preface
Simply record the notes about the image operation part of opencv
1, Auxiliary tool Image Watch
Image Watch is a free and powerful image data viewing plug-in. During image viewing, it can display its pixel value and channel number, which is very helpful for viewing results after image operation.
Download address attached
VS2019: Image Watch 2019 - Visual Studio Marketplace
VS2017: Image Watch 2017 - Visual Studio Marketplace
VS2015 and below: Image Watch - Visual Studio Marketplace
Open method: find image watch in View > Other windows and open it. It can be used with breakpoints in debugging.
2, Mat class
Creation method:
Mat a; //Create a matrix header named a a=imread("lena.jpg");
Data types in Opencv:
data type | Specific type | Value range |
---|---|---|
CV_8U | 8-bit unsigned integer | 0~255 |
CV_8S | 8-bit signed integer | -128~127 |
CV_16U | 16 bit unsigned integer | 0~65535 |
CV_16S | 16 bit signed integer | -32768~32767 |
CV_32S | 32-bit signed integer | -2147483648~2147483647 |
CV_32F | 32-bit floating-point integer | |
CV_64F | 64 bit floating point integer |
For channels, C1, C2, C3 and C4 represent single channel, dual channel, 3-channel and 4-channel respectively.
Several construction methods of Mat class:
Mode 1:
Mat(int rows,int cols,int type)
For example, Mat a (640480, CV_8UC3) indicates that a 3-channel matrix of 640 * 480 8-bit unsigned integer is created to store color images
Mode 2:
Mat(Size size(),int type)
For example, Mat b (Size (480640), CV_32FC3) indicates that a 3-channel matrix of 640 * 480 32-bit floating-point integer is created to store color images
Assignment of Mat class:
Mat(int rows,int cols,int type,const Scalar &s)
For example: Mat img1(2, 2, CV_8UC3, Scalar(0, 0, 255))
A three channel matrix is created. The channel value of the third layer is 255 and the first two layers are 0. Start Image Watch to see the effect as follows:
