-
Blog home page Xiao Wu_ Xiao Wu has an idea_ CSDN blog - notes, leetcode,java domain Blogger
-
Welcome to pay attention
give the thumbs-up
Collection and message
-
Heaven rewards diligence. Diligence can make up for weakness. Come on with Xiao Wu
-
Freshmen, the level is limited, please give advice, thank you very much!
-
🍊 Here's a little route for you to refer to JAVA implementation of customer information management system and suggestions for freshman winter vacation students_ Xiao Wu CSDN blog
catalogue
Blog home page: Xiao Wu_ Xiao Wu has an idea_ CSDN blog - notes, leetcode,java domain Blogger
Conversion between basic data type and String
Packaging related interview questions
Unpacking and packing
Video notes:
The basic data type is packaged into an instance of a wrapper class -- boxing
1. Through the constructor of packaging class:
int i=100; Integer t=new Integer(i);
2. Construct wrapper class objects through string parameters:
Float f=new Float("2.22"); Long l=new Long("sa");
Get the basic type variable of packaging in the packaging class object - unpacking
Call the of the wrapper class xxxValue() method:
boolean b=bObj.booleanValue();
JDK1. After 5, it supports automatic packing and unpacking. But the type must match.
Let's look at a piece of code (basic data type -- -- > wrapper class)
public class student { public static void main(String[] args) { int age=20; Integer m=new Integer(age); System.out.println(m.toString()); } }
You can do the same
public class student { public static void main(String[] args) { int age=20; Integer m=new Integer(age); System.out.println(m.toString()); Integer n=new Integer("222"); System.out.println(n.toString()); } }
However, when using the second method, only numbers can be written in parentheses. If a letter A is added after 222, an exception will be reported
It's not that you can't add letters under any circumstances, but it should correspond to its structure!
For example, after adding the following statement
Float k=new Float(12.2f); System.out.println(k); Boolean b=new Boolean("tt22"); System.out.println(b);
Let's look at a section of code (packaging class ----------- > basic data type)
public class student { public static void main(String[] args) { Integer m=new Integer(11); int a=m.intValue(); System.out.println(a); Float f=new Float(13.2); float f1=f.floatValue(); System.out.println(f1); } }
The following is due to jdk5 After version 0, it is convenient for many steps to directly and automatically pack and unpack;
Automatic packing:
public class student { public static void main(String[] args) { int c=1; Integer c1=c; System.out.println(c1); } }
Automatic unpacking:
public class student { public static void main(String[] args) { int c=1; Integer c1=c; System.out.println(c1);//Automatic packing int c2=c1; System.out.println(c2);//Automatic unpacking } }
Conversion between basic data type and String
1. Connection operation
public class student { public static void main(String[] args) { int c=1; String str=c+" s "; System.out.print(str); } }
2. Call valueOf(Xxx) of String
public class student { public static void main(String[] args) { float a1=22.2f; String ss=String.valueOf(a1); System.out.println(ss); } }
However, the processing of converting String to int is somewhat different
public class student { public static void main(String[] args) { String str="122"; //int a=(int)str;// error //Integer a1=(Integer)str;// error int A=Integer.parseInt(str); System.out.println(A); } }
Note: when str is copied, 122 should not be followed by letters
Packaging related interview questions
1. Check whether the output results of the two codes are the same
Object a=true?new Integer(1):new Double(2.0); System.out.println(a);
Object b; if(true) b=new Integer(1); else b=new Double(2.0); System.out.println(b);
The first code requires that the left and right sides must be unified when compiling, and will be upgraded to 1.0 when compiling
There is no such requirement in the form of the second piece of code
2.
Integer i=new Integer(10); Integer j=new Integer(10); System.out.println(i==j);//false is not the same object Integer m=1; Integer n=1; System.out.println(m==n);//True Integer a=128; Integer b=128; System.out.println(a==b);//false
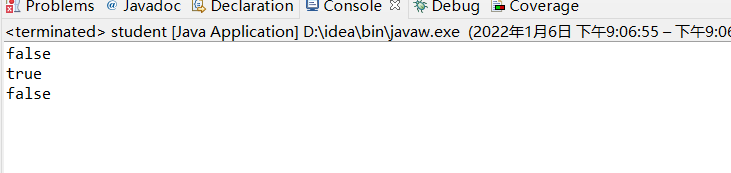
When a=127,b=127
Integer a=127; Integer b=127; System.out.println(a==b);
Here, a and B can omit the construction process and get true only if they meet the requirements of greater than or equal to - 128 and less than or equal to 127
That is, if we use the automatic packing method and assign values to Integer in the range of - 128 to 127, we can directly use the elements in the array without going to new to improve efficiency (refer to the API)
Learning is like sailing against the current. Come on with Xiao Wu!