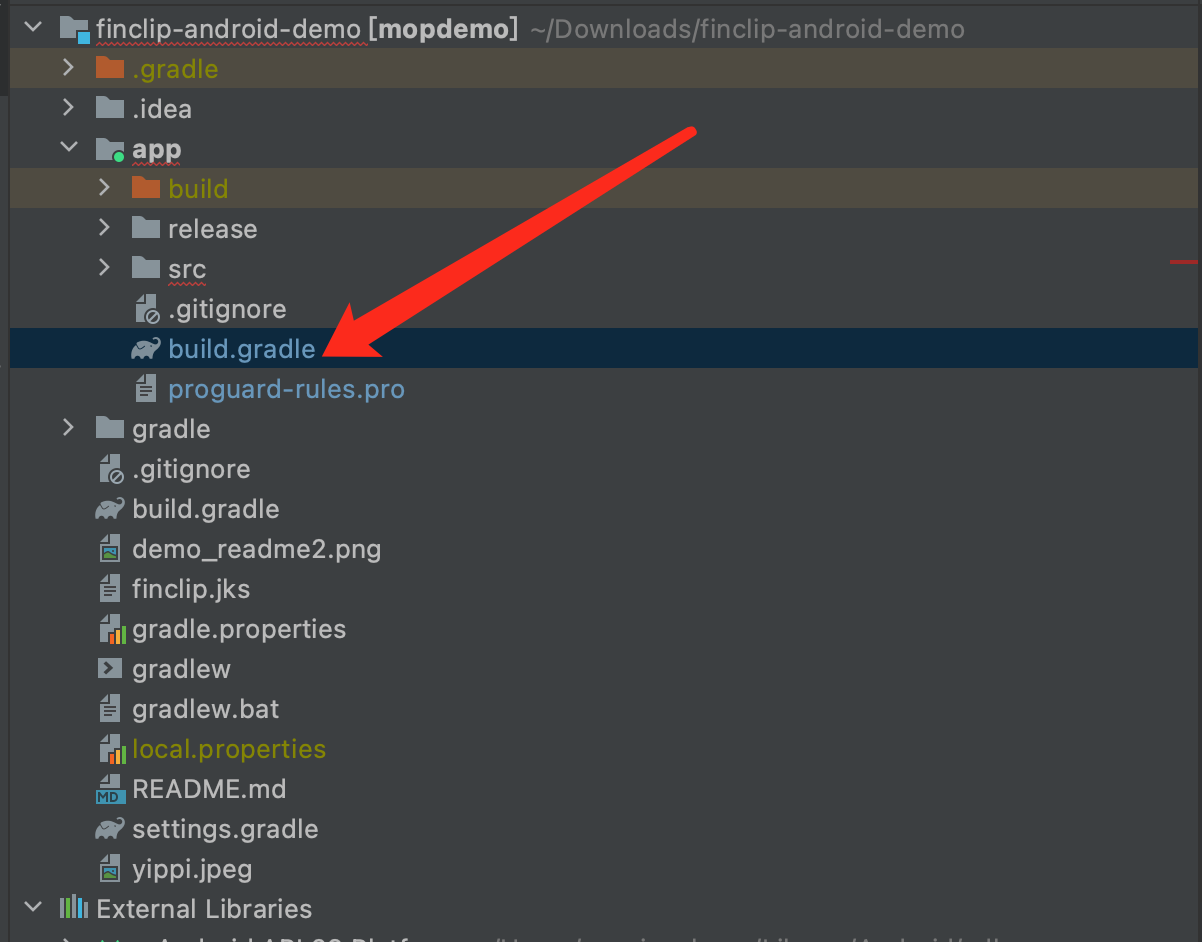
implementation 'com.finogeeks.lib:finapplet:2.34.5'
Step 2: modify the obfuscation configuration and add the obfuscation configuration of FinClip
In the Proguard rules Add confusion configuration in pro file
-keep class com.finogeeks.** {*;}
Step 3: initialize FinClip SDK
It is recommended to initialize the FinClip SDK in the Application#onCreate method, as shown in the following app_ KEY,APP_ Fields such as secret are in build Dynamically generated by gradle configuration compilation
- BuildConfig.APP_KEY / / the key of Finclip SDK is obtained on the platform
- BuildConfig. APP_ Secret / / the secret of the finclip SDK is obtained on the platform
- FinAppConfig.ENCRYPTION_TYPE_SM / / encryption type
- BuildConfig.API_URL / / if it is not a privatized deployment, it is filled in by default https://mp.finogeeks.com This will do
FinAppConfig config = new FinAppConfig.Builder() .setSdkKey(BuildConfig.APP_KEY) .setSdkSecret(BuildConfig.APP_SECRET) .setApiUrl(BuildConfig.API_URL) .setApiPrefix(BuildConfig.API_PREFIX) .setDebugMode(BuildConfig.DEBUG) .setEncryptionType(FinAppConfig.ENCRYPTION_TYPE_SM) .build(); FinAppClient.INSTANCE.init(this, config, new FinCallback<Object>() { @Override public void onSuccess(Object result) { Toast.makeText(MopApplication.this, "SDK Initialization succeeded", Toast.LENGTH_SHORT).show(); // Register custom applet API FinAppClient.INSTANCE.getExtensionApiManager().registerApi(new CustomApi(MopApplication.this)); // Register custom H5 API FinAppClient.INSTANCE.getExtensionWebApiManager().registerApi(new CustomH5Api(MopApplication.this)); // Set IAppletHandler implementation class FinAppClient.INSTANCE.setAppletHandler(new AppletHandler(getApplicationContext())); // Set the processing method of "applet process calls main process" in the main process // Developers can also choose to set processing methods in other appropriate code locations of the main process FinAppClient.INSTANCE.getAppletApiManager() .setAppletProcessCallHandler(new IAppletApiManager.AppletProcessCallHandler() { @Override public void onAppletProcessCall(@NotNull String name, @Nullable String params, @Nullable FinCallback<String> callback) { if (callback != null) { if (name.equals(LoginApi.API_NAME_LOGIN)) { // Obtain