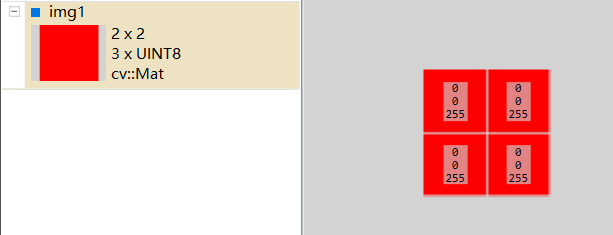
Other methods (lifting method):
Mat a=(Mat_<int>(3,3)<<1,2,3,4,5,6,7,8,9)
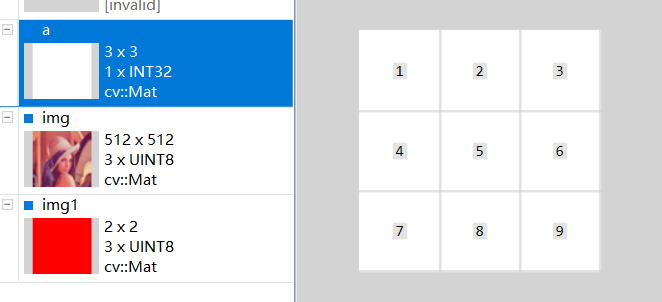
The Mat class can also support operations such as addition, subtraction, multiplication and division
Reading of Mat class elements
Common methods:
Read the elements in the single channel Mat class matrix through the at method
Mat a=(Mat_<uchar>(3,3)<<1,2,3,4,5,6,7,8,9); int value=(int)a.at<uchar>(0,0);
For multi-channel type, the storage method is shown in the figure below
3-channel storage mode:
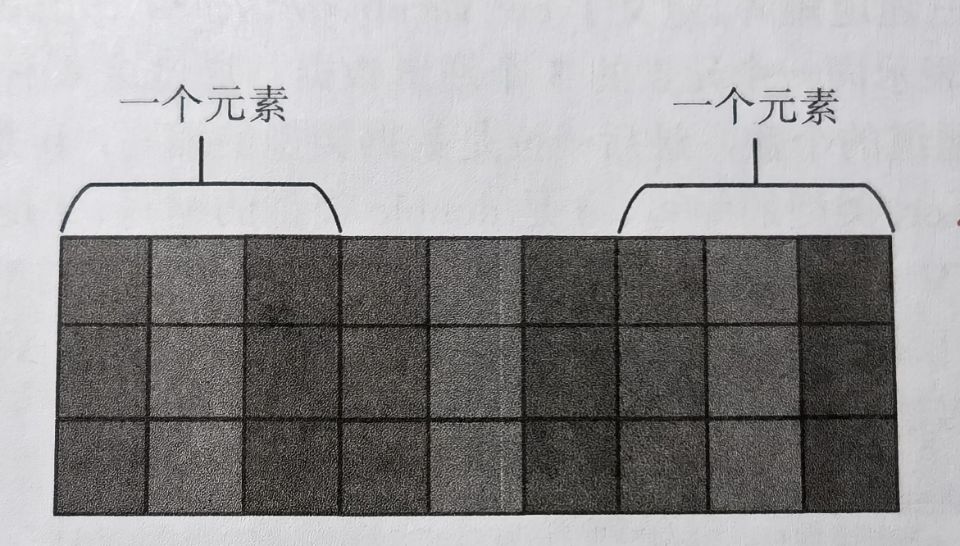
The figure shows the storage mode of a 3 * 3 channel. For a single element, it is stored according to the adjacent of each channel.
Then special data structures are needed to store these Vec3b, Vec3s, Vec3w, Vec3d, Vec3f and Vec3i
Read the elements in the multi-channel Mat class matrix through the at method
Mat b(3,4,CV_8UC3,Scalar(0,0,1)); Vec3b vc3 = b.at<Vec3b>(0, 0); int first = (int)vc3.val[0]; int second = (int)vc3.val[1]; int third = (int)vc3.val[2];
Mandatory conversion cannot be less!
Other methods include pointer ptr and iterator. There is no record here
2, Image manipulation
Image operation is the highlight of opencv, which is introduced from several functions
1.imread() function -- image reading
imread(const String&filename,int flags=IMREAD_COLOR)
The first parameter is the complete address of the image, such as "D:/vs project / opencv4_test/opencv4_test/lena.jpg", and the second parameter is the reading mode, such as IMREAD_GRAYSCALE and IMREAD_COLOR
2.imshow() function -- image display
imshow(const String & winname,InputArray mat)
The first parameter is the window name of the image to be displayed, and the second parameter is the image matrix to be displayed
3.namedWindow() function -- image display window
