The use of customized view is very common in android. Here, we introduce a simple customized view in the container of listview.
This is a functional presentation.
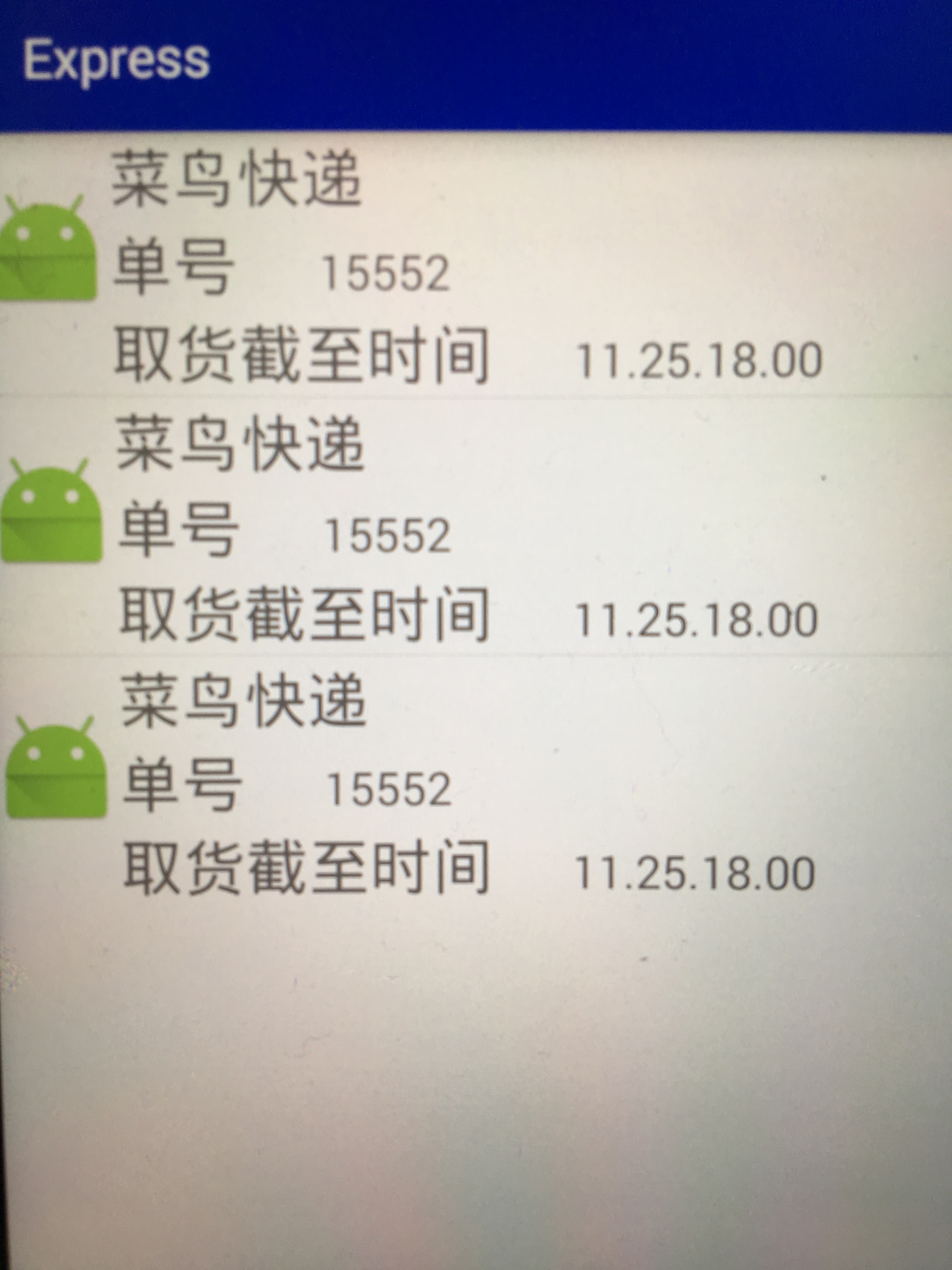
First, you need to create an xml file in the layout folder to determine the composition of each small item, that is, listitem.xml
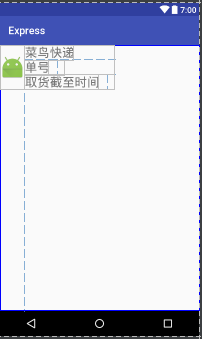
Then, we define a list view to display our small item s (no more details);
Let's start with the adapter. First, it's an adapter that inherits the parent class of baseadpter. It needs to add some functions into the class automatically (see code). The main statements are: 1. Context 2. Container 3. Layout loader 4. Data source. It's important to make full use of historical cache objects to achieve a display optimization; and there's also a viewhold. The optimization method of Er needs attention.