the login information from the main process and return it to the applet process // The virtual user login information is returned here. Developers should obtain the user login information from the APP JSONObject jsonObject = new JSONObject(); try { jsonObject.put("userId", "123"); } catch (JSONException e) { e.printStackTrace(); } callback.onSuccess(jsonObject.toString()); } } } }); } @Override public void onError(int code, String error) { Toast.makeText(MopApplication.this, "SDK initialization failed", Toast.LENGTH_SHORT).show(); } @Override public void onProgress(int status, String error) { } });
defaultConfig { applicationId "com.finogeeks.finclip.demo" minSdkVersion 19 targetSdkVersion 29 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" multiDexEnabled true buildConfigField "String", "APP_KEY", "\"22LyZEib0gLTQdU3MUauATBwgfnTCJjdr7FCnywmAEM=\"" // App Secret buildConfigField "String", "APP_SECRET", "\"bdfd76cae24d4313\"" // API service address buildConfigField "String", "API_URL", "\"https://mp.finogeeks.com\"" // API service prefix buildConfigField "String", "API_PREFIX", "\"/api/v1/mop/\"" ndk { abiFilters "x86", "armeabi", 'armeabi-v7a', 'arm64-v8a' } }
Step 4: start the applet
Map<String, String> params = new HashMap<>(); params.put("path", "pages/index/index"); String appId = "5fc8934aefb8c600019e9747"; FinAppClient.INSTANCE.getAppletApiManager().startApplet(MainActivity.this, appId, params);
matters needing attention
The applet is a multi process design, and Application#onCreate will initialize many times. It is recommended to add if code to prevent the applet process from initializing your other components.
@Override public void onCreate() { super.onCreate(); if (FinAppClient.INSTANCE.isFinAppProcess(this)) { return; } }
Since part of the code of FinClip SDK is written in C + + and reinforced, it cannot be compressed. The following configuration needs to be added:
packagingOptions { // libsdkcore.so is reinforced and cannot be compressed. Otherwise, an error will be reported when loading the dynamic library doNotStrip "*/x86/libsdkcore.so" doNotStrip "*/x86_64/libsdkcore.so" doNotStrip "*/armeabi/libsdkcore.so" doNotStrip "*/armeabi-v7a/libsdkcore.so" doNotStrip "*/arm64-v8a/libsdkcore.so" }