This function is an image window function, which is used to create a window for the image to be displayed
void namedWindow(const String & winname,int flags=WINDOW_AUTOSIZE)
4.imwrite() function -- image saving
bool imwrite(const String & filename,InputArray img,Const vector<int>& params =vector<int>())
filename: the address and file name where the image is saved
img: matrix variable of Mat class to be saved
params: save picture format attribute setting flag
The imwrite() function uses an example program:
#include<opencv2\opencv. HPP > / / load the header file of OpenCV4 #include<iostream> using namespace std; using namespace cv; //OpenCV namespace void AlphaMat(Mat &mat) { CV_Assert(mat.channels() == 4); for (int i = 0; i < mat.rows; i++) { for(int j=0;j<mat.cols;j++) { Vec4b& bgra = mat.at<Vec4b>(i, j); bgra[0] = UCHAR_MAX; //Blue channel B bgra[1] = saturate_cast<uchar>((float(mat.cols - j)) / ((float)mat.cols)*UCHAR_MAX); //Green channel G bgra[2] = saturate_cast<uchar>((float(mat.cols - i)) / ((float)mat.rows)*UCHAR_MAX); //Red channel R bgra[3] = saturate_cast<uchar>(0.5*(bgra[1] + bgra[2])); //Alpha Channel } } } int main() { Mat mat(480, 640, CV_8UC4); AlphaMat(mat); vector<int> compression_params; compression_params.push_back(IMWRITE_PNG_COMPRESSION); //PNG format image compression flag compression_params.push_back(9); //Set maximum compression quality bool result = imwrite("alpha.png", mat, compression_params); if (!result) { cout << "Preservation PNG Failed to format image" << endl; return -1; } cout << "Saved successfully" << endl; return 0; //Program end }
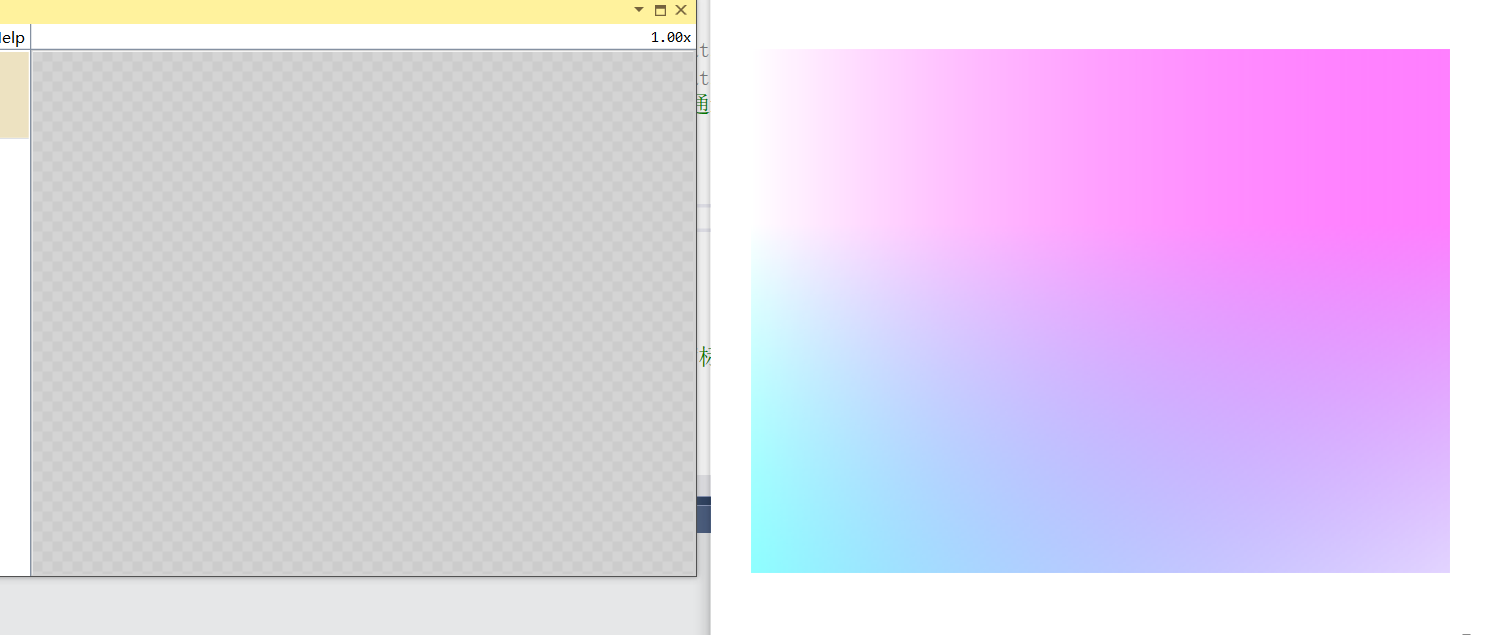
Compared with the images successfully saved after conversion, 4-channel (Alpha channel) images can be saved as PNG format files.
Thanks for reading! You are also welcome to pay attention to Xiaobai blogger and give more encouragement!