Next, we use the adapter written in the activity file that contains our listview to fill in and display the data.
The above is a simple custom view of the thought process, the specific code see below.
This is part of the adapter code
package com.example.administrator.express.adapter;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.TextView;
import com.example.administrator.express.bean.bean_express;
import com.example.administrator.express.R;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Cerian on 2017/5/4.
*/
public class Adapter_express extends BaseAdapter {
/**
* context
* container
* Layout loader
* data source
*/
private Context context;
private List<bean_express> mylist =new ArrayList<>();
private LayoutInflater inflater;
private bean_express bean;
public Adapter_express(Context context, List<bean_express> mylist){
this.context=context;
this.mylist=mylist;
inflater= (LayoutInflater) context.getSystemService(context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
return mylist.size();
}
@Override
public Object getItem(int position) {
return mylist.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder vHolder=null;
if(convertView==null){
vHolder=new ViewHolder();
convertView=inflater.inflate(R.layout.item_express,null);
vHolder.tv_dateline= (TextView) convertView.findViewById(R.id.tv_datelinelist);
vHolder.tv_ticket= (TextView) convertView.findViewById(R.id.tv_ticketlist);
convertView.setTag(vHolder);
}
else{
vHolder = (ViewHolder) convertView.getTag();
}
bean= (bean_express) getItem(position);
vHolder.tv_ticket.setText(bean.getTicket());
vHolder.tv_dateline.setText(bean.getDataline());
return convertView;
}
class ViewHolder {
private TextView tv_ticket;
private TextView tv_dateline;
}
}
This is part of the data source code.
package com.example.administrator.express.bean;
/**
* Created by Cerian on 2017/5/4.
*/
public class bean_express {
private String ticket;
private String dataline;
public String getTicket() {
return ticket;
}
public void setTicket(String ticket) {
this.ticket = ticket;
}
public String getDataline() {
return dataline;
}
public void setDataline(String dataline) {
this.dataline = dataline;
}
This is the activity part of the code that contains listview
package com.example.administrator.express.activity;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.ListView;
import com.example.administrator.express.adapter.Adapter_express;
import com.example.administrator.express.bean.bean_express;
import com.example.administrator.express.R;
import java.util.ArrayList;
import java.util.List;
public class listview_express extends AppCompatActivity {
private ListView mListView;
private List<bean_express> mlist=new ArrayList<>();
private Adapter_express adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.listexpress);
initview();
}
private void initview() {
mListView = (ListView) findViewById(R.id.mlistview);
bean_express bean = new bean_express();
for(int i =0;i<3;i++) {
bean.setDataline("11.25.18.00");
bean.setTicket("15552");
mlist.add(bean);
}
adapter=new Adapter_express(this,mlist) ;
mListView.setAdapter(adapter);
}
}
In this way, a basic customized view is realized, of course, it is only a very simple customization, such as QQ, Sina News and so on, all evolved from this simple view. We believe that through our efforts, there will be more beautiful and practical customized views in our hands.
If there are any deficiencies in the above contents, I hope to make more corrections. Thank you.