I mainly use the serial port class here. The function is to open the serial port read and write, click the Send Data button to send the data of the sending area to the buffer, and then display it in the receiving area. The interface is as follows: (Source code can be in
Here Download)
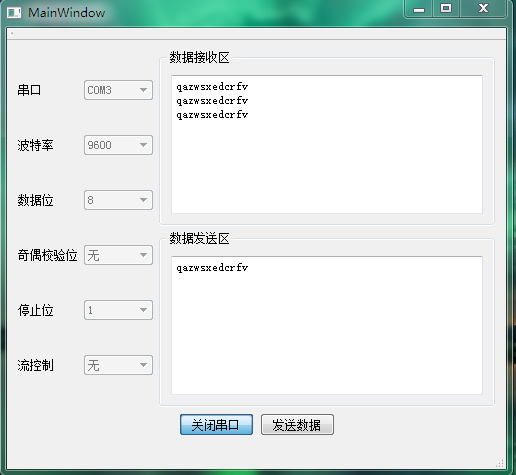
This uses two classes provided by the QSerialPort module: the QSerialPort class and the QSerialPortInfo class, the QSerialPort class provides operations on the serial port, and the QSerialPortInfo class provides access to the serial port information.The following is the main code, which contains a simple use of the serial class.
First, be sure to add in the.pro file: QT += serialport
The serial port is initialized as follows:
-
-
void MainWindow::initPort()
-
{
-
-
foreach (const QSerialPortInfo &info, QSerialPortInfo::availablePorts())
-
{
-
qDebug()<<"Name:"<<info.portName();
-
qDebug()<<"Description:"<<info.description();
-
qDebug()<<"Manufacturer:"<<info.manufacturer();
-
-
-
QSerialPort serial;
-
serial.setPort(info);
-
if(serial.open(QIODevice::ReadWrite))
-
{
-
-
ui->cmbPortName->addItem(info.portName());
-
-
serial.close();
-
}
-
}
-
-
QStringList baudList;
-
QStringList parityList;
-
QStringList dataBitsList;
-
QStringList stopBitsList;
-
-
baudList<<"50"<<"75"<<"100"<<"134"<<"150"<<"200"<<"300"
-
<<"600"<<"1200"<<"1800"<<"2400"<<"4800"<<"9600"
-
<<"14400"<<"19200"<<"38400"<<"56000"<<"57600"
-
<<"76800"<<"115200"<<"128000"<<"256000";
-
-
ui->cmbBaudRate->addItems(baudList);
-
ui->cmbBaudRate->setCurrentIndex(12);
-
-
parityList<<"nothing"<<"odd"<<"even";
-
parityList<<"sign";
-
parityList<<"Spaces";
-
-
ui->cmbParity->addItems(parityList);
-
ui->cmbParity->setCurrentIndex(0);
-
-
dataBitsList<<"5"<<"6"<<"7"<<"8";
-
ui->cmbDataBits->addItems(dataBitsList);
-
ui->cmbDataBits->setCurrentIndex(3);
-
-
stopBitsList<<"1";
-
stopBitsList<<"1.5";
-
stopBitsList<<"2";
-
-
ui->cmbStopBits->addItems(stopBitsList);
-
ui->cmbStopBits->setCurrentIndex(0);
-
-
-
ui->btnOpen->setCheckable(true);
-
}
* Here we add the serial port we need to use to the combox by traversing all the serial ports. If you want to select manually, you can add the serial port name to the combox by listing it, and then select it when you use it.
The serial port settings are as follows:
-
-
void MainWindow::on_btnOpen_clicked()
-
{
-
if(ui->btnOpen->text() == "Open Serial Port")
-
{
-
my_serialport = new QSerialPort(this);
-
-
-
my_serialport->setPortName(ui->cmbPortName->currentText());
-
-
if(my_serialport->open(QIODevice::ReadWrite))
-
{
-
-
my_serialport->setBaudRate(ui->cmbBaudRate->currentText().toInt());
-
-
my_serialport->setDataBits(QSerialPort::Data8);
-
-
my_serialport->setParity(QSerialPort::NoParity);
-
-
my_serialport->setFlowControl(QSerialPort::NoFlowControl);
-
-
my_serialport->setStopBits(QSerialPort::OneStop);
-
-
-
timer = new QTimer(this);
-
connect(timer, SIGNAL(timeout()), this, SLOT(readComDataSlot()));
-
timer->start(1000);
-
-
setNonSelectable();
-
}
-
else
-
{
-
QMessageBox::about(NULL, "Tips", "Serial port not open!");
-
return;
-
}
-
}
-
else
-
{
-
timer->stop();
-
setSelectable();
-
my_serialport->close();
-
}
-
}
This is the setting of the serial port. You need to open the serial port before you can set the parameters of the serial port.Once the parameters are set, you can read and write the data through the read() and write() functions. I use a 1-second timer here to read the data from the buffer.
The data is sent and received as follows:
-
-
void MainWindow::readComDataSlot()
-
{
-
-
QByteArray readComData = my_serialport->readAll();
-
-
-
if(readComData != NULL)
-
{
-
ui->teReceiveData->append(readComData);
-
}
-
-
-
readComData.clear();
-
}
-
-
void MainWindow::on_btnSend_clicked()
-
{
-
-
QString sendData = ui->teSendData->toPlainText();
-
QByteArray sendData_2 = sendData.toLatin1();
-
-
-
my_serialport->write(sendData_2);
-
}